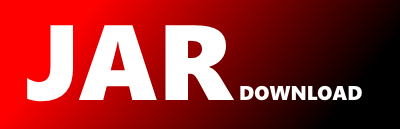
com.automationrockstars.design.gir.webdriver.WebCache Maven / Gradle / Ivy
package com.automationrockstars.design.gir.webdriver;
import java.lang.ref.SoftReference;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import org.openqa.selenium.By;
import org.openqa.selenium.SearchContext;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebDriverException;
import org.openqa.selenium.WebElement;
import com.automationrockstars.base.ConfigLoader;
import com.automationrockstars.design.gir.webdriver.plugin.UiDriverPlugin;
import com.automationrockstars.design.gir.webdriver.plugin.UiDriverPluginService;
import com.google.common.base.Predicate;
import com.google.common.collect.FluentIterable;
import com.google.common.collect.Maps;
public class WebCache {
private final static CacheCleaner cleaner = new CacheCleaner();
static {
UiDriverPluginService.registerPlugin(cleaner);
}
static class CacheCleaner implements UiDriverPlugin{
@Override
public void beforeInstantiateDriver() {
}
@Override
public void beforeGetDriver() {
}
@Override
public void afterGetDriver(WebDriver driver) {
}
@Override
public void beforeCloseDriver(WebDriver driver) {
}
@Override
public void afterCloseDriver() {
webCache.remove();
}
@Override
public void afterInstantiateDriver(WebDriver driver) {
}
}
private static final ThreadLocal
© 2015 - 2025 Weber Informatics LLC | Privacy Policy