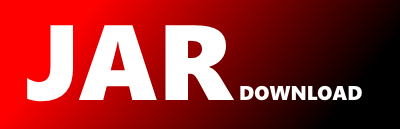
com.authlete.common.dto.TokenResponse Maven / Gradle / Ivy
Show all versions of authlete-java-common Show documentation
/*
* Copyright (C) 2014-2023 Authlete, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.authlete.common.dto;
import java.net.URI;
import com.authlete.common.types.ClientAuthMethod;
import com.authlete.common.types.GrantType;
import com.authlete.common.types.TokenType;
import com.authlete.common.util.Utils;
/**
* Response from Authlete's {@code /auth/token} API.
*
*
* Authlete's {@code /auth/token} API returns JSON which can
* be mapped to this class. The service implementation should retrieve the
* value of {@code "action"} from the response and take the following steps
* according to the value.
*
*
*
* {@link Action#INVALID_CLIENT INVALID_CLIENT}
*
*
* When the value of {@code "action"} is {@code "INVALID_CLIENT"}, it means
* that authentication of the client failed. In this case, the HTTP status
* of the response to the client application is either {@code "400 Bad
* Request"} or {@code "401 Unauthorized"}. This requirement comes from
* RFC 6749, 5.2.
* Error Response. The description about {@code "invalid_client"} shown
* below is an excerpt from RFC 6749.
*
*
*
*
* invalid_client
* -
*
* Client authentication failed (e.g., unknown client, no client
* authentication included, or unsupported authentication method).
* The authorization server MAY return an HTTP 401 (Unauthorized)
* status code to indicate which HTTP authentication schemes are
* supported. If the client attempted to authenticate via the
* "Authorization" request header field, the authorization server
* MUST respond with an HTTP 401 (Unauthorized) status code and
* include the "WWW-Authenticate" response header field matching the
* authentication scheme used by the client.
*
*
*
*
*
*
* In either case, the JSON string returned by {@link #getResponseContent()}
* can be used as the entity body of the response to the client application.
*
*
*
* The following illustrate the response which the service implementation
* should generate and return to the client application.
*
*
*
* HTTP/1.1 400 Bad Request
* Content-Type: application/json
* Cache-Control: no-store
* Pragma: no-cache
*
* (The value returned from {@link #getResponseContent()})
*
*
* HTTP/1.1 401 Unauthorized
* WWW-Authenticate: (challenge)
* Content-Type: application/json
* Cache-Control: no-store
* Pragma: no-cache
*
* (The value returned from {@link #getResponseContent()})
*
*
* {@link Action#INTERNAL_SERVER_ERROR INTERNAL_SERVER_ERROR}
*
*
* When the value of {@code "action"} is {@code "INTERNAL_SERVER_ERROR"},
* it means that the request from the service implementation
* ({@link AuthorizationIssueRequest}) was wrong or that an error occurred
* in Authlete.
*
*
*
* In either case, from the viewpoint of the client application, it is an
* error on the server side. Therefore, the service implementation should
* generate a response to the client application with the HTTP status of
* {@code "500 Internal Server Error"}.
*
*
*
* {@link #getResponseContent()} returns a JSON string which describes
* the error, so it can be used as the entity body of the response.
*
*
*
* The following illustrates the response which the service implementation
* should generate and return to the client application.
*
*
*
* HTTP/1.1 500 Internal Server Error
* Content-Type: application/json
* Cache-Control: no-store
* Pragma: no-cache
*
* (The value returned from {@link #getResponseContent()})
*
*
* {@link Action#BAD_REQUEST BAD_REQUEST}
*
*
* When the value of {@code "action"} is {@code "BAD_REQUEST"}, it means
* that the request from the client application is invalid.
*
*
*
* The HTTP status of the response returned to the client application
* must be {@code "400 Bad Request"} and the content type must be
* {@code "application/json"}.
*
*
*
* {@link #getResponseContent()} returns a JSON string which describes
* the error, so it can be used as the entity body of the response.
*
*
*
* The following illustrates the response which the service implementation
* should generate and return to the client application.
*
*
*
* HTTP/1.1 400 Bad Request
* Content-Type: application/json
* Cache-Control: no-store
* Pragma: no-cache
*
* (The value returned from {@link #getResponseContent()})
*
*
* {@link Action#PASSWORD PASSWORD}
*
*
* When the value of {@code "action"} is {@code "PASSWORD"}, it means that
* the request from the client application is valid and {@code grant_type}
* is {@code "password"}. That is, the flow is
* "Resource Owner
* Password Credentials".
*
*
*
* In this case, {@link #getUsername()} returns the value of {@code "username"}
* request parameter and {@link #getPassword()} returns the value of {@code
* "password"} request parameter which were contained in the token request
* from the client application. The service implementation must validate the
* credentials of the resource owner (= end-user) and take either of the
* actions below according to the validation result.
*
*
*
* -
*
* When the credentials are valid, call Authlete's {@code
* /auth/token/issue} API to generate an access token for the client
* application. The API requires {@code "ticket"} request parameter and
* {@code "subject"} request parameter.
* Use the value returned from {@link #getTicket()} method as the value
* for {@code "ticket"} parameter.
*
*
* The response from {@code /auth/token/issue} API ({@link
* TokenIssueResponse}) contains data (an access token and others)
* which should be returned to the client application. Use the data
* to generate a response to the client application.
*
* -
*
* When the credentials are invalid, call Authlete's {@code
* /auth/token/fail} API with {@code reason=}{@link
* TokenFailRequest.Reason#INVALID_RESOURCE_OWNER_CREDENTIALS
* INVALID_RESOURCE_OWNER_CREDENTIALS} to generate an error response
* for the client application. The API requires {@code "ticket"}
* request parameter. Use the value returned from {@link #getTicket()}
* method as the value for {@code "ticket"} parameter.
*
*
* The response from {@code /auth/token/fail} API ({@link
* TokenFailResponse}) contains error information which should be
* returned to the client application. Use it to generate a response
* to the client application.
*
*
*
*
* {@link Action#OK OK}
*
*
* When the value of {@code "action"} is {@code "OK"}, it means that
* the request from the client application is valid and an access token,
* and optionally an ID token, is ready to be issued.
*
*
*
* The HTTP status of the response returned to the client application
* must be {@code "200 OK"} and the content type must be
* {@code "application/json"}.
*
*
*
* {@link #getResponseContent()} returns a JSON string which contains
* an access token (and optionally an ID token), so it can be used as
* the entity body of the response.
*
*
*
* The following illustrates the response which the service implementation
* should generate and return to the client application.
*
*
*
* HTTP/1.1 200 OK
* Content-Type: application/json
* Cache-Control: no-store
* Pragma: no-cache
*
* (The value returned from {@link #getResponseContent()})
*
*
* {@link Action#TOKEN_EXCHANGE TOKEN_EXCHANGE} (Authlete 2.3 onwards)
*
*
* When the value of {@code "action"} is {@code "TOKEN_EXCHANGE"}, it means
* that the request from the client application is a valid token exchange
* request (cf. RFC
* 8693 OAuth 2.0 Token Exchange) and that the request has already passed
* the following validation steps.
*
*
*
* -
*
* Confirm that the value of the {@code requested_token_type} request parameter
* is one of the registered token type identifiers if the request parameter is
* given and its value is not empty.
*
*
* -
*
* Confirm that the {@code subject_token} request parameter is given and its
* value is not empty.
*
*
* -
*
* Confirm that the {@code subject_token_type} request parameter is given and
* its value is one of the registered token type identifiers.
*
*
* -
*
* Confirm that the {@code actor_token_type} request parameter is given and
* its value is one of the registered token type identifiers if the
* {@code actor_token} request parameter is given and its value is not empty.
*
*
* -
*
* Confirm that the {@code actor_token_type} request parameter is not given
* or its value is empty when the {@code actor_token} request parameter is
* not given or its value is empty.
*
*
*
*
* Furthermore, Authlete performs additional validation on the tokens specified
* by the {@code subject_token} request parameter and the {@code actor_token}
* request parameter according to their respective token types as shown below.
*
*
*
* Token Validation Steps
*
* Token Type
* urn:ietf:params:oauth:token-type:jwt
*
*
*
*
* -
*
* Confirm that the format conforms to the JWT specification (RFC 7519).
*
* -
*
* Check if the JWT is encrypted and if it is encrypted, then (a) reject
* the token exchange request when the {@link
* Service#isTokenExchangeEncryptedJwtRejected()
* tokenExchangeEncryptedJwtRejected} flag of the service is {@code true}
* or (b) skip remaining validation steps when the flag is {@code false}.
* Note that Authlete does not verify an encrypted JWT because there is
* no standard way to obtain the key to decrypt the JWT with. This means
* that you must verify an encrypted JWT by yourself when one is used as
* an input token with the token type
* {@code "urn:ietf:params:oauth:token-type:jwt"}.
*
* -
*
* Confirm that the current time has not reached the time indicated by
* the {@code exp} claim if the JWT contains the claim.
*
* -
*
* Confirm that the current time is equal to or after the time indicated
* by the {@code iat} claim if the JWT contains the claim.
*
* -
*
* Confirm that the current time is equal to or after the time indicated
* by the {@code nbf} claim if the JWT contains the claim.
*
* -
*
* Check if the JWT is signed and if it is not signed, then (a) reject
* the token exchange request when the {@link
* Service#isTokenExchangeUnsignedJwtRejected()
* tokenExchangeUnsignedJwtRejected} flag of the service is {@code true}
* or (b) finish validation on the input token. Note that Authlete does
* not verify the signature of the JWT because there is no standard way
* to obtain the key to verify the signature of a JWT with. This means
* that you must verify the signature by yourself when a signed JWT is
* used as an input token with the token type
* {@code "urn:ietf:params:oauth:token-type:jwt"}.
*
*
*
*
*
* Token Type
* urn:ietf:params:oauth:token-type:access_token
*
*
*
*
* -
*
* Confirm that the token is an access token that has been issued by
* the Authlete server of your service. This implies that access
* tokens issued by other systems cannot be used as a subject token
* or an actor token with the token type
* urn:ietf:params:oauth:token-type:access_token
.
*
* -
*
* Confirm that the access token has not expired.
*
* -
*
* Confirm that the access token belongs to the service.
*
*
*
*
*
* Token Type
* urn:ietf:params:oauth:token-type:refresh_token
*
*
*
*
* -
*
* Confirm that the token is a refresh token that has been issued by
* the Authlete server of your service. This implies that refresh
* tokens issued by other systems cannot be used as a subject token
* or an actor token with the token type
* urn:ietf:params:oauth:token-type:refresh_token
.
*
* -
*
* Confirm that the refresh token has not expired.
*
* -
*
* Confirm that the refresh token belongs to the service.
*
*
*
*
*
* Token Type
* urn:ietf:params:oauth:token-type:id_token
*
*
*
*
* -
*
* Confirm that the format conforms to the JWT specification (RFC 7519).
*
* -
*
* Check if the ID Token is encrypted and if it is encrypted, then (a)
* reject the token exchange request when the {@link
* Service#isTokenExchangeEncryptedJwtRejected()
* tokenExchangeEncryptedJwtRejected} flag of the service is {@code true}
* or (b) skip remaining validation steps when the flag is {@code false}.
* Note that Authlete does not verify an encrypted ID Token because
* there is no standard way to obtain the key to decrypt the ID Token
* with in the context of token exchange where the client ID for the
* encrypted ID Token cannot be determined. This means that you must
* verify an encrypted ID Token by yourself when one is used as an
* input token with the token type
* {@code "urn:ietf:params:oauth:token-type:id_token"}.
*
* -
*
* Confirm that the ID Token contains the {@code exp} claim and the
* current time has not reached the time indicated by the claim.
*
* -
*
* Confirm that the ID Token contains the {@code iat} claim and the
* current time is equal to or after the time indicated by the claim.
*
* -
*
* Confirm that the current time is equal to or after the time indicated
* by the {@code nbf} claim if the ID Token contains the claim.
*
* -
*
* Confirm that the ID Token contains the {@code iss} claim and the
* value is a valid URI. In addition, confirm that the URI has the
* {@code https} scheme, no query component and no fragment component.
*
* -
*
* Confirm that the ID Token contains the {@code aud} claim and its
* value is a JSON string or an array of JSON strings.
*
* -
*
* Confirm that the value of the {@code nonce} claim is a JSON string
* if the ID Token contains the claim.
*
* -
*
* Check if the ID Token is signed and if it is not signed, then (a)
* reject the token exchange request when the {@link
* Service#isTokenExchangeUnsignedJwtRejected()
* tokenExchangeUnsignedJwtRejected} flag of the service is {@code true}
* or (b) finish validation on the input token.
*
* -
*
* Confirm that the signature algorithm is asymmetric. This implies that
* ID Tokens whose signature algorithm is symmetric ({@code HS256},
* {@code HS384} or {@code HS512}) cannot be used as a subject token or
* an actor token with the token type
* {@code urn:ietf:params:oauth:token-type:id_token}.
*
* -
*
* Verify the signature of the ID Token. Signature verification is
* performed even in the case where the issuer of the ID Token is not
* your service. But in that case, the issuer must support the discovery
* endpoint defined in OpenID
* Connect Discovery 1.0. Otherwise, signature verification fails.
*
*
*
*
*
* Token Type
* urn:ietf:params:oauth:token-type:saml1
*
*
*
*
* -
*
* (Authlete does not perform any validation for this token type.)
*
*
*
*
*
* Token Type
* urn:ietf:params:oauth:token-type:saml2
*
*
*
*
* -
*
* (Authlete does not perform any validation for this token type.)
*
*
*
*
*
*
*
* The specification of Token Exchange (RFC 8693) is very
* flexible. In other words, the specification has abandoned the task of
* determining details. Therefore, for secure token exchange, you have
* to complement the specification with your own rules. For that purpose,
* Authlete provides some configuration options as listed below.
* Authorization server implementers may utilize them and/or implement
* their own rules.
*
*
*
* -
*
* {@link Service#isTokenExchangeByIdentifiableClientsOnly()
* Service.tokenExchangeByIdentifiableClientsOnly} -
* whether to reject token exchange requests that contain no client
* identifier.
*
*
* -
*
* {@link Service#isTokenExchangeByConfidentialClientsOnly()
* Service.tokenExchangeByConfidentialClientsOnly} -
* whether to reject token exchange requests by public clients.
*
*
* -
*
* {@link Service#isTokenExchangeByPermittedClientsOnly()
* Service.tokenExchangeByPermittedClientsOnly} -
* whether to reject token exchange requests by clients that have no
* explicit permission.
*
*
* -
*
* {@link Service#isTokenExchangeEncryptedJwtRejected()
* Service.tokenExchangeEncryptedJwtRejected} -
* whether to reject token exchange requests which use encrypted JWTs
* as input tokens.
*
*
* -
*
* {@link Service#isTokenExchangeUnsignedJwtRejected()
* Service.tokenExchangeUnsignedJwtRejected} -
* whether to reject token exchange requests which use unsigned JWTs
* as input tokens.
*
*
*
*
* In the case of {@link Action#TOKEN_EXCHANGE TOKEN_EXCHANGE}, the {@link
* #getResponseContent()} method returns {@code null}. You have to construct
* the token response by yourself.
*
*
*
* For example, you may generate an access token by calling Authlete's
* {@code /api/auth/token/create} API and construct a token response like
* below.
*
*
*
* HTTP/1.1 200 OK
* Content-Type: application/json
* Cache-Control: no-cache, no-store
*
* {
* "access_token": "{@link TokenCreateResponse#getAccessToken()}",
* "issued_token_type": "urn:ietf:params:oauth:token-type:access_token",
* "token_type": "Bearer",
* "expires_in": {@link TokenCreateResponse#getExpiresIn()},
* "scope": "String.join(" ", {@link TokenCreateResponse#getScopes()})"
* }
*
*
* {@link Action#JWT_BEARER JWT_BEARER} (Authlete 2.3 onwards)
*
*
* When the value of {@code "action"} is {@code "JWT_BEARER"}, it means that
* the request from the client application is a valid token request with the
* grant type {@code "urn:ietf:params:oauth:grant-type:jwt-bearer"} (RFC 7523 JSON Web Token (JWT)
* Profile for OAuth 2.0 Client Authentication and Authorization Grants)
* and that the request has already passed the following validation steps.
*
*
*
* -
*
* Confirm that the {@code assertion} request parameter is given and its value
* is not empty.
*
*
* -
*
* Confirm that the format of the assertion conforms to the JWT specification
* (RFC 7519).
*
*
* -
*
* Check if the JWT is encrypted and if it is encrypted, then (a) reject the
* token request when the {@link Service#isJwtGrantEncryptedJwtRejected()
* jwtGrantEncryptedJwtRejected} flag of the service is {@code true} or (b)
* skip remaining validation steps when the flag is {@code false}. Note that
* Authlete does not verify an encrypted JWT because there is no standard way
* to obtain the key to decrypt the JWT with. This means that you must verify
* an encrypted JWT by yourself.
*
*
* -
*
* Confirm that the JWT contains the {@code iss} claim and its value is a
* JSON string.
*
*
* -
*
* Confirm that the JWT contains the {@code sub} claim and its value is a
* JSON string.
*
*
* -
*
* Confirm that the JWT contains the {@code aud} claim and its value is
* either a JSON string or an array of JSON strings.
*
*
* -
*
* Confirm that the issuer identifier of the service (cf. {@link Service#getIssuer()})
* or the URL of the token endpoint (cf. {@link Service#getTokenEndpoint()})
* is listed as audience in the {@code aud} claim.
*
*
* -
*
* Confirm that the JWT contains the {@code exp} claim and the current time
* has not reached the time indicated by the claim.
*
*
* -
*
* Confirm that the current time is equal to or after the time indicated by
* by the {@code iat} claim if the JWT contains the claim.
*
*
* -
*
* Confirm that the current time is equal to or after the time indicated by
* by the {@code nbf} claim if the JWT contains the claim.
*
*
* -
*
* Check if the JWT is signed and if it is not signed, then (a) reject the
* token request when the {@link Service#isJwtGrantUnsignedJwtRejected()
* jwtGrantUnsignedJwtRejected} flag of the service is {@code true} or (b)
* finish validation on the JWT. Note that Authlete does not verify the
* signature of the JWT because there is no standard way to obtain the key
* to verify the signature of a JWT with. This means that you must verify
* the signature by yourself.
*
*
*
*
* Authlete provides some configuration options for the grant type as listed
* below. Authorization server implementers may utilize them and/or implement
* their own rules.
*
*
*
* -
*
* {@link Service#isJwtGrantByIdentifiableClientsOnly()
* Service.jwtGrantByIdentifiableClientsOnly} -
* whether to reject token requests that use the grant type
* {@code "urn:ietf:params:oauth:grant-type:jwt-bearer"} but contain no client
* identifier.
*
*
* -
*
* {@link Service#isJwtGrantEncryptedJwtRejected()
* Service.jwtGrantEncryptedJwtRejected} -
* whether to reject token requests that use an encrypted JWT as an
* authorization grant with the grant type
* {@code "urn:ietf:params:oauth:grant-type:jwt-bearer"}.
*
*
* -
*
* {@link Service#isJwtGrantUnsignedJwtRejected()
* Service.jwtGrantUnsignedJwtRejected} -
* whether to reject token requests that use an unsigned JWT as an
* authorization grant with the grant type
* {@code "urn:ietf:params:oauth:grant-type:jwt-bearer"}.
*
*
*
*
* In the case of {@link Action#JWT_BEARER JWT_BEARER}, the {@link
* #getResponseContent()} method returns {@code null}. You have to construct
* the token response by yourself.
*
*
*
* For example, you may generate an access token by calling Authlete's
* {@code /api/auth/token/create} API and construct a token response like
* below.
*
*
*
* HTTP/1.1 200 OK
* Content-Type: application/json
* Cache-Control: no-cache, no-store
*
* {
* "access_token": "{@link TokenCreateResponse#getAccessToken()}",
* "token_type": "Bearer",
* "expires_in": {@link TokenCreateResponse#getExpiresIn()},
* "scope": "String.join(" ", {@link TokenCreateResponse#getScopes()})"
* }
*
*
* Finally, note again that Authlete does not verify the signature of the JWT
* specified by the {@code assertion} request parameter. You must verify the
* signature by yourself.
*
*
*
* {@link Action#ID_TOKEN_REISSUABLE ID_TOKEN_REISSUABLE} (Authlete 2.3.8 onwards)
*
*
* The "{@code action}" value "{@code ID_TOKEN_REISSUABLE}" indicates that an
* ID token can be reissued by the token request. This {@code action} value
* is returned when the following conditions are met.
*
*
*
* - The service's "{@code idTokenReissuable}" property is {@code true} (cf.
* {@link Service#isIdTokenReissuable()}).
*
- The flow of the token request is the refresh token flow.
*
- The scope set after processing the token request still contains the
* "{@code openid}" scope.
*
- The access token is associated with the subject of a user.
*
- The access token is associated with a client application.
*
*
*
* When receiving this {@code action} value, the implementation of the token
* endpoint can take either of the following actions.
*
*
*
* - Execute the same steps as for the case of the "{@code OK}" action. This
* will result in that the token endpoint behaves as before, and no ID
* token is reissued.
*
- Call Authlete's {@code /idtoken/reissue} API to reissue an ID token
* and let the API prepare a token response including a new ID token
* together with the new access token and a refresh token.
*
*
*
* If you choose to reissue a new ID token, the following steps should be taken.
*
*
*
* - Identify the user associated with the access token based on the
* "{@code subject}" parameter in the response from the {@code /auth/token}
* API (cf. {@link #getSubject()}).
*
- Get the list of requested claims for ID tokens by referring to the value
* of the "{@code requestedIdTokenClaims}" parameter in the response from
* the {@code /auth/token} API. Note that, however, the parameter always
* holds null when the Authlete server you are using is older than the
* version 3.0. See the description of the {@link #getRequestedIdTokenClaims()}
* method for details.
*
- Get the values of the requested claims of the user from your user
* database.
*
- Construct a JSON object that includes the name-value pairs of the
* requested claims. The JSON is used as the value of the "{@code claims}"
* request parameter passed to the {@code /idtoken/reissue} API.
*
- Select the representation of the access token based on the following
* logic: if the value of the "{@code jwtAccessToken}" parameter (cf.
* {@link #getJwtAccessToken()}) is not null, use the value. Otherwise,
* use the value of the "{@code accessToken}" parameter (cf. {@link
* #getAccessToken()}). The selected representation is used as the value
* of the "{@code accessToken}" parameter passed to the
* {@code /idtoken/reissue} API.
*
- Get the value of the refresh token (cf. {@link #getRefreshToken()}).
* The value is used as the value of the "{@code refreshToken}" parameter
* passed to the {@code /idtoken/reissue} API.
*
- Call the {@code /idtoken/reissue} API and follow the instruction of
* the API. See the descriptions of the {@link IDTokenReissueRequest}
* class and the {@link IDTokenReissueResponse} class for details.
*
*
*
* DPoP Nonce (Authlete 3.0 onwards)
*
*
* Since version 3.0, Authlete recognizes the {@code nonce} claim in DPoP
* proof JWTs. If the {@code nonce} claim is required (= if the service's
* {@code dpopNonceRequired} property is {@code true}, or the value of the
* {@code dpopNonceRequired} request parameter passed to the Authlete API
* is {@code true}), the Authlete API checks whether the {@code nonce}
* claim in the presented DPoP proof JWT is identical to the expected value.
*
*
*
* If the {@code dpopNonce} response parameter from the API is not null, its
* value is the expected nonce value for DPoP proof JWT. The expected value
* needs to be conveyed to the client application as the value of the
* {@code DPoP-Nonce} HTTP header.
*
*
* DPoP-Nonce: (The value returned from {@link #getDpopNonce()})
*
*
* See RFC 9449 OAuth
* 2.0 Demonstrating Proof of Possession (DPoP) for details.
*
*
* @see RFC 6749 The OAuth 2.0 Authorization Framework
*
* @see RFC 7521
* Assertion Framework for OAuth 2.0 Client Authentication and
* Authorization Grants
*
* @see RFC 7523
* JSON Web Token (JWT) Profile for OAuth 2.0 Client Authentication
* and Authorization Grants
*
* @see RFC 8693 OAuth 2.0 Token Exchange
*
* @see RFC 9449 OAuth 2.0 Demonstrating Proof of Possession (DPoP)
*/
public class TokenResponse extends ApiResponse
{
private static final long serialVersionUID = 20L;
/**
* The next action that the service implementation should take.
*/
public enum Action
{
/**
* Authentication of the client application failed. The service
* implementation should return either {@code "400 Bad Request"}
* or {@code "401 Unauthorized"} to the client application.
*/
INVALID_CLIENT,
/**
* The request from the service was wrong or an error occurred
* in Authlete. The service implementation should return {@code
* "500 Internal Server Error"} to the client application.
*/
INTERNAL_SERVER_ERROR,
/**
* The token request from the client was wrong. The service
* implementation should return {@code "400 Bad Request"} to the
* client application.
*/
BAD_REQUEST,
/**
* The token request from the client application was valid and
* the grant type is {@code "password"}. The service implementation
* should validate the credentials of the resource owner and call
* Authlete's {@code /auth/token/issue} API or {@code /auth/token/fail}
* API according to the result of the validation.
*/
PASSWORD,
/**
* The token request from the client was valid. The service
* implementation should return {@code "200 OK"} to the client
* application with an access token.
*/
OK,
/**
* The token request from the client was a valid token exchange
* request. The service implementation should take necessary
* actions (e.g. create an access token), generate a response and
* return it to the client application.
*
* @see RFC 8693 OAuth 2.0 Token Exchange
*
* @since 3.26
* @since Authlete 2.3
*/
TOKEN_EXCHANGE,
/**
* The token request from the client was a valid token request with
* the grant type {@code "urn:ietf:params:oauth:grant-type:jwt-bearer"}.
* The service implementation must verify the signature of the assertion,
* create an access token, generate a response and return it to the
* client application.
*
* @see RFC 7521
* Assertion Framework for OAuth 2.0 Client Authentication and
* Authorization Grants
*
* @see RFC 7523
* JSON Web Token (JWT) Profile for OAuth 2.0 Client Authentication
* and Authorization Grants
*
* @since 3.30
* @since Authlete 2.3
*/
JWT_BEARER,
/**
* The token request from the client was a valid token request using
* the refresh token flow and an ID token can be reissued.
*
*
* The implementation of the token endpoint can choose either (1) to
* execute the same steps as for the "{@code OK}" action which results
* in the same behavior as before, or (2) to call the
* {@code /idtoken/reissue} API to reissue a new ID token together with
* a new access token and a refresh token.
*
*
*
* See the description of the {@link TokenResponse} class for details.
*
*
* @since 3.68
* @since Authlete 2.3.8
* @since Authlete 3.0
*/
ID_TOKEN_REISSUABLE,
}
private static final String SUMMARY_FORMAT
= "action=%s, username=%s, password=%s, ticket=%s, responseContent=%s, "
+ "accessToken=%s, accessTokenExpiresAt=%d, accessTokenDuration=%d, "
+ "refreshToken=%s, refreshTokenExpiresAt=%d, refreshTokenDuration=%d, "
+ "idToken=%s, grantType=%s, "
+ "clientId=%d, clientIdAlias=%s, clientIdAliasUsed=%s, "
+ "subject=%s, scopes=%s, properties=%s, jwtAccessToken=%s, "
+ "clientAuthMethod=%s";
/**
* @since Authlete 1.1
*/
private Action action;
/**
* @since Authlete 1.1
*/
private String responseContent;
/**
* @since Authlete 1.1
*/
private String username;
/**
* @since Authlete 1.1
*/
private String password;
/**
* @since Authlete 1.1
*/
private String ticket;
/**
* @since Authlete 2.2.0
*/
private String accessToken;
/**
* @since Authlete 2.2.0
*/
private long accessTokenExpiresAt;
/**
* @since Authlete 2.2.0
*/
private long accessTokenDuration;
/**
* @since Authlete 2.2.0
*/
private String refreshToken;
/**
* @since Authlete 2.2.0
*/
private long refreshTokenExpiresAt;
/**
* @since Authlete 2.2.0
*/
private long refreshTokenDuration;
/**
* @since Authlete 2.2.0
*/
private String idToken;
/**
* @since Authlete 1.1.9
*/
private GrantType grantType;
/**
* @since Authlete 1.1.9
*/
private long clientId;
/**
* @since Authlete 1.1.9
*/
private String clientIdAlias;
/**
* @since Authlete 1.1.9
*/
private boolean clientIdAliasUsed;
/**
* @since Authlete 2.3
*/
private URI clientEntityId;
/**
* @since Authlete 2.3
*/
private boolean clientEntityIdUsed;
/**
* @since Authlete 1.1.9
*/
private String subject;
/**
* @since Authlete 1.1.9
*/
private String[] scopes;
/**
* @since Authlete 1.1.9
*/
private Property[] properties;
/**
* @since Authlete 2.1
*/
private String jwtAccessToken;
/**
* @since Authlete 2.3.13
*/
private ClientAuthMethod clientAuthMethod;
/**
* @since Authlete 2.2.1
*/
private URI[] resources;
/**
* @since Authlete 2.2.0
*/
private URI[] accessTokenResources;
/**
* @since Authlete 2.2.0
*/
private AuthzDetails authorizationDetails;
/**
* @since Authlete 2.3.0
*/
private String grantId;
/**
* @since Authlete 2.2.3
*/
private Pair[] serviceAttributes;
/**
* @since Authlete 2.2.3
*/
private Pair[] clientAttributes;
/**
* For RFC 8693 OAuth 2.0 Token Exchange
*
* @since Authlete 2.3
*/
private String[] audiences;
/**
* @since Authlete 2.3.0
*/
private TokenType requestedTokenType;
/**
* @since Authlete 2.3.0
*/
private String subjectToken;
/**
* @since Authlete 2.3.0
*/
private TokenType subjectTokenType;
/**
* @since Authlete 2.3.0
*/
private TokenInfo subjectTokenInfo;
/**
* @since Authlete 2.3.0
*/
private String actorToken;
/**
* @since Authlete 2.3.0
*/
private TokenType actorTokenType;
/**
* @since Authlete 2.3.0
*/
private TokenInfo actorTokenInfo;
/**
* For RFC 7523 JSON Web Token (JWT) Profile for OAuth 2.0
* Client Authentication and Authorization Grants
* @since Authlete 2.3.0
*/
private String assertion;
/**
* A flag indicating whether the previous refresh token that had been kept
* in the database for a short time was used.
* @since Authlete 2.3.0
*/
private boolean previousRefreshTokenUsed;
/**
* The {@code c_nonce}.
*
*
* The {@code cNonce} field added by the version 3.63 has been renamed
* to {@code cnonce} by the version 3.90.
*
*
* @since 3.90
* @since Authlete 3.0.0
*/
private String cnonce;
/**
* The time at which the {@code c_nonce} expires.
*
*
* The {@code cNonceExpiresAt} field added by the version 3.63 has been
* renamed to {@code cnonceExpiresAt} by the version 3.90.
*
*
* @since 3.90
* @since Authlete 3.0.0
*/
private long cnonceExpiresAt;
/**
* The duration of {@code c_nonce}.
*
*
* The {@code cNonceDuration} field added by the version 3.63 has been
* renamed to {@code cnonceDuration} by the version 3.90.
*
*
* @since 3.90
* @since Authlete 3.0.0
*/
private long cnonceDuration;
/**
* The names of the claims that the authorization request (which resulted in
* generation of the access token) requested to be embedded in ID tokens.
*
* @since 3.68
* @since Authlete 3.0
*/
private String[] requestedIdTokenClaims;
/**
* The expected nonce value for DPoP proof JWT, which should be used
* as the value of the {@code DPoP-Nonce} HTTP header.
*
* @since 3.82
* @since Authlete 3.0
*/
private String dpopNonce;
/**
* Scopes associated with the refresh token.
*
* @since 3.89
* @since Authlete 3.0.0
*/
private String[] refreshTokenScopes;
/**
* Get the next action that the service implementation should take.
*/
public Action getAction()
{
return action;
}
/**
* Set the next action that the service implementation should take.
*/
public void setAction(Action action)
{
this.action = action;
}
/**
* Get the response content which can be used as the entity body
* of the response returned to the client application.
*/
public String getResponseContent()
{
return responseContent;
}
/**
* Set the response content which can be used as the entity body
* of the response returned to the client application.
*/
public void setResponseContent(String responseContent)
{
this.responseContent = responseContent;
}
/**
* Get the value of {@code "username"} request parameter.
*
*
* This method returns a non-null value only when the value of
* {@code "grant_type"} request parameter in the token request
* is {@code "password"}.
*
*
*
* {@code getSubject()} method was renamed to {@code getUsername()}
* on version 1.13.
*
*
* @since 1.13
*
* @see RFC 6749, 4.3.2. Access Token Request
*/
public String getUsername()
{
return username;
}
/**
* Set the value of {@code "username"} request parameter.
*
*
* {@code setSubject(String}} was renamed to {@code setUsername(String)}
* on version 1.13.
*
*
* @since 1.13
*/
public void setUsername(String username)
{
this.username = username;
}
/**
* Get the value of {@code "password"} request parameter.
*
*
* This method returns a non-null value only when the value of
* {@code "grant_type"} request parameter in the token request
* is {@code "password"}.
*
*
* @see RFC 6749, 4.3.2. Access Token Request
*/
public String getPassword()
{
return password;
}
/**
* Set the value of {@code "password"} request parameter.
*/
public void setPassword(String password)
{
this.password = password;
}
/**
* Get the ticket issued from Authlete's {@code /auth/token} endpoint.
* The value is to be used as the value of {@code "ticket"} request
* parameter for {@code /auth/token/issue} API or {@code /auth/token/fail}
* API.
*
*
* This method returns a non-null value only when {@code "action"} is
* {@link Action#PASSWORD PASSWORD}.
*
*/
public String getTicket()
{
return ticket;
}
/**
* Set the ticket used for {@code /auth/token/issue} API or {@code
* /auth/token/fail} API.
*/
public void setTicket(String ticket)
{
this.ticket = ticket;
}
/**
* Get the summary of this instance.
*/
public String summarize()
{
return String.format(SUMMARY_FORMAT,
action, username, password, ticket, responseContent,
accessToken, accessTokenExpiresAt, accessTokenDuration,
refreshToken, refreshTokenExpiresAt, refreshTokenDuration,
idToken, grantType, clientId, clientIdAlias, clientIdAliasUsed,
subject, Utils.join(scopes, " "),
Utils.stringifyProperties(properties), jwtAccessToken,
clientAuthMethod);
}
/**
* Get the newly issued access token. This method returns a non-null
* value only when {@link #getAction()} returns {@link Action#OK}.
*
*
* If the service is configured to issue JWT-based access tokens,
* a JWT-based access token is issued additionally. In the case,
* {@link #getJwtAccessToken()} returns the JWT-based access token.
*
*
* @return
* The newly issued access token.
*
* @see #getJwtAccessToken()
*
* @since 1.34
*/
public String getAccessToken()
{
return accessToken;
}
/**
* Set the newly issued access token.
*
* @param accessToken
* The newly issued access token.
*
* @since 1.34
*/
public void setAccessToken(String accessToken)
{
this.accessToken = accessToken;
}
/**
* Get the date in milliseconds since the Unix epoch (1970-01-01)
* at which the access token will expire.
*
* @return
* The expiration date in milliseconds since the Unix epoch
* (1970-01-01) at which the access token will expire.
*
* @since 1.34
*/
public long getAccessTokenExpiresAt()
{
return accessTokenExpiresAt;
}
/**
* Set the date in milliseconds since the Unix epoch (1970-01-01)
* at which the access token will expire.
*
* @param expiresAt
* The expiration date in milliseconds since the Unix epoch
* (1970-01-01) at which the access token will expire.
*
* @since 1.34
*/
public void setAccessTokenExpiresAt(long expiresAt)
{
this.accessTokenExpiresAt = expiresAt;
}
/**
* Get the duration of the access token in seconds.
*
* @return
* Duration in seconds.
*
* @since 1.34
*/
public long getAccessTokenDuration()
{
return accessTokenDuration;
}
/**
* Set the duration of the access token in seconds.
*
* @param duration
* Duration in seconds.
*
* @since 1.34
*/
public void setAccessTokenDuration(long duration)
{
this.accessTokenDuration = duration;
}
/**
* Get the newly issued refresh token. This method returns a non-null
* value only when {@link #getAction()} returns {@link Action#OK} and
* the service supports the refresh token flow.
*
* @return
* The newly issued refresh token.
*
* @since 1.34
*/
public String getRefreshToken()
{
return refreshToken;
}
/**
* Set the newly issued refresh token.
*
* @param refreshToken
* The newly issued refresh token.
*
* @since 1.34
*/
public void setRefreshToken(String refreshToken)
{
this.refreshToken = refreshToken;
}
/**
* Get the date in milliseconds since the Unix epoch (1970-01-01)
* at which the refresh token will expire.
*
* @return
* The expiration date in milliseconds since the Unix epoch
* (1970-01-01) at which the refresh token will expire.
* If the refresh token is null, this method returns 0.
*
* @since 1.34
*/
public long getRefreshTokenExpiresAt()
{
return refreshTokenExpiresAt;
}
/**
* Set the date in milliseconds since the Unix epoch (1970-01-01)
* at which the refresh token will expire.
*
* @param expiresAt
* The expiration date in milliseconds since the Unix epoch
* (1970-01-01) at which the refresh token will expire.
* If the refresh token is null, this method returns 0.
*
* @since 1.34
*/
public void setRefreshTokenExpiresAt(long expiresAt)
{
this.refreshTokenExpiresAt = expiresAt;
}
/**
* Get the duration of the refresh token in seconds.
*
* @return
* Duration in seconds.
*
* @since 1.34
*/
public long getRefreshTokenDuration()
{
return refreshTokenDuration;
}
/**
* Set the duration of the refresh token in seconds.
*
* @param duration
* Duration in seconds.
*
* @since 1.34
*/
public void setRefreshTokenDuration(long duration)
{
this.refreshTokenDuration = duration;
}
/**
* Get the ID token.
*
*
* An ID token is issued from a token endpoint when the authorization code
* flow is used and "openid"
is included in the scope list.
*
*
* @return
* ID token.
*
* @see Authentication using the Authorization Code Flow
*
* @since 1.34
*/
public String getIdToken()
{
return idToken;
}
/**
* Set the ID token.
*
* @param idToken
* ID token.
*
* @since 1.34
*/
public void setIdToken(String idToken)
{
this.idToken = idToken;
}
/**
* Get the grant type of the token request.
*
* @since 2.8
*/
public GrantType getGrantType()
{
return grantType;
}
/**
* Set the grant type of the token request.
*
* @param grantType
* Grant type of the token request.
*
* @since 2.8
*/
public void setGrantType(GrantType grantType)
{
this.grantType = grantType;
}
/**
* Get the client ID.
*
* @since 2.8
*/
public long getClientId()
{
return clientId;
}
/**
* Set the client ID.
*
* @since 2.8
*/
public void setClientId(long clientId)
{
this.clientId = clientId;
}
/**
* Get the client ID alias when the token request was made.
*
*
* If the client did not have an alias, this method returns
* {@code null}. Also, if the token request was invalid and
* it failed to identify a client, this method returns
* {@code null}.
*
*
* @return
* The client ID alias.
*
* @since 2.8
*/
public String getClientIdAlias()
{
return clientIdAlias;
}
/**
* Set the client ID alias when the token request was made.
*
* @param alias
* The client ID alias.
*
* @since 2.8
*/
public void setClientIdAlias(String alias)
{
this.clientIdAlias = alias;
}
/**
* Get the flag which indicates whether the client ID alias was used
* when the token request was made.
*
* @return
* {@code true} if the client ID alias was used when the token
* request was made.
*
* @since 2.8
*/
public boolean isClientIdAliasUsed()
{
return clientIdAliasUsed;
}
/**
* Set the flag which indicates whether the client ID alias was used
* when the token request was made.
*
* @param used
* {@code true} if the client ID alias was used when the token
* request was made.
*
* @since 2.8
*/
public void setClientIdAliasUsed(boolean used)
{
this.clientIdAliasUsed = used;
}
/**
* Get the entity ID of the client.
*
*
* "Entity ID" is a technical term defined in OpenID
* Federation 1.0.
*
*
* @return
* The entity ID of the client.
*
* @since 3.37
* @since Authlete 2.3
*
* @see OpenID Federation 1.0
*/
public URI getClientEntityId()
{
return clientEntityId;
}
/**
* Set the entity ID of the client.
*
*
* "Entity ID" is a technical term defined in OpenID
* Federation 1.0.
*
*
* @param entityId
* The entity ID of the client.
*
* @since 3.37
* @since Authlete 2.3
*
* @see OpenID Federation 1.0
*/
public void setClientEntityId(URI entityId)
{
this.clientEntityId = entityId;
}
/**
* Get the flag which indicates whether the entity ID of the client was
* used when the request for the access token was made.
*
*
* "Entity ID" is a technical term defined in OpenID
* Federation 1.0.
*
*
* @return
* {@code true} if the entity ID of the client was used when the
* request for the access token was made.
*
* @since 3.37
* @since Authlete 2.3
*
* @see OpenID Federation 1.0
*/
public boolean isClientEntityIdUsed()
{
return clientEntityIdUsed;
}
/**
* Set the flag which indicates whether the entity ID of the client was
* used when the request for the access token was made.
*
*
* "Entity ID" is a technical term defined in OpenID
* Federation 1.0.
*
*
* @param used
* {@code true} to indicate that the entity ID of the client was
* used when the request for the access token was made.
*
* @since 3.37
* @since Authlete 2.3
*
* @see OpenID Federation 1.0
*/
public void setClientEntityIdUsed(boolean used)
{
this.clientEntityIdUsed = used;
}
/**
* Get the subject (= resource owner's ID) of the access token.
*
*
* Even if an access token has been issued by the call of
* {@code /api/auth/token} API, this method returns {@code null}
* if the flow of the token request was Client
* Credentials Flow ({@code grant_type=client_credentials})
* because it means the access token is not associated with any
* specific end-user.
*
*
* @since 2.8
*/
public String getSubject()
{
return subject;
}
/**
* Set the subject (= resource owner's ID) of the access token.
*
* @since 2.8
*/
public void setSubject(String subject)
{
this.subject = subject;
}
/**
* Get the scopes covered by the access token.
*
* @since 2.8
*/
public String[] getScopes()
{
return scopes;
}
/**
* Set the scopes covered by the access token.
*
* @since 2.8
*/
public void setScopes(String[] scopes)
{
this.scopes = scopes;
}
/**
* Get the extra properties associated with the access token.
* This method returns {@code null} when no extra property is
* associated with the issued access token.
*
* @return
* Extra properties associated with the issued access token.
*
* @since 2.8
*/
public Property[] getProperties()
{
return properties;
}
/**
* Set the extra properties associated with the access token.
*
* @param properties
* Extra properties.
*
* @since 2.8
*/
public void setProperties(Property[] properties)
{
this.properties = properties;
}
/**
* Get the newly issued access token in JWT format.
*
*
* If the authorization server is configured to issue JWT-based access
* tokens (= if {@link Service#getAccessTokenSignAlg()} returns a non-null
* value), a JWT-based access token is issued along with the original
* random-string one.
*
*
*
* Regarding the detailed format of the JWT-based access token, see the
* description of the {@link Service} class.
*
*
* @return
* The newly issued access token in JWT format. If the service is
* not configured to issue JWT-based access tokens, this method
* always returns null.
*
* @see #getAccessToken()
*
* @since 2.37
*/
public String getJwtAccessToken()
{
return jwtAccessToken;
}
/**
* Set the newly issued access token in JWT format.
*
* @param jwtAccessToken
* The newly issued access token in JWT format.
*
* @since 2.37
*/
public void setJwtAccessToken(String jwtAccessToken)
{
this.jwtAccessToken = jwtAccessToken;
}
/**
* Get the client authentication method that should be performed at the
* token endpoint.
*
*
* If the client could not be identified by the information in the request,
* this method returns {@code null}.
*
*
* @return
* The client authentication method that should be performed at
* the token endpoint.
*
* @since 2.50
*/
public ClientAuthMethod getClientAuthMethod()
{
return clientAuthMethod;
}
/**
* Set the client authentication method that should be performed at the
* token endpoint.
*
* @param method
* The client authentication method that should be performed at
* the token endpoint.
*
* @since 2.50
*/
public void setClientAuthMethod(ClientAuthMethod method)
{
this.clientAuthMethod = method;
}
/**
* Get the resources specified by the {@code resource} request parameters
* in the token request.
*
*
* See "Resource Indicators for OAuth 2.0" for details.
*
*
* @return
* Resources specified by the {@code resource} request parameters
* in the token request.
*
* @since 2.62
*/
public URI[] getResources()
{
return resources;
}
/**
* Set the resources specified by the {@code resource} request parameters
* in the token request.
*
*
* See "Resource Indicators for OAuth 2.0" for details.
*
*
* @param resources
* Resources specified by the {@code resource} request parameters
* in the token request.
*
* @since 2.62
*/
public void setResources(URI[] resources)
{
this.resources = resources;
}
/**
* Get the target resources of the access token being issued.
*
*
* See "Resource Indicators for OAuth 2.0" for details.
*
*
* @return
* The target resources of the access token.
*
* @since 2.62
*/
public URI[] getAccessTokenResources()
{
return accessTokenResources;
}
/**
* Set the target resources of the access token being issued.
*
*
* See "Resource Indicators for OAuth 2.0" for details.
*
*
* @param resources
* The target resources of the access token.
*
* @since 2.62
*/
public void setAccessTokenResources(URI[] resources)
{
this.accessTokenResources = resources;
}
/**
* Get the authorization details. This represents the value of the
* {@code "authorization_details"} request parameter which is defined in
* "OAuth 2.0 Rich Authorization Requests".
*
*
* When the {@code action} (= the value returned from {@link #getAction()}
* is {@link Action#PASSWORD PASSWORD}, this method returns an array that
* represents the {@code authorization_details} request parameter included
* in the token request. In other successful cases, this method returns the
* authorization details associated with the issued access token.
*
*
* @return
* Authorization details.
*
* @since 2.56
*/
public AuthzDetails getAuthorizationDetails()
{
return authorizationDetails;
}
/**
* Set the authorization details. This represents the value of the
* {@code "authorization_details"} request parameter which is defined in
* "OAuth 2.0 Rich Authorization Requests".
*
* @param details
* Authorization details.
*
* @since 2.56
*/
public void setAuthorizationDetails(AuthzDetails details)
{
this.authorizationDetails = details;
}
/**
* Get the value of the {@code grant_id} parameter in the token response.
*
* @return
* The value of the {@code grant_id} response parameter.
*
* @see Grant Management for OAuth 2.0
*
* @since 3.1
*/
public String getGrantId()
{
return grantId;
}
/**
* Set the value of the {@code grant_id} parameter in the token response.
*
* @param grantId
* The value of the {@code grant_id} response parameter.
*
* @see Grant Management for OAuth 2.0
*
* @since 3.1
*/
public void setGrantId(String grantId)
{
this.grantId = grantId;
}
/**
* Get the attributes of the service that the client application belongs to.
*
*
* This property is available since Authlete 2.2.
*
*
* @return
* The attributes of the service.
*
* @since 2.88
*/
public Pair[] getServiceAttributes()
{
return serviceAttributes;
}
/**
* Set the attributes of the service that the client application belongs to.
*
*
* This property is available since Authlete 2.2.
*
*
* @param attributes
* The attributes of the service.
*
* @since 2.88
*/
public void setServiceAttributes(Pair[] attributes)
{
this.serviceAttributes = attributes;
}
/**
* Get the attributes of the client.
*
*
* This property is available since Authlete 2.2.
*
*
* @return
* The attributes of the client.
*
* @since 2.88
*/
public Pair[] getClientAttributes()
{
return clientAttributes;
}
/**
* Set the attributes of the client.
*
*
* This property is available since Authlete 2.2.
*
*
* @param attributes
* The attributes of the client.
*
* @since 2.88
*/
public void setClientAttributes(Pair[] attributes)
{
this.clientAttributes = attributes;
}
/**
* Get the values of the {@code audience} request parameters that are
* contained in the token exchange request (cf. RFC 8693).
*
*
* The {@code audience} request parameter is defined in RFC 8693 OAuth 2.0 Token
* Exchange. Although RFC 6749 The OAuth 2.0
* Authorization Framework states "Request and response parameters
* MUST NOT be included more than once", RFC 8693 allows a token
* exchange request to include the {@code audience} request parameter
* multiple times.
*
*
* @return
* The values of the {@code audience} request parameters.
*
* @see RFC 8693 OAuth 2.0 Token Exchange
*
* @since 3.26
* @since Authlete 2.3
*/
public String[] getAudiences()
{
return audiences;
}
/**
* Set the values of the {@code audience} request parameters that are
* contained in the token exchange request (cf. RFC 8693).
*
*
* The {@code audience} request parameter is defined in RFC 8693 OAuth 2.0 Token
* Exchange. Although RFC 6749 The OAuth 2.0
* Authorization Framework states "Request and response parameters
* MUST NOT be included more than once", RFC 8693 allows a token
* exchange request to include the {@code audience} request parameter
* multiple times.
*
*
* @param audiences
* The values of the {@code audience} request parameters.
*
* @see RFC 8693 OAuth 2.0 Token Exchange
*
* @since 3.26
* @since Authlete 2.3
*/
public void setAudiences(String[] audiences)
{
this.audiences = audiences;
}
/**
* Get the value of the {@code requested_token_type} request parameter.
*
*
* The {@code requested_token_type} request parameter is defined in
* RFC 8693 OAuth
* 2.0 Token Exchange.
*
*
* @return
* The value of the {@code requested_token_type} request parameter.
*
* @see RFC 8693 OAuth 2.0 Token Exchange
*
* @since 3.26
* @since Authlete 2.3
*/
public TokenType getRequestedTokenType()
{
return requestedTokenType;
}
/**
* Set the value of the {@code requested_token_type} request parameter.
*
*
* The {@code requested_token_type} request parameter is defined in
* RFC 8693 OAuth
* 2.0 Token Exchange.
*
*
* @param tokenType
* The value of the {@code requested_token_type} request parameter.
*
* @see RFC 8693 OAuth 2.0 Token Exchange
*
* @since 3.26
* @since Authlete 2.3
*/
public void setRequestedTokenType(TokenType tokenType)
{
this.requestedTokenType = tokenType;
}
/**
* Get the value of the {@code subject_token} request parameter.
*
*
* The {@code subject_token} request parameter is defined in
* RFC 8693 OAuth
* 2.0 Token Exchange.
*
*
* @return
* The value of the {@code subject_token} request parameter.
*
* @see RFC 8693 OAuth 2.0 Token Exchange
*
* @since 3.26
* @since Authlete 2.3
*/
public String getSubjectToken()
{
return subjectToken;
}
/**
* Set the value of the {@code subject_token} request parameter.
*
*
* The {@code subject_token} request parameter is defined in
* RFC 8693 OAuth
* 2.0 Token Exchange.
*
*
* @param token
* The value of the {@code subject_token} request parameter.
*
* @see RFC 8693 OAuth 2.0 Token Exchange
*
* @since 3.26
* @since Authlete 2.3
*/
public void setSubjectToken(String token)
{
this.subjectToken = token;
}
/**
* Get the value of the {@code subject_token_type} request parameter.
*
*
* The {@code subject_token_type} request parameter is defined in
* RFC 8693 OAuth
* 2.0 Token Exchange.
*
*
* @return
* The value of the {@code subject_token_type} request parameter.
*
* @see RFC 8693 OAuth 2.0 Token Exchange
*
* @since 3.26
* @since Authlete 2.3
*/
public TokenType getSubjectTokenType()
{
return subjectTokenType;
}
/**
* Set the value of the {@code subject_token_type} request parameter.
*
*
* The {@code subject_token_type} request parameter is defined in
* RFC 8693 OAuth
* 2.0 Token Exchange.
*
*
* @param tokenType
* The value of the {@code subject_token_type} request parameter.
*
* @see RFC 8693 OAuth 2.0 Token Exchange
*
* @since 3.26
* @since Authlete 2.3
*/
public void setSubjectTokenType(TokenType tokenType)
{
this.subjectTokenType = tokenType;
}
/**
* Get the information about the token specified by the
* {@code subject_token} request parameter.
*
*
* This property holds a non-null value only when the value of the
* {@code subject_token_type} request parameter is either
* {@code "urn:ietf:params:oauth:token-type:access_token"} or
* {@code "urn:ietf:params:oauth:token-type:refresh_token"} (= only
* when the {@code subjectTokenType} property is either
* "{@link TokenType#ACCESS_TOKEN ACCESS_TOKEN}"
or
* "{@link TokenType#REFRESH_TOKEN REFRESH_TOKEN}"
).
*
*
* @return
* The information about the token specified by the
* {@code subject_token} request parameter.
*
* @see RFC 8693 OAuth 2.0 Token Exchange
*
* @since 3.26
* @since Authlete 2.3
*/
public TokenInfo getSubjectTokenInfo()
{
return subjectTokenInfo;
}
/**
* Set the information about the token specified by the
* {@code subject_token} request parameter.
*
*
* This property holds a non-null value only when the value of the
* {@code subject_token_type} request parameter is either
* {@code "urn:ietf:params:oauth:token-type:access_token"} or
* {@code "urn:ietf:params:oauth:token-type:refresh_token"} (= only
* when the {@code subjectTokenType} property is either
* "{@link TokenType#ACCESS_TOKEN ACCESS_TOKEN}"
or
* "{@link TokenType#REFRESH_TOKEN REFRESH_TOKEN}"
).
*
*
* @param tokenInfo
* The information about the token specified by the
* {@code subject_token} request parameter.
*
* @see RFC 8693 OAuth 2.0 Token Exchange
*
* @since 3.26
* @since Authlete 2.3
*/
public void setSubjectTokenInfo(TokenInfo tokenInfo)
{
this.subjectTokenInfo = tokenInfo;
}
/**
* Get the value of the {@code actor_token} request parameter.
*
*
* The {@code actor_token} request parameter is defined in
* RFC 8693 OAuth
* 2.0 Token Exchange.
*
*
* @return
* The value of the {@code actor_token} request parameter.
*
* @see RFC 8693 OAuth 2.0 Token Exchange
*
* @since 3.26
* @since Authlete 2.3
*/
public String getActorToken()
{
return actorToken;
}
/**
* Set the value of the {@code actor_token} request parameter.
*
*
* The {@code actor_token} request parameter is defined in
* RFC 8693 OAuth
* 2.0 Token Exchange.
*
*
* @param token
* The value of the {@code actor_token} request parameter.
*
* @see RFC 8693 OAuth 2.0 Token Exchange
*
* @since 3.26
* @since Authlete 2.3
*/
public void setActorToken(String token)
{
this.actorToken = token;
}
/**
* Get the value of the {@code actor_token_type} request parameter.
*
*
* The {@code actor_token_type} request parameter is defined in
* RFC 8693 OAuth
* 2.0 Token Exchange.
*
*
* @return
* The value of the {@code actor_token_type} request parameter.
*
* @see RFC 8693 OAuth 2.0 Token Exchange
*
* @since 3.26
* @since Authlete 2.3
*/
public TokenType getActorTokenType()
{
return actorTokenType;
}
/**
* Set the value of the {@code actor_token_type} request parameter.
*
*
* The {@code actor_token_type} request parameter is defined in
* RFC 8693 OAuth
* 2.0 Token Exchange.
*
*
* @param tokenType
* The value of the {@code actor_token_type} request parameter.
*
* @see RFC 8693 OAuth 2.0 Token Exchange
*
* @since 3.26
* @since Authlete 2.3
*/
public void setActorTokenType(TokenType tokenType)
{
this.actorTokenType = tokenType;
}
/**
* Get the information about the token specified by the
* {@code actor_token} request parameter.
*
*
* This property holds a non-null value only when the value of the
* {@code actor_token_type} request parameter is either
* {@code "urn:ietf:params:oauth:token-type:access_token"} or
* {@code "urn:ietf:params:oauth:token-type:refresh_token"} (= only
* when the {@code actorTokenType} property is either
* "{@link TokenType#ACCESS_TOKEN ACCESS_TOKEN}"
or
* "{@link TokenType#REFRESH_TOKEN REFRESH_TOKEN}"
).
*
*
* @return
* The information about the token specified by the
* {@code actor_token} request parameter.
*
* @see RFC 8693 OAuth 2.0 Token Exchange
*
* @since 3.26
* @since Authlete 2.3
*/
public TokenInfo getActorTokenInfo()
{
return actorTokenInfo;
}
/**
* Set the information about the token specified by the
* {@code actor_token} request parameter.
*
*
* This property holds a non-null value only when the value of the
* {@code actor_token_type} request parameter is either
* {@code "urn:ietf:params:oauth:token-type:access_token"} or
* {@code "urn:ietf:params:oauth:token-type:refresh_token"} (= only
* when the {@code actorTokenType} property is either
* "{@link TokenType#ACCESS_TOKEN ACCESS_TOKEN}"
or
* "{@link TokenType#REFRESH_TOKEN REFRESH_TOKEN}"
).
*
*
* @param tokenInfo
* The information about the token specified by the
* {@code actor_token} request parameter.
*
* @see RFC 8693 OAuth 2.0 Token Exchange
*
* @since 3.26
* @since Authlete 2.3
*/
public void setActorTokenInfo(TokenInfo tokenInfo)
{
this.actorTokenInfo = tokenInfo;
}
/**
* Get the value of the {@code assertion} request parameter.
*
*
* The {@code assertion} request parameter is defined in Section
* 4.1 of RFC 7521 Assertion Framework for OAuth 2.0 Client
* Authentication and Authorization Grants.
*
*
* @return
* The value of the {@code assertion} request parameter.
*
* @see RFC 7521
* Assertion Framework for OAuth 2.0 Client Authentication and
* Authorization Grants
*
* @see RFC 7523
* JSON Web Token (JWT) Profile for OAuth 2.0 Client Authentication
* and Authorization Grants
*
* @since 3.30
* @since Authlete 2.3
*/
public String getAssertion()
{
return assertion;
}
/**
* Set the value of the {@code assertion} request parameter.
*
*
* The {@code assertion} request parameter is defined in Section
* 4.1 of RFC 7521 Assertion Framework for OAuth 2.0 Client
* Authentication and Authorization Grants.
*
*
* @param assertion
* The value of the {@code assertion} request parameter.
*
* @see RFC 7521
* Assertion Framework for OAuth 2.0 Client Authentication and
* Authorization Grants
*
* @see RFC 7523
* JSON Web Token (JWT) Profile for OAuth 2.0 Client Authentication
* and Authorization Grants
*
* @since 3.30
* @since Authlete 2.3
*/
public void setAssertion(String assertion)
{
this.assertion = assertion;
}
/**
* Get the flag indicating whether the previous refresh token that had been
* kept in the database for a short time was used.
*
*
* If the {@code /auth/token} API succeeds and includes a refresh token and
* if this flag is true, the refresh token is the same renewed refresh token
* that was issued on the previous refresh token request.
*
*
*
* If the {@code /auth/token} API reports that the refresh token presented
* by the client application does not exist but if this flag is true, it
* implies that the previous refresh token was used but the short time had
* already passed.
*
*
*
* This flag will never become true if the feature of "Idempotent Refresh
* Token" is not enabled. See the description of
* {@link Service#isRefreshTokenIdempotent()} about the feature.
*
*
* @return
* {@code true} if the previous refresh token that had been kept
* in the database for a short time was used.
*
* @see Service#isRefreshTokenIdempotent()
*
* @since 3.50
* @since Authlete 2.3
*/
public boolean isPreviousRefreshTokenUsed()
{
return previousRefreshTokenUsed;
}
/**
* Set the flag indicating whether the previous refresh token that had been
* kept in the database for a short time was used.
*
*
* If the {@code /auth/token} API succeeds and includes a refresh token and
* if this flag is true, the refresh token is the same renewed refresh token
* that was issued on the previous refresh token request.
*
*
*
* If the {@code /auth/token} API reports that the refresh token presented
* by the client application does not exist but if this flag is true, it
* implies that the previous refresh token was used but the short time had
* already passed.
*
*
*
* This flag will never become true if the feature of "Idempotent Refresh
* Token" is not enabled. See the description of
* {@link Service#isRefreshTokenIdempotent()} about the feature.
*
*
* @param used
* {@code true} to indicate that the previous refresh token that
* had been kept in the database for a short time was used.
*
* @see Service#isRefreshTokenIdempotent()
*
* @since 3.50
* @since Authlete 2.3
*/
public void setPreviousRefreshTokenUsed(boolean used)
{
this.previousRefreshTokenUsed = used;
}
/**
* Get the {@code c_nonce}.
*
*
* {@code c_nonce} is issued in the pre-authorized code flow. In addition,
* a new {@code c_nonce} may be issued in the refresh token flow. See OpenID for Verifiable Credentials Issuance for details.
*
*
*
* The {@code getCNonce()} method added by the version 3.63 has been
* renamed to {@code getCnonce()} by the version 3.90.
*
*
* @return
* The {@code c_nonce}.
*
* @since 3.90
* @since Authlete 3.0
*
* @see OpenID for Verifiable Credentials Issuance
*/
public String getCnonce()
{
return cnonce;
}
/**
* Set the {@code c_nonce}.
*
*
* {@code c_nonce} is issued in the pre-authorized code flow. In addition,
* a new {@code c_nonce} may be issued in the refresh token flow. See OpenID for Verifiable Credentials Issuance for details.
*
*
*
* The {@code setCNonce(String)} method added by the version 3.63 has been
* renamed to {@code setCnonce(String)} by the version 3.90.
*
*
* @param nonce
* The {@code c_nonce}.
*
* @since 3.90
* @since Authlete 3.0
*
* @see OpenID for Verifiable Credentials Issuance
*/
public void setCnonce(String nonce)
{
this.cnonce = nonce;
}
/**
* Get the time at which the {@code c_nonce} expires in milliseconds since
* the Unix epoch (1970-01-01).
*
*
* The {@code getCNonceExpiresAt()} method added by the version 3.63 has
* been renamed to {@code getCnonceExpiresAt()} by the version 3.90.
*
*
* @return
* The time at which the {@code c_nonce} expires.
*
* @since 3.90
* @since Authlete 3.0
*
* @see OpenID for Verifiable Credentials Issuance
*/
public long getCnonceExpiresAt()
{
return cnonceExpiresAt;
}
/**
* Set the time at which the {@code c_nonce} expires in milliseconds since
* the Unix epoch (1970-01-01).
*
*
* The {@code setCNonceExpiresAt(long)} method added by the version 3.63 has
* been renamed to {@code setCnonceExpiresAt(long)} by the version 3.90.
*
*
* @param expiresAt
* The time at which the {@code c_nonce} expires.
*
* @since 3.90
* @since Authlete 3.0
*
* @see OpenID for Verifiable Credentials Issuance
*/
public void setCnonceExpiresAt(long expiresAt)
{
this.cnonceExpiresAt = expiresAt;
}
/**
* Get the duration of the {@code c_nonce} in seconds.
*
*
* The {@code getCNonceDuration()} method added by the version 3.63 has
* been renamed to {@code getCnonceDuration()} by the version 3.90.
*
*
* @return
* The duration of the {@code c_nonce} in seconds.
*
* @since 3.90
* @since Authlete 3.0
*
* @see OpenID for Verifiable Credentials Issuance
*/
public long getCnonceDuration()
{
return cnonceDuration;
}
/**
* Set the duration of the {@code c_nonce} in seconds.
*
*
* The {@code setCNonceDuration(long)} method added by the version 3.63 has
* been renamed to {@code setCnonceDuration(long)} by the version 3.90.
*
*
* @param duration
* The duration of the {@code c_nonce} in seconds.
*
* @since 3.90
* @since Authlete 3.0
*
* @see OpenID for Verifiable Credentials Issuance
*/
public void setCnonceDuration(long duration)
{
this.cnonceDuration = duration;
}
/**
* Get the names of the claims that the authorization request (which resulted
* in generation of the access token) requested to be embedded in ID tokens.
*
*
* Basically the value of this parameter corresponds to the content of the
* {@code id_token} object in the {@code claims} request parameter (OpenID Connect Core 1.0, Section 5.5) of the authorization request.
*
*
*
* Note that, however, the value of this parameter is always null when the
* Authlete server you are using is older than the version 3.0. It's because
* database records for access tokens issued by old Authlete servers do not
* maintain information about "requested claims for ID tokens".
*
*
*
* It is expected that this response parameter is referred to when the
* {@code action} parameter in the response from the {@code /auth/token}
* API is {@link Action#ID_TOKEN_REISSUABLE ID_TOKEN_REISSUABLE}.
*
*
* @return
* The names of the claims that the authorization request requested
* to be embedded in ID tokens.
*
* @since 3.68
* @since Authlete 3.0
*/
public String[] getRequestedIdTokenClaims()
{
return requestedIdTokenClaims;
}
/**
* Set the names of the claims that the authorization request (which resulted
* in generation of the access token) requested to be embedded in ID tokens.
*
*
* Basically the value of this parameter corresponds to the content of the
* {@code id_token} object in the {@code claims} request parameter (OpenID Connect Core 1.0, Section 5.5) of the authorization request.
*
*
*
* Note that, however, the value of this parameter is always null when the
* Authlete server you are using is older than the version 3.0. It's because
* database records for access tokens issued by old Authlete servers do not
* maintain information about "requested claims for ID tokens".
*
*
*
* It is expected that this response parameter is referred to when the
* {@code action} parameter in the response from the {@code /auth/token}
* API is {@link Action#ID_TOKEN_REISSUABLE ID_TOKEN_REISSUABLE}.
*
*
* @param claims
* The names of the claims that the authorization request requested
* to be embedded in ID tokens.
*
* @since 3.68
* @since Authlete 3.0
*/
public void setRequestedIdTokenClaims(String[] claims)
{
this.requestedIdTokenClaims = claims;
}
/**
* Get the expected nonce value for DPoP proof JWT, which should be used
* as the value of the {@code DPoP-Nonce} HTTP header.
*
*
* When this response parameter is not null, the implementation of the
* token endpoint should add the {@code DPoP-Nonce} HTTP header in the
* response from the endpoint to the client application, using the value
* of this response parameter as the value of the HTTP header.
*
*
*
* DPoP-Nonce: (The value of this {@code dpopNonce} response parameter)
*
*
* @return
* The expected nonce value for DPoP proof JWT.
*
* @since 3.82
* @since Authlete 3.0
*
* @see RFC 9449 OAuth 2.0 Demonstrating Proof of Possession (DPoP)
*/
public String getDpopNonce()
{
return dpopNonce;
}
/**
* Set the expected nonce value for DPoP proof JWT, which should be used
* as the value of the {@code DPoP-Nonce} HTTP header.
*
*
* When this response parameter is not null, the implementation of the
* token endpoint should add the {@code DPoP-Nonce} HTTP header in the
* response from the endpoint to the client application, using the value
* of this response parameter as the value of the HTTP header.
*
*
*
* DPoP-Nonce: (The value of this {@code dpopNonce} response parameter)
*
*
* @param dpopNonce
* The expected nonce value for DPoP proof JWT.
*
* @since 3.82
* @since Authlete 3.0
*
* @see RFC 9449 OAuth 2.0 Demonstrating Proof of Possession (DPoP)
*/
public void setDpopNonce(String dpopNonce)
{
this.dpopNonce = dpopNonce;
}
/**
* Get the scopes associated with the refresh token.
*
* @return
* The scopes associated with the refresh token. May be
* {@code null}.
*
* @since 3.89
* @since Authlete API 3.0
*/
public String[] getRefreshTokenScopes()
{
return refreshTokenScopes;
}
/**
* Set the scopes associated with the refresh token.
*
* @param refreshTokenScopes
* The scopes associated with the refresh token.
*
* @since 3.89
* @since Authlete API 3.0
*/
public void setRefreshTokenScopes(String[] refreshTokenScopes)
{
this.refreshTokenScopes = refreshTokenScopes;
}
}