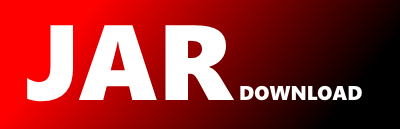
com.att.nsa.util.base64.rrcBase64OutputStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of saToolkit Show documentation
Show all versions of saToolkit Show documentation
Library of code used in various service assurance systems
/*******************************************************************************
* Copyright (c) 2016 AT&T Intellectual Property. All rights reserved.
*******************************************************************************/
/*
* This code was originally pulled from Apache licensed code from Rathravane LLC
*/
package com.att.nsa.util.base64;
import java.io.IOException;
import java.io.OutputStream;
import java.util.LinkedList;
public class rrcBase64OutputStream
extends OutputStream
{
public rrcBase64OutputStream ( OutputStream downstream )
{
fDownstream = downstream;
fPendings = new LinkedList ();
fWrittenToLine = 0;
}
@Override
public void write ( int b )
throws IOException
{
fPendings.add ( (byte) b );
writePendings ( false );
}
@Override
public void close () throws IOException
{
writePendings ( true );
fDownstream.close ();
}
private int writeNow ()
{
int result = 0;
int pending = fPendings.size ();
if ( pending > kBufferSize )
{
result = kBufferSize;
}
return result;
}
private void writePendings ( boolean pad ) throws IOException
{
int thisWrite = fPendings.size ();
if ( !pad )
{
thisWrite = writeNow ();
}
if ( thisWrite > 0 )
{
byte[] bb = new byte [ thisWrite ];
for ( int i=0; i>> 2;
int o1 = ( ( i0 & 3 ) << 4 ) | ( i1 >>> 4 );
int o2 = ( ( i1 & 0xf ) << 2 ) | ( i2 >>> 6 );
int o3 = i2 & 0x3F;
out[op++] = rrcBase64Constants.nibblesToB64[o0];
out[op++] = rrcBase64Constants.nibblesToB64[o1];
out[op] = op < oDataLen ? rrcBase64Constants.nibblesToB64[o2] : '=';
op++;
out[op] = op < oDataLen ? rrcBase64Constants.nibblesToB64[o3] : '=';
op++;
}
return out;
}
private static final int kMaxLine = 80;
private static final int kBufferSize = 3*64; // multiple of 3 for no padding
private OutputStream fDownstream;
private int fWrittenToLine;
private final LinkedList fPendings;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy