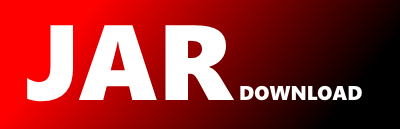
com.authlete.cose.COSEMessage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cbor Show documentation
Show all versions of cbor Show documentation
A Java library for CBOR, COSE, CWT and mdoc.
The newest version!
/*
* Copyright (C) 2023 Authlete, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.authlete.cose;
import com.authlete.cbor.CBORByteArray;
import com.authlete.cbor.CBORItem;
import com.authlete.cbor.CBORNull;
import com.authlete.cbor.CBORTaggedItem;
/**
* COSE Message
*
*
* This class represents {@code COSE_Untagged_Message} which is defined in
* 2. Basic
* COSE Structure of RFC 9052.
*
*
* @since 1.1
*
* @see RFC 9052, 2. Basic COSE Structure
*/
public abstract class COSEMessage extends COSEObject
{
private final COSEMessageType type;
/**
* A constructor.
*
* @param type
* The type of this COSE message.
*
* @param protectedHeader
* The protected header. Must not be null.
*
* @param unprotectedHeader
* The unprotected header. Must not be null.
*
* @param content
* The content. Must be either {@link CBORByteArray} or
* {@link CBORNull}.
*
* @param additionalItems
* Additional items.
*
* @throws IllegalArgumentException
* {@code type} is null,
* {@code protectedHeader} is null, {@code unprotectedHeader} is
* null, or {@code content} is neither a {@link CBORByteArray}
* instance nor a {@link CBORNull} instance.
*/
public COSEMessage(
COSEMessageType type,
COSEProtectedHeader protectedHeader,
COSEUnprotectedHeader unprotectedHeader,
CBORItem content,
CBORItem... additionalItems) throws IllegalArgumentException
{
super(protectedHeader, unprotectedHeader, content, additionalItems);
if (type == null)
{
throw new IllegalArgumentException("The COSE message type is missing.");
}
this.type = type;
setComment(type.getName());
}
/**
* Get the type of this COSE message.
*
* @return
* The type of this COSE message.
*
* @since 1.4
*/
public COSEMessageType getType()
{
return type;
}
/**
* Get the tag number of this COSE message.
*
*
*
*
*
* CBOR Tag
* Data Item
* Java Class
*
*
*
* 16
* {@code COSE_Encrypt0}
* {@link COSEEncrypt0}
*
*
*
* 17
* {@code COSE_Mac0}
* {@link COSEMac0}
*
*
*
* 18
* {@code COSE_Sign1}
* {@link COSESign1}
*
*
*
* 96
* {@code COSE_Encrypt}
* {@link COSEEncrypt}
*
*
*
* 97
* {@code COSE_Mac}
* {@link COSEMac}
*
*
*
* 98
* {@code COSE_Sign}
* {@link COSESign}
*
*
*
*
*
* @return
* The tag number.
*
* @see RFC 9052, 2. Basic COSE Structure
*/
public int getTagNumber()
{
return getType().getTagNumber();
}
/**
* Wrap this COSE message with a CBOR tag.
*
*
* This method is equivalent to "new {@link CBORTaggedItem#CBORTaggedItem(Number, CBORItem)
* CBORTaggedItem}({@link #getTagNumber()}, this)
".
*
*
* @return
* A tagged COSE message.
*/
public CBORTaggedItem getTagged()
{
return new CBORTaggedItem(getTagNumber(), this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy