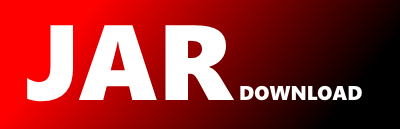
com.atlassian.connect.spring.internal.jwt.RsaJwtReader Maven / Gradle / Ivy
Show all versions of atlassian-connect-spring-boot-jwt Show documentation
package com.atlassian.connect.spring.internal.jwt;
import com.nimbusds.jose.Algorithm;
import com.nimbusds.jose.JWSAlgorithm;
import com.nimbusds.jose.JWSObject;
import com.nimbusds.jose.crypto.RSASSAVerifier;
import java.security.KeyFactory;
import java.security.NoSuchAlgorithmException;
import java.security.interfaces.RSAPublicKey;
import java.security.spec.InvalidKeySpecException;
import java.security.spec.X509EncodedKeySpec;
import java.text.ParseException;
import java.util.Base64;
public class RsaJwtReader extends AbstractJwtReader {
private static final Algorithm SUPPORTED_ALGORITHM = JWSAlgorithm.RS256;
public static final String BEGIN = "-----BEGIN PUBLIC KEY-----";
public static final String END = "-----END PUBLIC KEY-----";
/**
* Parses the jwt and returns the key id if the jwt is signed with RS256
*
* Note that this method can be removed once signed installs is completely rolled out
* and we can assume all installs are signed with the asymmetric key
*
* @return The key ID parameter
* @throws JwtInvalidSigningAlgorithmException if the algorithm used to sign the JWT was not RS256
* @throws JwtParseException if parsing the JWT failed
*/
public static String getKeyIdAndCheckSigningAlgorithm(String jwt) throws JwtInvalidSigningAlgorithmException, JwtParseException {
final JWSObject jwsObject;
try {
jwsObject = JWSObject.parse(jwt);
} catch (ParseException e) {
throw new JwtParseException(e);
}
Algorithm algorithm = jwsObject.getHeader().getAlgorithm();
if (!SUPPORTED_ALGORITHM.equals(algorithm)) {
throw new JwtInvalidSigningAlgorithmException(String.format("Expected JWT to be signed with '%s' but it was signed with '%s' instead",
SUPPORTED_ALGORITHM, algorithm));
}
return jwsObject.getHeader().getKeyID();
}
@Override
protected Algorithm getSupportedAlgorithm() {
return SUPPORTED_ALGORITHM;
}
private static RSAPublicKey fromPEMEncodedKey(String pemEncodedKey) throws NoSuchAlgorithmException, InvalidKeySpecException {
String base64 = pemEncodedKey
.replace(BEGIN, "")
.replace(END, "")
.replaceAll("\\R", "");
byte[] bytes = Base64.getDecoder().decode(base64);
KeyFactory keyFactory = KeyFactory.getInstance("RSA");
X509EncodedKeySpec keySpec = new X509EncodedKeySpec(bytes);
return (RSAPublicKey) keyFactory.generatePublic(keySpec);
}
public RsaJwtReader(String pemEncodedKey) throws NoSuchAlgorithmException, InvalidKeySpecException {
this(fromPEMEncodedKey(pemEncodedKey));
}
public RsaJwtReader(RSAPublicKey key) {
super(new RSASSAVerifier(key));
}
}