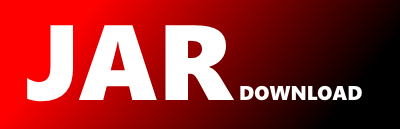
com.authlete.common.assurance.constraint.BaseConstraint Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of authlete-java-common Show documentation
Show all versions of authlete-java-common Show documentation
Authlete Java library used commonly by service implementations and the Authlete server.
/*
* Copyright (C) 2019 Authlete, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific
* language governing permissions and limitations under the
* License.
*/
package com.authlete.common.assurance.constraint;
import java.util.LinkedHashMap;
import java.util.Map;
/**
* The base class for classes that represent constraints in
* {@code verified_claims}.
*
*
* Note that {@link EvidenceArrayConstraint} and {@link ClaimsConstraint}
* don't inherit this class. They implement the {@link Constraint}
* interface by themselves.
*
*
* @see OpenID Connect for Identity Assurance 1.0
*
* @since 2.63
*/
public class BaseConstraint implements Constraint
{
private boolean exists;
private boolean isNull;
@Override
public boolean exists()
{
return exists;
}
/**
* Set the existence of the constraint.
*
* @param exists
* {@code true} to indicate that the constraint exists.
*/
public void setExists(boolean exists)
{
this.exists = exists;
}
@Override
public boolean isNull()
{
return isNull;
}
/**
* Set the boolean flag that indicates that the value of the constraint is null.
*
* @param isNull
* {@code true} to indicate that the value of the constraint is null.
*/
public void setNull(boolean isNull)
{
this.isNull = isNull;
}
/**
* Create a {@code Map} instance that represents this object in the way
* conforming to the structure defined in 5. Requesting Verified Claims of OpenID Connect for Identity Assurance 1.0.
*
* @return
* A {@code Map} instance that represents this object.
* If {@link #exists()} returns {@code false} or {@link #isNull()}
* returns {@code true}, this method returns null.
*/
public Map toMap()
{
if (!exists || isNull)
{
return null;
}
return new LinkedHashMap();
}
static void addIfAvailable(Map map, String name, BaseConstraint constraint)
{
if (constraint != null && constraint.exists())
{
map.put(name, constraint.toMap());
}
}
/**
* Convert this object into JSON in the way conforming to the structure
* defined in 5. Requesting Verified Claims of OpenID Connect for Identity Assurance 1.0.
*
*
* This method is an alias of {@link #toJson(boolean) toJson}{@code (false)}.
*
*
* @return
* JSON that represents this object. If {@link #toMap()} returns
* null, this method returns {@code "null"} (a {@code String}
* instance which consists of {@code 'n'}, {@code 'u'}, {@code 'l'}
* and {@code 'l'}).
*/
public String toJson()
{
return Helper.toJson(toMap());
}
/**
* Convert this object into JSON in the way conforming to the structure
* defined in 5. Requesting Verified Claims of OpenID Connect for Identity Assurance 1.0.
*
* @param pretty
* {@code true} to make the output more human-readable.
*
* @return
* JSON that represents this object. If {@link #toMap()} returns
* null, this method returns {@code "null"} (a {@code String}
* instance which consists of {@code 'n'}, {@code 'u'}, {@code 'l'}
* and {@code 'l'}).
*/
public String toJson(boolean pretty)
{
return Helper.toJson(toMap(), pretty);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy