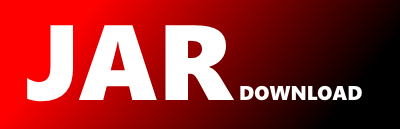
com.atlassian.connect.spring.internal.jwt.CanonicalRequestUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of atlassian-connect-spring-boot-jwt Show documentation
Show all versions of atlassian-connect-spring-boot-jwt Show documentation
Provides JSON Web Token handling for Atlassian Connect for Spring Boot
The newest version!
package com.atlassian.connect.spring.internal.jwt;
import org.springframework.core.style.ToStringCreator;
import org.springframework.util.ObjectUtils;
import org.springframework.util.StringUtils;
import java.util.Arrays;
import java.util.Iterator;
import java.util.Map;
import java.util.Optional;
public class CanonicalRequestUtil {
private CanonicalRequestUtil() {
throw new IllegalStateException("Utility class");
}
public static String getRelativePath(String requestPath, String contextPath) {
String contextPathToRemove = null == contextPath || "/".equals(contextPath) ? "" : contextPath;
return Optional.ofNullable(requestPath)
.filter(s -> !ObjectUtils.isEmpty(s))
.map(s -> s.startsWith(contextPathToRemove) ? s.substring(contextPathToRemove.length()) : s)
.map(s -> StringUtils.trimTrailingCharacter(s, '/'))
.orElse("/");
}
// So we can share between different impls.
// Likely too large to be a useful toString
public static String toVerboseString(CanonicalHttpRequest request) {
return new ToStringCreator(request)
.append("method", request.getMethod())
.append("relativePath", request.getRelativePath())
.append("parameterMap", mapToString(request.getParameterMap()))
.toString();
}
private static String mapToString(Map parameterMap) {
StringBuilder sb = new StringBuilder()
.append('[');
for (Map.Entry entry : parameterMap.entrySet()) {
sb.append(entry.getKey()).append(" -> ");
String[] value = entry.getValue();
if (value != null) {
sb.append("(");
appendTo(sb, Arrays.asList(value), ",");
sb.append(")");
}
sb.append(','); // I know being lazy
}
return sb.append(']')
.toString();
}
// borrowed from guava Join so can avoid guava dependency and OSGI fun
private static StringBuilder appendTo(StringBuilder appendable, Iterable> parts, CharSequence separator) {
Iterator> iterator = parts.iterator();
if (iterator.hasNext()) {
appendable.append(toString(iterator.next()));
while (iterator.hasNext()) {
appendable.append(separator);
appendable.append(toString(iterator.next()));
}
}
return appendable;
}
private static CharSequence toString(Object part) {
if (part == null) {
return "";
}
if (part instanceof CharSequence charSequence) {
return charSequence;
}
return part.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy