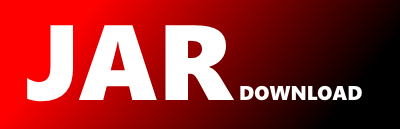
com.authlete.common.dto.IntrospectionResponse Maven / Gradle / Ivy
Show all versions of authlete-java-common Show documentation
/*
* Copyright (C) 2014-2024 Authlete, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.authlete.common.dto;
import java.net.URI;
import com.authlete.common.types.GrantType;
import com.authlete.common.util.Utils;
/**
* Response from Authlete's {@code /auth/introspection} API.
*
*
* Authlete's {@code /auth/introspection} API returns JSON which can
* be mapped to this class. The service implementation should retrieve
* the value of {@code "action"} from the response and take the following
* steps according to the value.
*
*
*
* - {@link Action#INTERNAL_SERVER_ERROR INTERNAL_SERVER_ERROR}
* -
*
* When the value of {@code "action"} is {@code "INTERNAL_SERVER_ERROR"},
* it means that the request from the service implementation was wrong or
* that an error occurred in Authlete.
*
*
*
* In either case, from the viewpoint of the client application, it is an
* error on the server side. Therefore, the service implementation should
* generate a response to the client application with the HTTP status of
* {@code "500 Internal Server Error"}.
*
*
*
* {@link #getResponseContent()} returns a string which describes the error
* in the format of RFC 6750
* (OAuth 2.0 Bearer Token Usage), so if the protected resource of the service
* implementation wants to return an error response to the client application
* in the way that complies with RFC 6750, the string returned from {@link
* #getResponseContent()} can be used as the value of {@code WWW-Authenticate}.
*
*
*
* The following is an example response which complies with RFC 6750.
*
*
*
* HTTP/1.1 500 Internal Server Error
* WWW-Authenticate: (The value returned from {@link #getResponseContent()})
* Cache-Control: no-store
* Pragma: no-cache
*
*
* - {@link Action#BAD_REQUEST BAD_REQUEST}
* -
*
* When the value of {@code "action"} is {@code "BAD_REQUEST"}, it means
* that the request from the client application does not contain an access
* token (= the request from the service implementation to Authlete does
* not contain {@code "token"} parameter).
*
*
*
* {@link #getResponseContent()} returns a string which describes the error
* in the format of RFC 6750
* (OAuth 2.0 Bearer Token Usage), so if the protected resource of the service
* implementation wants to return an error response to the client application
* in the way that complies with RFC 6750, the string returned from {@link
* #getResponseContent()} can be used as the value of {@code WWW-Authenticate}.
*
*
*
* The following is an example response which complies with RFC 6750.
*
*
*
* HTTP/1.1 400 Bad Request
* WWW-Authenticate: (The value returned from {@link #getResponseContent()})
* Cache-Control: no-store
* Pragma: no-cache
*
*
* - {@link Action#UNAUTHORIZED UNAUTHORIZED}
* -
*
* When the value of {@code "action"} is {@code "UNAUTHORIZED"}, it means
* that the access token does not exist or has expired. Or the client
* application associated with the access token does not exist any longer.
*
*
*
* {@link #getResponseContent()} returns a string which describes the error
* in the format of RFC 6750
* (OAuth 2.0 Bearer Token Usage), so if the protected resource of the service
* implementation wants to return an error response to the client application
* in the way that complies with RFC 6750, the string returned from {@link
* #getResponseContent()} can be used as the value of {@code WWW-Authenticate}.
*
*
*
* The following is an example response which complies with RFC 6750.
*
*
*
* HTTP/1.1 401 Unauthorized
* WWW-Authenticate: (The value returned from {@link #getResponseContent()})
* Cache-Control: no-store
* Pragma: no-cache
*
*
* - {@link Action#FORBIDDEN FORBIDDEN}
* -
*
* When the value of {@code "action"} is {@code "FORBIDDEN"}, it means
* that the access token does not cover the required scopes or that the
* subject associated with the access token is different from the subject
* contained in the request.
*
*
*
* {@link #getResponseContent()} returns a string which describes the error
* in the format of RFC 6750
* (OAuth 2.0 Bearer Token Usage), so if the protected resource of the service
* implementation wants to return an error response to the client application
* in the way that complies with RFC 6750, the string returned from {@link
* #getResponseContent()} can be used as the value of {@code WWW-Authenticate}.
*
*
*
* The following is an example response which complies with RFC 6750.
*
*
*
* HTTP/1.1 403 Forbidden
* WWW-Authenticate: (The value returned from {@link #getResponseContent()})
* Cache-Control: no-store
* Pragma: no-cache
*
*
* - {@link Action#OK OK}
* -
*
* When the value of {@code "action"} is {@code "OK"}, it means that the
* access token which the client application presented is valid (= exists
* and has not expired).
*
*
*
* The service implementation is supposed to return the protected resource
* to the client application.
*
*
*
* When {@code "action"} is {@code "OK"}, {@link #getResponseContent()}
* returns {@code "Bearer error=\"invalid_request\""}. This is the
* simplest string which can be used as the value of {@code WWW-Authenticate}
* header to indicate {@code "400 Bad Request"}. The service implementation
* may use this string to tell the client application that the request was
* bad. But in such a case, the service should generate a more informative
* error message to help developers of client applications.
*
*
*
* The following is an example error response which complies with RFC 6750.
*
*
*
* HTTP/1.1 400 Bad Request
* WWW-Authenticate: (The value returned from {@link #getResponseContent()})
* Cache-Control: no-store
* Pragma: no-cache
*
*
*
*
* Basically, {@link #getResponseContent()} returns a string which describes
* the error in the format of RFC 6750 (OAuth 2.0 Bearer Token Usage). So, if the service has
* selected {@code "Bearer"} as the token type, the string returned from
* {@link #getResponseContent()} can be used directly as the value for
* {@code WWW-Authenticate}. However, if the service has selected another
* different token type, the service has to generate error messages for
* itself.
*
*
* JWT-based access token
*
*
* Since version 2.1, Authlete provides a feature to issue access tokens in
* JWT format. This feature can be enabled by setting a non-null value to the
* {@code accessTokenSignAlg} property of the service (see the description of
* the {@link Service} class for details). {@code /auth/introspection} API
* can accept access tokens in JWT format. However, note that the API does not
* return information contained in a given JWT-based access token but returns
* information stored in the database record which corresponds to the given
* JWT-based access token. Because attributes of the database record can be
* modified after the access token is issued (for example, by using {@code
* /auth/token/update} API), information returned by {@code
* /auth/introspection} API and information the given JWT-based access
* token holds may be different.
*
*
* HTTP Message Signatures
*
*
* If the {@code responseSigningRequired} response parameter from the API is
* {@code true}, the response from the protected resource endpoint must contain
* the {@code Signature} and {@code Signature-Input} HTTP fields (cf. RFC 9421 HTTP Message
* Signatures) that comply with FAPI 2.0
* Message Signing.
*
*
* DPoP Nonce (Authlete 3.0 onwards)
*
*
* Since version 3.0, Authlete recognizes the {@code nonce} claim in DPoP
* proof JWTs. If the presented access token is bound to a public key through
* the DPoP mechanism, and if the {@code nonce} claim is required (= if the
* service's {@code dpopNonceRequired} property is {@code true}, or the value
* of the {@code dpopNonceRequired} request parameter passed to the Authlete
* API is {@code true}), the Authlete API checks whether the {@code nonce}
* claim in the presented DPoP proof JWT is identical to the expected value.
*
*
*
* If the {@code dpopNonce} response parameter from the API is not null, its
* value is the expected nonce value for DPoP proof JWT. The expected value
* needs to be conveyed to the client application as the value of the
* {@code DPoP-Nonce} HTTP header.
*
*
* DPoP-Nonce: (The value returned from {@link #getDpopNonce()})
*
*
* See RFC 9449 OAuth
* 2.0 Demonstrating Proof of Possession (DPoP) for details.
*
*
* @author Takahiko Kawasaki
*
* @see RFC 9421 HTTP Message Signatures
*
* @see RFC 9449 OAuth 2.0 Demonstrating Proof of Possession (DPoP)
*
* @see FAPI 2.0 Message Signing
*/
public class IntrospectionResponse extends ApiResponse
{
private static final long serialVersionUID = 23L;
/**
* The next action the service implementation should take.
*/
public enum Action
{
/**
* The request from the service implementation was wrong or
* an error occurred in Authlete. The service implementation
* should return {@code "500 Internal Server Error"} to the
* client application.
*/
INTERNAL_SERVER_ERROR,
/**
* The request does not contain an access token. The service
* implementation should return {@code "400 Bad Request"} to
* the client application.
*/
BAD_REQUEST,
/**
* The access token does not exist or has expired. The service
* implementation should return {@code "401 Unauthorized"} to
* the client application.
*/
UNAUTHORIZED,
/**
* The access token does not cover the required scopes. The
* service implementation should return {@code "403 Forbidden"}
* to the client application.
*/
FORBIDDEN,
/**
* The access token is valid. The service implementation should
* return the protected resource to the client application.
*/
OK
}
private static final String SUMMARY_FORMAT
= "action=%s, clientId=%d, subject=%s, existent=%s, "
+ "usable=%s, sufficient=%s, refreshable=%s, expiresAt=%d, "
+ "scopes=%s, properties=%s, clientIdAlias=%s, clientIdAliasUsed=%s, "
+ "confirmation=%s";
/**
* The next action the service implementation should take.
* @since Authlete 1.1
*/
private Action action;
/**
* The client ID.
* @since Authlete 1.1
*/
private long clientId;
/**
* Resource owner's user account.
* @since Authlete 1.1
*/
private String subject;
/**
* Scopes.
* @since Authlete 1.1
*/
private String[] scopes;
/**
* Scope details.
*
* @since 3.16
* @since Authlete 2.3.0
*/
private Scope[] scopeDetails;
/**
* Flag to indicate whether the access token exists.
* @since Authlete 1.1
*/
private boolean existent;
/**
* Flag to indicate whether the access token is usable
* (= exists and has not expired).
* @since Authlete 1.1
*/
private boolean usable;
/**
* Flag to indicate whether the access token covers the required scopes.
* @since Authlete 1.1
*/
private boolean sufficient;
/**
* Flag to indicate whether the access token is refreshable.
* @since Authlete 1.1
*/
private boolean refreshable;
/**
* Entity body of the response to the client.
* @since Authlete 1.1
*/
private String responseContent;
/**
* The time at which the access token expires.
* @since Authlete 1.1
*/
private long expiresAt;
/**
* Extra properties associated with the access token.
* @since Authlete 1.1
*/
private Property[] properties;
/**
* The client ID alias when the authorization request or the token request for
* the access token was made.
* @since Authlete 1.1
*/
private String clientIdAlias;
/**
* Flag which indicates whether the client ID alias was used when the authorization
* request or the token request for the access token was made.
* @since Authlete 1.1
*/
private boolean clientIdAliasUsed;
/**
* The entity ID of the client.
*
* @since 3.37
* @since Authlete 2.3
*/
private URI clientEntityId;
/**
* Flag which indicates whether the entity ID of the client was used when
* the request for the access token was made.
*
* @since 3.37
* @since Authlete 2.3
*/
private boolean clientEntityIdUsed;
/**
* Confirmation hash for MTLS-bound access tokens. Currently only the S256
* type is supported and is assumed.
* @since Authlete 1.1.17
*/
private String certificateThumbprint;
/**
* The target resources specified by the initial request.
* @since Authlete 2.2.1
*/
private URI[] resources;
/**
* The target resources of the access token.
* @since Authlete 2.2.0
*/
private URI[] accessTokenResources;
/**
* The content of the {@code "authorization_details"} request parameter which was
* included in the request that obtained the token.
* @since Authlete 2.2.0
*/
private AuthzDetails authorizationDetails;
/**
* Grant ID that this access token is tied to.
* @since Authlete 2.3.0
*/
private String grantId;
/**
* Grant that this access token has inherited.
* @since Authlete 2.3.0
*/
private Grant grant;
/**
* Claims that the user has consented for the client application to know.
* @since Authlete 2.3.0
*/
private String[] consentedClaims;
/**
* The attributes of the service that the client belongs to.
* @since Authlete 2.2.3
*/
private Pair[] serviceAttributes;
/**
* The attributes of the client that the access token has been issued to.
* @since Authlete 2.2.3
*/
private Pair[] clientAttributes;
/**
* Flag that indicates whether the token is for an external attachment.
*
* @since 3.16
* @since Authlete 2.3.0
*/
private boolean forExternalAttachment;
/**
* The Authentication Context Class Reference of the user authentication
* that the authorization server performed during the course of issuing
* the access token.
*
* @since 3.40
* @since Authlete 2.3
*/
private String acr;
/**
* The time when the user authentication was performed during the course
* of issuing the access token.
*
* @since 3.40
* @since Authlete 2.3
*/
private long authTime;
/**
* The grant type that was used for the issuance of the access token.
*
* @since 3.41
* @since Authlete 2.1.24
* @since Authlete 2.2.36
* @since Authlete 2.3
*/
private GrantType grantType;
/**
* The flag indicating whether the token is for credential issuance.
*
* @since 3.62
* @since Authlete 3.0
*/
private boolean forCredentialIssuance;
/**
* The {@code c_nonce}.
*
*
* The {@code cNonce} field added by the version 3.63 has been renamed
* to {@code cnonce} by the version 3.90.
*
*
* @since 3.90
* @since Authlete 3.0
*/
private String cnonce;
/**
* The time at which the {@code c_nonce} expires.
*
*
* The {@code cNonceExpiresAt} field added by the version 3.63 has been
* renamed to {@code cnonceExpiresAt} by the version 3.90.
*
*
* @since 3.90
* @since Authlete 3.0
*/
private long cnonceExpiresAt;
/**
* The credentials that can be obtained by presenting the access token.
*
* @since 3.78
* @since Authlete 3.0
*/
private String issuableCredentials;
/**
* The expected nonce value for DPoP proof JWT, which should be used
* as the value of the {@code DPoP-Nonce} HTTP header.
*
* @since 3.82
* @since Authlete 3.0
*/
private String dpopNonce;
/**
* The flag indicating whether the HTTP response from the protected
* resource endpoint must include an HTTP message signature in
* compliance with FAPI 2.0 Message Signing.
*
* @since 4.13
* @since Authlete 2.3.27
*/
private boolean responseSigningRequired;
/**
* Get the next action the service implementation should take.
*/
public Action getAction()
{
return action;
}
/**
* Set the next action the service implementation should take.
*/
public void setAction(Action action)
{
this.action = action;
}
/**
* Get the client ID.
*/
public long getClientId()
{
return clientId;
}
/**
* Set the client ID.
*/
public void setClientId(long clientId)
{
this.clientId = clientId;
}
/**
* Get the subject (= resource owner's ID).
*
*
* This method returns {@code null} if the access token was generated
* by Client Credentials Grant, which means that the access token
* is not associated with any specific end-user.
*
*/
public String getSubject()
{
return subject;
}
/**
* Set the subject (= resource owner's ID).
*/
public void setSubject(String subject)
{
this.subject = subject;
}
/**
* Get the scopes covered by the access token.
*/
public String[] getScopes()
{
return scopes;
}
/**
* Set the scopes covered by the access token.
*/
public void setScopes(String[] scopes)
{
this.scopes = scopes;
}
/**
* Get the details of the scopes.
*
*
* The {@code scopes} property of this class is a list of scope names.
* The property does not hold information about scope attributes.
* This {@code scopeDetails} property was newly created to convey
* information about scope attributes.
*
*
* @return
* The details of the scopes.
*
* @since 3.16
*/
public Scope[] getScopeDetails()
{
return scopeDetails;
}
/**
* Set the details of the scopes.
*
*
* The {@code scopes} property of this class is a list of scope names.
* The property does not hold information about scope attributes.
* This {@code scopeDetails} property was newly created to convey
* information about scope attributes.
*
*
* @param details
* The details of the scopes.
*
* @since 3.16
*/
public void setScopeDetails(Scope[] details)
{
this.scopeDetails = details;
}
/**
* Get the flag which indicates whether the access token exists.
*
* @return
* {@code true} if the access token exists.
* {@code false} if the access token does not exist.
*/
public boolean isExistent()
{
return existent;
}
/**
* Set the flag which indicates whether the access token exists.
*/
public void setExistent(boolean existent)
{
this.existent = existent;
}
/**
* Get the flag which indicates whether the access token is usable
* (= exists and has not expired).
*
* @return
* {@code true} if the access token is usable. {@code false}
* if the access token does not exist or has expired.
*/
public boolean isUsable()
{
return usable;
}
/**
* Set the flag which indicates whether the access token is usable
* (= exists and has not expired).
*/
public void setUsable(boolean usable)
{
this.usable = usable;
}
/**
* Get the flag which indicates whether the access token covers
* the required scopes.
*
* @return
* {@code true} if the access token covers all the required
* scopes. {@code false} if any one of the required scopes
* is not covered by the access token.
*/
public boolean isSufficient()
{
return sufficient;
}
/**
* Set the flag which indicates whether the access token covers
* the required scopes.
*/
public void setSufficient(boolean sufficient)
{
this.sufficient = sufficient;
}
/**
* Get the flag which indicates whether the access token can be
* refreshed using the associated refresh token.
*
* @return
* {@code true} if the access token can be refreshed using
* the associated refresh token. {@code false} if the refresh
* token for the access token has expired or the access token
* has no associated refresh token.
*/
public boolean isRefreshable()
{
return refreshable;
}
/**
* Set the flag which indicates whether the access token can be
* refreshed using the associated refresh token.
*/
public void setRefreshable(boolean refreshable)
{
this.refreshable = refreshable;
}
/**
* Get the response content which can be used as a part of the
* response to the client application.
*/
public String getResponseContent()
{
return responseContent;
}
/**
* Set the response content which can be used as a part of the
* response to the client application.
*/
public void setResponseContent(String responseContent)
{
this.responseContent = responseContent;
}
/**
* Get the time at which the access token expires in milliseconds
* since the Unix epoch (1970-01-01).
*
* @return
* The time at which the access token expires.
*/
public long getExpiresAt()
{
return expiresAt;
}
/**
* Set the time at which the access token expires in milliseconds
* since the Unix epoch (1970-01-01).
*
* @param expiresAt
* The time at which the access token expires.
*/
public void setExpiresAt(long expiresAt)
{
this.expiresAt = expiresAt;
}
/**
* Get the extra properties associated with the access token.
*
* @return
* Extra properties. This method returns {@code null} when
* no extra property is associated with the access token.
*
* @since 1.30
*/
public Property[] getProperties()
{
return properties;
}
/**
* Set the extra properties associated with the access token.
*
* @param properties
* Extra properties.
*
* @since 1.30
*/
public void setProperties(Property[] properties)
{
this.properties = properties;
}
/**
* Get the summary of this instance.
*/
public String summarize()
{
return String.format(SUMMARY_FORMAT,
action, clientId, subject, existent, usable,
sufficient, refreshable, expiresAt,
Utils.join(scopes, " "),
Utils.stringifyProperties(properties),
clientIdAlias, clientIdAliasUsed,
certificateThumbprint);
}
/**
* Get the client ID alias when the authorization request or the token
* request for the access token was made. Note that this value may be
* different from the current client ID alias.
*
* @return
* The client ID alias when the authorization request or the
* token request for the access token was made.
*
* @since 2.3
*/
public String getClientIdAlias()
{
return clientIdAlias;
}
/**
* Set the client ID alias when the authorization request or the token
* request for the access token was made.
*
* @param alias
* The client ID alias.
*
* @since 2.3
*/
public void setClientIdAlias(String alias)
{
this.clientIdAlias = alias;
}
/**
* Get the flag which indicates whether the client ID alias was used
* when the authorization request or the token request for the access
* token was made.
*
* @return
* {@code true} if the client ID alias was used when the
* authorization request or the token request for the access
* token was made.
*
* @since 2.3
*/
public boolean isClientIdAliasUsed()
{
return clientIdAliasUsed;
}
/**
* Set the flag which indicates whether the client ID alias was used
* when the authorization request or the token request for the access
* token was made.
*
* @param used
* {@code true} if the client ID alias was used when the
* authorization request or the token request for the access
* token was made.
*
* @since 2.3
*/
public void setClientIdAliasUsed(boolean used)
{
this.clientIdAliasUsed = used;
}
/**
* Get the entity ID of the client.
*
*
* "Entity ID" is a technical term defined in OpenID
* Federation 1.0.
*
*
* @return
* The entity ID of the client.
*
* @since 3.37
* @since Authlete 2.3
*
* @see OpenID Federation 1.0
*/
public URI getClientEntityId()
{
return clientEntityId;
}
/**
* Set the entity ID of the client.
*
*
* "Entity ID" is a technical term defined in OpenID
* Federation 1.0.
*
*
* @param entityId
* The entity ID of the client.
*
* @since 3.37
* @since Authlete 2.3
*
* @see OpenID Federation 1.0
*/
public void setClientEntityId(URI entityId)
{
this.clientEntityId = entityId;
}
/**
* Get the flag which indicates whether the entity ID of the client was
* used when the request for the access token was made.
*
*
* "Entity ID" is a technical term defined in OpenID
* Federation 1.0.
*
*
* @return
* {@code true} if the entity ID of the client was used when the
* request for the access token was made.
*
* @since 3.37
* @since Authlete 2.3
*
* @see OpenID Federation 1.0
*/
public boolean isClientEntityIdUsed()
{
return clientEntityIdUsed;
}
/**
* Set the flag which indicates whether the entity ID of the client was
* used when the request for the access token was made.
*
*
* "Entity ID" is a technical term defined in OpenID
* Federation 1.0.
*
*
* @param used
* {@code true} to indicate that the entity ID of the client was
* used when the request for the access token was made.
*
* @since 3.37
* @since Authlete 2.3
*
* @see OpenID Federation 1.0
*/
public void setClientEntityIdUsed(boolean used)
{
this.clientEntityIdUsed = used;
}
/**
* Get the client certificate thumbprint used to validate the access token.
*
* @return
* The certificate thumbprint, calculated as the SHA256 hash
* of the DER-encoded certificate value.
*
* @since 2.14
*/
public String getCertificateThumbprint()
{
return certificateThumbprint;
}
/**
* Set the client certificate thumbprint used to validate the access token.
*
* @param thumbprint
* The certificate thumbprint, calculated as the SHA256 hash
* of the DER-encoded certificate value.
*
* @since 2.14
*/
public void setCertificateThumbprint(String thumbprint)
{
this.certificateThumbprint = thumbprint;
}
/**
* Get the target resources. This represents the resources specified by
* the {@code resource} request parameters or by the {@code resource}
* property in the request object.
*
*
* See "Resource Indicators for OAuth 2.0" for details.
*
*
* @return
* Target resources.
*
* @see #getAccessTokenResources()
*
* @since 2.62
*/
public URI[] getResources()
{
return resources;
}
/**
* Set the target resources. This represents the resources specified by
* the {@code resource} request parameters or by the {@code resource}
* property in the request object.
*
* @param resources
* Target resources.
*
* @see #setAccessTokenResources(URI[])
*
* @since 2.62
*/
public void setResources(URI[] resources)
{
this.resources = resources;
}
/**
* Get the target resources of the access token.
*
*
* The target resources returned by this method may be the same as or
* different from the ones returned by {@link #getResources()}.
*
*
*
* In some flows, the initial request and the subsequent token request
* are sent to different endpoints. Example flows are the Authorization
* Code Flow, the Refresh Token Flow, the CIBA Ping Mode, the CIBA Poll
* Mode and the Device Flow. In these flows, not only the initial request
* but also the subsequent token request can include the {@code resource}
* request parameters. The purpose of the {@code resource} request
* parameters in the token request is to narrow the range of the target
* resources from the original set of target resources requested by the
* preceding initial request. If narrowing down is performed, the target
* resources returned by {@link #getResources()} and the ones returned by
* this method are different. This method returns the narrowed set of
* target resources.
*
*
*
* See "Resource Indicators for OAuth 2.0" for details.
*
*
* @return
* The target resources of the access token.
*
* @see #getResources()
*
* @since 2.62
*/
public URI[] getAccessTokenResources()
{
return accessTokenResources;
}
/**
* Set the target resources of the access token.
*
*
* See the description of {@link #getAccessTokenResources()} for details
* about the target resources of the access token.
*
*
* @param resources
* The target resources of the access token.
*
* @see #setResources(URI[])
*
* @since 2.62
*/
public void setAccessTokenResources(URI[] resources)
{
this.accessTokenResources = resources;
}
/**
* Get the authorization details. This represents the value of the
* {@code "authorization_details"} request parameter which is defined in
* "OAuth 2.0 Rich Authorization Requests".
*
* @return
* Authorization details.
*
* @since 2.56
*/
public AuthzDetails getAuthorizationDetails()
{
return authorizationDetails;
}
/**
* Set the authorization details. This represents the value of the
* {@code "authorization_details"} request parameter which is defined in
* "OAuth 2.0 Rich Authorization Requests".
*
* @param details
* Authorization details.
*
* @since 2.56
*/
public void setAuthorizationDetails(AuthzDetails details)
{
this.authorizationDetails = details;
}
/**
* Get the grant ID which this access token is tied to.
*
*
* In Authlete, when an authorization request includes the
* {@code grant_management_action} request parameter, a grant ID (which
* may be a newly-generated one or an existing one specified by the
* {@code grant_id} request parameter) is tied to the access token which
* is created as a result of the authorization request.
*
*
* @return
* The grant ID tied to this access token.
*
* @see Grant Management for OAuth 2.0
*
* @since 3.1
*/
public String getGrantId()
{
return grantId;
}
/**
* Set the grant ID which this access token is tied to.
*
*
* In Authlete, when an authorization request includes the
* {@code grant_management_action} request parameter, a grant ID (which
* may be a newly-generated one or an existing one specified by the
* {@code grant_id} request parameter) is tied to the access token which
* is created as a result of the authorization request.
*
*
* @param grantId
* The grant ID tied to this access token.
*
* @see Grant Management for OAuth 2.0
*
* @since 3.1
*/
public void setGrantId(String grantId)
{
this.grantId = grantId;
}
/**
* Get the grant that this access token has inherited.
*
*
* When an authorization request includes {@code grant_id} and
* {@code grant_management_action=update}, privileges identified by the
* grant ID are additionally given to the access token which is created
* as a result of the authorization request. This property represents
* the grant.
*
*
* @return
* The grant that this access token has inherited.
*
* @see Grant Management for OAuth 2.0
*
* @since 3.1
*/
public Grant getGrant()
{
return grant;
}
/**
* Set the grant that this access token has inherited.
*
*
* When an authorization request includes {@code grant_id} and
* {@code grant_management_action=update}, privileges identified by the
* grant ID are additionally given to the access token which is created
* as a result of the authorization request. This property represents
* the grant.
*
*
* @param grant
* The grant that this access token has inherited.
*
* @see Grant Management for OAuth 2.0
*
* @since 3.1
*/
public void setGrant(Grant grant)
{
this.grant = grant;
}
/**
* Get the claims that the user has consented for the client application
* to know.
*
*
* The following Authlete APIs accept a {@code consentedClaims} request
* parameter (which is supported from Authlete 2.3).
*
*
*
* - {@code /auth/authorization/issue}
*
- {@code /backchannel/authentication/complete}
*
- {@code /device/complete}
*
*
*
* The request parameter is used to convey consented claims to Authlete.
* This property holds the consented claims. See the description of
* {@link AuthorizationIssueRequest#setConsentedClaims(String[])} for
* details.
*
*
* @return
* Consented claims.
*
* @see AuthorizationIssueRequest#setConsentedClaims(String[])
* @see BackchannelAuthenticationCompleteRequest#setConsentedClaims(String[])
* @see DeviceCompleteRequest#setConsentedClaims(String[])
*
* @since 3.7
*/
public String[] getConsentedClaims()
{
return consentedClaims;
}
/**
* Set the claims that the user has consented for the client application
* to know.
*
*
* The following Authlete APIs accept a {@code consentedClaims} request
* parameter (which is supported from Authlete 2.3).
*
*
*
* - {@code /auth/authorization/issue}
*
- {@code /backchannel/authentication/complete}
*
- {@code /device/complete}
*
*
*
* The request parameter is used to convey consented claims to Authlete.
* This property holds the consented claims. See the description of
* {@link AuthorizationIssueRequest#setConsentedClaims(String[])} for
* details.
*
*
* @param claims
* Consented claims.
*
* @see AuthorizationIssueRequest#setConsentedClaims(String[])
* @see BackchannelAuthenticationCompleteRequest#setConsentedClaims(String[])
* @see DeviceCompleteRequest#setConsentedClaims(String[])
*
* @since 3.7
*/
public void setConsentedClaims(String[] claims)
{
this.consentedClaims = claims;
}
/**
* Get the attributes of the service that the client application belongs to.
*
*
* This property is available since Authlete 2.2.
*
*
* @return
* The attributes of the service.
*
* @since 2.88
*/
public Pair[] getServiceAttributes()
{
return serviceAttributes;
}
/**
* Set the attributes of the service that the client application belongs to.
*
*
* This property is available since Authlete 2.2.
*
*
* @param attributes
* The attributes of the service.
*
* @since 2.88
*/
public void setServiceAttributes(Pair[] attributes)
{
this.serviceAttributes = attributes;
}
/**
* Get the attributes of the client that the access token has been issued to.
*
*
* This property is available since Authlete 2.2.
*
*
* @return
* The attributes of the client.
*
* @since 2.88
*/
public Pair[] getClientAttributes()
{
return clientAttributes;
}
/**
* Set the attributes of the client that the access token has been issued to.
*
*
* This property is available since Authlete 2.2.
*
*
* @param attributes
* The attributes of the client.
*
* @since 2.88
*/
public void setClientAttributes(Pair[] attributes)
{
this.clientAttributes = attributes;
}
/**
* Get the flag which indicates whether the token is for an external attachment.
*
*
* OpenID Provider implementations can make Authlete generate access tokens
* for external attachments and embed them in ID tokens and userinfo responses
* by setting true to the {@code accessTokenForExternalAttachmentEmbedded}
* property of the service. If the token presented at Authlete's
* {@code /auth/introspection} API has been generated by the feature,
* this {@code forExternalAttachment} property in the response from the
* Authlete API becomes true. See the description of
* {@link Service#isAccessTokenForExternalAttachmentEmbedded()} for details
* about the feature.
*
*
* @return
* {@code true} if the token is for an external attachment.
*
* @since 3.16
*
* @see Service#isAccessTokenForExternalAttachmentEmbedded()
*/
public boolean isForExternalAttachment()
{
return forExternalAttachment;
}
/**
* Set the flag which indicates whether the token is for an external attachment.
*
*
* OpenID Provider implementations can make Authlete generate access tokens
* for external attachments and embed them in ID tokens and userinfo responses
* by setting true to the {@code accessTokenForExternalAttachmentEmbedded}
* property of the service. If the token presented at Authlete's
* {@code /api/auth/introspection} API has been generated by the feature,
* this {@code forExternalAttachment} property in the response from the
* Authlete API becomes true. See the description of
* {@link Service#isAccessTokenForExternalAttachmentEmbedded()} for details
* about the feature.
*
*
* @param forExternalAttachment
* {@code true} to indicate that the token is for an external attachment.
*
* @since 3.16
*
* @see Service#isAccessTokenForExternalAttachmentEmbedded()
*/
public void setForExternalAttachment(boolean forExternalAttachment)
{
this.forExternalAttachment = forExternalAttachment;
}
/**
* Get the Authentication Context Class Reference of the user authentication
* that the authorization server performed during the course of issuing the
* access token.
*
* @return
* The Authentication Context Class Reference.
*
* @since 3.40
* @since Authlete 2.3
*
* @see RFC 9470 OAuth 2.0 Step Up Authentication Challenge Protocol
*/
public String getAcr()
{
return acr;
}
/**
* Set the Authentication Context Class Reference of the user authentication
* that the authorization server performed during the course of issuing the
* access token.
*
* @param acr
* The Authentication Context Class Reference.
*
* @since 3.40
* @since Authlete 2.3
*
* @see RFC 9470 OAuth 2.0 Step Up Authentication Challenge Protocol
*/
public void setAcr(String acr)
{
this.acr = acr;
}
/**
* Get the time when the user authentication was performed during the course
* of issuing the access token.
*
* @return
* The time of the user authentication in seconds since the Unix epoch.
*
* @since 3.40
* @since Authlete 2.3
*
* @see RFC 9470 OAuth 2.0 Step Up Authentication Challenge Protocol
*/
public long getAuthTime()
{
return authTime;
}
/**
* Set the time when the user authentication was performed during the course
* of issuing the access token.
*
* @param authTime
* The time of the user authentication in seconds since the Unix epoch.
*
* @since 3.40
* @since Authlete 2.3
*
* @see RFC 9470 OAuth 2.0 Step Up Authentication Challenge Protocol
*/
public void setAuthTime(long authTime)
{
this.authTime = authTime;
}
/**
* Get the grant type that was used for issuance of the access token.
*
* @return
* The grant type.
*
* @since 3.41
* @since Authlete 2.1.24
* @since Authlete 2.2.36
* @since Authlete 2.3
*/
public GrantType getGrantType()
{
return grantType;
}
/**
* Set the grant type that was used for issuance of the access token.
*
* @param grantType
* The grant type.
*
* @since 3.41
* @since Authlete 2.1.24
* @since Authlete 2.2.36
* @since Authlete 2.3
*/
public void setGrantType(GrantType grantType)
{
this.grantType = grantType;
}
/**
* Get the flag indicating whether the token is for credential issuance.
*
*
* OpenID for Verifiable Credential Issuance defines flows to issue
* Verifiable Credentials. In the flows, a wallet presents an access token
* at the credential endpoint of a credential issuer and gets a Verifiable
* Credential in return.
*
*
*
* To get an access token for the purpose, a client application uses either
* the pre-authorized code flow or the authorization code flow with the
* {@code authorization_details} request parameter whose {@code type} is
* {@code openid_credential}.
*
*
*
* For access tokens obtained by the flows described above, this
* {@code forCredentialIssuance} flag is set.
*
*
* @return
* {@code true} if the token is for credential issuance.
*
* @see OpenID for Verifiable Credential Issuance
*
* @since 3.62
* @since Authlete 3.0
*/
public boolean isForCredentialIssuance()
{
return forCredentialIssuance;
}
/**
* Set the flag indicating whether the token is for credential issuance.
*
*
* OpenID for Verifiable Credential Issuance defines flows to issue
* Verifiable Credentials. In the flows, a wallet presents an access token
* at the credential endpoint of a credential issuer and gets a Verifiable
* Credential in return.
*
*
*
* To get an access token for the purpose, a client application uses either
* the pre-authorized code flow or the authorization code flow with the
* {@code authorization_details} request parameter whose {@code type} is
* {@code openid_credential}.
*
*
*
* For access tokens obtained by the flows described above, this
* {@code forCredentialIssuance} flag should be set.
*
*
* @param forCredentialIssuance
* {@code true} to indicate that the token is for credential issuance.
*
* @see OpenID for Verifiable Credential Issuance
*
* @since 3.62
* @since Authlete 3.0
*/
public void setForCredentialIssuance(boolean forCredentialIssuance)
{
this.forCredentialIssuance = forCredentialIssuance;
}
/**
* Get the {@code c_nonce} associated with the access token.
*
*
* {@code c_nonce} is issued from the token endpoint of an authorization
* server in the pre-authorized code flow, and from the credential endpoint
* and the batch credential endpoint of a credential issuer.
*
*
*
* In Authlete's implementation, {@code c_nonce} is managed in an access
* token record. Therefore, when the database record is deleted, the
* {@code c_nonce} is deleted together.
*
*
*
* See OpenID for Verifiable Credentials Issuance for details about {@code
* c_nonce}.
*
*
*
* The {@code getCNonce()} method added by the version 3.63 has been renamed
* to {@code getCnonce()} by the version 3.90.
*
*
* @return
* The {@code c_nonce}.
*
* @since 3.90
* @since Authlete 3.0
*
* @see OpenID for Verifiable Credentials Issuance
*/
public String getCnonce()
{
return cnonce;
}
/**
* Set the {@code c_nonce} associated with the access token.
*
*
* {@code c_nonce} is issued from the token endpoint of an authorization
* server in the pre-authorized code flow, and from the credential endpoint
* and the batch credential endpoint of a credential issuer.
*
*
*
* In Authlete's implementation, {@code c_nonce} is managed in an access
* token record. Therefore, when the database record is deleted, the
* {@code c_nonce} is deleted together.
*
*
*
* See OpenID for Verifiable Credentials Issuance for details about {@code
* c_nonce}.
*
*
*
* The {@code setCNonce(String)} method added by the version 3.63 has been
* renamed to {@code setCnonce(String)} by the version 3.90.
*
*
* @param nonce
* The {@code c_nonce}.
*
* @since 3.90
* @since Authlete 3.0
*
* @see OpenID for Verifiable Credentials Issuance
*/
public void setCnonce(String nonce)
{
this.cnonce = nonce;
}
/**
* Get the time at which the {@code c_nonce} expires in milliseconds since
* the Unix epoch (1970-01-01).
*
*
* The {@code getCNonceExpiresAt()} method added by the version 3.63 has
* been renamed to {@code getCnonceExpiresAt()} by the version 3.90.
*
*
* @return
* The time at which the {@code c_nonce} expires in milliseconds
* since the Unix epoch (1970-01-01).
*
* @since 3.90
* @since Authlete 3.0
*
* @see OpenID for Verifiable Credentials Issuance
*/
public long getCnonceExpiresAt()
{
return cnonceExpiresAt;
}
/**
* Set the time at which the {@code c_nonce} expires in milliseconds since
* the Unix epoch (1970-01-01).
*
*
* The {@code setCNonceExpiresAt(long)} method added by the version 3.63 has
* been renamed to {@code setCnonceExpiresAt(long)} by the version 3.90.
*
*
* @param expiresAt
* The time at which the {@code c_nonce} expires in milliseconds
* since the Unix epoch (1970-01-01).
*
* @since 3.90
* @since Authlete 3.0
*
* @see OpenID for Verifiable Credentials Issuance
*/
public void setCnonceExpiresAt(long expiresAt)
{
this.cnonceExpiresAt = expiresAt;
}
/**
* Get the credentials that can be obtained by presenting the access token.
*
*
* The value of this property comes from one or more of the following sources.
*
*
*
* -
* The "{@code credential_configurations}" property in the credential offer
* that was used when the access token was issued. A credential offer may
* contain either or both an issuer state and a pre-authorized code. The
* issuer state can be used as the value of the "{@code issuer_state}"
* request parameter of an authorization request. The pre-authorized code
* can be used as the value of the "{@code pre-authorized_code}" request
* parameter of a token request whose "{@code grant_type}" is
* "{@code urn:ietf:params:oauth:grant-type:pre-authorized_code}".
*
*
-
* The content of a RAR object whose "{@code type}" is
* "{@code openid_credential}". RAR objects can be listed in the
* "{@code authorization_details}" request parameter, which is defined in
* RFC 9396 OAuth 2.0
* Rich Authorization Requests.
*
*
-
* The content of an entry in the "{@code credential_configurations_supported}"
* metadata of the credential issuer. The "{@code scope}" property of an entry
* in the "{@code credential_configurations_supported}" metadata can be used
* as a value in the "{@code scope}" request parameter.
*
*
*
* The format of this property is a JSON array whose elements are JSON
* objects. The following is a simple example.
*
*
*
* [
* {
* "format": "vc+sd-jwt",
* "credential_definition": {
* "vct": "https://credentials.example.com/identity_credential"
* }
* }
* ]
*
*
* @return
* The credentials that can be obtained by presenting the access
* token.
*
* @see OpenID for Verifiable Credential Issuance
*
* @since 3.78
* @since Authlete 3.0
*/
public String getIssuableCredentials()
{
return issuableCredentials;
}
/**
* Set the credentials that can be obtained by presenting the access token.
*
*
* See the description of the {@link #getIssuableCredentials()} method for
* details about the content of this property.
*
*
* @param credentials
* The credentials that can be obtained by presenting the access
* token.
*
* @see OpenID for Verifiable Credential Issuance
*
* @since 3.78
* @since Authlete 3.0
*/
public void setIssuableCredentials(String credentials)
{
this.issuableCredentials = credentials;
}
/**
* Get the expected nonce value for DPoP proof JWT, which should be used
* as the value of the {@code DPoP-Nonce} HTTP header.
*
*
* When this response parameter is not null, the implementation of the
* protected resource endpoint should add the {@code DPoP-Nonce} HTTP
* header in the response from the endpoint to the client application,
* using the value of this response parameter as the value of the HTTP
* header.
*
*
*
* DPoP-Nonce: (The value of this {@code dpopNonce} response parameter)
*
*
* @return
* The expected nonce value for DPoP proof JWT.
*
* @since 3.82
* @since Authlete 3.0
*
* @see RFC 9449 OAuth 2.0 Demonstrating Proof of Possession (DPoP)
*/
public String getDpopNonce()
{
return dpopNonce;
}
/**
* Set the expected nonce value for DPoP proof JWT, which should be used
* as the value of the {@code DPoP-Nonce} HTTP header.
*
*
* When this response parameter is not null, the implementation of the
* protected resource endpoint should add the {@code DPoP-Nonce} HTTP
* header in the response from the endpoint to the client application,
* using the value of this response parameter as the value of the HTTP
* header.
*
*
*
* DPoP-Nonce: (The value of this {@code dpopNonce} response parameter)
*
*
* @param dpopNonce
* The expected nonce value for DPoP proof JWT.
*
* @since 3.82
* @since Authlete 3.0
*
* @see RFC 9449 OAuth 2.0 Demonstrating Proof of Possession (DPoP)
*/
public void setDpopNonce(String dpopNonce)
{
this.dpopNonce = dpopNonce;
}
/**
* Get the flag indicating whether the HTTP response from the protected
* resource endpoint must include an HTTP message signature (RFC 9421 HTTP Message
* Signatures) in compliance with FAPI 2.0 Message Signing.
*
*
* When this response parameter is {@code true}, the HTTP response from
* the protected resource endpoint must include the {@code Signature}
* and {@code Signature-Input} HTTP fields.
*
*
* @return
* True if the HTTP response from the protected resource endpoint
* must include an HTTP message signature in compliance with
* FAPI 2.0 Message Signing.
*
* @since 4.13
* @since Authlete 2.3.27
*/
public boolean isResponseSigningRequired()
{
return responseSigningRequired;
}
/**
* Set the flag indicating whether the HTTP response from the protected
* resource endpoint must include an HTTP message signature (RFC 9421 HTTP Message
* Signatures) in compliance with FAPI 2.0 Message Signing.
*
*
* When this response parameter is {@code true}, the HTTP response from
* the protected resource endpoint must include the {@code Signature}
* and {@code Signature-Input} HTTP fields.
*
*
* @param responseSigningRequired
* True if the HTTP response from the protected resource endpoint
* must include an HTTP message signature in compliance with
* FAPI 2.0 Message Signing.
*
* @since 4.13
* @since Authlete 2.3.27
*/
public void setResponseSigningRequired(boolean responseSigningRequired)
{
this.responseSigningRequired = responseSigningRequired;
}
}