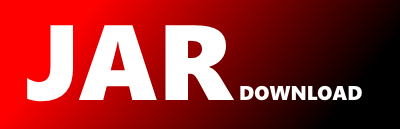
com.autonomouslogic.commons.rxjava3.internal.ZipAll Maven / Gradle / Ivy
package com.autonomouslogic.commons.rxjava3.internal;
import io.reactivex.rxjava3.core.Flowable;
import io.reactivex.rxjava3.functions.Function;
import io.reactivex.rxjava3.functions.Predicate;
import java.util.Optional;
import lombok.NonNull;
import lombok.RequiredArgsConstructor;
import org.reactivestreams.Publisher;
@RequiredArgsConstructor
@SuppressWarnings("unchecked")
public class ZipAll {
private static final Predicate> PREDICATE = in -> {
var objs = (Object[]) in;
for (var obj : objs) {
if (((Optional) obj).isPresent()) {
return true;
}
}
return false;
};
private static final Function
© 2015 - 2024 Weber Informatics LLC | Privacy Policy