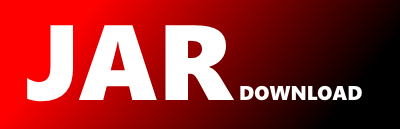
com.authlete.common.dto.AuthorizationFailRequest Maven / Gradle / Ivy
Show all versions of authlete-java-common Show documentation
/*
* Copyright (C) 2014-2022 Authlete, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.authlete.common.dto;
import java.io.Serializable;
/**
* Request to Authlete's {@code /auth/authorization/fail} API.
*
*
*
* ticket
(REQUIRED)
* -
*
* The ticket issued by Authlete's {@code /auth/authorization} API
* to the service implementation. It is the value of {@code "ticket"}
* contained in the response from Authlete's {@code
* /auth/authorization} API ({@link AuthorizationResponse}).
*
*
*
* reason
(REQUIRED)
* -
*
* The reason of the failure of the authorization request.
* See the description of {@link AuthorizationResponse} as to which
* reason should be chosen.
*
*
*
*
*
* @see AuthorizationResponse
*
* @author Takahiko Kawasaki
*/
public class AuthorizationFailRequest implements Serializable
{
private static final long serialVersionUID = 3L;
/**
* Failure reasons of authorization requests.
*/
public enum Reason
{
/**
* Unknown reason.
*
*
* Using this reason will result in {@code error=server_error}.
*
*/
UNKNOWN,
/**
* The authorization request from the client application contained
* {@code prompt=none}, but any end-user has not logged in.
*
*
* See "OpenID Connect Core 1.0, 3.1.2.1. Authentication Request"
* for {@code prompt} request parameter.
*
*
*
* Using this reason will result in {@code error=login_required}.
*
*
* @see OpenID Connect Core 1.0, 3.1.2.1. Authentication Request
*/
NOT_LOGGED_IN,
/**
* The authorization request from the client application contained
* {@code max_age} parameter with a non-zero value or the client's
* configuration has a non-zero value for {@code default_max_age}
* configuration parameter, but the service implementation cannot
* behave properly based on the max age value mainly because the
* service implementation does not manage authentication time of
* end-users.
*
*
* See "OpenID Connect Core 1.0, 3.1.2.1. Authentication Request"
* for {@code max_age} request parameter.
*
*
*
* See "OpenID Connect Dynamic Client Registration 1.0, 2. Client Metadata"
* for {@code default_max_age} configuration parameter.
*
*
*
* Using this reason will result in {@code error=login_required}.
*
*
* @see OpenID Connect Core 1.0, 3.1.2.1. Authentication Request
*
* @see OpenID Connect Dynamic Client Registration 1.0, 2. Client Metadata
*/
MAX_AGE_NOT_SUPPORTED,
/**
* The authorization request from the client application contained
* {@code prompt=none}, but the time specified by {@code max_age}
* request parameter or by {@code default_max_age} configuration
* parameter has passed since the time at which the end-user logged
* in.
*
*
* See "OpenID Connect Core 1.0, 3.1.2.1. Authentication Request"
* for {@code prompt} and {@code max_age} request parameters.
*
*
*
* See "OpenID Connect Dynamic Client Registration 1.0, 2. Client Metadata"
* for {@code default_max_age} configuration parameter.
*
*
*
* Using this reason will result in {@code error=login_required}.
*
*
* @see OpenID Connect Core 1.0, 3.1.2.1. Authentication Request
*
* @see OpenID Connect Dynamic Client Registration 1.0, 2. Client Metadata
*/
EXCEEDS_MAX_AGE,
/**
* The authorization request from the client application requested
* a specific value for {@code sub} claim, but the current end-user
* (in the case of {@code prompt=none}) or the end-user after the
* authentication is different from the specified value.
*
*
* Using this reason will result in {@code error=login_required}.
*
*/
DIFFERENT_SUBJECT,
/**
* The authorization request from the client application contained
* {@code "acr"} claim in {@code "claims"} request parameter and
* the claim was marked as essential, but the ACR performed for the
* end-user does not match any one of the requested ACRs.
*
*
* Using this reason will result in {@code error=login_required}
* when the version of your Authlete server is 2.2 or older.
* Otherwise, this reason will result in
* {@code error=unmet_authentication_requirements}. See "OpenID Connect Core Error Code unmet_authentication_requirements"
* for details about {@code unmet_authentication_requirements}.
*
*
* @see OpenID Connect Core Error Code unmet_authentication_requirements
*/
ACR_NOT_SATISFIED,
/**
* The end-user denied the authorization request from the client
* application.
*
*
* Using this reason will result in {@code error=access_denied}.
*
*/
DENIED,
/**
* Server error.
*
*
* Using this reason will result in {@code error=server_error}.
*
*
* @since 1.3
*/
SERVER_ERROR,
/**
* The end-user was not authenticated.
*
*
* Using this reason will result in {@code error=login_required}.
*
*
* @since 1.3
*/
NOT_AUTHENTICATED,
/**
* The authorization server cannot obtain an account selection choice
* made by the end-user.
*
*
* Using this reason will result in {@code error=account_selection_required}.
*
*
* @since 2.11
*/
ACCOUNT_SELECTION_REQUIRED,
/**
* The authorization server cannot obtain consent from the end-user.
*
*
* Using this reason will result in {@code error=consent_required}.
*
*
* @since 2.11
*/
CONSENT_REQUIRED,
/**
* The authorization server needs interaction with the end-user.
*
*
* Using this reason will result in {@code error=interaction_required}.
*
*
* @since 2.11
*/
INTERACTION_REQUIRED,
/**
* The requested resource is invalid, missing, unknown, or malformed.
* See "Resource Indicators for OAuth 2.0" for details.
*
*
* Using this reason will result in {@code error=invalid_target}.
*
*
* @since 2.62
*/
INVALID_TARGET,
}
/**
* The ticket issued by Authlete's /auth/authorization API.
*/
private String ticket;
/**
* The reason of the failure.
*/
private Reason reason;
/**
* The custom description about the failure.
*/
private String description;
/**
* Get the value of {@code "ticket"} which is the ticket
* issued by Authlete's {@code /auth/authorization} API
* to the service implementation.
*
* @return
* The ticket.
*/
public String getTicket()
{
return ticket;
}
/**
* Set the value of {@code "ticket"} which is the ticket
* issued by Authlete's {@code /auth/authorization} API
* to the service implementation.
*
* @param ticket
* The ticket.
*
* @return
* {@code this} object.
*/
public AuthorizationFailRequest setTicket(String ticket)
{
this.ticket = ticket;
return this;
}
/**
* Get the value of {@code "reason"} which is the reason
* of the failure of the authorization request.
*
* @return
* The reason of the failure.
*/
public Reason getReason()
{
return reason;
}
/**
* Set the value of {@code "reason"} which is the reason
* of the failure of the authorization request.
*
* @param reason
* The reason of the failure.
*
* @return
* {@code this} object.
*/
public AuthorizationFailRequest setReason(Reason reason)
{
this.reason = reason;
return this;
}
/**
* Get the custom description about the authorization failure.
*
* @return
* The custom description.
*
* @since 1.3
*/
public String getDescription()
{
return description;
}
/**
* Set the custom description about the authorization failure.
*
* @param description
* The custom description.
*
* @return
* {@code this} object.
*
* @since 1.3
*/
public AuthorizationFailRequest setDescription(String description)
{
this.description = description;
return this;
}
}