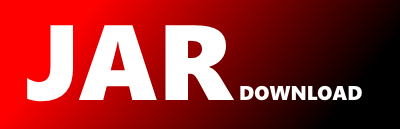
com.authlete.common.dto.IntrospectionRequest Maven / Gradle / Ivy
Show all versions of authlete-java-common Show documentation
/*
* Copyright (C) 2014-2024 Authlete, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.authlete.common.dto;
import java.io.Serializable;
import java.net.URI;
/**
* Request to Authlete's {@code /auth/introspection} API.
*
*
*
* token
(REQUIRED)
* -
*
* An access token to introspect.
*
*
*
* scopes
(OPTIONAL)
* -
*
* Scopes that should be covered by the access token.
*
*
*
* subject
(OPTIONAL)
* -
*
* The subject that should be associated with the access token.
*
*
*
* clientCertificate
(OPTIONAL)
* -
*
* The client certificate used in the mutual TLS connection established
* between the client application and the protected resource endpoint.
* See RFC 8705
* OAuth 2.0 Mutual-TLS Client Authentication and Certificate-Bound Access
* Tokens for details.
*
*
*
* dpop
(OPTIONAL)
* -
*
* The value of the {@code DPoP} HTTP header.
* See RFC 9449 OAuth
* 2.0 Demonstrating Proof of Possession (DPoP) for details.
*
*
*
* htm
(OPTIONAL)
* -
*
* The HTTP method of the request to the protected resource endpoint.
* See RFC 9449 OAuth
* 2.0 Demonstrating Proof of Possession (DPoP) for details.
*
*
*
* htu
(OPTIONAL)
* -
*
* The URL of the protected resource endpoint.
* See RFC 9449 OAuth
* 2.0 Demonstrating Proof of Possession (DPoP) for details.
*
*
*
* resources
(OPTIONAL)
* -
*
* Resource indicators that should be covered by the access token.
* See RFC 8707
* Resource Indicators for OAuth 2.0 for details.
*
*
*
* targetUri
(OPTIONAL; Authlete 2.3 onwards)
* -
*
* The full URI of the resource request, including the query part, if any.
*
*
*
* headers
(OPTIONAL; Authlete 2.3 onwards)
* -
*
* The HTTP headers included in the resource request. They are used to compute
* component values, which will be part of the signature base for HTTP message
* signatures.
*
*
*
* requestBodyContained
(OPTIONAL; Authlete 2.3 onwards)
* -
*
* The flag indicating whether the resource request contains a request body.
*
*
*
* acrValues
(OPTIONAL; Authlete 2.3 onwards)
* -
*
* The list of Authentication Context Class Reference values one of which
* the user authentication performed during the course of issuing the access
* token must satisfy.
*
*
*
* maxAge
(OPTIONAL; Authlete 2.3 onwards)
* -
*
* The maximum authentication age which is the maximum allowable elapsed time
* since the user authentication was performed during the course of issuing the
* access token.
*
*
*
* dpopNonceRequired
(OPTIONAL; Authlete 3.0 onwards)
* -
*
* The flag indicating whether to require the DPoP proof JWT to include the
* {@code nonce} claim. Even if the service's {@code dpopNonceRequired} property
* is false, calling the {@code /auth/introspection} API with this
* {@code dpopNonceRequired} parameter true will force the Authlete API to check
* whether the DPoP proof JWT includes the expected nonce value.
*
*
*
*
*
*
* @author Takahiko Kawasaki
*
* @see RFC 6750 The OAuth 2.0 Authorization Framework: Bearer Token Usage
*
* @see RFC 8705 OAuth 2.0 Mutual-TLS Client Authentication and Certificate-Bound Access Tokens
*
* @see RFC 8707 Resource Indicators for OAuth 2.0
*
* @see RFC 9449 OAuth 2.0 Demonstrating Proof of Possession (DPoP)
*
* @see RFC 9470 OAuth 2.0 Step Up Authentication Challenge Protocol
*/
public class IntrospectionRequest implements Serializable
{
private static final long serialVersionUID = 8L;
/**
* Access token to introspect.
*/
private String token;
/**
* Required scopes for access to the protected resource endpoint.
*/
private String[] scopes;
/**
* Expected identifier of resource owner.
*/
private String subject;
/**
* Client certificate used in the mutual TLS connection between
* the client application and the protected resource endpoint.
*/
private String clientCertificate;
/**
* DPoP Header.
*/
private String dpop;
/**
* HTTP Method (for DPoP validation).
*/
private String htm;
/**
* HTTP URL base (for DPoP validation).
*/
private String htu;
/**
* Resource indicators.
*/
private URI[] resources;
/**
* The full URL of the resource server.
*
* @since 3.38
* @since Authlete 2.3
* @deprecated
*/
@Deprecated
private String uri;
/**
* The full URL of the resource request, including the query part, if any.
*
* @since 4.13
* @since Authlete 2.3.27
*/
private URI targetUri;
/**
* The HTTP message body of the request, if present.
*
* @since 3.38
* @since Authlete 2.3
* @deprecated
*/
@Deprecated
private String message;
/**
* The HTTP headers included in the resource request. They are used to
* compute component values, which will be part of the signature base
* for HTTP message signatures.
*
* @since 3.38
* @since Authlete 2.3
*/
private Pair[] headers;
/**
* The flag indicating whether the resource request contains a request body.
*
* @since 4.13
* @since Authlete 2.3.27
*/
private boolean requestBodyContained;
/**
* HTTP Message Components required to be in the signature. If absent,
* defaults to "@method", "@target-uri", and appropriate headers such as
* "authorization" and "dpop".
*
* @since 3.38
* @since Authlete 2.3
* @deprecated
*/
@Deprecated
private String[] requiredComponents;
/**
* Authentication Context Class Reference values one of which the
* user authentication performed during the course of issuing the
* access token must satisfy.
*
* @since 3.40
* @since Authlete 2.3
*/
private String[] acrValues;
/**
* The maximum authentication age which is the maximum allowable
* elapsed time since the user authentication was performed during
* the course of issuing the access token.
*
* @since 3.40
* @since Authlete 2.3
*/
private int maxAge;
/**
* Whether to check if the DPoP proof JWT includes the expected nonce value.
*
* @since 3.82
* @since Authlete 3.0
*/
private boolean dpopNonceRequired;
/**
* Get the access token to introspect.
*
* @return
* The access token.
*/
public String getToken()
{
return token;
}
/**
* Set the access token to introspect.
*
* @param token
* The access token.
*
* @return
* {@code this} object.
*/
public IntrospectionRequest setToken(String token)
{
this.token = token;
return this;
}
/**
* Get the scopes which are required to access the protected resource
* endpoint.
*
* @return
* Required scopes.
*/
public String[] getScopes()
{
return scopes;
}
/**
* Set the scopes which are required to access the protected resource
* endpoint.
*
*
* If the array contains a scope which is not covered by the access token,
* Authlete's {@code /auth/introspection} API returns {@code FORBIDDEN} as
* the action and {@code insufficent_scope} as the error code.
*
*
* @param scopes
* Scopes required to access the protected resource endpoint.
* If {@code null} is given, the {@code /auth/introspection}
* API does not perform scope checking.
*
* @return
* {@code this} object.
*/
public IntrospectionRequest setScopes(String[] scopes)
{
this.scopes = scopes;
return this;
}
/**
* Get the subject (= end-user ID managed by the service implementation)
* which is required to access the protected resource endpoint.
*
* @return
* Expected identifier of resource owner.
*/
public String getSubject()
{
return subject;
}
/**
* Set the subject (= end-user ID managed by the service implementation)
* which is required to access the protected resource endpoint.
*
*
* If the specified subject is different from the one associated with the
* access token, Authlete's {@code /auth/introspection} API returns
* {@code FORBIDDEN} as the action and {@code invalid_request} as the error
* code.
*
*
* @param subject
* Subject (= end-user ID managed by the service implementation)
* which is required to access the protected resource endpoint.
* If {@code null} is given, the {@code /auth/introspection} API
* does not perform subject checking.
*
* @return
* {@code this} object.
*/
public IntrospectionRequest setSubject(String subject)
{
this.subject = subject;
return this;
}
/**
* Get the client certificate used in the mutual TLS connection established
* between the client application and the protected resource endpoint.
*
* @return
* The client certificate in PEM format.
*
* @since 2.14
*
* @see RFC 8705 OAuth 2.0 Mutual-TLS Client Authentication and Certificate-Bound Access Tokens
*/
public String getClientCertificate()
{
return clientCertificate;
}
/**
* Set the client certificate used in the mutual TLS connection established
* between the client application and the protected resource endpoint.
*
*
* If the access token is bound to a client certificate, this parameter is
* used for validation.
*
*
* @param clientCertificate
* The client certificate in PEM format.
*
* @return
* {@code this} object.
*
* @since 2.14
*
* @see RFC 8705 OAuth 2.0 Mutual-TLS Client Authentication and Certificate-Bound Access Tokens
*/
public IntrospectionRequest setClientCertificate(String clientCertificate)
{
this.clientCertificate = clientCertificate;
return this;
}
/**
* Get the {@code DPoP} header presented by the client during the request
* to the resource server. This header contains a signed JWT which
* includes the public key that is paired with the private key used to
* sign it.
*
* @return
* The {@code DPoP} header string.
*
* @since 2.70
*
* @see RFC 9449 OAuth 2.0 Demonstrating Proof of Possession (DPoP)
*/
public String getDpop()
{
return dpop;
}
/**
* Set the {@code DPoP} header presented by the client during the request
* to the resource server. This header contains a signed JWT which
* includes the public key that is paired with the private key used to
* sign it.
*
*
* If the access token is bound to a public key via DPoP, this parameter
* is used for validation.
*
*
* @param dpop
* The {@code DPoP} header string.
*
* @return
* {@code this} object.
*
* @since 2.70
*
* @see RFC 9449 OAuth 2.0 Demonstrating Proof of Possession (DPoP)
*/
public IntrospectionRequest setDpop(String dpop)
{
this.dpop = dpop;
return this;
}
/**
* Get the HTTP method of the request from the client to the protected
* resource endpoint. This field is used to validate the {@code DPoP}
* header.
*
*
* NOTE: This parameter was added for DPoP, but now it is also used as the
* value of the {@code @method} derived component of HTTP message signatures
* (RFC
* 9421 HTTP Message Signatures, Section 2.2.1. Method).
*
*
* @return
* The HTTP method as a string. For example, {@code "GET"}.
*
* @since 2.70
*
* @see RFC 9449 OAuth 2.0 Demonstrating Proof of Possession (DPoP)
*
* @see RFC 9421 HTTP Message Signatures, Section 2.2.1. Method
*/
public String getHtm()
{
return htm;
}
/**
* Set the HTTP method of the request from the client to the protected
* resource endpoint. This field is used to validate the {@code DPoP}
* header.
*
*
* If the access token is bound to a public key via DPoP, this parameter
* is used for validation.
*
*
*
* NOTE: This parameter was added for DPoP, but now it is also used as the
* value of the {@code @method} derived component of HTTP message signatures
* (RFC
* 9421 HTTP Message Signatures, Section 2.2.1. Method).
*
*
* @param htm
* The HTTP method as a string. For example, {@code "GET"}.
*
* @return
* {@code this} object.
*
* @since 2.70
*
* @see RFC 9449 OAuth 2.0 Demonstrating Proof of Possession (DPoP)
*
* @see RFC 9421 HTTP Message Signatures, Section 2.2.1. Method
*/
public IntrospectionRequest setHtm(String htm)
{
this.htm = htm;
return this;
}
/**
* Get the URL of the protected resource endpoint. This field is used
* to validate the {@code DPoP} header.
*
* @return
* The URL of the protected resource endpoint.
*
* @since 2.70
*
* @see RFC 9449 OAuth 2.0 Demonstrating Proof of Possession (DPoP)
*/
public String getHtu()
{
return htu;
}
/**
* Set the URL of the protected resource endpoint. This field is used
* to validate the {@code DPoP} header.
*
*
* If the access token is bound to a public key via DPoP, this parameter
* is used for validation.
*
*
* @param htu
* The URL of the protected resource endpoint.
*
* @return
* {@code this} object.
*
* @since 2.70
*
* @see RFC 9449 OAuth 2.0 Demonstrating Proof of Possession (DPoP)
*/
public IntrospectionRequest setHtu(String htu)
{
this.htu = htu;
return this;
}
/**
* Get the resource indicators that the access token should cover.
*
* @return
* The resource indicators.
*
* @since 3.1
*
* @see RFC 8707 Resource Indicators for OAuth 2.0
*/
public URI[] getResources()
{
return resources;
}
/**
* Set the resource indicators that the access token should cover.
*
* @param resources
* The resource indicators that the access token should cover to
* access the protected resource endpoint. If {@code null} is
* given, the {@code /auth/introspection} API does not perform
* resource indicator checking.
*
* @return
* {@code this} object.
*
* @since 3.3
*
* @see RFC 8707 Resource Indicators for OAuth 2.0
*/
public IntrospectionRequest setResources(URI[] resources)
{
this.resources = resources;
return this;
}
/**
* Get the URL of the resource server. This field is used to validate
* the HTTP Message Signature.
*
* @return
* The URL of the resource server.
*
* @since 3.38
* @since Authlete 2.3
* @deprecated
*/
@Deprecated
public String getUri()
{
return uri;
}
/**
* Set the URL of the resource server. This field is used to validate
* the HTTP Message Signature.
*
* @param uri
* The URL of the resource server.
*
* @return
* {@code this} object.
*
* @since 3.38
* @since Authlete 2.3
* @deprecated
*/
@Deprecated
public IntrospectionRequest setUri(String uri)
{
this.uri = uri;
return this;
}
/**
* Get the target URI of the resource request, including the query part,
* if any.
*
*
* This parameter is used as the value of the {@code @target-uri} derived
* component for HTTP message signatures (RFC 9421
* HTTP Message Signatures, Section 2.2.2. Target URI). Additionally,
* other derived components such as {@code @authority}, {@code @scheme},
* {@code @path}, {@code @query} and {@code @query-param} are computed
* from this parameter.
*
*
*
* When this parameter is omitted, the value of the {@code htu} parameter
* is used. The {@code htu} parameter represents the URL of the resource
* endpoint, which is identical to the target URI of the resource request
* as long as the request does not include a query component. Conversely,
* if the resource request includes a query component, the value of the
* {@code htu} parameter will not match the target URI, and in that case,
* the HTTP message signature verification will fail.
*
*
*
* If neither this {@code targetUri} parameter nor the {@code htu}
* parameter is specified, the target URI is considered unavailable.
* If HTTP message signing requires the {@code target-uri} derived
* component or other derived components computed based on the target
* URI, the HTTP message signature verification will fail.
*
*
* @return
* The target URI of the resource request.
*
* @since 4.13
* @since Authlete 2.3.27
*
* @see RFC 9421 HTTP Message Signatures, Section 2.2.2. Target URI
*/
public URI getTargetUri()
{
return targetUri;
}
/**
* Set the target URI of the resource request, including the query part,
* if any.
*
*
* This parameter is used as the value of the {@code @target-uri} derived
* component for HTTP message signatures (RFC 9421
* HTTP Message Signatures, Section 2.2.2. Target URI). Additionally,
* other derived components such as {@code @authority}, {@code @scheme},
* {@code @path}, {@code @query} and {@code @query-param} are computed
* from this parameter.
*
*
*
* When this parameter is omitted, the value of the {@code htu} parameter
* is used. The {@code htu} parameter represents the URL of the resource
* endpoint, which is identical to the target URI of the resource request
* as long as the request does not include a query component. Conversely,
* if the resource request includes a query component, the value of the
* {@code htu} parameter will not match the target URI, and in that case,
* the HTTP message signature verification will fail.
*
*
*
* If neither this {@code targetUri} parameter nor the {@code htu}
* parameter is specified, the target URI is considered unavailable.
* If HTTP message signing requires the {@code target-uri} derived
* component or other derived components computed based on the target
* URI, the HTTP message signature verification will fail.
*
*
* @param targetUri
* The target URI of the resource request.
*
* @return
* {@code this} object.
*
* @since 4.13
* @since Authlete 2.3.27
*
* @see RFC 9421 HTTP Message Signatures, Section 2.2.2. Target URI
*/
public IntrospectionRequest setTargetUri(URI targetUri)
{
this.targetUri = targetUri;
return this;
}
/**
* Get the HTTP message body, if present. If provided, this will be used to calculate
* the expected value of the {@code Content-Digest} in the headers of the request
* covered by the HTTP Message Signature.
*
* @return
* The HTTP message body.
*
* @since 3.38
* @since Authlete 2.3
* @deprecated
*/
@Deprecated
public String getMessage()
{
return message;
}
/**
* Set the HTTP message body, if present. If provided, this will be used to calculate
* the expected value of the {@code Content-Digest} in the headers of the request
* covered by the HTTP Message Signature.
*
* @param message
* The HTTP message body.
*
* @return
* {@code this} object.
*
* @since 3.38
* @since Authlete 2.3
* @deprecated
*/
@Deprecated
public IntrospectionRequest setMessage(String message)
{
this.message = message;
return this;
}
/**
* Get the HTTP headers included in the resource request. They are used
* to compute component values, which will be part of the signature base
* for HTTP message signatures.
*
* @return
* The HTTP headers.
*
* @since 3.38
* @since Authlete 2.3
*/
public Pair[] getHeaders()
{
return headers;
}
/**
* Set the HTTP headers included in the resource request. They are used
* to compute component values, which will be part of the signature base
* for HTTP message signatures.
*
* @param headers
* The HTTP headers.
*
* @return
* {@code this} object.
*
* @since 3.38
* @since Authlete 2.3
*/
public IntrospectionRequest setHeaders(Pair[] headers)
{
this.headers = headers;
return this;
}
/**
* Get the flag indicating whether the resource request contains a request
* body.
*
*
* When the resource request must comply with the HTTP message signing
* requirements defined in the FAPI 2.0 Message Signing specification, the
* {@code "content-digest"} component identifier must be included in the
* signature base of the HTTP message signature (see RFC 9421 HTTP Message
* Signatures) if the resource request contains a request body.
*
*
*
* When this {@code requestBodyContained} parameter is {@code true},
* Authlete checks whether {@code "content-digest"} is included in the
* signature base, if the FAPI profile applies to the resource request.
*
*
* @return
* {@code true} if the resource request contains a request body.
*
* @since 4.13
* @since Authlete 2.3.27
*/
public boolean isRequestBodyContained()
{
return requestBodyContained;
}
/**
* Set the flag indicating whether the resource request contains a request
* body.
*
*
* When the resource request must comply with the HTTP message signing
* requirements defined in the FAPI 2.0 Message Signing specification, the
* {@code "content-digest"} component identifier must be included in the
* signature base of the HTTP message signature (see RFC 9421 HTTP Message
* Signatures) if the resource request contains a request body.
*
*
*
* When this {@code requestBodyContained} parameter is {@code true},
* Authlete checks whether {@code "content-digest"} is included in the
* signature base, if the FAPI profile applies to the resource request.
*
*
* @param contained
* {@code true} to indicate that the resource request contains
* a request body.
*
* @return
* {@code this} object.
*
* @since 4.13
* @since Authlete 2.3.27
*/
public IntrospectionRequest setRequestBodyContained(boolean contained)
{
this.requestBodyContained = contained;
return this;
}
/**
* Get the list of component identifiers required to be covered by
* the signature on this message. If this is omitted, the set defaults to
* including the {@code @method} and {@code @target-uri} derived components
* as well the {@code Authorization} header and, if present,
* the {@code DPoP} header.
*
* @return
* The component identifiers to cover in the signature.
*
* @since 3.38
* @since Authlete 2.3
* @deprecated
*/
@Deprecated
public String[] getRequiredComponents()
{
return requiredComponents;
}
/**
* Set the list of component identifiers required to be covered by
* the signature on this message. If this is omitted, the set defaults to
* including the {@code @method} and {@code @target-uri} derived components
* as well the {@code Authorization} header and, if present,
* the {@code DPoP} header.
*
* @param requiredComponents
* The component identifiers to cover in the signature.
*
* @return
* {@code this} object.
*
* @since 3.38
* @since Authlete 2.3
* @deprecated
*/
@Deprecated
public IntrospectionRequest setRequiredComponents(String[] requiredComponents)
{
this.requiredComponents = requiredComponents;
return this;
}
/**
* Get the list of Authentication Context Class Reference values one of
* which the user authentication performed during the course of issuing
* the access token must satisfy.
*
* @return
* The list of Authentication Context Class Reference values.
*
* @since 3.40
* @since Authlete 2.3
*
* @see RFC 9470 OAuth 2.0 Step Up Authentication Challenge Protocol
*/
public String[] getAcrValues()
{
return acrValues;
}
/**
* Set the list of Authentication Context Class Reference values one of
* which the user authentication performed during the course of issuing
* the access token must satisfy.
*
* @param acrValues
* The list of Authentication Context Class Reference values.
* If {@code null} is given, the {@code /auth/introspection} API
* does not perform ACR checking.
*
* @return
* {@code this} object.
*
* @since 3.40
* @since Authlete 2.3
*
* @see RFC 9470 OAuth 2.0 Step Up Authentication Challenge Protocol
*/
public IntrospectionRequest setAcrValues(String[] acrValues)
{
this.acrValues = acrValues;
return this;
}
/**
* Get the maximum authentication age which is the maximum allowable
* elapsed time since the user authentication was performed during
* the course of issuing the access token.
*
* @return
* The maximum authentication age in seconds.
*
* @since 3.40
* @since Authlete 2.3
*
* @see RFC 9470 OAuth 2.0 Step Up Authentication Challenge Protocol
*/
public int getMaxAge()
{
return maxAge;
}
/**
* Set the maximum authentication age which is the maximum allowable
* elapsed time since the user authentication was performed during
* the course of issuing the access token.
*
* @param maxAge
* The maximum authentication age in seconds. If 0 or a negative
* value is given, the {@code /auth/introspection} API does not
* perform max age checking.
*
* @return
* {@code this} object.
*
* @since 3.40
* @since Authlete 2.3
*
* @see RFC 9470 OAuth 2.0 Step Up Authentication Challenge Protocol
*/
public IntrospectionRequest setMaxAge(int maxAge)
{
this.maxAge = maxAge;
return this;
}
/**
* Get the flag indicating whether to check if the DPoP proof JWT includes
* the expected {@code nonce} value.
*
*
* If this request parameter is {@code true} or if the service's
* {@code dpopNonceRequired} property ({@link Service#isDpopNonceRequired()})
* is {@code true}, the {@code /auth/introspection} API checks if the DPoP
* proof JWT includes the expected {@code nonce} value. In this case, the
* response from the {@code /auth/introspection} API will include the
* {@code dpopNonce} response parameter, which should be used as the value
* of the {@code DPoP-Nonce} HTTP header.
*
*
* @return
* {@code true} if the {@code /auth/introspection} API checks
* whether the DPoP proof JWT includes the expected {@code nonce}
* value, even if the service's {@code dpopNonceRequired} property
* is false.
*
* @since 3.82
* @since Authlete 3.0
*
* @see RFC 9449 OAuth 2.0 Demonstrating Proof of Possession (DPoP)
*/
public boolean isDpopNonceRequired()
{
return dpopNonceRequired;
}
/**
* Set the flag indicating whether to check if the DPoP proof JWT includes
* the expected {@code nonce} value.
*
*
* If this request parameter is {@code true} or if the service's
* {@code dpopNonceRequired} property ({@link Service#isDpopNonceRequired()})
* is {@code true}, the {@code /auth/introspection} API checks if the DPoP
* proof JWT includes the expected {@code nonce} value. In this case, the
* response from the {@code /auth/introspection} API will include the
* {@code dpopNonce} response parameter, which should be used as the value
* of the {@code DPoP-Nonce} HTTP header.
*
*
* @param required
* {@code true} to have the {@code /auth/introspection} API check
* whether the DPoP proof JWT includes the expected {@code nonce}
* value, even if the service's {@code dpopNonceRequired} property
* is false.
*
* @return
* {@code this} object.
*
* @since 3.82
* @since Authlete 3.0
*
* @see RFC 9449 OAuth 2.0 Demonstrating Proof of Possession (DPoP)
*/
public IntrospectionRequest setDpopNonceRequired(boolean required)
{
this.dpopNonceRequired = required;
return this;
}
}