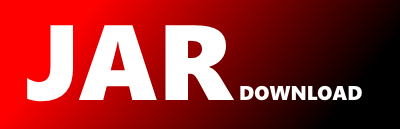
com.autonomousapps.internal.utils.utils.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dependency-analysis-gradle-plugin Show documentation
Show all versions of dependency-analysis-gradle-plugin Show documentation
Analyzes dependency usage in Android and JVM projects
// Copyright (c) 2024. Tony Robalik.
// SPDX-License-Identifier: Apache-2.0
@file:Suppress("UnstableApiUsage")
package com.autonomousapps.internal.utils
import okio.BufferedSource
import okio.buffer
import okio.source
import org.gradle.api.artifacts.result.DependencyResult
import org.gradle.api.artifacts.result.ResolvedDependencyResult
import org.gradle.api.file.RegularFile
import org.gradle.api.file.RegularFileProperty
import org.gradle.api.provider.Provider
import java.io.File
import java.util.*
/**
* Resolves the file from the property and deletes its contents, then returns the file.
*/
internal fun RegularFileProperty.getAndDelete(): File {
val file = get().asFile
file.delete()
return file
}
/**
* Resolves the file from the provider and deletes its contents, then returns the file.
*/
internal fun Provider.getAndDelete(): File {
val file = get().asFile
file.delete()
return file
}
/**
* Buffer reads of the nullable RegularFileProperty from disk to the set.
*/
internal inline fun RegularFileProperty.fromNullableJsonSet(): Set {
return orNull?.fromJsonSet() ?: emptySet()
}
/**
* Buffers reads of the RegularFileProperty from disk to the set.
*/
internal inline fun RegularFileProperty.fromJsonSet(): Set = get().fromJsonSet()
/**
* Buffers reads of the RegularFile from disk to the set.
*/
internal inline fun RegularFile.fromJsonSet(): Set {
asFile.bufferRead().use { reader ->
return getJsonSetAdapter().fromJson(reader)!!
}
}
/**
* Buffers reads of the RegularFileProperty from disk to the set.
*/
internal inline fun RegularFileProperty.fromJsonList(): List = get().fromJsonList()
/**
* Buffers reads of the RegularFile from disk to the set.
*/
internal inline fun RegularFile.fromJsonList(): List {
asFile.bufferRead().use { reader ->
return getJsonListAdapter().fromJson(reader)!!
}
}
internal inline fun RegularFileProperty.fromJsonMapList(): Map> {
return get().fromJsonMapList()
}
internal inline fun RegularFileProperty.fromJsonMapSet(): Map> {
return get().fromJsonMapSet()
}
internal inline fun RegularFile.fromJsonMapList(): Map> {
return asFile.fromJsonMapList()
}
internal inline fun RegularFile.fromJsonMapSet(): Map> {
return asFile.fromJsonMapSet()
}
internal inline fun File.fromJsonMapList(): Map> {
return bufferRead().fromJsonMapList()
}
internal inline fun File.fromJsonMapSet(): Map> {
return bufferRead().fromJsonMapSet()
}
/**
* Buffers reads of the RegularFileProperty from disk to the set.
*/
internal inline fun RegularFileProperty.fromJsonMap(): Map = get().fromJsonMap()
/**
* Buffers reads of the RegularFile from disk to the set.
*/
internal inline fun RegularFile.fromJsonMap(): Map = asFile.fromJsonMap()
/**
* Buffers reads of the File from disk to the set.
*/
internal inline fun File.fromJsonMap(): Map {
bufferRead().use { reader ->
return getJsonMapAdapter().fromJson(reader)!!
}
}
/**
* Buffer reads of the RegularFileProperty from disk to the set.
*/
internal inline fun RegularFileProperty.fromJson(): T = get().fromJson()
/**
* Buffer reads of the RegularFile from disk to the set.
*/
internal inline fun RegularFile.fromJson(): T = asFile.fromJson()
/**
* Buffer reads of the File from disk to the set.
*/
internal inline fun File.fromJson(): T {
bufferRead().use { reader ->
return getJsonAdapter().fromJson(reader)!!
}
}
internal fun RegularFileProperty.readLines(): List = get().readLines()
internal fun RegularFile.readLines(): List = asFile.readLines()
internal fun RegularFileProperty.readText(): String = get().asFile.readText()
private fun File.bufferRead(): BufferedSource = source().buffer()
// copied from StringsJVM.kt
internal fun String.capitalizeSafely(locale: Locale = Locale.ROOT): String {
if (isNotEmpty()) {
val firstChar = this[0]
if (firstChar.isLowerCase()) {
return buildString {
val titleChar = firstChar.titlecaseChar()
if (titleChar != firstChar.uppercaseChar()) {
append(titleChar)
} else {
append([email protected](0, 1).uppercase(locale))
}
append([email protected](1))
}
}
}
return this
}
// copied from StringsJVM.kt
@Suppress("PLATFORM_CLASS_MAPPED_TO_KOTLIN")
internal fun String.lowercase(): String = (this as java.lang.String).toLowerCase(Locale.ROOT)
// Print dependency tree (like running the `dependencies` task).
@Suppress("unused")
internal fun printDependencyTree(dependencies: Set, level: Int = 0) {
dependencies.filterIsInstance().forEach { result ->
val resolvedComponentResult = result.selected
println("${" ".repeat(level)}- ${resolvedComponentResult.id}")
printDependencyTree(resolvedComponentResult.dependencies, level + 1)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy