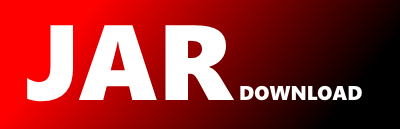
com.att.research.xacml.std.StdRequestAttributes Maven / Gradle / Ivy
/*
* AT&T - PROPRIETARY
* THIS FILE CONTAINS PROPRIETARY INFORMATION OF
* AT&T AND IS NOT TO BE DISCLOSED OR USED EXCEPT IN
* ACCORDANCE WITH APPLICABLE AGREEMENTS.
*
* Copyright (c) 2013 AT&T Knowledge Ventures
* Unpublished and Not for Publication
* All Rights Reserved
*/
package com.att.research.xacml.std;
import java.util.Collection;
import javax.xml.xpath.XPathConstants;
import javax.xml.xpath.XPathExpression;
import javax.xml.xpath.XPathExpressionException;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import com.att.research.xacml.api.Attribute;
import com.att.research.xacml.api.Identifier;
import com.att.research.xacml.api.RequestAttributes;
import com.att.research.xacml.util.ObjUtil;
/**
* Immutable implementation of the {@link com.att.research.xacml.api.RequestAttributes} interface.
*
* @author Christopher A. Rath
* @version $Revision: 1.1 $
*/
public class StdRequestAttributes extends StdAttributeCategory implements RequestAttributes {
private Log logger = LogFactory.getLog(this.getClass());
private Node contentRoot;
private String xmlId;
/**
* Creates a new StdRequestAttributes
with the given {@link com.att.research.xacml.api.Identifier} representing its XACML Category,
* the given Collection
of {@link com.att.research.xacml.api.Attribute}s, the given {@link org.w3c.dom.Node} representing the XACML Content element
* and the given String
as the optional xml:Id.
*
* @param identifierCategory the Identifier
representing the XACML Category for the new StdRequestAttributes
* @param listAttributes the Collection
of Attribute
s included in the new StdRequestAttributes
* @param nodeContentRoot the Node
representing the XACML Content element for the new StdRequestAttributes
* @param xmlIdIn the String
representing the xml:Id of the XACML Attributes element represented by this StdRequestAttributes
*/
public StdRequestAttributes(Identifier identifierCategory, Collection listAttributes, Node nodeContentRoot, String xmlIdIn) {
super(identifierCategory, listAttributes);
this.contentRoot = nodeContentRoot;
this.xmlId = xmlIdIn;
}
/**
* Creates a new StdRequestAttributes
by copying the given {@link com.att.research.xacml.api.RequestAttributes}.
*
* @param requestAttributes the RequestAttributes
to copy
*/
public StdRequestAttributes(RequestAttributes requestAttributes) {
super(requestAttributes);
this.contentRoot = requestAttributes.getContentRoot();
this.xmlId = requestAttributes.getXmlId();
}
@Override
public String getXmlId() {
return this.xmlId;
}
@Override
public Node getContentRoot() {
return this.contentRoot;
}
@Override
public Node getContentNodeByXpathExpression(XPathExpression xpathExpression) {
if (xpathExpression == null) {
throw new NullPointerException("Null XPathExpression");
}
Node nodeRootThis = this.getContentRoot();
if (nodeRootThis == null) {
return null;
}
Node matchingNode = null;
try {
matchingNode = (Node)xpathExpression.evaluate(nodeRootThis, XPathConstants.NODE);
} catch (XPathExpressionException ex) {
this.logger.warn("Failed to retrieve node for \"" + xpathExpression.toString() + "\"", ex);
}
return matchingNode;
}
@Override
public NodeList getContentNodeListByXpathExpression(XPathExpression xpathExpression) {
if (xpathExpression == null) {
throw new NullPointerException("Null XPathExpression");
}
Node nodeRootThis = this.getContentRoot();
if (nodeRootThis == null) {
return null;
}
NodeList matchingNodeList = null;
try {
matchingNodeList = (NodeList)xpathExpression.evaluate(nodeRootThis, XPathConstants.NODESET);
} catch (XPathExpressionException ex) {
this.logger.warn("Failed to retrieve nodelist for \"" + xpathExpression.toString() + "\"", ex);
}
return matchingNodeList;
}
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
} else if (obj == null || !(obj instanceof RequestAttributes)) {
return false;
} else {
RequestAttributes objRequestAttributes = (RequestAttributes)obj;
// Content nodes need to be handled specially because content.equals(content) does not work unless they are the same object, and
// content.isEqualNode(content) only works if the nodes have identical newlines and spaces, which is not what we care about.
if (ObjUtil.xmlEqualsAllowNull(this.getContentRoot(), objRequestAttributes.getContentRoot()) == false) {
return false;
}
return super.equals(objRequestAttributes) &&
ObjUtil.equalsAllowNull(this.getXmlId(), objRequestAttributes.getXmlId());
}
}
@Override
public String toString() {
StringBuilder stringBuilder = new StringBuilder("{");
stringBuilder.append("super=");
stringBuilder.append(super.toString());
Object objectToDump;
if ((objectToDump = this.getContentRoot()) != null) {
stringBuilder.append(',');
stringBuilder.append("contentRoot=");
stringBuilder.append(objectToDump.toString());
}
if ((objectToDump = this.getXmlId()) != null) {
stringBuilder.append(',');
stringBuilder.append("xmlId=");
stringBuilder.append((String)objectToDump);
}
stringBuilder.append('}');
return stringBuilder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy