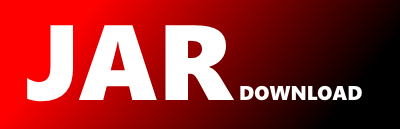
com.authlete.common.dto.HskCreateRequest Maven / Gradle / Ivy
Show all versions of authlete-java-common Show documentation
/*
* Copyright (C) 2021 Authlete, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.authlete.common.dto;
import java.io.Serializable;
/**
* Request to Authlete's {@code /api/hsk/create} API.
*
*
* Note that parameter values specified in the request to the API cannot be
* changed later. Especially, the key ID cannot be changed later. Therefore,
* if you explicitly specify a key ID in the request, the value has to be
* determined carefully before calling the {@code /api/hsk/create} API.
*
*
* @since 2.97
*/
public class HskCreateRequest implements Serializable
{
private static final long serialVersionUID = 1L;
private String kty;
private String use;
private String alg;
private String kid;
private String hsmName;
/**
* Get the key type.
*
* @return
* The key type. {@code "EC"} or {@code "RSA"}.
*
* @see RFC 7517 JSON Web Key (JWK), 4.1. "kty" (Key Type) Parameter
*/
public String getKty()
{
return kty;
}
/**
* Set the key type.
*
* @param kty
* The key type. {@code "EC"} or {@code "RSA"}.
*
* @return
* {@code this} object.
*
* @see RFC 7517 JSON Web Key (JWK), 4.1. "kty" (Key Type) Parameter
*/
public HskCreateRequest setKty(String kty)
{
this.kty = kty;
return this;
}
/**
* Get the use of the key on the HSM.
*
*
* When the key use is {@code "sig"} (signature), the private key on the
* HSM is used to sign data and the corresponding public key is used to
* verify the signature.
*
*
*
* When the key use is {@code "enc"} (encryption), the private key on the
* HSM is used to decrypt encrypted data which have been encrypted with the
* corresponding public key.
*
*
* @return
* The key use. {@code "sig"} (signature) or {@code "enc"}
* (encryption).
*
* @see RFC 7517 JSON Web Key (JWK), 4.2. "use" (Public Key Use) Parameter
*/
public String getUse()
{
return use;
}
/**
* Set the use of the key on the HSM.
*
*
* When the key use is {@code "sig"} (signature), the private key on the
* HSM is used to sign data and the corresponding public key is used to
* verify the signature.
*
*
*
* When the key use is {@code "enc"} (encryption), the private key on the
* HSM is used to decrypt encrypted data which have been encrypted with the
* corresponding public key.
*
*
* @param use
* The key use. {@code "sig"} (signature) or {@code "enc"}
* (encryption).
*
* @return
* {@code this} object.
*
* @see RFC 7517 JSON Web Key (JWK), 4.2. "use" (Public Key Use) Parameter
*/
public HskCreateRequest setUse(String use)
{
this.use = use;
return this;
}
/**
* Get the algorithm of the key on the HSM.
*
*
* When the key use is {@code "sig"}, the algorithm represents a signing
* algorithm such as {@code "ES256"}.
*
*
*
* When the key use is {@code "enc"}, the algorithm represents an
* encryption algorithm such as {@code "RSA-OAEP-256"}.
*
*
*
* It is rare that HSMs support all the algorithms listed in RFC 7518 JSON Web
* Algorithms (JWA). When the specified algorithm is not supported
* by the HSM, the request to the {@code /api/hsk/create} API fails.
*
*
* @return
* The algorithm.
*
* @see RFC 7517 JSON Web Key (JWK), 4.4. "alg" (Algorithm) Parameter
*
* @see RFC 7518 JSON Web Algorithms (JWA), 3.1. "alg" (Algorithm) Header Parameter Values for JWS
*
* @see RFC 7518 JSON Web Algorithms (JWA), 4.1. "alg" (Algorithm) Header Parameter Values for JWE
*/
public String getAlg()
{
return alg;
}
/**
* Set the algorithm of the key on the HSM.
*
*
* When the key use is {@code "sig"}, the algorithm represents a signing
* algorithm such as {@code "ES256"}.
*
*
*
* When the key use is {@code "enc"}, the algorithm represents an
* encryption algorithm such as {@code "RSA-OAEP-256"}.
*
*
*
* It is rare that HSMs support all the algorithms listed in RFC 7518 JSON Web
* Algorithms (JWA). When the specified algorithm is not supported
* by the HSM, the request to the {@code /api/hsk/create} API fails.
*
*
* @param alg
* The algorithm.
*
* @return
* {@code this} object.
*
* @see RFC 7517 JSON Web Key (JWK), 4.4. "alg" (Algorithm) Parameter
*
* @see RFC 7518 JSON Web Algorithms (JWA), 3.1. "alg" (Algorithm) Header Parameter Values for JWS
*
* @see RFC 7518 JSON Web Algorithms (JWA), 4.1. "alg" (Algorithm) Header Parameter Values for JWE
*/
public HskCreateRequest setAlg(String alg)
{
this.alg = alg;
return this;
}
/**
* Get the key ID for the key on the HSM.
*
*
* Note that the key ID cannot be changed later. Determine the key ID
* carefully before calling the {@code /api/hsk/create} API. If the
* {@code kid} request parameter is missing or its value is empty,
* the API generates a random key ID (base64url-encoded 256-bit random
* value) for the request.
*
*
*
* Also note that Authlete does not check duplication of key IDs.
*
*
* @return
* The key ID.
*
* @see RFC 7517 JSON Web Key (JWK), 4.5. "kid" (Key ID) Parameter
*/
public String getKid()
{
return kid;
}
/**
* Set the key ID for the key on the HSM.
*
*
* Note that the key ID cannot be changed later. Determine the key ID
* carefully before calling the {@code /api/hsk/create} API. If the
* {@code kid} request parameter is missing or its value is empty,
* the API generates a random key ID (base64url-encoded 256-bit random
* value) for the request.
*
*
*
* Also note that Authlete does not check duplication of key IDs.
*
*
* @param kid
* The key ID.
*
* @return
* {@code this} object.
*
* @see RFC 7517 JSON Web Key (JWK), 4.5. "kid" (Key ID) Parameter
*/
public HskCreateRequest setKid(String kid)
{
this.kid = kid;
return this;
}
/**
* Get the name of the HSM.
*
*
* The identifier for the HSM that sits behind the Authlete server.
* For example, {@code "google"}. If the {@code hsmName} request parameter
* is missing or its value is empty, the API uses the default HSM. The
* value of the default HSM varies depending on the configuration of the
* Authlete server.
*
*
* @return
* The name of the HSM.
*/
public String getHsmName()
{
return hsmName;
}
/**
* Set the name of the HSM.
*
*
* The identifier for the HSM that sits behind the Authlete server.
* For example, {@code "google"}. If the {@code hsmName} request parameter
* is missing or its value is empty, the API uses the default HSM. The
* value of the default HSM varies depending on the configuration of the
* Authlete server.
*
*
* @param hsmName
* The name of the HSM.
*
* @return
* {@code this} object.
*/
public HskCreateRequest setHsmName(String hsmName)
{
this.hsmName = hsmName;
return this;
}
}