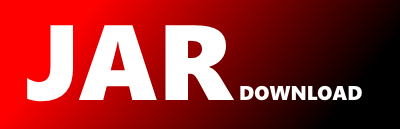
com.auth0.jwk.UrlJwkProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jwks-rsa Show documentation
Show all versions of jwks-rsa Show documentation
JSON Web Key Set parser library
package com.auth0.jwk;
import com.fasterxml.jackson.core.JsonFactory;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.common.annotations.VisibleForTesting;
import com.google.common.collect.Lists;
import java.io.IOException;
import java.io.InputStream;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.List;
import java.util.Map;
import static com.google.common.base.Preconditions.checkArgument;
import static com.google.common.base.Strings.isNullOrEmpty;
/**
* Jwk provider that loads them from a {@link URL}
*/
@SuppressWarnings("WeakerAccess")
public class UrlJwkProvider implements JwkProvider {
@VisibleForTesting
static final String WELL_KNOWN_JWKS_PATH = "/.well-known/jwks.json";
final URL url;
/**
* Creates a provider that loads from the given URL
* @param url to load the jwks
*/
public UrlJwkProvider(URL url) {
checkArgument(url != null, "A non-null url is required");
this.url = url;
}
/**
* Creates a provider that loads from the given domain's well-known directory.
*
It can be a url link 'https://samples.auth0.com' or just a domain 'samples.auth0.com'.
* If the protocol (http or https) is not provided then https is used by default.
* The default jwks path "/.well-known/jwks.json" is appended to the given string domain.
*
For example, when the domain is "samples.auth0.com"
* the jwks url that will be used is "https://samples.auth0.com/.well-known/jwks.json"
*
Use {@link #UrlJwkProvider(URL)} if you need to pass a full URL.
* @param domain where jwks is published
*/
public UrlJwkProvider(String domain) {
this(urlForDomain(domain));
}
static URL urlForDomain(String domain) {
checkArgument(!isNullOrEmpty(domain), "A domain is required");
if (!domain.startsWith("http")) {
domain = "https://" + domain;
}
try {
final URL url = new URL(domain);
return new URL(url, WELL_KNOWN_JWKS_PATH);
} catch (MalformedURLException e) {
throw new IllegalArgumentException("Invalid jwks uri", e);
}
}
private Map getJwks() throws SigningKeyNotFoundException {
try {
final InputStream inputStream = this.url.openStream();
final JsonFactory factory = new JsonFactory();
final JsonParser parser = factory.createParser(inputStream);
final TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy