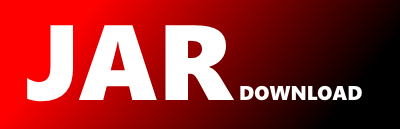
com.authlete.common.web.BearerToken Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of authlete-java-common Show documentation
Show all versions of authlete-java-common Show documentation
Authlete Java library used commonly by service implementations and the Authlete server.
/*
* Copyright (C) 2014 Authlete, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.authlete.common.web;
import java.io.UnsupportedEncodingException;
import java.net.URLDecoder;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* Utility class for Bearer Token defined in
* RFC 6750.
*
* @author Takahiko Kawasaki
*
* @see RFC 6750 (OAuth 2.0 Bearer Token Usage)
*/
public class BearerToken
{
/**
* Regular expression to parse {@code Authorization} header.
*/
private static final Pattern CHALLENGE_PATTERN
= Pattern.compile("^Bearer *([^ ]+) *$", Pattern.CASE_INSENSITIVE);
private BearerToken()
{
}
/**
* Extract the access token embedded in the input string.
*
*
* This method assumes that the input string comes from one of
* the following three places that are mentioned in "RFC 6750
* (OAuth 2.0 Bearer Token Usage),
* 2. Authenticated Requests".
*
*
*
*
*
*
*
* To be concrete, this method assumes that the format of the
* input string is either of the following two.
*
*
*
*
* "Bearer {access-token}"
* - Parameters formatted in
application/x-www-form-urlencoded
* containing access_token={access-token}
.
*
*
*
*
* For example, both {@link #parse(String) parse} method calls below
* return "hello-world"
.
*
*
*
* BearerToken.parse("Bearer hello-world");
* BearerToken.parse("key1=value1&access_token=hello-world");
*
*
* @param input
* The input string to be parsed.
*
* @return
* The extracted access token, or null
if not found.
*
* @see RFC 6750 (OAuth 2.0 Bearer Token Usage)
*/
public static String parse(String input)
{
if (input == null)
{
return null;
}
// First, check whether the input matches the pattern
// "Bearer {access-token}".
Matcher matcher = CHALLENGE_PATTERN.matcher(input);
// If the input matches the pattern.
if (matcher.matches())
{
// Return the value as is. Note that it is not Base64-encoded.
// See https://www.ietf.org/mail-archive/web/oauth/current/msg08489.html
return matcher.group(1);
}
else
{
// Assume that the input is formatted in
// application/x-www-form-urlencoded.
return extractFromFormParameters(input);
}
}
private static String extractFromFormParameters(String input)
{
for (String parameter : input.split("&"))
{
String[] pair = parameter.split("=", 2);
if (pair == null || pair.length != 2 || pair[1].length() == 0)
{
continue;
}
if (pair[0].equals("access_token") == false)
{
continue;
}
try
{
// URL-decode
return URLDecoder.decode(pair[1], "UTF-8");
}
catch (UnsupportedEncodingException e)
{
// This won't happen.
return null;
}
}
// Not found.
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy