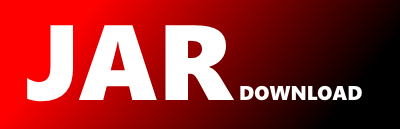
com.att.nsa.data.SaMultiMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of saToolkit Show documentation
Show all versions of saToolkit Show documentation
Library of code used in various service assurance systems
/*******************************************************************************
* Copyright (c) 2016 AT&T Intellectual Property. All rights reserved.
*******************************************************************************/
package com.att.nsa.data;
import java.util.Collection;
import java.util.Hashtable;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
public class SaMultiMap
{
public SaMultiMap ()
{
fMultiMap = new Hashtable> ();
}
public SaMultiMap ( SaMultiMap mm )
{
fMultiMap = new Hashtable> ();
for ( Entry> m : mm.getValues ().entrySet () )
{
fMultiMap.put ( m.getKey(), m.getValue () );
}
}
@Override
public String toString ()
{
return fMultiMap.toString ();
}
public synchronized void put ( K k )
{
getOrCreateFor ( k );
}
public synchronized void put ( K k, V v )
{
LinkedList list = new LinkedList();
list.add ( v );
put ( k, list );
}
public synchronized void put ( K k, Collection v )
{
List itemList = getOrCreateFor ( k );
itemList.removeAll ( v ); // only one of a given value allowed
itemList.addAll ( v );
}
public synchronized boolean containsKey ( K k )
{
return fMultiMap.containsKey ( k );
}
/**
* Get a list of values for the given key. Note this is a copy of the data
* stored in the map -- changes to it do not impact the map.
* @param k
* @return a list of values
*/
public synchronized List get ( K k )
{
List itemList = new LinkedList ();
if ( fMultiMap.containsKey ( k ) )
{
itemList = getOrCreateFor ( k );
}
return itemList;
}
public synchronized Collection getKeys ()
{
return fMultiMap.keySet ();
}
public synchronized Map> getValues ()
{
return fMultiMap;
}
public synchronized List remove ( K k )
{
return fMultiMap.remove ( k );
}
public synchronized void remove ( K k, V v )
{
List itemList = getOrCreateFor ( k );
itemList.remove ( v );
}
public synchronized void clear ()
{
fMultiMap.clear ();
}
public synchronized int size ()
{
return fMultiMap.size ();
}
public synchronized int size ( K k )
{
return getOrCreateFor ( k ).size ();
}
public int valueCount ()
{
int size = 0;
for ( List l : fMultiMap.values () )
{
size += l.size();
}
return size;
}
private final Hashtable> fMultiMap;
private synchronized List getOrCreateFor ( K k )
{
List itemList = fMultiMap.get ( k );
if ( itemList == null )
{
itemList = new LinkedList ();
fMultiMap.put ( k, itemList );
}
return itemList;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy