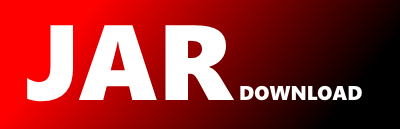
com.atlassian.bamboo.specs.codegen.emitters.value.LiteralEmitter Maven / Gradle / Ivy
package com.atlassian.bamboo.specs.codegen.emitters.value;
import com.atlassian.bamboo.specs.api.codegen.CodeEmitter;
import com.atlassian.bamboo.specs.api.codegen.CodeGenerationContext;
import com.atlassian.bamboo.specs.api.codegen.CodeGenerationException;
import org.apache.commons.lang3.StringEscapeUtils;
import org.jetbrains.annotations.NotNull;
/**
* Generates code for simple Java types.
*/
public class LiteralEmitter implements CodeEmitter {
@NotNull
@Override
public String emitCode(final CodeGenerationContext context, final Object value) throws CodeGenerationException {
if (value == null) {
return "null";
}
Class type = value.getClass();
if (type == Boolean.class || type == Boolean.TYPE) {
return Boolean.toString((Boolean) value);
} else if (type == Long.class || type == Long.TYPE) {
return Long.toString((Long) value);
} else if (type == Integer.class || type == Integer.TYPE) {
return Integer.toString((Integer) value);
} else if (type == String.class) {
return "\"" + StringEscapeUtils.escapeJava((String) value) + "\"";
} else if (type.isEnum()) {
return context.importClassName(type) + "." + ((Enum) value).name();
}
throw new CodeGenerationException("don't know how to generate code for " + type.getCanonicalName());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy