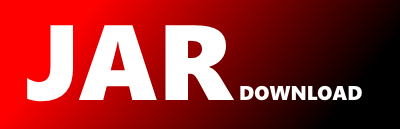
com.atlassian.usercontext.api.UserContextClaims Maven / Gradle / Ivy
package com.atlassian.usercontext.api;
import java.util.List;
import java.util.Optional;
import java.util.Set;
/**
* User-context specific claims.
*
* Reference: https://hello.atlassian.net/wiki/spaces/ARCH/pages/161912986/Specification+Standard+User+Context+Claims.
*/
public interface UserContextClaims {
/**
* Accessor for {@link UserContextRegisteredClaim#ACCOUNT_ID_CLAIM}
*
* Native user Identifier as provided by Identity (sometimes called Atlassian account Id,
* but may be a customer user account identifier at some point in the future).
*
* Normally this represents the user being impersonated as part of this request.
*
* However, when {@link UserContextRegisteredClaim#IMPERSONATION_CLAIM}s are provided, the accountId
* refers to the impersonator.
*
* @return the accountId of the user context
*/
AccountId getAccountId();
/**
* Accessor for {@link UserContextRegisteredClaim#SCOPE_CLAIM}
*
* Claim defining OAuth 2 scopes related to the request. If the claim is not present, downstream services
* consuming the token would assume no scope restrictions.
*
* @return a set of strings representing the scopes related to the request.
*/
Optional> getScopes();
/**
* Accessor for {@link UserContextRegisteredClaim#SESSION_ID_CLAIM}
*
* Claim that includes the user's session Id (if they are authenticating via web session).
*
* @return the user's session id
*/
Optional getSessionId();
/**
* Accessor for {@link UserContextRegisteredClaim#IMPERSONATION_CONTEXT_RESTRICTION_CLAIM}
*
* A user's context may be restricted such as:
*
* - OAuth API authenticated requests, where the audience of the request is restricted
* (i.e. a client is only granted access to specific sites).
* - Site-specific user session for accounts that have not had their email fully verified.
*
*
* It would be an array of Atlassian Resource Identifiers.
*
* @return A list of Atlassian Resource Identifiers representing context restrictions
*/
List getContextRestriction();
/**
* Accessor for {@link UserContextRegisteredClaim#EMAIL_DOMAIN_CLAIM}
*
* Domain of the user's primary email address. This is provided as email domain is used for privacy algorithms.
*
* @return the email domain of the uer's primary email address
*/
Optional getEmailDomain();
/**
* Accessor for {@link UserContextRegisteredClaim#UNVERIFIED_EMAIL_CLAIM}
*
* Some operations such as user administrations are blocked if the user has not yet verified their email.
* If this claim is not present, the email is assumed to be verified (which is the normal case).
*
* @return whether or not the email is verified
*/
Optional isUnverifiedEmail();
/**
* Accessor for {@link UserContextRegisteredClaim#IMPERSONATION_CLAIM}
*
* A user may impersonate another user (e.g. a support team member impersonating a customer user for
* debugging purposes). When this happens, the accountId claim refers to the impersonator i.e. support team member,
* and the impersonations claim is present including user context about the user being impersonated
* (i.e. customer user). Services that support impersonation should use the user context in this impersonations
* claim. It is designed so that services that do not support impersonation can safely ignore the impersonations
* claim and process the request as the impersonator (i.e. services that don't support impersonate fall back to
* the real user).
*
* The impersonations claim is an array of objects that describe active, unexpired impersonated sessions
*
* @return a list of active, unexpired impersonated sessions
*/
List getImpersonations();
/**
* Accessor for {@link UserContextRegisteredClaim#OAUTH_CLIENT_ID_CLAIM}
*
* For OAuth authenticated requests, this would include the OAuth client id
*
* @return the oauth client id for oauth authenticated requests
*/
Optional getOauthClientId();
/**
* Accessor for {@link UserContextRegisteredClaim#FIRST_PARTY_CLAIM}
*
* A boolean indicating whether the authentication call is from an Atlassian first-party client ("firstParty": true)
* or a 3rd party client ("firstParty": false).
*
* Caution: session authenticated requests are marked as firstParty: true, but they do not necessarily come from
* trusted clients.
*
* @return whether the request is from an Atlassian first-party client or not
*/
Optional isFirstParty();
/**
* Accessor for {@link UserContextRegisteredClaim#REQUEST_PRINCIPAL_CLAIM}
*
* Atlassian Resource Identifier that the Atlassian API provider can use to perform permission checks. Depending
* on the authentication method, it can be a user principal, system user principal or user-client principal
* e.g ari:cloud:identity::userGrant/5fba342526667-b6fb72938tqw
*
* @return the atlassian resource identifier for the request principal
*/
Optional getRequestPrincipal();
/**
* Accessor for {@link UserContextRegisteredClaim#ON_BEHALF_OF_CLAIM}
*
* Present for OAuth authenticated requests. True if this request is on behalf of a user (3LO), false for 2LO.
*
* @return true if this is a 3LO request, false for 2LO, and empty otherwise
*/
Optional isOnBehalfOf();
enum UserContextRegisteredClaim {
SCOPE_CLAIM("scope"),
ACCOUNT_ID_CLAIM("accountId"),
IMPERSONATION_CONTEXT_RESTRICTION_CLAIM("contextRestriction"),
IMPERSONATION_CLAIM("impersonations"),
EMAIL_DOMAIN_CLAIM("emailDomain"),
UNVERIFIED_EMAIL_CLAIM("unverifiedEmail"),
SESSION_ID_CLAIM("sessionId"),
OAUTH_CLIENT_ID_CLAIM("oauthClientId"),
FIRST_PARTY_CLAIM("firstParty"),
REQUEST_PRINCIPAL_CLAIM("requestPrincipal"),
ON_BEHALF_OF_CLAIM("onBehalfOf");
private final String key;
UserContextRegisteredClaim(String key) {
this.key = key;
}
public String key() {
return key;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy