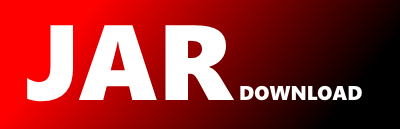
com.authlete.common.dto.ServiceConfigurationRequest Maven / Gradle / Ivy
Show all versions of authlete-java-common Show documentation
/*
* Copyright (C) 2022 Authlete, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.authlete.common.dto;
import java.io.Serializable;
/**
* The request parameters of Authlete's {@code /service/configuration} API.
*
* pretty
*
*
* If {@code pretty=true} is given to the Authlete API, the returned JSON is
* formatted in a human-friendly way.
*
*
* patch
*
*
* The {@code /service/configuration} API returns JSON that conforms to
* OpenID Connect Discovery 1.0. Implementations of the discovery
* endpoint may modify the JSON before returning it as a discovery response.
*
*
*
* Although implementations of the discovery endpoint have perfect control
* over the JSON, in some cases implementations may want to make the Authlete
* API execute adjustments on Authlete side so that the implementations can
* avoid modifying the received JSON after the API call.
*
*
*
* The {@code patch} request parameter has been added for the purpose.
*
*
*
* Implementations of the discovery endpoint may specify a JSON Patch
* by the {@code patch} request parameter. If the request parameter is given,
* the Authlete API applies the patch to the JSON before returning it to the
* API caller.
*
*
*
* The value of the {@code patch} request parameter must conform to the format
* that is defined in RFC 6902
* JavaScript Object Notation (JSON) Patch.
*
*
* Example 1: Replace
*
*
* $ curl -s -u $SERVICE_API_KEY:$SERVICE_API_SECRET $BASE_URL/api/service/configuration
* | jq .subject_types_supported
* [
* "public",
* "pairwise"
* ]
*
*
*
* $ curl -s -u $SERVICE_API_KEY:$SERVICE_API_SECRET $BASE_URL/api/service/configuration
* -d patch='[{"op":"replace","path":"/subject_types_supported","value":["public"]}]'
* | jq .subject_types_supported
* [
* "public"
* ]
*
*
* Example 2: Add
*
*
* $ curl -s -u $SERVICE_API_KEY:$SERVICE_API_SECRET $BASE_URL/api/service/configuration
* | jq .custom_metadata
* null
*
*
*
* $ curl -s -u $SERVICE_API_KEY:$SERVICE_API_SECRET $BASE_URL/api/service/configuration
* -d patch='[{"op":"add","path":"/custom_metadata","value":"custom_value"}]'
* | jq .custom_metadata
* "custom_value"
*
*
* Example 3: Add Array Elements
*
*
* $ curl -s -u $SERVICE_API_KEY:$SERVICE_API_SECRET $BASE_URL/api/service/configuration
* | jq .acr_values_supported
* [
* "acr1"
* ]
*
*
*
* $ curl -s -u $SERVICE_API_KEY:$SERVICE_API_SECRET $BASE_URL/api/service/configuration
* -d patch='[{"op":"add","path":"/acr_values_supported/0","value":"acr0"}]'
* | jq .acr_values_supported
* [
* "acr0",
* "acr1"
* ]
*
*
*
* $ curl -s -u $SERVICE_API_KEY:$SERVICE_API_SECRET $BASE_URL/api/service/configuration
* -d patch='[{"op":"add","path":"/acr_values_supported/0","value":"acr0"},
* {"op":"add","path":"/acr_values_supported/-","value":"acr2"}]'
* | jq .acr_values_supported
* [
* "acr0",
* "acr1",
* "acr2"
* ]
*
*
* Example 4: Add Object Elements
*
*
* $ curl -s -u $SERVICE_API_KEY:$SERVICE_API_SECRET $BASE_URL/api/service/configuration
* | jq .mtls_endpoint_aliases
* {
* "authorization_endpoint": "https://as.example.com/mtls/authorize"
* }
*
*
*
* $ curl -s -u $SERVICE_API_KEY:$SERVICE_API_SECRET $BASE_URL/api/service/configuration
* -d patch='[{"op":"add","path":"/mtls_endpoint_aliases/token_endpoint","value":"https://as.example.com/mtls/token"}]'
* | jq .mtls_endpoint_aliases
* {
* "authorization_endpoint": "https://as.example.com/mtls/authorize",
* "token_endpoint": "https://as.example.com/mtls/token"
* }
*
*
* Example 5: Remove
*
*
* $ curl -s -u $SERVICE_API_KEY:$SERVICE_API_SECRET $BASE_URL/api/service/configuration
* | jq .acr_values_supported
* [
* "acr1"
* ]
*
*
*
* $ curl -s -u $SERVICE_API_KEY:$SERVICE_API_SECRET $BASE_URL/api/service/configuration
* -d patch='[{"op":"remove","path":"/acr_values_supported"}]'
* | jq .acr_values_supported
* null
*
*
* Note
*
*
* The {@code patch} request parameter is supported since Authlete 2.2.36.
*
*
* @since 3.43
*
* @see OpenID Connect Discovery 1.0
*
* @see RFC 6902 JavaScript Object Notation (JSON) Patch
*/
public class ServiceConfigurationRequest implements Serializable
{
private static final long serialVersionUID = 1L;
private boolean pretty;
private String patch;
/**
* Get the flag indicating whether the JSON returned from the API is
* formatted in a human-friendly way.
*
* @return
* {@code true} if the JSON is formatted in a human-friendly way.
*/
public boolean isPretty()
{
return pretty;
}
/**
* Set the flag indicating whether the JSON returned from the API is
* formatted in a human-friendly way.
*
* @param pretty
* {@code true} to format the JSON in a human-friendly way.
*
* @return
* {@code this} object.
*/
public ServiceConfigurationRequest setPretty(boolean pretty)
{
this.pretty = pretty;
return this;
}
/**
* Get the JSON Patch (RFC 6902 JavaScript Object Notation (JSON) Patch) to be applied.
*
* @return
* The JSON Patch.
*
* @see RFC 6902 JavaScript Object Notation (JSON) Patch
*/
public String getPatch()
{
return patch;
}
/**
* Set a JSON Patch (RFC 6902 JavaScript Object Notation (JSON) Patch) to be applied.
*
* @param patch
* A JSON Patch.
*
* @return
* {@code this} object.
*
* @see RFC 6902 JavaScript Object Notation (JSON) Patch
*/
public ServiceConfigurationRequest setPatch(String patch)
{
this.patch = patch;
return this;
}
}