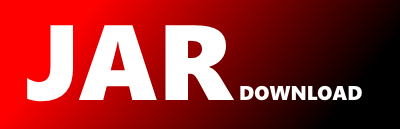
COSE.KeySetCollector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cose-java Show documentation
Show all versions of cose-java Show documentation
A Java implementation that supports the COSE secure message specification.
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy