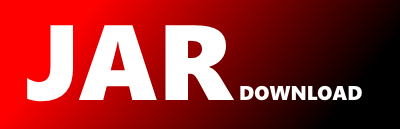
COSE.MacCommon Maven / Gradle / Ivy
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package COSE;
import com.upokecenter.cbor.CBORObject;
import java.nio.ByteBuffer;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
import java.util.Arrays;
import java.util.Formatter;
import javax.crypto.Cipher;
import javax.crypto.Mac;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
/**
*
* @author jimsch
*/
public abstract class MacCommon extends Message {
protected byte[] rgbTag;
protected String strContext;
protected SecureRandom random = new SecureRandom();
protected MacCommon() {
super();
}
protected void CreateWithKey(byte[] rgbKey) throws CoseException {
CBORObject algX = findAttribute(CBORObject.FromObject(1)); //HeaderKeys.Algorithm);
AlgorithmID alg = AlgorithmID.FromCBOR(algX);
if (rgbContent == null) throw new CoseException("No Content Specified");
switch (alg) {
case HMAC_SHA_256_64:
case HMAC_SHA_256:
case HMAC_SHA_384:
case HMAC_SHA_512:
rgbTag = HMAC(alg, rgbKey);
break;
case AES_CBC_MAC_128_64:
case AES_CBC_MAC_256_64:
case AES_CBC_MAC_128_128:
case AES_CBC_MAC_256_128:
rgbTag = AES_CBC_MAC(alg, rgbKey);
break;
default:
throw new CoseException("Unsupported MAC Algorithm");
}
ProcessCounterSignatures();
}
protected boolean Validate(byte[] rgbKey) throws CoseException {
boolean f;
int i;
byte[] rgbTest;
CBORObject algX = findAttribute(CBORObject.FromObject(1)); //HeaderKeys.Algorithm);
AlgorithmID alg = AlgorithmID.FromCBOR(algX);
switch (alg) {
case HMAC_SHA_256_64:
case HMAC_SHA_256:
case HMAC_SHA_384:
case HMAC_SHA_512:
rgbTest = HMAC(alg, rgbKey);
break;
case AES_CBC_MAC_128_64:
case AES_CBC_MAC_256_64:
case AES_CBC_MAC_128_128:
case AES_CBC_MAC_256_128:
rgbTest = AES_CBC_MAC(alg, rgbKey);
break;
default:
throw new CoseException("Unsupported MAC Algorithm");
}
if (rgbTest.length != rgbTag.length) return false;
f = true;
for (i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy