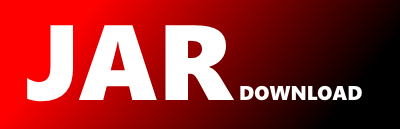
com.autonomousapps.internal.utils.document.document.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dependency-analysis-gradle-plugin Show documentation
Show all versions of dependency-analysis-gradle-plugin Show documentation
Analyzes dependency usage in Android and JVM projects
// Copyright (c) 2024. Tony Robalik.
// SPDX-License-Identifier: Apache-2.0
package com.autonomousapps.internal.utils.document
import org.w3c.dom.*
import kotlin.collections.ArrayList
import kotlin.collections.HashSet
import kotlin.collections.Iterable
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.collections.Set
import kotlin.collections.associateBy
import kotlin.collections.filter
import kotlin.collections.filterIsInstance
import kotlin.collections.map
internal fun Document.attrs(): List> {
return getElementsByTagName("*")
.map { it.attributes }
// this flatMap looks redundant but isn't!
.flatMap { it }
.filterIsInstance()
.map { it.name to it.value }
}
internal fun Document.contentReferences(): Map {
return getElementsByTagName("*")
.map { it.textContent }
.filter { it.startsWith('@') }
// placeholder value; meaningless.
.associateBy { "DIRECT-REFERENCE" }
}
internal fun NamedNodeMap.map(transform: (Node) -> R): List {
val destination = ArrayList()
for (i in 0 until length) {
destination.add(transform(item(i)))
}
return destination
}
internal fun Iterable.flatMap(transform: (Node) -> R): List {
val destination = ArrayList()
for (it in this) {
for (i in 0 until it.length) {
destination.add(transform(it.item(i)))
}
}
return destination
}
internal inline fun NodeList.mapNotNull(transform: (Node) -> R?): List {
val destination = ArrayList(length)
for (i in 0 until length) {
transform(item(i))?.let { destination.add(it) }
}
return destination
}
internal inline fun NodeList.map(transform: (Node) -> R): List {
val destination = ArrayList(length)
for (i in 0 until length) {
destination.add(transform(item(i)))
}
return destination
}
internal inline fun NodeList.mapToSet(transform: (Node) -> R): Set {
val destination = HashSet(length)
for (i in 0 until length) {
destination.add(transform(item(i)))
}
return destination
}
internal inline fun NodeList.filter(predicate: (Node) -> Boolean): List {
val destination = ArrayList(length)
for (i in 0 until length) {
if (predicate(item(i))) destination.add(item(i))
}
return destination
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy