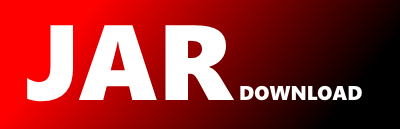
com.atlassian.connect.spring.AtlassianHostRestClients Maven / Gradle / Ivy
Show all versions of atlassian-connect-spring-boot-api Show documentation
package com.atlassian.connect.spring;
import org.springframework.http.HttpMethod;
import org.springframework.security.oauth2.core.OAuth2AccessToken;
import org.springframework.web.client.RestTemplate;
import java.net.URI;
/**
* A helper class for obtaining preconfigured {@link RestTemplate}s to make authenticated requests to Atlassian hosts.
*
* JWT
*
* To make requests using JWT, the add-on must specify the authentication type {@code jwt} in its add-on descriptor.
*
*
To obtain a {@code RestTemplate} using JWT authentication, use {@link #authenticatedAsAddon()}:
*
@Autowired
* private AtlassianHostRestClients restClients;
*
* public void makeRequest() {
* restClients.authenticatedAsAddon().getForObject(...);
* }
*
* OAuth 2.0 - JWT Bearer Token
*
* To make requests using OAuth 2.0, the add-on must request the {@code ACT_AS_USER} scope in its add-on descriptor.
*
*
To obtain a {@code RestTemplate} using OAuth 2.0 authentication, use {@link #authenticatedAsHostActor} or
* {@link #authenticatedAs(AtlassianHostUser)}:
*
@Autowired
* private AtlassianHostRestClients restClients;
*
* public void makeRequest() {
* restClients.authenticatedAsHostActor().getForObject(...);
* }
*
* @since 1.1.0
*/
public interface AtlassianHostRestClients {
/**
* Returns a {@code RestTemplate} for making requests to Atlassian hosts using JWT authentication.
* The principal of the request is the add-on.
*
* During processing of a request from an Atlassian host, relative URLs can be used to make requests to the
* current host.
*
*
When a request is made to an absolute URL, the request URL is used to resolve the destination Atlassian host.
* If no host matches, the request is not signed.
*
* @return a REST client for JWT authentication
* @see #authenticatedAsAddon(AtlassianHost)
*/
RestTemplate authenticatedAsAddon();
/**
* Returns a {@code RestTemplate} for making requests to Atlassian hosts using JWT authentication.
* The principal of the request is the add-on.
*
*
Relative URLs can be used to make requests to the given host.
*
*
When a request is made to an absolute URL, the URL must match the base URL of the given host.
*
* @param host the host to which the request should be made
* @return a REST client for JWT authentication
* @see #authenticatedAsAddon()
*/
RestTemplate authenticatedAsAddon(AtlassianHost host);
/**
* Creates a JSON Web Token for use when the {@code RestTemplate} provided by {@link #authenticatedAsAddon()}
* cannot be used to make requests to Atlassian hosts, such as when using Jersey.
*
WebTarget webTarget = ClientBuilder.newClient().target(host.getBaseUrl()).path(...);
* String jwt = atlassianHostRestClients.createJwt(HttpMethod.GET, webTarget.getUri());
* Response response = webTarget.request().header("Authorization", "JWT " + jwt).get();
*
* NOTE: Whenever possible, use of {@link #authenticatedAsAddon()} is recommended over use of
* this method.
*
*
The created JWT is restricted for use with the given HTTP method and request URL.
*
*
The request URL is used to resolve the destination Atlassian host. If no host matches, an
* {@code IllegalArgumentException} is thrown.
*
* @param method the HTTP method of the request to be authenticated
* @param uri the absolute URL of the request to be authenticated
* @return a JWT for use when authenticating as the add-on
* @throws IllegalArgumentException if the URL did not have the base URL of any installed host
* @see #authenticatedAsAddon()
* @see #authenticatedAsAddon(AtlassianHost)
* @since 1.3.0
*/
String createJwt(HttpMethod method, URI uri);
/**
* Returns a {@code RestTemplate} for making requests to the currently authenticated Atlassian host using
* OAuth 2.0 JWT Bearer Token authentication. The principal of the request is the currently authenticated user.
*
*
On first invocation, {@code OAuth2JwtTokenService} will request an access token from Atlassian's
* authorization server, and the token will be stored for further use. Once the token has expired, a new
* token will be fetched transparently. Additionally, the {@code RestTemplate} for a particular host user
* is cached between requests using
* Spring Caching.
*
* @return a REST client for OAuth 2.0 JWT Bearer Token authentication
* @see #authenticatedAs(AtlassianHostUser)
*/
RestTemplate authenticatedAsHostActor();
/**
* Returns a {@code RestTemplate} for making requests to the given Atlassian host using OAuth 2.0 JWT Bearer Token
* authentication. The principal of the request is the given user.
*
*
On first invocation, {@code OAuth2JwtTokenService} will request an access token from Atlassian's
* authorization server, and the token will be stored for further use. Once the token has expired, a new
* token will be fetched transparently. Additionally, the {@code RestTemplate} for a particular host user
* is cached between requests using
* Spring Caching.
*
* @param hostUser the host to which the request should be made, and the user principal
* @return a REST client for OAuth 2.0 JWT Bearer Token authentication
* @see #authenticatedAsHostActor()
*/
RestTemplate authenticatedAs(AtlassianHostUser hostUser);
/**
* Get the access token for use when authenticating as the host user {@link #authenticatedAs(AtlassianHostUser)}.
*
* For example, you may explicitly get the access token and add it to the Authorization header when making a request,
* such as when using Jersey.
*
* String token = atlassianHostRestClients.getAccessToken(hostUser).getTokenValue();
* requestContext.getHeaders().add("Authorization", String.format("Bearer %s", token));
*
*
* NOTE: You do not need to explicitly get and set access token if you are using {@link #authenticatedAsHostActor()}
* or {@link #authenticatedAs(AtlassianHostUser)}, as it is already handled for you.
*
* @param hostUser the host to which the request should be made, and the user principal
* @return an access token for use when authenticating as the host user
*/
OAuth2AccessToken getAccessToken(AtlassianHostUser hostUser);
/**
* Returns a {@code RestTemplate} for making authenticated requests to Atlassian hosts.
* The principal of the request is the add-on. Applicable for Connect-on-Forge apps only.
*
*
During processing of a request from an Atlassian host, relative URLs can be used to make requests to the
* current host.
*
*
When a request is made to an absolute URL, the request URL is used to resolve the destination Atlassian host.
* If no host matches, the request is not signed.
*
*
* When {@code AddonAuthenticationType} is set to "jwt", the returned {@code RestTemplate} can only make request using JWT authentication,
* and if {@code AddonAuthenticationType} is "oauth2" it can make request with OAuth 2.0 client credentials flow when your app has opted
* in oauth2 - in case your app has not opted in oauth2 this method will throw an exception.
*
* @param auth an authentication type to be used for making authenticated requests to Atlassian hosts.
* @return the REST template for making authenticated requests to Atlassian hosts.
*/
RestTemplate authenticatedAsAddon(AddonAuthenticationType auth);
/**
* Returns a {@code RestTemplate} for making authenticated requests to Atlassian hosts for a specified.
* The principal of the request is the add-on. Applicable for Connect-on-Forge apps only.
*
*
Relative URLs can be used to make requests to the given host.
*
*
When a request is made to an absolute URL, the URL must match the base URL of the given host.
* Available options for the auth argument are: "jwt", and "oauth2".
*
* When {@code AddonAuthenticationType} is set to "jwt", the returned {@code RestTemplate} can only make request using JWT authentication,
* and if {@code AddonAuthenticationType} is "oauth2" it can make request with OAuth 2.0 client credentials flow when your app has opted
* in oauth2 - in case your app has not opted in oauth2 this method will throw an exception.
*
* @param host the host to which the request should be made
* @param auth an authentication type to be used for making authenticated requests to Atlassian hosts.
* @return the REST template for making authenticated requests to Atlassian hosts.
*/
RestTemplate authenticatedAsAddon(AtlassianHost host, AddonAuthenticationType auth);
}