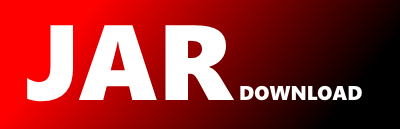
com.authlete.client.jaxrs.api.AuthleteApiImpl Maven / Gradle / Ivy
/*
* Copyright (C) 2014-2015 Authlete, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.authlete.client.jaxrs.api;
import static javax.ws.rs.core.HttpHeaders.AUTHORIZATION;
import static javax.ws.rs.core.MediaType.APPLICATION_JSON_TYPE;
import java.util.List;
import java.util.Map;
import javax.ws.rs.WebApplicationException;
import javax.ws.rs.client.ClientBuilder;
import javax.ws.rs.client.Entity;
import javax.ws.rs.client.ResponseProcessingException;
import javax.ws.rs.client.WebTarget;
import javax.ws.rs.core.Response;
import javax.ws.rs.core.Response.StatusType;
import com.authlete.common.api.AuthleteApi;
import com.authlete.common.api.AuthleteApiException;
import com.authlete.common.conf.AuthleteConfiguration;
import com.authlete.common.dto.AuthorizationFailRequest;
import com.authlete.common.dto.AuthorizationFailResponse;
import com.authlete.common.dto.AuthorizationIssueRequest;
import com.authlete.common.dto.AuthorizationIssueResponse;
import com.authlete.common.dto.AuthorizationRequest;
import com.authlete.common.dto.AuthorizationResponse;
import com.authlete.common.dto.Client;
import com.authlete.common.dto.ClientListResponse;
import com.authlete.common.dto.IntrospectionRequest;
import com.authlete.common.dto.IntrospectionResponse;
import com.authlete.common.dto.Service;
import com.authlete.common.dto.ServiceListResponse;
import com.authlete.common.dto.TokenCreateRequest;
import com.authlete.common.dto.TokenCreateResponse;
import com.authlete.common.dto.TokenFailRequest;
import com.authlete.common.dto.TokenFailResponse;
import com.authlete.common.dto.TokenIssueRequest;
import com.authlete.common.dto.TokenIssueResponse;
import com.authlete.common.dto.TokenRequest;
import com.authlete.common.dto.TokenResponse;
import com.authlete.common.dto.UserInfoIssueRequest;
import com.authlete.common.dto.UserInfoIssueResponse;
import com.authlete.common.dto.UserInfoRequest;
import com.authlete.common.dto.UserInfoResponse;
import com.authlete.common.web.BasicCredentials;
/**
* The implementation of {@link AuthleteApi} using JAX-RS 2.0 client API.
*
* @author Takahiko Kawasaki
*/
public class AuthleteApiImpl implements AuthleteApi
{
private interface AuthleteApiCall
{
TResponse call();
}
private static final String AUTH_AUTHORIZATION_API_PATH = "/api/auth/authorization";
private static final String AUTH_AUTHORIZATION_FAIL_API_PATH = "/api/auth/authorization/fail";
private static final String AUTH_AUTHORIZATION_ISSUE_API_PATH = "/api/auth/authorization/issue";
private static final String AUTH_TOKEN_API_PATH = "/api/auth/token";
private static final String AUTH_TOKEN_CREATE_API_PATH = "/api/auth/token/create";
private static final String AUTH_TOKEN_FAIL_API_PATH = "/api/auth/token/fail";
private static final String AUTH_TOKEN_ISSUE_API_PATH = "/api/auth/token/issue";
private static final String AUTH_USERINFO_API_PATH = "/api/auth/userinfo";
private static final String AUTH_USERINFO_ISSUE_API_PATH = "/api/auth/userinfo/issue";
private static final String AUTH_INTROSPECTION_API_PATH = "/api/auth/introspection";
private static final String SERVICE_CREATE_API_PATH = "/api/service/create";
private static final String SERVICE_DELETE_API_PATH = "/api/service/delete/%d";
private static final String SERVICE_GET_API_PATH = "/api/service/get/%d";
private static final String SERVICE_GET_LIST_API_PATH = "/api/service/get/list";
private static final String SERVICE_JWKS_GET_API_PATH = "/api/service/jwks/get";
private static final String SERVICE_UPDATE_API_PATH = "/api/service/update/%d";
private static final String CLIENT_CREATE_API_PATH = "/api/client/create";
private static final String CLIENT_DELETE_API_PATH = "/api/client/delete/%d";
private static final String CLIENT_GET_API_PATH = "/api/client/get/%d";
private static final String CLIENT_GET_LIST_API_PATH = "/api/client/get/list";
private static final String CLIENT_UPDATE_API_PATH = "/api/client/update/%d";
private final WebTarget mTarget;
private final String mServiceOwnerAuth;
private final String mServiceAuth;
/**
* The constructor with an instance of {@link AuthleteConfiguration}.
*
*
* The existence of a constructor of this type is a required by
* {@link com.authlete.common.api.AuthleteApiFactory AuthleteApiFactory}.
*
*
* @param configuration
* An instance of {@link AuthleteConfiguration}.
*/
public AuthleteApiImpl(AuthleteConfiguration configuration)
{
if (configuration == null)
{
throw new IllegalArgumentException("configuration is null.");
}
mTarget = ClientBuilder.newClient().target(configuration.getBaseUrl());
mServiceOwnerAuth = createServiceOwnerCredentials(configuration).format();
mServiceAuth = createServiceCredentials(configuration).format();
}
/**
* Create a {@link BasicCredentials} for the service owner.
*/
private BasicCredentials createServiceOwnerCredentials(AuthleteConfiguration configuration)
{
String key = configuration.getServiceOwnerApiKey();
String secret = configuration.getServiceOwnerApiSecret();
return new BasicCredentials(key, secret);
}
/**
* Create a {@link BasicCredentials} for the service.
*/
private BasicCredentials createServiceCredentials(AuthleteConfiguration configuration)
{
String key = configuration.getServiceApiKey();
String secret = configuration.getServiceApiSecret();
return new BasicCredentials(key, secret);
}
/**
* Execute an Authlete API call.
*/
private TResponse executeApiCall(AuthleteApiCall apiCall) throws AuthleteApiException
{
try
{
// Call the Authlete API.
return apiCall.call();
}
catch (WebApplicationException e)
{
// Throw an exception with HTTP response information.
throw createApiException(e, e.getResponse());
}
catch (ResponseProcessingException e)
{
// Throw an exception with HTTP response information.
throw createApiException(e, e.getResponse());
}
catch (Throwable t)
{
// Throw an exception without HTTP response information.
throw createApiException(t, null);
}
}
/**
* Create an {@link AuthleteApiException} instance.
*/
private AuthleteApiException createApiException(Throwable cause, Response response)
{
// Error message.
String message = cause.getMessage();
if (response == null)
{
// Create an exception without HTTP response information.
return new AuthleteApiException(message, cause);
}
// Status code and status message.
int statusCode = 0;
String statusMessage = null;
// Get the status information.
StatusType type = response.getStatusInfo();
if (type != null)
{
statusCode = type.getStatusCode();
statusMessage = type.getReasonPhrase();
}
// Response body.
String responseBody = null;
// If the response has response body.
if (response.hasEntity())
{
// Get the response body.
responseBody = extractResponseBody(response);
}
// Response headers.
Map> headers = response.getStringHeaders();
// Create an exception with HTTP response information.
return new AuthleteApiException(message, cause, statusCode, statusMessage, responseBody, headers);
}
private String extractResponseBody(Response response)
{
try
{
// Convert the entity body into a String.
return response.readEntity(String.class);
}
catch (Exception e)
{
// Failed to convert the entity body into a String.
e.printStackTrace();
// Response body is not available.
return null;
}
}
private TResponse callGetApi(String auth, String path, Class responseClass)
{
return mTarget
.path(path)
.request(APPLICATION_JSON_TYPE)
.header(AUTHORIZATION, auth)
.get(responseClass);
}
private TResponse callServiceOwnerGetApi(String path, Class responseClass)
{
return callGetApi(mServiceOwnerAuth, path, responseClass);
}
private TResponse callServiceGetApi(String path, Class responseClass)
{
return callGetApi(mServiceAuth, path, responseClass);
}
private Void callDeleteApi(String auth, String path)
{
mTarget
.path(path)
.request()
.header(AUTHORIZATION, auth)
.delete();
return null;
}
private Void callServiceOwnerDeleteApi(String path)
{
return callDeleteApi(mServiceOwnerAuth, path);
}
private Void callServiceDeleteApi(String path)
{
return callDeleteApi(mServiceAuth, path);
}
private TResponse callPostApi(String auth, String path, Object request, Class responseClass)
{
return mTarget
.path(path)
.request(APPLICATION_JSON_TYPE)
.header(AUTHORIZATION, auth)
.post(Entity.entity(request, APPLICATION_JSON_TYPE), responseClass);
}
private TResponse callServiceOwnerPostApi(String path, Object request, Class responseClass)
{
return callPostApi(mServiceOwnerAuth, path, request, responseClass);
}
private TResponse callServicePostApi(String path, Object request, Class responseClass)
{
return callPostApi(mServiceAuth, path, request, responseClass);
}
@Override
public AuthorizationResponse authorization(final AuthorizationRequest request) throws AuthleteApiException
{
return executeApiCall(new AuthleteApiCall() {
@Override
public AuthorizationResponse call()
{
return callServicePostApi(AUTH_AUTHORIZATION_API_PATH, request, AuthorizationResponse.class);
}
});
}
@Override
public AuthorizationFailResponse authorizationFail(final AuthorizationFailRequest request) throws AuthleteApiException
{
return executeApiCall(new AuthleteApiCall() {
@Override
public AuthorizationFailResponse call()
{
return callServicePostApi(AUTH_AUTHORIZATION_FAIL_API_PATH, request, AuthorizationFailResponse.class);
}
});
}
@Override
public AuthorizationIssueResponse authorizationIssue(final AuthorizationIssueRequest request) throws AuthleteApiException
{
return executeApiCall(new AuthleteApiCall() {
@Override
public AuthorizationIssueResponse call()
{
return callServicePostApi(AUTH_AUTHORIZATION_ISSUE_API_PATH, request, AuthorizationIssueResponse.class);
}
});
}
@Override
public TokenResponse token(final TokenRequest request) throws AuthleteApiException
{
return executeApiCall(new AuthleteApiCall() {
@Override
public TokenResponse call()
{
return callServicePostApi(AUTH_TOKEN_API_PATH, request, TokenResponse.class);
}
});
}
@Override
public TokenCreateResponse tokenCreate(final TokenCreateRequest request) throws AuthleteApiException
{
return executeApiCall(new AuthleteApiCall() {
@Override
public TokenCreateResponse call()
{
return callServicePostApi(AUTH_TOKEN_CREATE_API_PATH, request, TokenCreateResponse.class);
}
});
}
@Override
public TokenFailResponse tokenFail(final TokenFailRequest request) throws AuthleteApiException
{
return executeApiCall(new AuthleteApiCall() {
@Override
public TokenFailResponse call()
{
return callServicePostApi(AUTH_TOKEN_FAIL_API_PATH, request, TokenFailResponse.class);
}
});
}
@Override
public TokenIssueResponse tokenIssue(final TokenIssueRequest request) throws AuthleteApiException
{
return executeApiCall(new AuthleteApiCall() {
@Override
public TokenIssueResponse call()
{
return callServicePostApi(AUTH_TOKEN_ISSUE_API_PATH, request, TokenIssueResponse.class);
}
});
}
@Override
public UserInfoResponse userinfo(final UserInfoRequest request) throws AuthleteApiException
{
return executeApiCall(new AuthleteApiCall() {
@Override
public UserInfoResponse call()
{
return callServicePostApi(AUTH_USERINFO_API_PATH, request, UserInfoResponse.class);
}
});
}
@Override
public UserInfoIssueResponse userinfoIssue(final UserInfoIssueRequest request) throws AuthleteApiException
{
return executeApiCall(new AuthleteApiCall() {
@Override
public UserInfoIssueResponse call()
{
return callServicePostApi(AUTH_USERINFO_ISSUE_API_PATH, request, UserInfoIssueResponse.class);
}
});
}
@Override
public IntrospectionResponse introspection(final IntrospectionRequest request) throws AuthleteApiException
{
return executeApiCall(new AuthleteApiCall() {
@Override
public IntrospectionResponse call()
{
return callServicePostApi(AUTH_INTROSPECTION_API_PATH, request, IntrospectionResponse.class);
}
});
}
@Override
public Service createServie(final Service service) throws AuthleteApiException
{
return executeApiCall(new AuthleteApiCall() {
@Override
public Service call()
{
return callServiceOwnerPostApi(SERVICE_CREATE_API_PATH, service, Service.class);
}
});
}
@Override
public void deleteService(final long apiKey) throws AuthleteApiException
{
executeApiCall(new AuthleteApiCall() {
@Override
public Void call()
{
String path = String.format(SERVICE_DELETE_API_PATH, apiKey);
return callServiceOwnerDeleteApi(path);
}
});
}
@Override
public Service getService(final long apiKey) throws AuthleteApiException
{
return executeApiCall(new AuthleteApiCall() {
@Override
public Service call()
{
String path = String.format(SERVICE_GET_API_PATH, apiKey);
return callServiceOwnerGetApi(path, Service.class);
}
});
}
@Override
public ServiceListResponse getServiceList() throws AuthleteApiException
{
return getServiceList(0, 0, false);
}
@Override
public ServiceListResponse getServiceList(int start, int end) throws AuthleteApiException
{
return getServiceList(start, end, true);
}
private ServiceListResponse getServiceList(
final int start, final int end, final boolean rangeGiven) throws AuthleteApiException
{
return executeApiCall(new AuthleteApiCall() {
@Override
public ServiceListResponse call()
{
return callGetServiceList(start, end, rangeGiven);
}
});
}
/**
* Call /service/get/list
.
*/
private ServiceListResponse callGetServiceList(int start, int end, boolean rangeGiven)
{
WebTarget target = mTarget.path(SERVICE_GET_LIST_API_PATH);
if (rangeGiven)
{
target = target.queryParam("start", start).queryParam("end", end);
}
return target
.request(APPLICATION_JSON_TYPE)
.header(AUTHORIZATION, mServiceOwnerAuth)
.get(ServiceListResponse.class);
}
@Override
public Service updateService(final Service service) throws AuthleteApiException
{
return executeApiCall(new AuthleteApiCall() {
@Override
public Service call()
{
String path = String.format(SERVICE_UPDATE_API_PATH, service.getApiKey());
return callServiceOwnerPostApi(path, service, Service.class);
}
});
}
@Override
public String getServiceJwks() throws AuthleteApiException
{
return executeApiCall(new AuthleteApiCall() {
@Override
public String call()
{
return callServiceGetApi(SERVICE_JWKS_GET_API_PATH, String.class);
}
});
}
@Override
public Client createClient(final Client client) throws AuthleteApiException
{
return executeApiCall(new AuthleteApiCall() {
@Override
public Client call()
{
return callServicePostApi(CLIENT_CREATE_API_PATH, client, Client.class);
}
});
}
@Override
public void deleteClient(final long clientId) throws AuthleteApiException
{
executeApiCall(new AuthleteApiCall() {
@Override
public Void call()
{
String path = String.format(CLIENT_DELETE_API_PATH, clientId);
return callServiceDeleteApi(path);
}
});
}
@Override
public Client getClient(final long clientId) throws AuthleteApiException
{
return executeApiCall(new AuthleteApiCall() {
@Override
public Client call()
{
String path = String.format(CLIENT_GET_API_PATH, clientId);
return callServiceGetApi(path, Client.class);
}
});
}
@Override
public ClientListResponse getClientList() throws AuthleteApiException
{
return getClientList(null, 0, 0, false);
}
@Override
public ClientListResponse getClientList(String developer) throws AuthleteApiException
{
return getClientList(developer, 0, 0, false);
}
@Override
public ClientListResponse getClientList(int start, int end) throws AuthleteApiException
{
return getClientList(null, start, end, true);
}
@Override
public ClientListResponse getClientList(String developer, int start, int end) throws AuthleteApiException
{
return getClientList(developer, start, end, true);
}
private ClientListResponse getClientList(
final String developer, final int start, final int end, final boolean rangeGiven) throws AuthleteApiException
{
return executeApiCall(new AuthleteApiCall() {
@Override
public ClientListResponse call()
{
return callGetClientList(developer, start, end, rangeGiven);
}
});
}
private ClientListResponse callGetClientList(String developer, int start, int end, boolean rangeGiven)
{
WebTarget target = mTarget.path(CLIENT_GET_LIST_API_PATH);
if (developer != null)
{
target = target.queryParam("developer", developer);
}
if (rangeGiven)
{
target = target.queryParam("start", start).queryParam("end", end);
}
return target
.request(APPLICATION_JSON_TYPE)
.header(AUTHORIZATION, mServiceAuth)
.get(ClientListResponse.class);
}
@Override
public Client updateClient(final Client client) throws AuthleteApiException
{
return executeApiCall(new AuthleteApiCall() {
@Override
public Client call()
{
String path = String.format(CLIENT_UPDATE_API_PATH, client.getClientId());
return callServicePostApi(path, client, Client.class);
}
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy