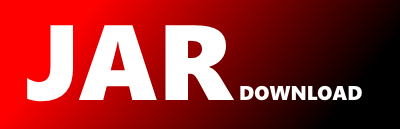
com.atlassian.connect.spring.AtlassianHostUser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of atlassian-connect-spring-boot-api Show documentation
Show all versions of atlassian-connect-spring-boot-api Show documentation
Provides the API of Atlassian Connect for Spring Boot
package com.atlassian.connect.spring;
import org.springframework.security.core.annotation.AuthenticationPrincipal;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.security.core.userdetails.User;
import java.util.Objects;
import java.util.Optional;
/**
* The Spring Security
* authentication principal for requests coming from an Atlassian host application in which the
* add-on is installed.
*
* This class is the Atlassian Connect equivalent of Spring Security's {@link User} and can be resolved as a Spring
* MVC controller argument by using the {@link AuthenticationPrincipal} annotation:
*
@Controller
* public class HelloWorldController {
*
* @RequestMapping(value = "/helloWorld", method = GET)
* public String (@AuthenticationPrincipal AtlassianHostUser hostUser) {
* ...
* }
* }
*
* Outside of the context of a controller, the current authentication principal can be obtained programmatically via
* {@link SecurityContextHolder}:
*
Optional<AtlassianHostUser> optionalHostUser = Optional.ofNullable(SecurityContextHolder.getContext().getAuthentication())
* .map(Authentication::getPrincipal)
* .filter(AtlassianHostUser.class::isInstance)
* .map(AtlassianHostUser.class::cast);
*
* @since 1.0.0
*/
public final class AtlassianHostUser {
private AtlassianHost host;
private Optional userAccountId;
/**
* Creates a new empty Atlassian host principal (used for deserialization).
*/
protected AtlassianHostUser() {
}
/**
* Returns the host from which the request originated.
*
* @return the Atlassian host
*/
public AtlassianHost getHost() {
return host;
}
/**
* The Atlassian Account ID of the user on whose behalf a request was made.
*
* @return the Atlassian Account ID
*/
public Optional getUserAccountId() {
return userAccountId;
}
/**
* {@inheritDoc}
*/
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AtlassianHostUser that = (AtlassianHostUser) o;
return Objects.equals(host, that.host)
&& Objects.equals(userAccountId, that.userAccountId);
}
/**
* {@inheritDoc}
*/
@Override
public int hashCode() {
return Objects.hash(host, userAccountId);
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
return String.format("AtlassianHostUser{host=%s, userAccountId='%s'}", host, userAccountId.orElse("null"));
}
/**
* Creates an {@link AtlassianHostUserBuilder} using the provided host.
*
* @param host the host to create the AtlassianHostUser for
* @return a builder.
*/
public static AtlassianHostUserBuilder builder(AtlassianHost host) {
return new AtlassianHostUserBuilder(host);
}
/**
* Builder used to create an {@link AtlassianHostUser}.
*/
public static class AtlassianHostUserBuilder {
private final AtlassianHostUser hostUser;
private AtlassianHostUserBuilder(AtlassianHost host) {
hostUser = new AtlassianHostUser();
if (host == null) {
throw new IllegalArgumentException("Host must be set for user");
}
hostUser.host = host;
hostUser.userAccountId = Optional.empty();
}
/**
* Adds an Atlassian Account ID to the {@link AtlassianHostUserBuilder}.
*
* @param userAccountId the Atlassian Account ID to add
* @return the builder
*/
public AtlassianHostUserBuilder withUserAccountId(String userAccountId) {
if (userAccountId == null) {
throw new IllegalArgumentException("The userAccountId can not be null.");
}
hostUser.userAccountId = Optional.of(userAccountId);
return this;
}
/**
* Builds an {@link AtlassianHostUser} from the provided details.
*
* @return the Atlassian Host User.
*/
public AtlassianHostUser build() {
return hostUser;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy