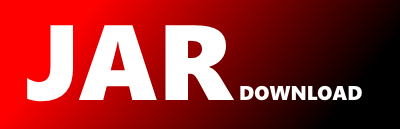
com.automationrockstars.gir.data.impl.SimpleTestDataService Maven / Gradle / Ivy
The newest version!
/*
*
*/
package com.automationrockstars.gir.data.impl;
import com.automationrockstars.gir.data.TestData;
import com.automationrockstars.gir.data.TestDataRecord;
import com.automationrockstars.gir.data.TestDataService;
import com.google.common.collect.Maps;
import com.google.common.io.Files;
import com.google.gson.GsonBuilder;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.Charset;
import java.nio.file.Paths;
import java.util.List;
import java.util.Map;
public class SimpleTestDataService implements TestDataService {
public static final String NAME = "DEFAULT";
private static final Logger LOG = LoggerFactory.getLogger(SimpleTestDataService.class);
private final Map, TestData extends TestDataRecord>> sharedTestDataCache = Maps.newConcurrentMap();
//cache disabled
// is sharing data disabled as well?
private final Map, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy