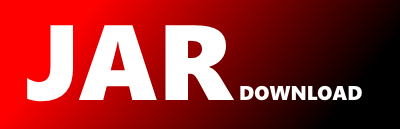
com.authlete.common.dto.AuthorizationResponse Maven / Gradle / Ivy
Show all versions of authlete-java-common Show documentation
/*
* Copyright (C) 2014-2023 Authlete, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.authlete.common.dto;
import java.net.URI;
import com.authlete.common.types.Display;
import com.authlete.common.types.GMAction;
import com.authlete.common.types.Prompt;
import com.authlete.common.util.Utils;
/**
* Response from Authlete's {@code /auth/authorization} API.
*
*
* Note: In the description below, "authorization server"
* is always used even where "OpenID provider" should be
* used.
*
*
*
* Authlete's {@code /auth/authorization} API returns
* JSON which can be mapped to this class. The authorization server
* implementation should retrieve the value of {@code "action"} from
* the response and take the following steps according to the value.
*
*
*
* - {@link Action#INTERNAL_SERVER_ERROR INTERNAL_SERVER_ERROR}
* -
*
* When the value of {@code "action"} is {@code "INTERNAL_SERVER_ERROR"},
* it means that the request from the authorization server implementation
* was wrong or that an error occurred in Authlete.
*
*
*
* In either case, from the viewpoint of the client application, it
* is an error on the server side. Therefore, the authorization server
* implementation should generate a response to the client application
* with the HTTP status of {@code "500 Internal Server Error"}. Authlete
* recommends {@code "application/json"} as the content type although
* OAuth 2.0 specification does not mention the format of the error
* response when the redirect URI is not usable.
*
*
*
* {@link #getResponseContent()} returns a JSON string which describes
* the error, so it can be used as the entity body of the response.
*
*
*
* The following illustrates the response which the authorization server
* implementation should generate and return to the client application.
*
*
*
* HTTP/1.1 500 Internal Server Error
* Content-Type: application/json
* Cache-Control: no-store
* Pragma: no-cache
*
* (The value returned from {@link #getResponseContent()})
*
*
* - {@link Action#BAD_REQUEST BAD_REQUEST}
* -
*
* When the value of {@code "action"} is {@code "BAD_REQUEST"}, it means
* that the request from the client application is invalid.
*
*
*
* The HTTP status of the response returned to the client application should
* be {@code "400 Bad Request"} and Authlete recommends {@code
* "application/json"} as the content type although OAuth 2.0 specification
* does not mention the format of the error response when the redirect URI
* is not usable.
*
*
*
* {@link #getResponseContent()} returns a JSON string which describes the error,
* so it can be used as the entity body of the response.
*
*
*
* The following illustrates the response which the authorization server
* implementation should generate and return to the client application.
*
*
*
* HTTP/1.1 400 Bad Request
* Content-Type: application/json
* Cache-Control: no-store
* Pragma: no-cache
*
* (The value returned from {@link #getResponseContent()})
*
*
*
* - {@link Action#LOCATION LOCATION}
* -
*
* When the value of {@code "action"} is {@code "LOCATION"}, it means
* that the request from the client application is invalid but the
* redirect URI to which the error should be reported has been determined.
*
*
*
* The HTTP status of the response returned to the client application should
* be {@code "302 Found"} and {@code "Location"} header must have a redirect
* URI with the {@code "error"} parameter.
*
*
*
* {@link #getResponseContent()} returns the redirect URI which has the {@code
* "error"} parameter, so it can be used as the value of {@code "Location"}
* header.
*
*
*
* The following illustrates the response which the authorization server
* implementation should generate and return to the client application.
*
*
*
* HTTP/1.1 302 Found
* Location: (The value returned from {@link #getResponseContent()})
* Cache-Control: no-store
* Pragma: no-cache
*
*
*
* - {@link Action#FORM FORM}
* -
*
* When the value of {@code "action"} is {@code "FORM"}, it means
* that the request from the client application is invalid but the
* redirect URI to which the error should be reported has been determined,
* and that the request contains {@code response_mode=form_post} as is
* defined in "OAuth 2.0 Form Post Response Mode".
*
*
*
* The HTTP status of the response returned to the client application should
* be {@code "200 OK"} and the content type should be {@code
* "text/html;charset=UTF-8"}.
*
*
*
* {@link #getResponseContent()} returns an HTML which satisfies the requirements
* of {@code response_mode=form_post}, so it can be used as the entity body
* of the response.
*
*
*
* The following illustrates the response which the authorization server
* implementation should generate and return to the client application.
*
*
*
* HTTP/1.1 200 OK
* Content-Type: text/html;charset=UTF-8
* Cache-Control: no-store
* Pragma: no-cache
*
* (The value returned from {@link #getResponseContent()})
*
*
*
* - {@link Action#NO_INTERACTION NO_INTERACTION}
* -
*
* When the value of {@code "action"} is {@code "NO_INTERACTION"}, it means
* that the request from the client application has no problem and requires
* the authorization server to process the request without displaying any
* user interface for authentication and/or consent. This happens when the
* request contains {@code prompt=none}.
*
*
*
* The authorization server implementation must follow the following steps.
*
*
*
*
* -
*
[END-USER AUTHENTICATION]
* Check whether an end-user has already logged in. If an end-user has
* logged in, go to the next step ([MAX_AGE]). Otherwise, call Authlete's
* {@code /auth/authorization/fail} API with {@code reason=}{@link
* AuthorizationFailRequest.Reason#NOT_LOGGED_IN NOT_LOGGED_IN} and use
* the response from the API to generate a response to the client
* application.
*
*
*
* -
*
[MAX_AGE]
* Get the value of the max age by {@link #getMaxAge()} method. The value
* represents the maximum authentication age which has come from {@code
* "max_age"} request parameter or {@code "default_max_age"} configuration
* parameter of the client application. If the value is 0, go to the next
* step ([SUBJECT]). Otherwise, follow the sub steps described below.
*
*
*
* -
*
* Get the time at which the end-user was authenticated. Note that
* this value is not managed by Authlete, meaning that it is expected
* that the authorization server implementation manages the value.
* If the authorization server implementation does not manage
* authentication time of end-users, call Authlete's {@code
* /auth/authorization/fail} API with {@code reason=}{@link
* AuthorizationFailRequest.Reason#MAX_AGE_NOT_SUPPORTED
* MAX_AGE_NOT_SUPPORTED} and use the response from the API to
* generate a response to the client application.
*
*
* -
*
* Add the value of the maximum authentication age (which is represented
* in seconds) to the authentication time.
*
*
* -
*
* Check whether the calculated value is equal to or greater than the
* current time. If this condition is satisfied, go to the next step
* ([SUBJECT]).
* Otherwise, call Authlete's {@code /auth/authorization/fail} API with
* {@code reason=}{@link AuthorizationFailRequest.Reason#EXCEEDS_MAX_AGE
* EXCEEDS_MAX_AGE} and use the response from the API to generate a
* response to the client application.
*
*
*
*
* -
*
[SUBJECT]
* Get the value of the requested subject by {@link #getSubject()} method.
* The value represents an end-user who the client application expects to
* grant authorization. If the value is {@code null}, go to the next step
* ([ACRs]). Otherwise, follow the sub steps described below.
*
*
*
* -
*
* Compare the value of the requested subject to the subject (= unique
* user ID) of the current end-user.
*
*
* -
*
* If they are equal, go to the next step ([ACRs]).
*
*
* -
*
* If they are not equal, call Authlete's {@code /auth/authorization/fail}
* API with {@code reason=}{@link AuthorizationFailRequest.Reason#DIFFERENT_SUBJECT
* DIFFERENT_SUBJECT} and use the response from the API to generate
* a response to the client application.
*
*
*
*
* -
*
[ACRs]
* Get the value of ACRs (Authentication Context Class References) by {@link
* #getAcrs()} method. The value has come from (1) {@code "acr"} claim in
* {@code "claims"} request parameter, (2) {@code "acr_values"} request
* parameter, or (3) {@code "default_acr_values"} configuration parameter
* of the client application.
*
*
*
* It is ensured that all the ACRs returned by {@link #getAcrs()} method
* are supported by the authorization server implementation. In other words,
* it is ensured that all the ACRs are listed in the {@code
* "acr_values_supported"} configuration parameter of the authorization server.
*
*
*
* If the value of ACRs is {@code null}, go to the next step ([SCOPES]).
* Otherwise, follow the sub steps described below.
*
*
*
* -
*
* Get the ACR performed for the authentication of the current end-user.
* Note that this value is managed not by Authlete but by the authorization
* server implementation. (If the authorization server implementation cannot
* handle ACRs, it should not have listed ACRs as {@code "acr_values_supported"}.)
*
*
* -
*
* Compare the ACR value obtained in the above step to each element in
* the ACR array obtained by {@link #getAcrs()} method in the listed order.
* If the ACR value was found in the array, go to the next step ([SCOPES]).
*
*
* -
*
* If the ACR value was not found in the ACR array (= the ACR performed
* for the authentication of the current end-user did not match any one
* of the ACRs requested by the client application), check whether one
* of the requested ACRs must be satisfied or not by calling {@link
* #isAcrEssential()} method. If {@link #isAcrEssential()} returns
* {@code true}, call Authlete's {@code /auth/authorization/fail} API
* with {@code reason=}{@link AuthorizationFailRequest.Reason#ACR_NOT_SATISFIED
* ACR_NOT_SATISFIED} and use the response from the API to generate a
* response to the client application. Otherwise, go to the next step
* ([SCOPES]).
*
*
*
* -
*
[SCOPES]
* Get the scopes by {@link #getScopes()}. If the array contains a scope
* which has not been granted to the client application by the end-user
* in the past, call Authlete's {@code /auth/authorization/fail} API with
* {@code reason=}{@link AuthorizationFailRequest.Reason#CONSENT_REQUIRED
* CONSENT_REQUIRED} and use the response from the API to generate a
* response to the client application. Otherwise, go to the next step
* ([DYNAMIC SCOPES]).
*
*
*
* Note that Authlete provides APIs to manage records of granted scopes
* ({@code /api/client/granted_scopes/*} APIs), but the APIs work only
* in the case the Authlete server you use is a dedicated Authlete server
* (contact [email protected]
* for details). In other words, the APIs of the shared Authlete server
* are disabled intentionally (in order to prevent garbage data from
* being accumulated) and they return {@code 403 Forbidden}.
*
*
* -
*
[DYNAMIC SCOPES]
* Get the dynamic scopes by {@link #getDynamicScopes()}. If the array
* contains a scope which has not been granted to the client application
* by the end-user in the past, call Authlete's
* {@code /auth/authorization/fail} API with
* {@code reason=}{@link AuthorizationFailRequest.Reason#CONSENT_REQUIRED
* CONSENT_REQUIRED} and use the response from the API to generate a
* response to the client application. Otherwise, go to the next step
* ([RESOURCES]).
*
*
*
* Note that Authlete provides APIs to manage records of granted scopes
* ({@code /api/client/granted_scopes/*} APIs) but dynamic scopes are
* not remembered as granted scopes.
*
*
*
* See the description of the {@link DynamicScope} class for details about
* dynamic scopes.
*
*
* -
*
[RESOURCES]
* Get the requested target resources by {@link #getResources()}. The array
* represents the values of the {@code resource} request parameters. If you
* want to reject the request, call Authlete's {@code /auth/authorization/fail}
* API with {@code reason=}{@link AuthorizationFailRequest.Reason#INVALID_TARGET
* INVALID_TARGET} and use the response from the API to generate a response
* to the client application. Otherwise, go to the next step ([ISSUE]).
*
*
*
* See "Resource Indicators for OAuth 2.0" for details. Note that the
* specification is supported since Authlete 2.2. If the Authlete server you
* are using is older than 2.2, {@code getResources()} always returns null.
*
*
* -
*
[ISSUE]
* If all the above steps succeeded, the last step is to issue an authorization
* code, an ID token and/or an access token. (There is a special case. When
* {@code response_type=none}, nothing is issued.) The last step can be
* performed by calling Authlete's {@code /auth/authorization/issue} API.
* The API requires the following parameters, which are represented as
* properties of {@link AuthorizationIssueRequest} class. Prepare these
* parameters and call the {@code /auth/authorization/issue} API.
*
*
*
* -
*
[ticket] (required)
* This parameter represents a ticket which is exchanged with tokens
* at the {@code /auth/authorization/issue} endpoint.
* Use the value returned by {@link #getTicket()} as it is.
*
*
* -
*
[subject] (required)
* This parameter represents the unique identifier of the current end-user.
* It is often called "user ID" and it may or may not be visible to the user.
* In any case, it is a number or a string assigned to an end-user by your
* service. Authlete does not care about the format of the value of {@code
* subject}, but it must consist of only ASCII letters and its length must
* be equal to or less than 100.
*
*
*
* When {@link #getSubject()} method returns a non-null value, the
* value of {@code subject} parameter is necessarily identical to the
* value returned from the method.
*
*
*
* The value of this parameter will be embedded in an ID token as the
* value of {@code "sub"} claim. When the value of {@code "subject_type"}
* configuration parameter of the client is {@link
* com.authlete.common.types.SubjectType#PAIRWISE PAIRWISE}, the value
* of {@code "sub"} claim is different from the value specified here.
* Note that the behavior for {@code PAIRWISE} is not supported by
* Authlete 2.1 and older versions. See 8. Subject Identifier Types of OpenID Connect Core 1.0 for
* details about subject types.
*
*
*
* You can use the sub
request parameter to adjust the value
* of the sub
claim in an ID token. See the description of the
* sub
request parameter for details.
*
*
* -
*
[authTime] (optional)
* This parameter represents the time when the end-user authentication
* occurred. Its value is the number of seconds from 1970-01-01. The
* value of this parameter will be embedded in an ID token as the value
* of {@code "auth_time"} claim.
*
*
*
* -
*
[acr] (optional)
* This parameter represents the ACR (Authentication Context Class
* Reference) which the authentication of the end-user satisfies.
* When {@link #getAcrs()} method returns a non-empty array and
* {@link #isAcrEssential()} method returns
* {@code true}, the value of this parameter must be one of the array
* elements. Otherwise, even {@code null} is allowed. The value of
* this parameter will be embedded in an ID token as the value of
* {@code "acr"} claim.
*
*
*
* -
*
[claims] (optional)
* This parameter represents claims of the end-user. "Claims" here
* are pieces of information about the end-user such as {@code "name"},
* {@code "email"} and {@code "birthdate"}. The authorization server
* implementation is required to gather claims of the end-user, format
* the claim values into a JSON and set the JSON string as the value
* of this parameter.
*
*
*
* The claims which the authorization server implementation is required
* to gather can be obtained by {@link #getClaims()} method.
*
*
*
* For example, if {@link #getClaims()} method returns an array which
* contains {@code "name"}, {@code "email"} and {@code "birthdate"},
* the value of this parameter should look like the following.
*
*
* {
* "name": "John Smith",
* "email": "[email protected]",
* "birthdate": "1974-05-06"
* }
*
*
* {@link #getClaimsLocales()} lists the end-user's preferred languages
* and scripts for claim values, ordered by preference. When {@link
* #getClaimsLocales()} returns a non-empty array, its elements should
* be taken into account when the authorization server implementation
* gathers claim values. Especially, note the excerpt below from
* 5.2. Claims Languages and Scripts of OpenID Connect Core 1.0.
*
*
*
* "When the OP determines, either through the {@code claims_locales}
* parameter, or by other means, that the End-User and Client are
* requesting Claims in only one set of languages and scripts, it
* is RECOMMENDED that OPs return Claims without language tags
* when they employ this language and script. It is also RECOMMENDED
* that Clients be written in a manner that they can handle and
* utilize Claims using language tags."
*
*
*
* If {@link #getClaims()} method returns {@code null} or an empty array,
* the value of this parameter should be {@code null}.
*
*
*
* See 5.1. Standard Claims of OpenID Connect Core 1.0 for claim names
* and their value formats. Note (1) that the authorization server
* implementation may support its special claims
* (5.1.2. Additional Claims) and (2) that claim names may be followed
* by a language tag
* (5.2. Claims Languages and Scripts). Read the specification of
* OpenID Connect Core 1.0 for details.
*
*
*
* The claim values in this parameter will be embedded in an ID token.
*
*
*
* {@link #getIdTokenClaims()} is available since version 2.25. The method
* returns the value of the {@code "id_token"} property in the {@code "claims"}
* request parameter or in the {@code "claims"} property in a request object.
* The value returned from the method should be considered when you prepare
* claim values. See the description of the method for details. Note that,
* however, old Authlete servers don't support this response parameter.
*
*
*
* -
*
[properties] (optional)
* Extra properties to associate with an access token and/or an authorization
* code that may be issued by this request. Note that properties
* parameter is accepted only when Content-Type of the request to Authlete's
* {@code /auth/authorization/issue} is application/json
, so
* don't use application/x-www-form-urlencoded
if you want to
* use this request parameter. See Extra Properties for details.
*
*
*
* -
*
[scopes] (optional)
* Scopes to associate with an access token and/or an authorization code.
* If this parameter is null, the scopes specified in the original authorization
* request from the client application are used. In other cases, including the
* case of an empty array, the specified scopes will replace the scopes
* contained in the original authorization request.
*
*
*
* Even scopes that are not included in the original authorization request
* can be specified. However, as an exception, {@code "openid"} scope is
* ignored on Authlete server side if it is not included in the original
* request. It is because the existence of {@code "openid"} scope considerably
* changes the validation steps and because adding {@code "openid"} triggers
* generation of an ID token (although the client application has not requested
* it) and the behavior is a major violation against the specification.
*
*
*
* If you add "offline_access"
scope although it is not included
* in the original request, keep in mind that the specification requires explicit
* consent from the user for the scope (OpenID
* Connect Core 1.0, 11. Offline Access). When "offline_access"
* is included in the original request, the current implementation of Authlete's
* {@code /auth/authorization} API checks whether the request has come along with
* prompt
request parameter and the value includes
* "consent"
. However, note that the implementation of Authlete's
* {@code /auth/authorization/issue} API does not perform such checking if
* "offline_access"
scope is added via this scopes parameter.
*
*
*
* -
*
[sub] (optional)
* The value of the {@code sub} claim in an ID token which may be issued.
* If the value of this request parameter is not empty, it is used as the
* value of the {@code sub} claim. Otherwise, the value of the {@code subject}
* request parameter is used as the value of the {@code sub} claim. The main
* purpose of this parameter is to hide the actual value of the subject from
* client applications.
*
*
*
*
*
* {@code /auth/authorization/issue} API returns a response in JSON format
* which can be mapped to {@link AuthorizationIssueResponse}. Use the response
* from the API to generate a response to the client application. See the
* description of {@link AuthorizationIssueResponse} for details.
*
*
*
*
*
*
* - {@link Action#INTERACTION INTERACTION}
* -
*
* When the value of {@code "action"} is {@code "INTERACTION"}, it means
* that the request from the client application has no problem and requires
* the authorization server to process the request with user interaction by
* an HTML form.
*
*
* The purpose of the UI displayed to the end-user is to ask the end-user
* to grant authorization to a client application. The items described
* below are some points which the authorization server implementation
* should take into account when it builds the UI.
*
*
*
*
* -
*
[DISPLAY MODE]
* {@code AuthorizationResponse} contains {@code "display"} parameter.
* The value can be obtained by {@link #getDisplay()} method and is
* one of {@link Display#PAGE PAGE} (default), {@link Display#POPUP
* POPUP}, {@link Display#TOUCH TOUCH} and {@link Display#WAP WAP}.
* The meanings of the values are described in 3.1.2.1. Authentication Request of OpenID Connect Core 1.0.
* Basically, the authorization server implementation should display
* the UI which is suitable for the display mode, but it is okay for
* the authorization server implementation to "attempt to detect
* the capabilities of the User Agent and present an appropriate
* display."
*
*
*
* It is ensured that the value returned by {@link #getDisplay()} is
* one of the supported display values which are specified by {@code
* "display_values_supported"} configuration parameter of the service.
*
*
*
* -
*
[UI LOCALE]
* {@code AuthorizationResponse} contains {@code "ui_locales"} parameter.
* The value can be obtained by {@link #getUiLocales()} and it is an
* array of language tag values (such as {@code "fr-CA"} and {@code
* "en"}) ordered by preference. The authorization server implementation
* should display the UI in one of the language listed in the {@code
* "ui_locales"} parameter when possible.
*
*
*
* It is ensured that language tags returned by {@link #getUiLocales()}
* are contained in the list of supported UI locales which are specified
* by {@code "ui_locales_supported"} configuration parameter of the
* service.
*
*
*
* -
*
[CLIENT INFORMATION]
* The authorization server implementation should show the end-user
* information about the client application. The information can be
* obtained by {@link #getClient()} method.
*
*
*
* -
*
[SCOPES]
* A client application requires authorization for specific permissions.
* In OAuth 2.0 specification, "scope" is a technical term which represents
* a permission. {@link #getScopes()} method returns scopes requested by
* the client application. The authorization server implementation should
* show the end-user the scopes.
*
*
*
* The authorization server implementation may choose not to show scopes
* to which the end-user has given consent in the past. To put it the
* other way around, the authorization server implementation may show
* only the scopes to which the end-user has not given consent yet.
* However, if the value returned from {@link #getPrompts()} contains
* {@link Prompt#CONSENT CONSENT}, the authorization server implementation
* has to obtain explicit consent from the end-user even if the end-user
* has given consent to all the requested scopes in the past.
*
*
*
* Note that Authlete provides APIs to manage records of granted scopes
* ({@code /api/client/granted_scopes/*} APIs), but the APIs work only
* in the case the Authlete server you use is a dedicated Authlete server
* (contact [email protected]
* for details). In other words, the APIs of the shared Authlete server
* are disabled intentionally (in order to prevent garbage data from
* being accumulated) and they return {@code 403 Forbidden}.
*
*
*
* It is ensured that scopes returned by {@link #getScopes()} are
* contained in the list of supported scopes which are specified by
* {@code "scopes_supported"} configuration parameter of the service.
*
*
*
* -
*
[DYNAMIC SCOPES]
* The authorization request may include dynamic scopes. The list of
* recognized dynamic scopes are accessible by {@link #getDynamicScopes()}
* method. See the description of the {@link DynamicScope} class for
* details about dynamic scopes.
*
*
*
* -
*
[AUTHORIZATION DETAILS]
* The authorization server implementation should show the end-user
* "authorization details" if the request includes it.
* {@link #getAuthorizationDetails()} returns the content of the
* {@code authorization_details} request parameter.
*
*
*
* See "OAuth 2.0 Rich Authorization Requests" for details. Note that the
* specification is supported since Authlete 2.2. If the Authlete server
* you are using is older than 2.2, {@code getAuthorizationDetails()}
* always returns null.
*
*
*
* -
*
[PURPOSE]
* The authorization server implementation must show the value of the
* {@code purpose} request parameter if it supports OpenID Connect for Identity Assurance 1.0. See 8. Transaction-specific Purpose in the specification for details.
*
*
*
* {@link #getPurpose()} returns the value of the {@code purpose} request
* parameter. However, if the Authlete server you are using does not support
* OpenID Connect for Identity Assurance 1.0 (in other words, if the Authlete
* server is older than 2.2), {@code getPurpose()} always returns null.
*
*
*
* -
*
[ISSUABLE CREDENTIALS]
* An authorization request may specify issuable credentials by using
* one or more of the following mechanisms in combination.
*
*
*
* - The "{@code issuer_state}" request parameter.
*
- The "{@code authorization_details}" request parameter.
*
- The "{@code scope}" request parameter.
*
*
* When the authorization request specifies one or more issuable credentials,
* the {@link #getIssuableCredentials()} method returns the information about
* the issuable credentials. When the information is available, the
* authorization server implementation should show the information to the user.
*
*
*
* Note that if scope values specifying issuable credentials are dropped
* due to user disapproval, the resulting set of issuable credentials will
* differ from the originally requested set in the authorization request.
*
*
*
* -
*
[END-USER AUTHENTICATION]
* Necessarily, the end-user must be authenticated (= must login your
* service) before granting authorization to the client application.
* Simply put, a login form is expected to be displayed for end-user
* authentication. The authorization server implementation must follow
* the steps described below to comply with OpenID Connect. (Or just
* always show a login form if it's too much of a bother to follow
* the steps below.)
*
*
*
* -
*
* Get the value of {@link #getPrompts()}. It corresponds to the
* value of the {@code prompt} request parameter. Details of the
* request parameter are described in
* 3.1.2.1. Authentication Request of OpenID Connect Core 1.0.
*
*
*
* -
*
* If the value returned from {@link #getPrompts()} contains
* {@link Prompt#SELECT_ACCOUNT SELECT_ACCOUNT}, display a form
* to urge the end-user to select one of his/her accounts for login.
* If {@link #getSubject()} returns a non-null value, it is the
* end-user ID that the client application expects, so the value
* should be used to determine the value of the login ID. Note
* that a subject and a login ID are not necessarily equal. If
* {@link #getSubject()} returns null, the value returned by
* {@link #getLoginHint()} should be referred to as a hint to
* determine the value of the login ID. {@link #getLoginHint()}
* method simply returns the value of the {@code login_hint}
* request parameter.
*
*
*
* Also, if {@link #getCredentialOfferInfo()} returns a non-null
* value, it may be appropriate to use the value returned from
* {@link #getCredentialOfferInfo()}{@code .}{@link
* CredentialOfferInfo#getSubject() getSubject()} as a hint. The
* value represents the unique identifier of the user who was
* authenticated when the credential offer was issued by the
* credential issuer. See the "OpenID for Verifiable Credential
* Issuance" specification for details about the credential offer.
*
*
*
* -
*
* If the value returned from {@link #getPrompts()} contains
* {@link Prompt#LOGIN LOGIN}, display a form to urge the end-user
* to login even if the end-user has already logged in. If {@link
* #getSubject()} returns a non-null value, it is the end-user
* ID that the client application expects, so the value should
* be used to determine the value of the login ID. Note that a
* subject and a login ID are not necessarily equal. If {@link
* #getSubject()} returns null, the value returned by {@link
* #getLoginHint()} should be referred to as a hint to determine
* the value of the login ID. {@link #getLoginHint()} method
* simply returns the value of the {@code login_hint} request
* parameter.
*
*
*
* Also, if {@link #getCredentialOfferInfo()} returns a non-null
* value, it may be appropriate to use the value returned from
* {@link #getCredentialOfferInfo()}{@code .}{@link
* CredentialOfferInfo#getSubject() getSubject()} as a hint. The
* value represents the unique identifier of the user who was
* authenticated when the credential offer was issued by the
* credential issuer. See the "OpenID for Verifiable Credential
* Issuance" specification for details about the credential offer.
*
*
*
* -
*
* If the value returned from {@link #getPrompts()} does not
* contain {@link Prompt#LOGIN LOGIN}, the authorization server
* implementation does not have to authenticate the end-user
* if all the conditions described below are satisfied. If
* any one of the conditions is not satisfied, show a login
* form to authenticate the end-user.
*
*
*
* -
*
* An end-user has already logged in your service.
*
*
* -
*
* The login ID of the current end-user matches the value
* returned by {@link #getSubject()}. This check is required
* only when {@link #getSubject()} returns a non-null value.
*
*
* -
*
* The max age, which is the number of seconds obtained by
* {@link #getMaxAge()} method, has not passed since the
* current end-user logged in your service. This check is
* required only when {@link #getMaxAge()} returns a
* non-zero value.
*
*
*
* If the authorization server implementation does not manage
* authentication time of end-users (= if the authorization
* server implementation cannot know when end-users logged in)
* and if {@link #getMaxAge()} returns a non-zero value, a
* login form should be displayed.
*
*
*
* -
*
* The ACR (Authentication Context Class Reference) of the
* authentication performed for the current end-user satisfies
* one of the ACRs listed by {@link #getAcrs()}. This check is
* required only when {@link #getAcrs()} returns a non-empty
* array.
*
*
*
*
* -
*
* If the value returned from {@link #getPrompts()} includes {@link
* Prompt#CREATE CREATE}, it indicates that the client desires that
* the user be shown the account creation UX rather than the login
* flow. See "Initiating User Registration via OpenID Connect 1.0" for
* details about the value "{@code create}".
*
*
*
*
*
* In every case, the end-user authentication must satisfy one of the ACRs
* listed by {@link #getAcrs()} when {@link #getAcrs()} returns a non-empty
* array and {@link #isAcrEssential()} returns {@code true}.
*
*
*
* -
*
[GRANT/DENY BUTTONS]
* The end-user is supposed to choose either (1) to grant authorization
* to the client application or (2) to deny the authorization request.
* The UI must have UI components to accept the decision by the user.
* Usually, a button to grant authorization and a button to deny the
* request are provided.
*
*
*
*
*
*
* When the subject returned by {@link #getSubject()} method is not {@code null},
* the end-user authentication must be performed for the subject, meaning that
* the authorization server implementation should repeatedly show a login form
* until the subject is successfully authenticated.
*
*
*
* The end-user will choose either (1) to grant authorization to the client
* application or (2) to deny the authorization request. When the end-user
* chose to deny the authorization request, call Authlete's {@code
* /auth/authorization/fail} API with {@code reason=}{@link
* AuthorizationFailRequest.Reason#DENIED DENIED} and use the response from
* the API to generate a response to the client application.
*
*
*
* When the end-user chose to grant authorization to the client application,
* the authorization server implementation has to issue an authorization code,
* an ID token, and/or an access token to the client application. (There is a
* special case. When {@code response_type=none}, nothing is issued.) Issuing
* the tokens can be performed by calling Authlete's {@code /auth/authorization/issue}
* API. Read [ISSUE] written above in the description for the case of {@code
* action=NO_INTERACTION}.
*
*
*
* Authlete 2.3 and newer versions support Grant Management for
* OAuth 2.0. An authorization request may contain a {@code grant_id}
* request parameter which is defined in the specification. If the value of
* the request parameter is valid, {@link #getGrantSubject()} will return the
* subject of the user who has given the grant to the client application.
* Authorization server implementations may use the value returned from
* {@link #getGrantSubject()} in order to determine the user to authenticate.
*
*
*
*
*
* Authlete 2.3 and newer version support "Transformed Claims". An
* authorization request may request "transformed claims". A transformed
* claim uses an existing claim as input. For example, an authorization server
* may predefine a transformed claim named {@code 18_or_over} which uses the
* {@code birthdate} claim as input. If a client application requests the
* {@code 18_or_over} transformed claim, the authorization server needs to
* prepare the value of the {@code birthdate} claim and passes it to Authlete's
* {@code /api/auth/authorization/issue} API so that Authlete can compute the
* value of the {@code 18_or_over} transformed claim. See the descriptions
* of {@link #getRequestedClaimsForTx()} and {@link #getRequestedVerifiedClaimsForTx()}
* for details.
*
*
* @see RFC 6749, OAuth 2.0
*
* @see OpenID Connect Core 1.0
*
* @see OpenID Connect Dynamic Client Registration 1.0
*
* @see OpenID Connect Discovery 1.0
*
* @author Takahiko Kawasaki
*/
public class AuthorizationResponse extends ApiResponse
{
private static final long serialVersionUID = 20L;
/**
* The next action that the service implementation should take.
*/
public enum Action
{
/**
* The request from the service implementation was wrong or
* an error occurred in Authlete. The service implementation
* should return {@code "500 Internal Server Error"} to the
* client application.
*/
INTERNAL_SERVER_ERROR,
/**
* The authorization request was wrong and the service implementation
* should notify the client application of the error by
* {@code "400 Bad Request"}.
*/
BAD_REQUEST,
/**
* The authorization request was wrong and the service implementation
* should notify the client application of the error by
* {@code "302 Found"}.
*/
LOCATION,
/**
* The authorization request was wrong and the service implementation
* should notify the client application of the error by
* {@code "200 OK"} with an HTML which triggers redirection by
* JavaScript.
*
* @see OAuth 2.0 Form Post Response Mode
*/
FORM,
/**
* The authorization request was valid and the service implementation
* should issue an authorization code, an ID token and/or an access
* token without interaction with the end-user.
*/
NO_INTERACTION,
/**
* The authorization request was valid and the service implementation
* should display UI to ask for authorization from the end-user.
*/
INTERACTION
}
private static final String SUMMARY_FORMAT
= "ticket=%s, action=%s, serviceNumber=%d, clientNumber=%d, clientId=%d, "
+ "clientSecret=%s, clientType=%s, developer=%s, display=%s, maxAge=%d, "
+ "scopes=%s, uiLocales=%s, claimsLocales=%s, claims=%s, acrEssential=%s, "
+ "clientIdAliasUsed=%s, "
+ "acrs=%s, subject=%s, loginHint=%s, lowestPrompt=%s, prompts=%s";
/*
* Do not change variable names. They must match the variable names
* in JSONs which are exchanged between clients and Authlete server.
*/
/**
* @since Authlete 1.1
*/
private Action action;
/**
* @since Authlete 1.1
*/
private Service service;
/**
* @since Authlete 1.1
*/
private Client client;
/**
* @since Authlete 1.1
*/
private Display display;
/**
* @since Authlete 1.1
*/
private int maxAge;
/**
* @since Authlete 1.1
*/
private Scope[] scopes;
/**
* @since Authlete 2.2.8
*/
private DynamicScope[] dynamicScopes;
/**
* @since Authlete 1.1
*/
private String[] uiLocales;
/**
* @since Authlete 1.1
*/
private String[] claimsLocales;
/**
* @since Authlete 1.1
*/
private String[] claims;
/**
* @since Authlete 3.0.0
*/
private String[] claimsAtUserInfo;
/**
* @since Authlete 1.1
*/
private boolean acrEssential;
/**
* @since Authlete 1.1
*/
private boolean clientIdAliasUsed;
/**
* @since Authlete 2.3
*/
private boolean clientEntityIdUsed;
/**
* @since Authlete 1.1
*/
private String[] acrs;
/**
* @since Authlete 1.1
*/
private String subject;
/**
* @since Authlete 1.1
*/
private String loginHint;
/**
* @since Authlete 1.1
*/
private Prompt lowestPrompt;
/**
* @since Authlete 1.1
*/
private Prompt[] prompts;
/**
* @since Authlete 1.1.22
*/
private String requestObjectPayload;
/**
* @since Authlete 1.1
*/
private String idTokenClaims;
/**
* @since Authlete 1.1
*/
private String userInfoClaims;
/**
* @since Authlete 2.3
*/
private String transformedClaims;
/**
* @since Authlete 2.2.1
*/
private URI[] resources;
/**
* @since Authlete 2.2.0
*/
private AuthzDetails authorizationDetails;
/**
* @since Authlete 2.2.1
*/
private String purpose;
/**
* @since Authlete 2.3.0
*/
private GMAction gmAction;
/**
* @since Authlete 2.3.0
*/
private String grantId;
/**
* @since Authlete 2.3.0
*/
private String grantSubject;
/**
* @since Authlete 2.3.0
*/
private Grant grant;
/**
* @since Authlete 2.3
*/
private String[] requestedClaimsForTx;
/**
* @since Authlete 2.3
*/
private StringArray[] requestedVerifiedClaimsForTx;
/**
* @since Authlete 3.0.0
*/
private CredentialOfferInfo credentialOfferInfo;
/**
* @since Authlete 3.0.0
*/
private String issuableCredentials;
/**
* @since Authlete 1.1
*/
private String responseContent;
/**
* @since Authlete 1.1
*/
private String ticket;
/**
* Get the next action that the service implementation should take.
*/
public Action getAction()
{
return action;
}
/**
* Set the next action that the service implementation should take.
*/
public void setAction(Action action)
{
this.action = action;
}
/**
* Get the information about the service.
*
* @return
* Information about the service.
*
* @since 1.23
*/
public Service getService()
{
return service;
}
/**
* Set the information about the service.
*
* @param service
* Information about the service.
*
* @since 1.23
*/
public void setService(Service service)
{
this.service = service;
}
/**
* Get the information about the client application which has made
* the authorization request.
*
* @see OpenID Connect Dynamic Client Registration 1.0
*/
public Client getClient()
{
return client;
}
/**
* Set the information about the client application which has made
* the authorization request.
*/
public void setClient(Client client)
{
this.client = client;
}
/**
* Get the display mode which the client application requests
* by {@code "display"} request parameter. When the authorization
* request does not contain {@code "display"} request parameter,
* this method returns {@link Display#PAGE} as the default value.
*
* @see OpenID Connect Core 1.0, 3.1.2.1. Authentication Request
*/
public Display getDisplay()
{
return display;
}
/**
* Set the display mode which the client application requires
* by {@code "display"} request parameter.
*/
public void setDisplay(Display display)
{
this.display = display;
}
/**
* Get the maximum authentication age which is the allowable
* elapsed time in seconds since the last time the end-user
* was actively authenticated by the service implementation.
* The value comes from {@code "max_age"} request parameter
* or {@code "default_max_age"} configuration parameter of
* the client application. 0 may be returned which means
* that the max age constraint does not have to be imposed.
*
* @see OpenID Connect Core 1.0, 3.1.2.1. Authentication Request
*
* @see OpenID Connect Dynamic Client Registration 1.0, 2. Client Metadata
*/
public int getMaxAge()
{
return maxAge;
}
/**
* Set the maximum authentication age.
*/
public void setMaxAge(int maxAge)
{
this.maxAge = maxAge;
}
/**
* Get the scopes which the client application requests by {@code
* "scope"} request parameter. When the authorization request does
* not contain {@code "scope"} request parameter, this method
* returns a list of scopes which are marked as default by the
* service implementation. {@code null} may be returned if the
* authorization request does not contain valid scopes and none
* of registered scopes is marked as default.
*
*
* You may want to enable end-users to select/deselect scopes in
* the authorization page. In other words, you may want to use
* a different set of scopes than the set specified by the original
* authorization request. You can replace scopes when you call
* Authlete's /auth/authorization/issue API. See the description
* of {@link AuthorizationIssueRequest#setScopes(String[])} for
* details.
*
*
* @see OAuth 2.0, 3.3. Access Token Scope
*/
public Scope[] getScopes()
{
return scopes;
}
/**
* Set the scopes which the client application requests or the
* default scopes when the authorization request does not contain
* {@code "scope"} request parameter.
*
*
* You may want to enable end-users to select/deselect scopes in
* the authorization page. In other words, you may want to use
* a different set of scopes than the set specified by the original
* authorization request. You can replace scopes when you call
* Authlete's /auth/authorization/issue API. See the description
* of {@link AuthorizationIssueRequest#setScopes(String[])} for
* details.
*
*/
public void setScopes(Scope[] scopes)
{
this.scopes = scopes;
}
/**
* Get the dynamic scopes which the client application requested
* by the {@code scope} request parameter. See the description of
* {@link DynamicScope} for details.
*
* @return
* The list of dynamic scopes.
*
* @since 2.92
*
* @see DynamicScope
*/
public DynamicScope[] getDynamicScopes()
{
return dynamicScopes;
}
/**
* Set the dynamic scopes which the client application requested
* by the {@code scope} request parameter. See the description of
* {@link DynamicScope} for details.
*
* @param dynamicScopes
* The list of dynamic scopes.
*
* @since 2.92
*
* @see DynamicScope
*/
public void setDynamicScopes(DynamicScope[] dynamicScopes)
{
this.dynamicScopes = dynamicScopes;
}
/**
* Get the list of preferred languages and scripts for the user
* interface. The value comes from {@code "ui_locales"} request
* parameter.
*
* @see OpenID Connect Core 1.0, 3.1.2.1. Authentication Request
*/
public String[] getUiLocales()
{
return uiLocales;
}
/**
* Set the list of preferred languages and scripts for the user
* interface.
*/
public void setUiLocales(String[] uiLocales)
{
this.uiLocales = uiLocales;
}
/**
* Get the list of preferred languages and scripts for claim
* values contained in the ID token. The value comes from
* {@code "claims_locales"} request parameter.
*
* @see OpenID Connect Core 1.0, 5.2. Claims Languages and Scripts
*/
public String[] getClaimsLocales()
{
return claimsLocales;
}
/**
* Set the list of preferred languages and scripts for claim
* values contained in the ID token.
*/
public void setClaimsLocales(String[] claimsLocales)
{
this.claimsLocales = claimsLocales;
}
/**
* Get the list of claims that the client application requests
* to be embedded in the ID token. The value comes from the
* {@code "scope"} and {@code "claims"} request parameters of
* the original authorization request.
*
* @return
* Claims that the client application requests to be embedded in
* the ID token.
*
* @see OpenID Connect Core 1.0, 5.4. Requesting Claims using Scope Values
*
* @see OpenID Connect Core 1.0, 5.5. Requesting Claims using
* the "claims" Request Parameter
*
* @see Service#isClaimShortcutRestrictive()
*/
public String[] getClaims()
{
return claims;
}
/**
* Set the list of claims that the client application requests
* to be embedded in the ID token.
*
* @param claims
* Claims that the client application requests to be embedded in
* the ID token.
*
* @see OpenID Connect Core 1.0, 5.4. Requesting Claims using Scope Values
*
* @see OpenID Connect Core 1.0, 5.5. Requesting Claims using
* the "claims" Request Parameter
*
* @see Service#isClaimShortcutRestrictive()
*/
public void setClaims(String[] claims)
{
this.claims = claims;
}
/**
* Get the list of claims that the client application requests to be
* embedded in userinfo responses. The value comes from the {@code "scope"}
* and {@code "claims"} request parameters of the original authorization
* request.
*
* @return
* Claims that the client application requests to be embedded in
* userinfo responses.
*
* @see OpenID Connect Core 1.0, 5.3. UserInfo Endpoint
*
* @see OpenID Connect Core 1.0, 5.4. Requesting Claims using Scope Values
*
* @see OpenID Connect Core 1.0, 5.5. Requesting Claims using
* the "claims" Request Parameter
*
* @since 3.52
*/
public String[] getClaimsAtUserInfo()
{
return claimsAtUserInfo;
}
/**
* Set the list of claims that the client application requests to be
* embedded in userinfo responses.
*
* @param claims
* Claims that the client application requests to be embedded in
* userinfo responses.
*
* @see OpenID Connect Core 1.0, 5.3. UserInfo Endpoint
*
* @see OpenID Connect Core 1.0, 5.4. Requesting Claims using Scope Values
*
* @see OpenID Connect Core 1.0, 5.5. Requesting Claims using
* the "claims" Request Parameter
*
* @since 3.52
*/
public void setClaimsAtUserInfo(String[] claims)
{
this.claimsAtUserInfo = claims;
}
/**
* Get the flag which indicates whether the end-user authentication
* must satisfy one of the requested ACRs.
*
*
* This method returns {@code true} only when the authorization
* request from the client contains {@code "claim"} request parameter
* and it contains an entry for {@code "acr"} claim with
* {@code "essential":true}.
*
*
* @see OpenID Connect Core 1.0, 5.5.1. Individual Claims Requests
*/
public boolean isAcrEssential()
{
return acrEssential;
}
/**
* Set the flag which indicates whether the end-user authentication
* must satisfy one of the requested ACRs.
*/
public void setAcrEssential(boolean essential)
{
this.acrEssential = essential;
}
/**
* Get the flag which indicates whether the value of the {@code client_id}
* request parameter included in the authorization request is the client
* ID alias.
*
* @since 2.3
*/
public boolean isClientIdAliasUsed()
{
return clientIdAliasUsed;
}
/**
* Set the flag which indicates whether the value of the {@code client_id}
* request parameter included in the authorization request is the client
* ID alias.
*
* @since 2.3
*/
public void setClientIdAliasUsed(boolean used)
{
clientIdAliasUsed = used;
}
/**
* Get the flag which indicates whether the value of the {@code client_id}
* request parameter included in the authorization request is the entity
* ID of the client.
*
*
* "Entity ID" is a technical term defined in OpenID
* Federation 1.0.
*
*
*
* When this flag is {@code true}, {@code client.}{@link Client#getEntityId()
* getEntityId()} returns the entity ID of the client.
*
*
* @return
* {@code true} if the value of the {@code client_id} request
* parameter is the entity ID of the client.
*
* @since 3.37
* @since Authlete 2.3
*
* @see OpenID Federation 1.0
*/
public boolean isClientEntityIdUsed()
{
return clientEntityIdUsed;
}
/**
* Set the flag which indicates whether the value of the {@code client_id}
* request parameter included in the authorization request is the entity
* ID of the client.
*
*
* "Entity ID" is a technical term defined in OpenID
* Federation 1.0.
*
*
*
* When this flag is {@code true}, {@code client.}{@link Client#getEntityId()
* getEntityId()} returns the entity ID of the client.
*
*
* @param used
* {@code true} to indicate that the value of the {@code client_id}
* request parameter is the entity ID of the client.
*
* @since 3.37
* @since Authlete 2.3
*
* @see OpenID Federation 1.0
*/
public void setClientEntityIdUsed(boolean used)
{
clientEntityIdUsed = used;
}
/**
* Get the list of ACRs (Authentication Context Class References)
* requested by the client application. The value come from (1)
* {@code "acr"} claim in {@code "claims"} request parameter, (2)
* {@code "acr_values"} request parameter, or (3) {@code
* "default_acr_values"} configuration parameter of the client
* application.
*
* @see OpenID Connect Core 1.0, 5.5. Requesting Claims using
* the "claims" Request Parameter
*
* @see OpenID Connect Core 1.0, 3.1.2.1. Authentication Request
*
* @see OpenID Connect Dynamic Client Registration 1.0, 2. Client Metadata
*/
public String[] getAcrs()
{
return acrs;
}
/**
* Set the list of ACRs (Authentication Context Class References)
* requested by the client application.
*/
public void setAcrs(String[] acrs)
{
this.acrs = acrs;
}
/**
* Get the subject (= end-user's unique ID) that the client
* application requests. The value comes from {@code "sub"}
* claim in {@code "claims"} request parameter. This method
* may return {@code null} (probably in most cases).
*
* @see OpenID Connect Core 1.0, 5.5. Requesting Claims using
* the "claims" Request Parameter
*/
public String getSubject()
{
return subject;
}
/**
* Set the subject (= end-user's login ID) that the client
* application requests.
*/
public void setSubject(String subject)
{
this.subject = subject;
}
/**
* Get the value of login hint, which is specified by the client
* application using {@code "login_hint"} request parameter.
*
* @see OpenID Connect Core 1.0, 3.1.2.1. Authentication Request
*/
public String getLoginHint()
{
return loginHint;
}
/**
* Set the value of login hint, which is specified by the client
* application using {@code "login_hint"} request parameter.
*/
public void setLoginHint(String loginHint)
{
this.loginHint = loginHint;
}
/**
* Get the prompt that the UI displayed to the end-user must satisfy
* at least. The value comes from {@code "prompt"} request parameter.
* When the authorization request does not contain {@code "prompt"}
* parameter, this method returns {@link Prompt#CONSENT CONSENT} as
* the default value.
*
*
* This method is deprecated. Use {@link #getPrompts()} instead.
*
*
* @see OpenID Connect Core 1.0, 3.1.2.1. Authentication Request
*
* @deprecated
*/
@Deprecated
public Prompt getLowestPrompt()
{
return lowestPrompt;
}
/**
* Set the prompt that the UI displayed to the end-user must satisfy
* at least.
*/
public void setLowestPrompt(Prompt prompt)
{
this.lowestPrompt = prompt;
}
/**
* Get the list of prompts contained in the authorization request
* (= the value of {@code prompt} request parameter).
*
* @return
* The list of prompts contained in the authorization request.
*
* @see OpenID Connect Core 1.0, 3.1.2.1. Authentication Request
*
* @see Initiating User Registration via OpenID Connect 1.0
*
* @since 1.34
*/
public Prompt[] getPrompts()
{
return prompts;
}
/**
* Set the list of prompts contained in the authorization request
* (= the value of {@code prompt} request parameter).
*
* @param prompts
* The list of prompts contained in the authorization request.
*
* @since 1.34
*/
public void setPrompts(Prompt[] prompts)
{
this.prompts = prompts;
}
/**
* Get the payload part of the request object.
*
*
* This method returns {@code null} if the authorization request does not
* include a request object.
*
*
* @return
* The payload part of the request object in JSON format.
*
* @since 2.22
*/
public String getRequestObjectPayload()
{
return requestObjectPayload;
}
/**
* Set the payload part of the request object.
*
* @param payload
* The payload part of the request object in JSON format.
*
* @since 2.22
*/
public void setRequestObjectPayload(String payload)
{
this.requestObjectPayload = payload;
}
/**
* Get the value of the {@code "id_token"} property in the {@code "claims"}
* request parameter or in the {@code "claims"} property in a request object.
*
*
* A client application may request certain claims be embedded in an ID
* token or in a response from the UserInfo endpoint. There are several
* ways. Including the {@code claims} request parameter and including the
* {@code claims} property in a request object are such examples. In both
* the cases, the value of the {@code claims} parameter/property is JSON.
* Its format is described in 5.5. Requesting Claims using the "claims" Request Parameter of
* OpenID
* Connect Core 1.0.
*
*
*
* The following is an excerpt from the specification. You can find
* {@code "userinfo"} and {@code "id_token"} are top-level properties.
*
*
*
* {
* "userinfo":
* {
* "given_name": {"essential": true},
* "nickname": null,
* "email": {"essential": true},
* "email_verified": {"essential": true},
* "picture": null,
* "http://example.info/claims/groups": null
* },
* "id_token":
* {
* "auth_time": {"essential": true},
* "acr": {"values": ["urn:mace:incommon:iap:silver"] }
* }
* }
*
*
*
* This method ({@code getIdTokenClaims()}) returns the value of the
* {@code "id_token"} property in JSON format. For example, if the
* JSON above is included in an authorization request, this method
* returns JSON equivalent to the following.
*
*
*
* {
* "auth_time": {"essential": true},
* "acr": {"values": ["urn:mace:incommon:iap:silver"] }
* }
*
*
*
* Note that if a request object is given and it contains the
* {@code claims} property and if the {@code claims} request
* parameter is also given, this method returns the value in
* the former.
*
*
* @return
* The value of the {@code "id_token"} property in the
* {@code "claims"} in JSON format.
*
* @since 2.25
*/
public String getIdTokenClaims()
{
return idTokenClaims;
}
/**
* Set the value of the {@code "id_token"} property in the {@code "claims"}
* request parameter or in the {@code "claims"} property in a request object.
*
* @param idTokenClaims
* The value of the {@code "id_token"} property in the
* {@code "claims"} in JSON format.
*
* @since 2.25
*/
public void setIdTokenClaims(String idTokenClaims)
{
this.idTokenClaims = idTokenClaims;
}
/**
* Get the value of the {@code "userinfo"} property in the {@code "claims"}
* request parameter or in the {@code "claims"} property in a request object.
*
*
* A client application may request certain claims be embedded in an ID
* token or in a response from the UserInfo endpoint. There are several
* ways. Including the {@code claims} request parameter and including the
* {@code claims} property in a request object are such examples. In both
* the cases, the value of the {@code claims} parameter/property is JSON.
* Its format is described in 5.5. Requesting Claims using the "claims" Request Parameter of
* OpenID
* Connect Core 1.0.
*
*
*
* The following is an excerpt from the specification. You can find
* {@code "userinfo"} and {@code "id_token"} are top-level properties.
*
*
*
* {
* "userinfo":
* {
* "given_name": {"essential": true},
* "nickname": null,
* "email": {"essential": true},
* "email_verified": {"essential": true},
* "picture": null,
* "http://example.info/claims/groups": null
* },
* "id_token":
* {
* "auth_time": {"essential": true},
* "acr": {"values": ["urn:mace:incommon:iap:silver"] }
* }
* }
*
*
*
* This method ({@code getUserInfoClaims()}) returns the value of the
* {@code "userinfo"} property in JSON format. For example, if the
* JSON above is included in an authorization request, this method
* returns JSON equivalent to the following.
*
*
*
* {
* "given_name": {"essential": true},
* "nickname": null,
* "email": {"essential": true},
* "email_verified": {"essential": true},
* "picture": null,
* "http://example.info/claims/groups": null
* }
*
*
*
* Note that if a request object is given and it contains the
* {@code claims} property and if the {@code claims} request
* parameter is also given, this method returns the value in
* the former.
*
*
* @return
* The value of the {@code "userinfo"} property in the
* {@code "claims"} in JSON format.
*
* @since 2.25
*/
public String getUserInfoClaims()
{
return userInfoClaims;
}
/**
* Set the value of the {@code "userinfo"} property in the {@code "claims"}
* request parameter or in the {@code "claims"} property in a request object.
*
* @param userInfoClaims
* The value of the {@code "userinfo"} property in the
* {@code "claims"} in JSON format.
*
* @since 2.25
*/
public void setUserInfoClaims(String userInfoClaims)
{
this.userInfoClaims = userInfoClaims;
}
/**
* Get the value of the {@code "transformed_claims"} property in the
* {@code "claims"} request parameter or in the {@code "claims"} property
* in a request object.
*
* @return
* The value of the {@code "transformed_claims"} property in the
* {@code "claims"} in JSON format.
*
* @see OpenID Connect Advanced Syntax for Claims (ASC) 1.0
*
* @since 3.8
*/
public String getTransformedClaims()
{
return transformedClaims;
}
/**
* Set the value of the {@code "transformed_claims"} property in the
* {@code "claims"} request parameter or in the {@code "claims"} property
* in a request object.
*
* @param transformedClaims
* The value of the {@code "transformed_claims"} property in the
* {@code "claims"} in JSON format.
*
* @see OpenID Connect Advanced Syntax for Claims (ASC) 1.0
*
* @since 3.8
*/
public void setTransformedClaims(String transformedClaims)
{
this.transformedClaims = transformedClaims;
}
/**
* Get the resources specified by the {@code resource} request parameters
* or by the {@code resource} property in the request object. If both are
* given, the values in the request object take precedence.
* See "Resource Indicators for OAuth 2.0" for details.
*
* @return
* Target resources.
*
* @since 2.62
*/
public URI[] getResources()
{
return resources;
}
/**
* Set the resources specified by the {@code resource} request parameters
* or by the {@code resource} property in the request object. If both are
* given, the values in the request object should be set.
* See "Resource Indicators for OAuth 2.0" for details.
*
* @param resources
* Target resources.
*
* @since 2.62
*/
public void setResources(URI[] resources)
{
this.resources = resources;
}
/**
* Get the authorization details. This represents the value of the
* {@code "authorization_details"} request parameter which is defined in
* "OAuth 2.0 Rich Authorization Requests".
*
* @return
* Authorization details.
*
* @since 2.56
*/
public AuthzDetails getAuthorizationDetails()
{
return authorizationDetails;
}
/**
* Set the authorization details. This represents the value of the
* {@code "authorization_details"} request parameter which is defined in
* "OAuth 2.0 Rich Authorization Requests".
*
* @param details
* Authorization details.
*
* @since 2.56
*/
public void setAuthorizationDetails(AuthzDetails details)
{
this.authorizationDetails = details;
}
/**
* Get the value of the {@code purpose} request parameter.
*
*
* The {@code purpose} request parameter is defined in 8. Transaction-specific Purpose of OpenID Connect for Identity Assurance 1.0 as follows:
*
*
*
*
* {@code purpose} OPTIONAL. String describing the purpose for obtaining
* certain user data from the OP. The purpose MUST NOT be shorter than 3
* characters and MUST NOT be longer than 300 characters. If these rules
* are violated, the authentication request MUST fail and the OP returns
* an error {@code invalid_request} to the RP.
*
*
*
*
* NOTE: This method works only when Authlete server you are using supports
* OpenID Connect for Identity Assurance 1.0.
*
*
* @return
* The value of the {@code purpose} request parameter.
*
* @see OpenID Connect for Identity Assurance 1.0, 8. Transaction-specific Purpose
*
* @since 2.63
*/
public String getPurpose()
{
return purpose;
}
/**
* Set the value of the {@code purpose} request parameter.
*
*
* The {@code purpose} request parameter is defined in 8. Transaction-specific Purpose of OpenID Connect for Identity Assurance 1.0 as follows:
*
*
*
*
* {@code purpose} OPTIONAL. String describing the purpose for obtaining
* certain user data from the OP. The purpose MUST NOT be shorter than 3
* characters and MUST NOT be longer than 300 characters. If these rules
* are violated, the authentication request MUST fail and the OP returns
* an error {@code invalid_request} to the RP.
*
*
*
* @param purpose
* The value of the {@code purpose} request parameter.
*
* @see OpenID Connect for Identity Assurance 1.0, 8. Transaction-specific Purpose
*
* @since 2.63
*/
public void setPurpose(String purpose)
{
this.purpose = purpose;
}
/**
* Get the value of the {@code grant_management_action} request parameter.
*
*
* The {@code grant_management_action} request parameter is defined in
* Grant
* Management for OAuth 2.0, which is supported by Authlete 2.3 and
* newer versions.
*
*
* @return
* A grant management action. {@code null} or one of
* {@link GMAction#CREATE CREATE}, {@link GMAction#REPLACE REPLACE}
* and {@link GMAction#MERGE MERGE}.
*
* @see Grant Management for OAuth 2.0
*
* @since 3.1
*/
public GMAction getGmAction()
{
return gmAction;
}
/**
* Set the value of the {@code grant_management_action} request parameter.
*
*
* The {@code grant_management_action} request parameter is defined in
* Grant
* Management for OAuth 2.0, which is supported by Authlete 2.3 and
* newer versions.
*
*
* @param action
* A grant management action. {@code null} or one of
* {@link GMAction#CREATE CREATE}, {@link GMAction#REPLACE REPLACE}
* and {@link GMAction#MERGE MERGE}.
*
* @see Grant Management for OAuth 2.0
*
* @since 3.1
*/
public void setGmAction(GMAction action)
{
this.gmAction = action;
}
/**
* Get the value of the {@code grant_id} request parameter.
*
*
* The {@code grant_id} request parameter is defined in
* Grant
* Management for OAuth 2.0, which is supported by Authlete 2.3 and
* newer versions.
*
*
* @return
* A grant ID.
*
* @see Grant Management for OAuth 2.0
*
* @since 3.1
*/
public String getGrantId()
{
return grantId;
}
/**
* Set the value of the {@code grant_id} request parameter.
*
*
* The {@code grant_id} request parameter is defined in
* Grant
* Management for OAuth 2.0, which is supported by Authlete 2.3 and
* newer versions.
*
*
* @param grantId
* A grant ID.
*
* @see Grant Management for OAuth 2.0
*
* @since 3.1
*/
public void setGrantId(String grantId)
{
this.grantId = grantId;
}
/**
* Get the subject of the user who has given the grant which is identified
* by the {@code grant_id} request parameter.
*
*
* Authlete 2.3 and newer versions support Grant Management
* for OAuth 2.0. An authorization request may contain a {@code grant_id}
* request parameter which is defined in the specification. If the value of
* the request parameter is valid, {@link #getGrantSubject()} will return
* the subject of the user who has given the grant to the client application.
* Authorization server implementations may use the value returned from
* {@link #getGrantSubject()} in order to determine the user to authenticate.
*
*
*
* The user your system will authenticate during the authorization process
* (or has already authenticated) may be different from the user of the
* grant. The first implementer's draft of "Grant Management for OAuth 2.0"
* does not mention anything about the case, so the behavior in the case is
* left to implementations. Authlete will not perform the grant management
* action when the {@code subject} passed to Authlete does not match the
* user of the grant.
*
*
* @return
* The subject of the user who has given the grant.
*
* @see Grant Management for OAuth 2.0
*
* @since 3.1
*/
public String getGrantSubject()
{
return grantSubject;
}
/**
* Set the subject of the user who has given the grant which is identified
* by the {@code grant_id} request parameter.
*
*
* Authlete 2.3 and newer versions support Grant Management
* for OAuth 2.0. An authorization request may contain a {@code grant_id}
* request parameter which is defined in the specification. If the value of
* the request parameter is valid, {@link #getGrantSubject()} will return
* the subject of the user who has given the grant to the client application.
* Authorization server implementations may use the value returned from
* {@link #getGrantSubject()} in order to determine the user to authenticate.
*
*
*
* The user your system will authenticate during the authorization process
* (or has already authenticated) may be different from the user of the
* grant. The first implementer's draft of "Grant Management for OAuth 2.0"
* does not mention anything about the case, so the behavior in the case is
* left to implementations. Authlete will not perform the grant management
* action when the {@code subject} passed to Authlete does not match the
* user of the grant.
*
*
* @param subject
* The subject of the user who has given the grant.
*
* @see Grant Management for OAuth 2.0
*
* @since 3.1
*/
public void setGrantSubject(String subject)
{
this.grantSubject = subject;
}
/**
* Get the content of the grant which is identified by the {@code grant_id}
* request parameter.
*
*
* The user your system will authenticate during the authorization process
* (or has already authenticated) may be different from the user of the grant.
* Be careful when your system displays the content of the grant.
*
*
* @return
* The content of the grant.
*
* @see Grant Management for OAuth 2.0
*
* @since 3.1
*/
public Grant getGrant()
{
return grant;
}
/**
* Set the content of the grant which is identified by the {@code grant_id}
* request parameter.
*
*
* The user your system will authenticate during the authorization process
* (or has already authenticated) may be different from the user of the grant.
* Be careful when your system displays the content of the grant.
*
*
* @param grant
* The content of the grant.
*
* @see Grant Management for OAuth 2.0
*
* @since 3.1
*/
public void setGrant(Grant grant)
{
this.grant = grant;
}
/**
* Get names of claims that are requested indirectly by "transformed
* claims".
*
*
* A client application can request "transformed claims" by adding
* names of transformed claims in the {@code claims} request parameter.
* The following is an example of the {@code claims} request parameter
* that requests a predefined transformed claim named {@code 18_or_over}
* and a transformed claim named {@code nationality_usa} to be embedded
* in an ID Token.
*
*
*
* {
* "transformed_claims": {
* "nationality_usa": {
* "claim": "nationalities",
* "fn": [
* [ "eq", "USA" ],
* "any"
* ]
* }
* },
* "id_token": {
* "::18_or_over": null,
* ":nationality_usa": null
* }
* }
*
*
*
* The example above assumes that a transformed claim named {@code 18_or_over}
* is predefined by the authorization server like below.
*
*
*
* {
* "18_or_over": {
* "claim": "birthdate",
* "fn": [
* "years_ago",
* [ "gte", 18 ]
* ]
* }
* }
*
*
*
* In the example, the {@code nationalities} claim is requested indirectly
* by the {@code nationality_usa} transformed claim. Likewise, the
* {@code birthdate} claim is requested indirectly by the {@code 18_or_over}
* transformed claim.
*
*
*
* When the {@code claims} request parameter of an authorization request is
* like the example above, this {@code requestedClaimsForTx} property will
* hold the following value.
*
*
*
* [ "birthdate", "nationalities" ]
*
*
*
* It is expected that the authorization server implementation prepares values
* of the listed claims and passes them as the value of the {@code claimsForTx}
* request parameter when it calls the {@code /api/auth/authorization/issue}
* API (cf. {@link AuthorizationIssueRequest#setClaimsForTx(String)}). The
* following is an example of the value of the {@code claimsForTx} request
* parameter.
*
*
*
* {
* "birthdate": "1970-01-23",
* "nationalities": [ "DEU", "USA" ]
* }
*
*
*
* This {@code requestedClaimsForTx} property is available from Authlete 2.3
* onwards.
*
*
* @return
* Names of claims that are requested indirectly by
* "transformed claims"
*
* @see OpenID Connect Advanced Syntax for Claims (ASC) 1.0
*
* @since 3.8
*/
public String[] getRequestedClaimsForTx()
{
return requestedClaimsForTx;
}
/**
* Set names of claims that are requested indirectly by "transformed
* claims".
*
*
* See the description of {@link #getRequestedClaimsForTx()} for details.
*
*
* @param claims
* Names of claims that are requested indirectly by
* "transformed claims"
*
* @see OpenID Connect Advanced Syntax for Claims (ASC) 1.0
*
* @see #getRequestedClaimsForTx()
*
* @since 3.8
*/
public void setRequestedClaimsForTx(String[] claims)
{
this.requestedClaimsForTx = claims;
}
/**
* Get names of verified claims that are requested indirectly by
* "transformed claims".
*
*
* A client application can request "transformed claims" by adding
* names of transformed claims in the {@code claims} request parameter.
* The following is an example of the {@code claims} request parameter
* that requests a predefined transformed claim named {@code 18_or_over}
* and a transformed claim named {@code nationality_usa} to be embedded
* in an ID Token.
*
*
*
* {
* "transformed_claims": {
* "nationality_usa": {
* "claim": "nationalities",
* "fn": [
* [ "eq", "USA" ],
* "any"
* ]
* }
* },
* "id_token": {
* "verified_claims": {
* "verification": {
* "trust_framework": null
* },
* "claims": {
* "::18_or_over": null,
* ":nationality_usa": null
* }
* }
* }
* }
*
*
*
* The example above assumes that a transformed claim named {@code 18_or_over}
* is predefined by the authorization server like below.
*
*
*
* {
* "18_or_over": {
* "claim": "birthdate",
* "fn": [
* "years_ago",
* [ "gte", 18 ]
* ]
* }
* }
*
*
*
* In the example, the {@code nationalities} claim is requested indirectly
* by the {@code nationality_usa} transformed claim. Likewise, the
* {@code birthdate} claim is requested indirectly by the {@code 18_or_over}
* transformed claim.
*
*
*
* When the {@code claims} request parameter of an authorization request is
* like the example above, this {@code requestedVerifiedClaimsForTx} property
* will hold the following value.
*
*
*
* [
* { "array": [ "birthdate", "nationalities" ] }
* ]
*
*
*
* It is expected that the authorization server implementation prepares
* values of the listed verified claims and passes them as the value of
* the {@code verifiedClaimsForTx} request parameter when it calls the
* {@code /api/auth/authorization/issue} API (cf.
* {@link AuthorizationIssueRequest#setVerifiedClaimsForTx(String[])}).
* The following is an example of the value of the
* {@code verifiedClaimsForTx} request parameter.
*
*
*
* [
* "{\"birthdate\":\"1970-01-23\",\"nationalities\":[\"DEU\",\"USA\"]}"
* ]
*
*
*
* The reason that this {@code requestedVerifiedClaimsForTx} property and
* the {@code verifiedClaimsForTx} request parameter are arrays is that
* the {@code "verified_claims"} property in the {@code claims} request
* parameter can be an array like below.
*
*
*
* {
* "transformed_claims": {
* "nationality_usa": {
* "claim": "nationalities",
* "fn": [
* [ "eq", "USA" ],
* "any"
* ]
* }
* },
* "id_token": {
* "verified_claims": [
* {
* "verification": { "trust_framework": { "value": "gold" } },
* "claims": { "::18_or_above": null }
* },
* {
* "verification": { "trust_framework": { "value": "silver" } },
* "claims": { ":nationality_usa": null }
* }
* ]
* }
* }
*
*
*
* The order of elements in {@code requestedVerifiedClaimsForTx} matches
* the order of elements in the {@code "verified_claims"} array.
*
*
*
* This {@code requestedVerifiedClaimsForTx} property is available from
* Authlete 2.3 onwards.
*
*
* @return
* Names of verified claims that are requested indirectly by
* "transformed claims"
*
* @see OpenID Connect Advanced Syntax for Claims (ASC) 1.0
*
* @see OpenID Connect for Identity Assurance 1.0
*
* @since 3.8
*/
public StringArray[] getRequestedVerifiedClaimsForTx()
{
return requestedVerifiedClaimsForTx;
}
/**
* Set names of verified claims that are requested indirectly by
* "transformed claims".
*
*
* See the description of {@link #getRequestedVerifiedClaimsForTx()} for
* details.
*
*
*
* This {@code requestedVerifiedClaimsForTx} property is available from
* Authlete 2.3 onwards.
*
*
* @param claimsArray
* Names of verified claims that are requested indirectly by
* "transformed claims"
*
* @see OpenID Connect Advanced Syntax for Claims (ASC) 1.0
*
* @see OpenID Connect for Identity Assurance 1.0
*
* @see #getRequestedVerifiedClaimsForTx()
*
* @since 3.8
*/
public void setRequestedVerifiedClaimsForTx(StringArray[] claimsArray)
{
this.requestedVerifiedClaimsForTx = claimsArray;
}
/**
* Get the information about the credential offer identified by the
* "{@code issuer_state}" request parameter.
*
*
* Before making an authorization request, a client application may obtain
* a credential offer from a credential issuer. If the
* credential offer contains an issuer state, the client can include
* the issuer state in an authorization request by using the request
* parameter, "{@code issuer_state}". The request parameter is defined in
* the "OpenID for Verifiable Credential Issuance" specification.
*
*
*
* When the feature of "Verifiable Credentials" is enabled, the Authlete
* server recognizes the "{@code issuer_state}" request parameter. When
* an authorization request contains the request parameter, Authlete
* retrieves information about the credential offer identified by the
* issuer state from the database and sets the information to this
* "{@code credentialOfferInfo}" response parameter.
*
*
* @return
* Information about the credential offer identified by the
* "{@code issuer_state}" request parameter.
*
* @since 3.78
* @since Authlete 3.0
*
* @see OpenID for Verifiable Credential Issuance
*/
public CredentialOfferInfo getCredentialOfferInfo()
{
return credentialOfferInfo;
}
/**
* Set the information about the credential offer identified by the
* "{@code issuer_state}" request parameter.
*
*
* See the description of the {@link #getCredentialOfferInfo()} method
* for details.
*
*
* @param info
* Information about the credential offer identified by the
* "{@code issuer_state}" request parameter.
*
* @since 3.78
* @since Authlete 3.0
*
* @see OpenID for Verifiable Credential Issuance
*/
public void setCredentialOfferInfo(CredentialOfferInfo info)
{
this.credentialOfferInfo = info;
}
/**
* Get the information about the issuable credentials that can
* be obtained by presenting the access token that will be issued as a
* result of the authorization request.
*
*
* An authorization request can specify issuable credentials
* by using one or more of the following mechanisms in combination.
*
*
*
* - The "{@code issuer_state}" request parameter.
*
- The "{@code authorization_details}" request parameter.
*
- The "{@code scope}" request parameter.
*
*
*
* When the authorization request specifies one or more issuable
* credentials, this property contains the information about the
* issuable credentials.
*
*
*
* The format of this property is a JSON array whose elements are JSON
* objects. The following is a simple example.
*
*
*
* [
* {
* "format": "vc+sd-jwt",
* "credential_definition": {
* "vct": "https://credentials.example.com/identity_credential"
* }
* }
* ]
*
*
* @return
* Information about the issuable credentials specified by the
* authorization request.
*
* @since 3.78
* @since Authlete 3.0
*
* @see OpenID for Verifiable Credential Issuance
*/
public String getIssuableCredentials()
{
return issuableCredentials;
}
/**
* Set the information about the issuable credentials that can
* be obtained by presenting the access token that will be issued as a
* result of the authorization request.
*
*
* See the description of the {@link #getIssuableCredentials()} method
* for details.
*
*
* @param credentials
* Information about the issuable credentials specified by the
* authorization request.
*
* @since 3.78
* @since Authlete 3.0
*
* @see OpenID for Verifiable Credential Issuance
*/
public void setIssuableCredentials(String credentials)
{
this.issuableCredentials = credentials;
}
/**
* Get the response content which can be used to generate a response
* to the client application. The format of the value varies depending
* on the value of {@code "action"}.
*/
public String getResponseContent()
{
return responseContent;
}
/**
* Set the response content which can be used to generate a response
* to the client application.
*/
public void setResponseContent(String content)
{
this.responseContent = content;
}
/**
* Get the ticket which has been issued to the service implementation
* from Authlete's {@code /auth/authorization} API. This ticket is
* needed for {@code /auth/authorization/issue} API and
* {@code /auth/authorization/fail} API.
*/
public String getTicket()
{
return ticket;
}
/**
* Set the ticket for the service implementation to call
* {@code /auth/authorization/issue} API and
* {@code /auth/authorization/fail} API.
*/
public void setTicket(String ticket)
{
this.ticket = ticket;
}
/**
* Get the summary of this instance.
*/
public String summarize()
{
return String.format(SUMMARY_FORMAT,
ticket,
action,
(client != null ? client.getServiceNumber() : 0),
(client != null ? client.getNumber() : 0),
(client != null ? client.getClientId() : 0),
(client != null ? client.getClientSecret() : null),
(client != null ? client.getClientType() : null),
(client != null ? client.getDeveloper() : null),
display,
maxAge,
Utils.stringifyScopeNames(scopes),
Utils.join(uiLocales, " "),
Utils.join(claimsLocales, " "),
Utils.join(claims, " "),
acrEssential,
clientIdAliasUsed,
Utils.join(acrs, " "),
subject,
loginHint,
lowestPrompt,
Utils.stringifyPrompts(prompts)
);
}
}