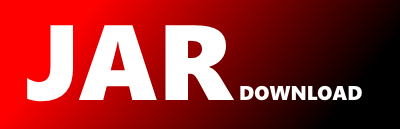
com.authlete.jakarta.ConfigurationRequestHandler Maven / Gradle / Ivy
Show all versions of authlete-java-jakarta Show documentation
/*
* Copyright (C) 2016-2022 Authlete, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific
* language governing permissions and limitations under the
* License.
*/
package com.authlete.jakarta;
import jakarta.ws.rs.WebApplicationException;
import jakarta.ws.rs.core.Response;
import com.authlete.common.api.AuthleteApi;
import com.authlete.common.dto.ServiceConfigurationRequest;
/**
* Handler for requests to an OpenID Provider configuration endpoint.
*
*
* An OpenID Provider that supports OpenID Connect
* Discovery 1.0 must provide an endpoint that returns its configuration
* information in a JSON format. Details about the format are described in
* "3. OpenID Provider Metadata" in OpenID Connect Discovery 1.0.
*
*
*
* In an implementation of configuration endpoint, call {@link #handle()} method
* and use the response as the response from the endpoint to the client application.
*
*
*
* Note that the URI of an OpenID Provider configuration endpoint is defined in
* "4.1. OpenID Provider Configuration Request" in OpenID Connect Discovery
* 1.0. In short, the URI must be:
*
*
*
* Issuer Identifier + {@code /.well-known/openid-configuration}
*
*
*
* Issuer Identifier is a URL to identify an OpenID Provider. For example,
* {@code https://example.com}. For details about Issuer Identifier, See {@code issuer}
* in "3. OpenID Provider Metadata" (OpenID Connect Discovery 1.0) and {@code iss} in
* "2. ID Token"
* (OpenID Connect Core 1.0).
*
*
*
* You can change the Issuer Identifier of your service using the management console
* (Service Owner Console).
* Note that the default value of Issuer Identifier is not appropriate for commercial
* use, so you should change it.
*
*
* @since 1.1
*
* @author Takahiko Kawasaki
*/
public class ConfigurationRequestHandler extends BaseHandler
{
/**
* Constructor with an implementation of {@link AuthleteApi} interface.
*
* @param api
* Implementation of {@link AuthleteApi} interface.
*/
public ConfigurationRequestHandler(AuthleteApi api)
{
super(api);
}
/**
* Handle a request to an OpenID Provider configuration endpoint. This
* method is an alias of {@link #handle(boolean) handle}{@code (true)}.
*
* @return
* A response that should be returned from the endpoint to
* the client application.
*
* @throws WebApplicationException
* An error occurred.
*/
public Response handle() throws WebApplicationException
{
return handle(true);
}
/**
* Handle a request to an OpenID Provider configuration endpoint. This
* method internally calls Authlete's {@code /api/service/configuration}
* API.
*
* @param pretty
* {@code true} to return the output JSON in pretty format.
*
* @return
* A response that should be returned from the endpoint to
* the client application.
*
* @throws WebApplicationException
* An error occurred.
*/
public Response handle(boolean pretty) throws WebApplicationException
{
try
{
// Call Authlete's /api/service/configuration API.
// The API returns a JSON that complies with
// OpenID Connect Discovery 1.0.
String json = getApiCaller().callServiceConfiguration(pretty);
// Response as "application/json;charset=UTF-8" with 200 OK.
return ResponseUtil.ok(json);
}
catch (WebApplicationException e)
{
// The API call raised an exception.
throw e;
}
catch (Throwable t)
{
// Unexpected error.
throw unexpected("Unexpected error in ConfigurationRequestHandler", t);
}
}
/**
* Handle a request to an OpenID Provider configuration endpoint. This
* method internally calls Authlete's {@code /api/service/configuration}
* API.
*
* @param request
* Request parameters to the Authlete API.
*
* @return
* A response that should be returned from the discovery endpoint.
*
* @throws WebApplicationException
* An error occurred.
*
* @since 2.50
*/
public Response handle(ServiceConfigurationRequest request) throws WebApplicationException
{
try
{
// Call Authlete's /api/service/configuration API.
// The API returns a JSON that complies with
// OpenID Connect Discovery 1.0.
String json = getApiCaller().callServiceConfiguration(request);
// Response as "application/json;charset=UTF-8" with 200 OK.
return ResponseUtil.ok(json);
}
catch (WebApplicationException e)
{
// The API call raised an exception.
throw e;
}
catch (Throwable t)
{
// Unexpected error.
throw unexpected("Unexpected error in ConfigurationRequestHandler", t);
}
}
}