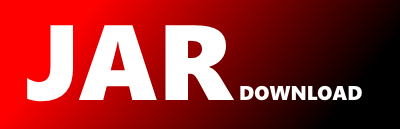
com.auth0.web.Auth0User Maven / Gradle / Ivy
package com.auth0.web;
import com.auth0.authentication.result.UserIdentity;
import com.auth0.authentication.result.UserProfile;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import java.io.Serializable;
import java.security.Principal;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.Map;
/**
* Auth0User represents the User Profile
* and implements Principal interface
*/
public class Auth0User implements Principal, Serializable {
private static final long serialVersionUID = 2371882820082543721L;
private String userId;
private String name;
private String nickname;
private String picture;
private String email;
private boolean emailVerified;
private String givenName;
private String familyName;
private Map userMetadata;
private Map appMetadata;
private Date createdAt;
private List identities;
private Map extraInfo;
private List roles = new ArrayList();
private List groups = new ArrayList();
public Auth0User(final UserProfile userProfile) {
// for now, copy out attributes from userProfile
this.userId = userProfile.getId();
this.name = userProfile.getName();
this.nickname = userProfile.getNickname();
this.picture = userProfile.getPictureURL();
this.email = userProfile.getEmail();
this.emailVerified = userProfile.isEmailVerified();
this.givenName = userProfile.getGivenName();
this.familyName = userProfile.getFamilyName();
this.userMetadata = userProfile.getUserMetadata();
this.appMetadata = userProfile.getAppMetadata();
this.createdAt = userProfile.getCreatedAt();
this.identities = userProfile.getIdentities();
this.extraInfo = userProfile.getExtraInfo();
if (extraInfo != null && extraInfo.containsKey("roles")) {
roles = (List) extraInfo.get("roles");
}
if (extraInfo != null && extraInfo.containsKey("groups")) {
groups = (List) extraInfo.get("groups");
}
}
public String getUserId() {
return userId;
}
@Override
public String getName() {
return name;
}
public String getNickname() {
return nickname;
}
public String getPicture() {
return picture;
}
public String getEmail() {
return email;
}
public boolean isEmailVerified() {
return emailVerified;
}
public String getGivenName() {
return givenName;
}
public String getFamilyName() {
return familyName;
}
public Map getUserMetadata() {
return userMetadata;
}
public Map getAppMetadata() {
return appMetadata;
}
public Date getCreatedAt() {
return createdAt;
}
public List getRoles() {
return roles;
}
public List getGroups() {
return groups;
}
public List getIdentities() {
return identities;
}
public Map getExtraInfo() {
return extraInfo;
}
public boolean equals(Object obj) {
if (obj == null) { return false; }
if (obj == this) { return true; }
if (obj.getClass() != getClass()) {
return false;
}
Auth0User rhs = (Auth0User) obj;
return new EqualsBuilder()
.appendSuper(super.equals(obj))
.append(userId, rhs.userId)
.isEquals();
}
public int hashCode() {
return new HashCodeBuilder(17, 37).
append(userId).
toHashCode();
}
public String toString() {
return new ToStringBuilder(this).
append("userId", userId).
append("name", name).
append("email", email).
toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy