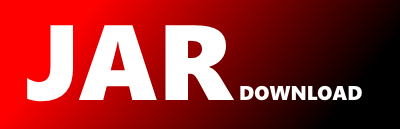
com.authlete.common.dto.UserInfoIssueRequest Maven / Gradle / Ivy
Show all versions of authlete-java-common Show documentation
/*
* Copyright (C) 2015-2022 Authlete, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.authlete.common.dto;
import java.io.Serializable;
import java.util.List;
import java.util.Map;
import com.authlete.common.util.Utils;
/**
* Request to Authlete's {@code /auth/userinfo/issue} API.
*
*
*
* token
(REQUIRED)
* -
*
* The access token that has been passed to the service's userinfo endpoint by the client application. In other words,
* the access token which was contained in the userinfo request.
*
*
*
* claims
(OPTIONAL)
* -
*
* Claims in JSON format. As for the format, see {@link #setClaims(String)}
* and "OpenID Connect Core 1.0, 5.1. Standard Claims".
*
*
*
* sub
(OPTIONAL)
* -
*
* The value of the {@code sub} claim. If the value of this request parameter
* is not empty, it is used as the value of the 'sub' claim. Otherwise, the
* value of the subject associated with the access token is used.
*
*
*
* claimsForTx
(OPTIONAL; Authlete 2.3 onwards)
* -
*
* Claim data that are referenced when Authlete computes values of
* transformed claims. See the description of
* {@link #setClaimsForTx(String)} for details.
*
*
*
* verifiedClaimsForTx
(OPTIONAL; Authlete 2.3 onwards)
* -
*
* Verified claim data that are referenced when Authlete computes values of
* transformed claims. See the description of
* {@link #setVerifiedClaimsForTx(String[])} for details.
*
*
*
* requestSignature
(REQUIRED; Authlete 2.3 onwards)
* -
*
* The {@code Signature} header value from the request to the RS. All
* signatures in this header will be included in the output signature.
*
*
*
* headers
(REQUIRED; Authlete 2.3 onwards)
* -
*
* The HTTP response headers, all will be included in the output signature.
*
*
*
*
*
*
* @author Takahiko Kawasaki
*/
public class UserInfoIssueRequest implements Serializable
{
private static final long serialVersionUID = 7L;
/**
* The access token.
*/
private String token;
/**
* Claims in JSON format.
*/
private String claims;
/**
* The value of the 'sub' claim. If this field is empty, the value of
* the subject that is associated with the access token is used as the
* value of the 'sub' claim.
*/
private String sub;
/**
* Claim key-value pairs that are used to compute transformed claims.
*/
private String claimsForTx;
/**
* Verified claim key-value pairs that are used to compute transformed
* claims.
*/
private String[] verifiedClaimsForTx;
/**
* The {@code Signature} header value from the request.
*
* @since 3.38
* @since Authlete 2.3
*/
private String requestSignature;
/**
* The HTTP headers to include in the signed response.
*
* @since 3.38
* @since Authlete 2.3
*/
private Pair[] headers;
/**
* Get the access token which has come along with the userinfo
* request from the client application.
*/
public String getToken()
{
return token;
}
/**
* Set the access token which has been issued by Authlete.
* The access token is the one that has come along with the
* userinfo request from the client application.
*/
public UserInfoIssueRequest setToken(String token)
{
this.token = token;
return this;
}
/**
* Get the claims of the subject in JSON format.
*
* @return
* The claims of the subject in JSON format. See the description
* of {@link #setClaims(String)} for details about the format.
*
* @see #setClaims(String)
*/
public String getClaims()
{
return claims;
}
/**
* Set the claims of the subject in JSON format.
*
*
* The service implementation is required to retrieve claims of the subject
* (= information about the end-user) from its database and format them in
* JSON format.
*
*
*
* For example, if "given_name"
claim, "family_name"
* claim and "email"
claim are requested, the service implementation
* should generate a JSON object like the following:
*
*
*
* {
* "given_name": "Takahiko",
* "family_name": "Kawasaki",
* "email": "[email protected]"
* }
*
*
*
* and set its String representation by this method.
*
*
*
* See OpenID Connect Core 1.0, 5.1. Standard Claims for further details
* about the format.
*
*
* @param claims
* The claims of the subject in JSON format.
*
* @return
* {@code this} object.
*
* @see OpenID Connect Core 1.0, 5.1. Standard Claims
*/
public UserInfoIssueRequest setClaims(String claims)
{
this.claims = claims;
return this;
}
/**
* Set the value of {@code "claims"} which is the claims of the subject.
* The argument is converted into a JSON string and passed to {@link
* #setClaims(String)} method.
*
* @param claims
* The claims of the subject. Keys are claim names.
*
* @return
* {@code this} object.
*
* @since 1.24
*/
public UserInfoIssueRequest setClaims(Map claims)
{
if (claims == null || claims.size() == 0)
{
this.claims = null;
return this;
}
String json = Utils.toJson(claims);
return setClaims(json);
}
/**
* Get the value of the {@code sub} claim. If this method returns a non-empty value,
* it is used as the value of the 'sub' claim. Otherwise, the value of the subject
* associated with the access token is used.
*
* @return
* The value of the {@code sub} claim.
*
* @since 1.35
*/
public String getSub()
{
return sub;
}
/**
* Set the value of the {@code sub} claim. If a non-empty value is given, it is
* used as the value of the 'sub' claim. Otherwise, the value of the subject
* associated with the access token is used.
*
* @param sub
* The value of the {@code sub} claim.
*
* @return
* {@code this} object.
*
* @since 1.35
*/
public UserInfoIssueRequest setSub(String sub)
{
this.sub = sub;
return this;
}
/**
* Get values of claims requested indirectly by "transformed claims".
*
*
* See the description of {@link #setClaimsForTx(String)} for details.
*
*
* @return
* Values of claims requested indirectly by "transformed
* claims". The format is JSON.
*
* @see OpenID Connect Advanced Syntax for Claims (ASC) 1.0
*
* @see #setClaimsForTx(String)
*
* @since 3.8
*/
public String getClaimsForTx()
{
return claimsForTx;
}
/**
* Set values of claims requested indirectly by "transformed claims".
*
*
* A client application may request "transformed claims". Each of
* transformed claims uses an existing claim as input. As a result, to
* compute the value of a transformed claim, the value of the referenced
* existing claim is needed. This {@code claimsForTx} request parameter
* has to be used to provide values of existing claims for computation of
* transformed claims.
*
*
*
* A response from the {@code /api/auth/userinfo} API may include the
* {@code requestedClaimsForTx} response parameter which is a list of
* claims that are referenced indirectly by transformed claims (cf.
* {@link UserInfoResponse#getRequestedClaimsForTx()}). The authorization
* server implementation should prepare values of the claims listed in
* {@code requestedClaimsForTx} and pass them as the value of this {@code
* claimsForTx} request parameter.
*
*
*
* The following is an example of the value of this request parameter.
*
*
*
* {
* "birthdate": "1970-01-23",
* "nationalities": [ "DEU", "USA" ]
* }
*
*
*
* This request parameter ({@code claimsForTx}) is recognized by Authlete
* 2.3 onwards.
*
*
* @param claims
* Values of claims requested indirectly by "transformed claims".
* The format is JSON.
*
* @return
* {@code this} object.
*
* @see OpenID Connect Advanced Syntax for Claims (ASC) 1.0
*
* @see UserInfoResponse#getRequestedClaimsForTx()
*
* @since 3.8
*/
public UserInfoIssueRequest setClaimsForTx(String claims)
{
this.claimsForTx = claims;
return this;
}
/**
* Set the value of {@code "claimsForTx"} which is the claims of the
* subject. The argument is converted into a JSON string and passed
* to {@link #setClaimsForTx(String)} method.
*
* @param claims
* The claims of the subject. Keys are claim names.
*
* @return
* {@code this} object.
*
* @since 3.9
*/
public UserInfoIssueRequest setClaimsForTx(Map claims)
{
if (claims == null || claims.size() == 0)
{
return setClaimsForTx((String)null);
}
String json = Utils.toJson(claims);
return setClaimsForTx(json);
}
/**
* Get values of verified claims requested indirectly by
* "transformed claims".
*
*
* See the description of {@link #setVerifiedClaimsForTx(String[])}
* for details.
*
*
* @return
* Values of verified claims requested indirectly by "transformed
* claims". The format of elements in the array is JSON.
*
* @see OpenID Connect Advanced Syntax for Claims (ASC) 1.0
*
* @see OpenID Connect for Identity Assurance 1.0
*
* @see #setVerifiedClaimsForTx(String[])
*
* @since 3.8
*/
public String[] getVerifiedClaimsForTx()
{
return verifiedClaimsForTx;
}
/**
* Set values of verified claims requested indirectly by
* "transformed claims".
*
*
* A client application may request "transformed claims". Each of
* transformed claims uses an existing claim as input. As a result, to
* compute the value of a transformed claim, the value of the referenced
* existing claim is needed. This {@code verifiedClaimsForTx} request
* parameter has to be used to provide values of existing claims for
* computation of transformed claims.
*
*
*
* A response from the {@code /api/auth/userinfo} API may include the
* {@code requestedVerifiedClaimsForTx} response parameter which is a list
* of verified claims that are referenced indirectly by transformed claims
* (cf. {@link UserInfoResponse#getRequestedVerifiedClaimsForTx()}).
* The authorization server implementation should prepare values of the
* verified claims listed in {@code requestedVerifiedClaimsForTx} and pass
* them as the value of this {@code verifiedClaimsForTx} request parameter.
*
*
*
* The following is an example of the value of this request parameter.
*
*
*
* [
* "{\"birthdate\":\"1970-01-23\",\"nationalities\":[\"DEU\",\"USA\"]}"
* ]
*
*
*
* The reason that this {@code verifiedClaimsForTx} property is an array
* is that the {@code "verified_claims"} property in the {@code claims}
* request parameter of an authorization request can be an array like below.
*
*
*
* {
* "transformed_claims": {
* "nationality_usa": {
* "claim": "nationalities",
* "fn": [
* [ "eq", "USA" ],
* "any"
* ]
* }
* },
* "userinfo": {
* "verified_claims": [
* {
* "verification": { "trust_framework": { "value": "gold" } },
* "claims": { "::18_or_above": null }
* },
* {
* "verification": { "trust_framework": { "value": "silver" } },
* "claims": { ":nationality_usa": null }
* }
* ]
* }
* }
*
*
*
* For the example above, the value of this {@code verifiedClaimsForTx}
* property should be an array of size 2 and look like below. The first
* element is JSON including claims which have been verified under the
* trust framework "gold", and the second element is JSON including
* claims which have been verified under the trust framework "silver".
*
*
*
* [
* "{\"birthdate\":\"1970-01-23\"}",
* "{\"nationalities\":[\"DEU\",\"USA\"]}"
* ]
*
*
*
* This request parameter ({@code verifiedClaimsForTx}) is recognized by
* Authlete 2.3 onwards.
*
*
* @param claims
* Values of verified claims requested indirectly by
* "transformed claims". The format of elements in the
* array is JSON.
*
* @return
* {@code this} object.
*
* @see OpenID Connect Advanced Syntax for Claims (ASC) 1.0
*
* @see OpenID Connect for Identity Assurance 1.0
*
* @see UserInfoResponse#getRequestedVerifiedClaimsForTx()
*
* @since 3.8
*/
public UserInfoIssueRequest setVerifiedClaimsForTx(String[] claims)
{
this.verifiedClaimsForTx = claims;
return this;
}
/**
* Set the value of {@code "verifiedClaimsForTx"} which is the verified
* claims of the subject. Each element in the given list is converted to
* a JSON string and a newly created string array containing the converted
* elements is passed to {@link #setVerifiedClaimsForTx(String[])}.
*
* @param list
* List of clusters of verified claims.
*
* @return
* {@code this} object.
*
* @since 3.9
*/
public UserInfoIssueRequest setVerifiedClaimsForTx(List