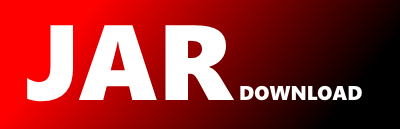
com.autonomousapps.extension.IssueHandler.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dependency-analysis-gradle-plugin Show documentation
Show all versions of dependency-analysis-gradle-plugin Show documentation
Analyzes dependency usage in Android and JVM projects
// Copyright (c) 2024. Tony Robalik.
// SPDX-License-Identifier: Apache-2.0
package com.autonomousapps.extension
import com.autonomousapps.services.GlobalDslService
import org.gradle.api.Action
import org.gradle.api.provider.Provider
import javax.inject.Inject
/**
* Defers to `all` and `projects` for customization behavior.
*
* ```
* dependencyAnalysis {
* issues {
* all { ... }
* project(':lib') { ... }
* }
* }
* ```
*/
abstract class IssueHandler @Inject constructor(
globalDslService: Provider,
) {
private val globalDslService = globalDslService.get()
fun all(action: Action) {
globalDslService.all(action)
}
fun project(projectPath: String, action: Action) {
globalDslService.project(projectPath, action)
}
internal fun shouldAnalyzeSourceSet(sourceSetName: String, projectPath: String): Boolean {
return globalDslService.shouldAnalyzeSourceSet(sourceSetName, projectPath)
}
internal fun anyIssueFor(projectPath: String): List> {
return globalDslService.anyIssueFor(projectPath)
}
internal fun unusedDependenciesIssueFor(projectPath: String): List> {
return globalDslService.unusedDependenciesIssueFor(projectPath)
}
internal fun usedTransitiveDependenciesIssueFor(projectPath: String): List> {
return globalDslService.usedTransitiveDependenciesIssueFor(projectPath)
}
internal fun incorrectConfigurationIssueFor(projectPath: String): List> {
return globalDslService.incorrectConfigurationIssueFor(projectPath)
}
internal fun compileOnlyIssueFor(projectPath: String): List> {
return globalDslService.compileOnlyIssueFor(projectPath)
}
internal fun runtimeOnlyIssueFor(projectPath: String): List> {
return globalDslService.runtimeOnlyIssueFor(projectPath)
}
internal fun unusedAnnotationProcessorsIssueFor(projectPath: String): List> {
return globalDslService.unusedAnnotationProcessorsIssueFor(projectPath)
}
internal fun redundantPluginsIssueFor(projectPath: String): Provider {
return globalDslService.redundantPluginsIssueFor(projectPath)
}
internal fun moduleStructureIssueFor(projectPath: String): Provider {
return globalDslService.moduleStructureIssueFor(projectPath)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy