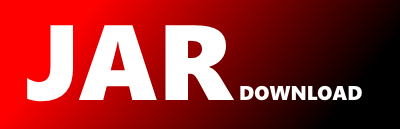
com.atlassian.connect.spring.AtlassianHost Maven / Gradle / Ivy
package com.atlassian.connect.spring;
import org.springframework.data.annotation.CreatedBy;
import org.springframework.data.annotation.CreatedDate;
import org.springframework.data.annotation.Id;
import org.springframework.data.annotation.LastModifiedBy;
import org.springframework.data.annotation.LastModifiedDate;
import java.util.Calendar;
import java.util.Objects;
/**
* An Atlassian host in which the add-on is or has been installed. Hosts are stored in {@link AtlassianHostRepository}.
*
* During processing of a request from an Atlassian host, the details of the host and of the user at the browser can
* be obtained from the {@link AtlassianHostUser}.
*
* @see AtlassianHostRepository
* @since 1.0.0
*/
public class AtlassianHost {
@Id
private String clientKey; // "unique-client-identifier"
private String oauthClientId; // "OpaQUeCliEnt1DStR1Ng"
private String sharedSecret; // "a-secret-key-not-to-be-lost"
private AddonAuthenticationType authentication; // "jwt" or null
private String cloudId; // "string" or null
private String baseUrl; // "http://example.atlassian.net"
private String displayUrl; // "http://example.customdomain.com"
private String displayUrlServicedeskHelpCenter; // "http://example.customdomain.com"
private String capabilitySet; //"capabilityStandard" or "capabilityAdvanced"
private String productType; // "jira"
private String description; // "Atlassian JIRA at https://example.atlassian.net"
private String serviceEntitlementNumber; // "SEN-number"
private String entitlementId; // "Entitlement ID"
private String entitlementNumber; // "Entitlement Number"
private String installationId; // "ari:cloud:ecosystem::installation/installation-identifier"
private boolean addonInstalled;
@CreatedDate
private Calendar createdDate;
@LastModifiedDate
private Calendar lastModifiedDate;
@CreatedBy
private String createdBy;
@LastModifiedBy
private String lastModifiedBy;
/**
* Creates a new empty Atlassian host (used by persistence mechanism).
*/
public AtlassianHost() {
}
/**
* The identifying key for the Atlassian product instance that the add-on was installed into.
* This will never change for a given instance, and is unique across all Atlassian product tenants.
* This value should be used to key tenant details in your add-on.
*
* @return the client key of the host
*/
public String getClientKey() {
return clientKey;
}
/**
* Used by persistence mechanism.
*
* @param clientKey the client key of the host
*/
public void setClientKey(String clientKey) {
this.clientKey = clientKey;
}
/**
* Returns the client identifier for use with OAuth 2.0 authentication.
*
* @return the OAuth 2.0 client ID
*/
public String getOauthClientId() {
return oauthClientId;
}
/**
* Used by persistence mechanism.
*
* @param oauthClientId the OAuth 2.0 client ID
*/
public void setOauthClientId(String oauthClientId) {
this.oauthClientId = oauthClientId;
}
/**
* The shared secret issued by the Atlassian product instance on installation of the add-on. This string is used
* to validate incoming JWT tokens and to sign outgoing JWT tokens.
*
* @return the shared secret of the installation
*/
public String getSharedSecret() {
return sharedSecret;
}
/**
* Used by persistence mechanism.
*
* @param sharedSecret the shared secret of the installation
*/
public void setSharedSecret(String sharedSecret) {
this.sharedSecret = sharedSecret;
}
/**
* Returns the authentication type to use when communicating with the host products.
*
* @return AddonAuthenticationType as authentication scheme
*/
public AddonAuthenticationType getAuthentication() {
return authentication;
}
/**
* Used by persistence mechanism.
*
* @param authentication the authentication type to use when communicating with the host products.
*/
public void setAuthentication(AddonAuthenticationType authentication) {
this.authentication = authentication;
}
/**
* Returns the tenant identifier for use with OAuth 2.0 client credentials flow.
*
* @return cloudId
*/
public String getCloudId() {
return cloudId;
}
/**
* Used by persistence mechanism.
*
* @param cloudId the tenant identifier for use with OAuth 2.0 client credentials flow.
*/
public void setCloudId(String cloudId) {
this.cloudId = cloudId;
}
/**
* The URL prefix for the Atlassian product instance. All of its REST endpoints begin with this URL.
*
* @return the base URL of the host
*/
public String getBaseUrl() {
return baseUrl;
}
/**
* Used by persistence mechanism.
*
* @param baseUrl the base URL of the host
*/
public void setBaseUrl(String baseUrl) {
this.baseUrl = baseUrl;
}
/**
* Return the Display Url ie the Custom Domain Url for Jira/Confluence
* If Custom Domain doesn't exist this equals to base URL
*
* @return the display URL of the host
*/
public String getDisplayUrl() {
return displayUrl;
}
/**
* Used by persistence mechanism.
*
* @param displayUrl the display URL of the host
*/
public void setDisplayUrl(String displayUrl) {
this.displayUrl = displayUrl;
}
/**
* Return the Display Url of Servicedesk Help Center ie the Custom Domain Url for JSD
* If Custom Domain doesn't exist this equals to base URL
*
* @return the display URL of SD Helper Center of the host
*/
public String getDisplayUrlServicedeskHelpCenter() {
return displayUrlServicedeskHelpCenter;
}
/**
* Used by persistence mechanism.
*
* @param displayUrlServicedeskHelpCenter the display servicedesk help center URL of the host
*/
public void setDisplayUrlServicedeskHelpCenter(String displayUrlServicedeskHelpCenter) {
this.displayUrlServicedeskHelpCenter = displayUrlServicedeskHelpCenter;
}
/**
* The identifier of the category of Atlassian product.
*
* @return the host product type, e.g. jira
or confluence
*/
public String getProductType() {
return productType;
}
/**
* Used by persistence mechanism.
*
* @param productType the host product type, e.g. jira
or confluence
*/
public void setProductType(String productType) {
this.productType = productType;
}
/**
* The host product description. This string is customisable by an instance administrator.
*
* @return the description of the host
*/
public String getDescription() {
return description;
}
/**
* Used by persistence mechanism.
*
* @param description the description of the host
*/
public void setDescription(String description) {
this.description = description;
}
/**
* The Support Entitlement Number (SEN) is the add-on license identifier.
* This attribute will only be included during installation of a paid add-on.
*
* @return the SEN
*/
public String getServiceEntitlementNumber() {
return serviceEntitlementNumber;
}
/**
* Used by persistence mechanism.
*
* @param serviceEntitlementNumber the SEN
*/
public void setServiceEntitlementNumber(String serviceEntitlementNumber) {
this.serviceEntitlementNumber = serviceEntitlementNumber;
}
/**
* Entitlement ID is the add-on license identifier.
* This attribute will only be included during installation of a paid add-on.
*
* @return the Entitlement ID
*/
public String getEntitlementId() {
return entitlementId;
}
/**
* Used by persistence mechanism.
*
* @param entitlementId the Entitlement ID
*/
public void setEntitlementId(String entitlementId) {
this.entitlementId = entitlementId;
}
/**
* Entitlement Number is the add-on license identifier.
* This attribute will only be included during installation of a paid add-on.
*
* @return the Entitlement Number
*/
public String getEntitlementNumber() {
return entitlementNumber;
}
/**
* Used by persistence mechanism.
*
* @param entitlementNumber the Entitlement Number
*/
public void setEntitlementNumber(String entitlementNumber) {
this.entitlementNumber = entitlementNumber;
}
/**
* Installation ID is the Forge identifier of an installation to workspace.
*
* @return the Forge installation ID string (in ARI format, e.g. ari:cloud:ecosystem::installation/c3658f0f-8380-41e5-bb1e-68903f8efdca)
* if the installed version is registered on Forge, otherwise null
*/
public String getInstallationId() {
return installationId;
}
/**
* Used by persistence mechanism.
*
* @param installationId the Installation ID
*/
public void setInstallationId(String installationId) {
this.installationId = installationId;
}
/**
* Returns true if the add-on is currently installed on the host. Upon uninstallation, the value of this flag will
* be set to false
.
*
* @return true if the add-on is installed
*/
public boolean isAddonInstalled() {
return addonInstalled;
}
/**
* Used by persistence mechanism.
*
* @param addonInstalled true if the add-on is installed
*/
public void setAddonInstalled(boolean addonInstalled) {
this.addonInstalled = addonInstalled;
}
/**
* The time at which the add-on was first installed on the host.
*
* @return the creation time
*/
public Calendar getCreatedDate() {
return createdDate;
}
/**
* Used by persistence mechanism.
*
* @param createdDate the creation time
*/
public void setCreatedDate(Calendar createdDate) {
this.createdDate = createdDate;
}
/**
* The time at which the add-on installation was last modified.
*
* @return the last modification time
*/
public Calendar getLastModifiedDate() {
return lastModifiedDate;
}
/**
* Used by persistence mechanism.
*
* @param lastModifiedDate the last modification time
*/
public void setLastModifiedDate(Calendar lastModifiedDate) {
this.lastModifiedDate = lastModifiedDate;
}
/**
* The key of the Atlassian user that first installed the add-on on the host, if any.
*
* @return the user key of the creator
*/
public String getCreatedBy() {
return createdBy;
}
/**
* Used by persistence mechanism.
*
* @param createdBy the user key of the creator
*/
public void setCreatedBy(String createdBy) {
this.createdBy = createdBy;
}
/**
* The key of the Atlassian user that last modified the add-on installation, if any.
*
* @return the user key of the last modifier
*/
public String getLastModifiedBy() {
return lastModifiedBy;
}
/**
* Used by persistence mechanism.
*
* @param lastModifiedBy the user key of the last modifier
*/
public void setLastModifiedBy(String lastModifiedBy) {
this.lastModifiedBy = lastModifiedBy;
}
/**
* The Capability Set of the app is the type of app edition installed (capabilityStandard or capabilityAdvanced).
* This attribute will only be included during installation of a paid add-on.
*
* @return the Capability Set
*/
public String getCapabilitySet() {
return capabilitySet;
}
/**
* Used by persistence mechanism.
*
* @param capabilitySet the Capability Set
*/
public void setCapabilitySet(String capabilitySet) {
this.capabilitySet = capabilitySet;
}
/**
* {@inheritDoc}
*/
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AtlassianHost that = (AtlassianHost) o;
return addonInstalled == that.addonInstalled &&
Objects.equals(clientKey, that.clientKey) &&
Objects.equals(oauthClientId, that.oauthClientId) &&
Objects.equals(sharedSecret, that.sharedSecret) &&
Objects.equals(authentication, that.authentication) &&
Objects.equals(cloudId, that.cloudId) &&
Objects.equals(baseUrl, that.baseUrl) &&
Objects.equals(displayUrl, that.displayUrl) &&
Objects.equals(displayUrlServicedeskHelpCenter, that.displayUrlServicedeskHelpCenter) &&
Objects.equals(productType, that.productType) &&
Objects.equals(description, that.description) &&
Objects.equals(serviceEntitlementNumber, that.serviceEntitlementNumber) &&
Objects.equals(entitlementId, that.entitlementId) &&
Objects.equals(entitlementNumber, that.entitlementNumber) &&
Objects.equals(installationId, that.installationId) &&
Objects.equals(capabilitySet, that.capabilitySet);
}
/**
* {@inheritDoc}
*/
@Override
public int hashCode() {
return Objects.hash(clientKey,
oauthClientId,
sharedSecret,
authentication,
cloudId,
baseUrl,
displayUrl,
displayUrlServicedeskHelpCenter,
productType,
description,
serviceEntitlementNumber,
entitlementId,
entitlementNumber,
addonInstalled,
installationId,
capabilitySet);
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
return String.format("AtlassianHost{clientKey='%s', oauthClientId='%s', sharedSecret='%s', authentication=%s, cloudId='%s', baseUrl='%s', displayUrl='%s', displayUrlServicedeskHelpCenter='%s', capabilitySet=%s, productType='%s', description='%s', serviceEntitlementNumber='%s', entitlementId='%s', entitlementNumber='%s', installationId='%s', addonInstalled=%s}",
clientKey,
oauthClientId,
sharedSecret,
authentication,
cloudId,
baseUrl,
displayUrl,
displayUrlServicedeskHelpCenter,
capabilitySet,
productType,
description,
serviceEntitlementNumber,
entitlementId,
entitlementNumber,
installationId,
addonInstalled);
}
}