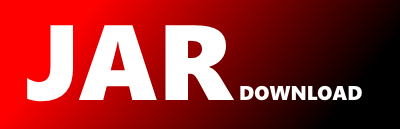
com.att.aft.dme2.util.DME2Utils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dme2-api Show documentation
Show all versions of dme2-api Show documentation
Direct Messaging Engine dme2-api
The newest version!
package com.att.aft.dme2.util;
import java.net.InetAddress;
import java.net.NetworkInterface;
import java.net.URLDecoder;
import java.net.URLEncoder;
import java.net.UnknownHostException;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
import com.att.aft.dme2.api.DME2Exception;
import com.att.aft.dme2.api.DME2Manager;
import com.att.aft.dme2.config.DME2Configuration;
import com.att.aft.dme2.logging.LogMessage;
import com.att.aft.dme2.logging.Logger;
import com.att.aft.dme2.logging.LoggerFactory;
import com.att.scld.grm.types.v1.NameValuePair;
import com.att.scld.grm.types.v1.VersionDefinition;
public class DME2Utils {
private static final Logger logger = LoggerFactory.getLogger(DME2Utils.class.getName());
private DME2Utils() {
}
public static List convertPropertiestoNameValuePairs(Properties props)
{
List nameValuePairs = new ArrayList();
if(props != null) {
for(Object obj : props.keySet()) {
String key = (String) obj;
String value = props.getProperty(key);
NameValuePair nameValPair = new NameValuePair();
nameValPair.setName(key);
nameValPair.setValue(value);
nameValuePairs.add(nameValPair);
}
}
return nameValuePairs;
}
public static Properties convertNameValuePairToProperties( List properties ) {
return null;
}
public static boolean isInIgnoreList(DME2Configuration config, String queueName){
String ignoreList = config.getProperty(DME2Constants.AFT_DME2_METRICS_SVC_LIST_IGNORE);
String splitStr[] = ignoreList.split(",");
logger.info(null, "isInIgnoreList", " inside isInIgnoreList queueName : {}", queueName);
for(int i=0; i targetType)
{
try
{
if (targetType == Integer.class)
{
Integer.parseInt(value);
}
else if (targetType == Long.class)
{
Long.parseLong(value);
}
else if (targetType == Double.class)
{
Double.parseDouble(value);
}
}
catch(NumberFormatException e)
{
return false;
}
return true;
}
/**Adds query string to the URI path string. If no query string was passed in then the path is just returned.*/
public static String appendQueryStringToPath(String path,final String newQueryStr)
{
String queryStr=newQueryStr;
if(queryStr == null){
return path;
}
if(!queryStr.startsWith("?")){
queryStr = "?" + queryStr;
}
return path + queryStr;
}
public static Map splitServiceURIString(final String newServiceURI)
{
String serviceURI=newServiceURI;
Map serviceURIValues = new HashMap();
if(!serviceURI.startsWith("/")){
serviceURI = "/" + serviceURI;
}
String[] toks = serviceURI.split("/");
for(String tok : toks)
{
if(tok.contains("="))
{
String[] pair = tok.split("=");
String key = pair[0];
String value = pair[1];
serviceURIValues.put(key, value);
}
}
return serviceURIValues;
}
/**
*
* @return
*/
public static String getRunningInstanceName(DME2Configuration config) {
// if this container is LRM managed, use LRM provided JVM args to decide on serviceName
// If application user is setting a uniq name to use, use it as override for LRM attributes
String userProvidedServiceNameStr = config.getProperty("AFT_DME2_PF_SERVICE_NAME");
if(userProvidedServiceNameStr != null){
return userProvidedServiceNameStr;
}
String resName = config.getProperty(config.getProperty(DME2Constants.AFT_DME2_CONTAINER_NAME_KEY));
if(resName != null) {
// Chances are this is a LRM managed container
String resVer = config.getProperty(config.getProperty(DME2Constants.AFT_DME2_CONTAINER_VERSION_KEY));
String resEnv= config.getProperty(config.getProperty(DME2Constants.AFT_DME2_CONTAINER_ENV_KEY));
String resRO= config.getProperty(config.getProperty(DME2Constants.AFT_DME2_CONTAINER_ROUTEOFFER_KEY));
if(resName != null && resVer != null && resRO != null && resEnv != null) {
return resName + "/" + resVer + "/" + resEnv + "/" + resRO;
}
}
else {
return null;
}
return null;
}
public static Object loadClass(DME2Configuration config, String url, String handlerName) throws Exception {
Object obj = null;
Class> cls = null;
try {
cls = Thread.currentThread().getContextClassLoader().loadClass(handlerName);
obj = cls.newInstance();
return obj;
} catch (Exception e) {
// if any exception, try loading from ClassLoader
if (config.getBoolean(DME2Constants.DME2_DEBUG)) {
logger.error(null, "loadClass", DME2Constants.AFT_DME2_0712,
new ErrorContext().add("ServerURL", url).add("handlerName", handlerName),
e);
}
}
try {
// Check system classloader
cls = Class.forName(handlerName);
obj = cls.newInstance();
return obj;
} catch (Exception e) {
// if any exception, try loading from ClassLoader
if (config.getBoolean(DME2Constants.DME2_DEBUG)) {
logger.error(null, "loadClass", DME2Constants.AFT_DME2_0712,
new ErrorContext().add("ServerURL", url).add("handlerName", handlerName),
e);
}
}
try {
// try with current class classLoader
cls = DME2Utils.class.getClassLoader().loadClass(handlerName);
obj = cls.newInstance();
return obj;
} catch (Exception e) {
if (config.getBoolean(DME2Constants.DME2_DEBUG)) {
logger.error(null, "loadClass", DME2Constants.AFT_DME2_0712,
new ErrorContext().add("ServerURL", url).add("handlerName", handlerName),
e);
}
throw new DME2Exception(DME2Constants.AFT_DME2_0712,
new ErrorContext().add("ServerURL", url).add("handlerName", handlerName));
}
}
public static String[] getFailoverHandlers(DME2Configuration config, Map headers) {
String defaultHandler = "com.att.aft.dme2.handler.DefaultLoggingFailoverFaultHandler";
Set replyHandlers = new HashSet();
if(headers != null) {
// get the configured handlers from the request
String handlers = headers.get(config.getProperty(DME2Constants.AFT_DME2_EXCHANGE_FAILOVER_HANDLERS_KEY));
if (handlers != null && handlers.length() > 0) {
try {
for (String handler : handlers.split(",")) {
replyHandlers.add(handler);
}
} catch (Exception e) {
logger.debug(null, "getFailoverHandlers", LogMessage.EXCH_READ_HANDLER_FAIL, "getFailoverHandlers", e);
}
}
}
// if we are configured for logging failovers
if (config.getBoolean(DME2Constants.AFT_DME2_ENABLE_FAILOVER_LOGGING)) {
replyHandlers.add(defaultHandler);
}
logger.debug(null, "getFailoverHandlers", "FAILOVER_HANDLERS_CHAIN_HEADER_PROPERTY", replyHandlers.toString());
if (replyHandlers.size() > 0) {
return replyHandlers.toArray(new String[0]);
} else {
return null;
}
}
public static Map getQueryParamsAsMap(String qp){
if(qp == null) {
return new HashMap();
}
else {
String[] qpArr = qp.split("&");
HashMap qpMap = new HashMap();
for(int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy