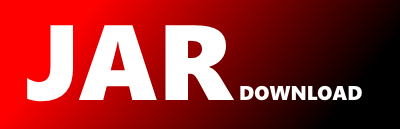
com.authlete.cose.constants.COSEAlgorithms Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cbor Show documentation
Show all versions of cbor Show documentation
A Java library for CBOR, COSE, CWT and mdoc.
The newest version!
/*
* Copyright (C) 2023 Authlete, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.authlete.cose.constants;
import java.util.LinkedHashMap;
import java.util.Map;
/**
* COSE Algorithms
*
*
*
*
*
* Name
* Value
* Description
*
*
*
* {@link #RS512}
* -259
* RSASSA-PKCS1-v1_5 using SHA-512
*
*
*
* {@link #RS384}
* -258
* RSASSA-PKCS1-v1_5 using SHA-384
*
*
*
* {@link #RS256}
* -257
* RSASSA-PKCS1-v1_5 using SHA-256
*
*
*
* {@link #ES256K}
* -47
* ECDSA using secp256k1 curve and SHA-256
*
*
*
* {@link #PS512}
* -39
* RSASSA-PSS w/ SHA-512
*
*
*
* {@link #PS384}
* -38
* RSASSA-PSS w/ SHA-384
*
*
*
* {@link #PS256}
* -37
* RSASSA-PSS w/ SHA-256
*
*
*
* {@link #ES512}
* -36
* ECDSA w/ SHA-512
*
*
*
* {@link #ES384}
* -35
* ECDSA w/ SHA-384
*
*
*
* {@link #EdDSA}
* -8
* EdDSA
*
*
*
* {@link #ES256}
* -7
* ECDSA w/ SHA-256
*
*
*
*
*
* @since 1.1
*
* @see IANA: COSE Algorithms
*/
public final class COSEAlgorithms
{
/**
* RS512 (-259); RSASSA-PKCS1-v1_5 using SHA-512
*
* @since 1.3
*/
public static final int RS512 = -259;
/**
* RS384 (-258); RSASSA-PKCS1-v1_5 using SHA-384
*
* @since 1.3
*/
public static final int RS384 = -258;
/**
* RS256 (-257); RSASSA-PKCS1-v1_5 using SHA-256
*
* @since 1.3
*/
public static final int RS256 = -257;
/**
* ES256K (-47); ECDSA using secp256k1 curve and SHA-256
*
* @since 1.3
*/
public static final int ES256K = -47;
/**
* PS512 (-39); RSASSA-PSS w/ SHA-512
*/
public static final int PS512 = -39;
/**
* PS384 (-38); RSASSA-PSS w/ SHA-384
*/
public static final int PS384 = -38;
/**
* PS256 (-37); RSASSA-PSS w/ SHA-256
*/
public static final int PS256 = -37;
/**
* ES512 (-36); ECDSA w/ SHA-512
*/
public static final int ES512 = -36;
/**
* ES384 (-35); ECDSA w/ SHA-384
*/
public static final int ES384 = -35;
/**
* EdDSA (-8); EdDSA
*/
public static final int EdDSA = -8;
/**
* ES256 (-7); ECDSA w/ SHA-256
*/
public static final int ES256 = -7;
private static final int[] values = {
RS512, RS384, RS256, ES256K,
PS512, PS384, PS256, ES512, ES384, EdDSA, ES256,
};
private static final String[] names = {
"RS512", "RS384", "RS256", "ES256K",
"PS512", "PS384", "PS256", "ES512", "ES384", "EdDSA", "ES256",
};
private static final Map valueToNameMap = createValueToNameMap();
private static final Map nameToValueMap = createNameToValueMap();
private static Map createValueToNameMap()
{
Map map = new LinkedHashMap<>();
for (int i = 0; i < values.length; i++)
{
map.put(values[i], names[i]);
}
return map;
}
private static Map createNameToValueMap()
{
Map map = new LinkedHashMap<>();
for (int i = 0; i < names.length; i++)
{
map.put(names[i], values[i]);
}
return map;
}
/**
* Get the integer identifier assigned to the algorithm.
*
* @param name
* An algorithm name such as {@code "ES256"}.
* If {@code null} is given, 0 is returned.
*
* @return
* The integer identifier assigned to the algorithm.
* If the given name is not recognized, 0 is returned.
*/
public static int getValueByName(String name)
{
if (name == null)
{
return 0;
}
return nameToValueMap.getOrDefault(name, 0);
}
/**
* Get the name of the algorithm to which the integer identifier
* has been assigned to.
*
* @param value
* An integer identifier assigned to an algorithm.
*
* @return
* The name of the algorithm to which the integer identifier
* has been assigned to. If the given identifier is not
* recognized, {@code null} is returned.
*/
public static String getNameByValue(int value)
{
return valueToNameMap.getOrDefault(value, null);
}
private COSEAlgorithms()
{
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy