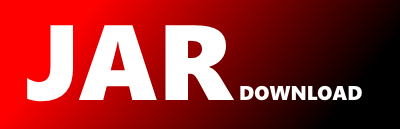
com.autocheckinsurance.sdk.model.HistoryRecord Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aci-java Show documentation
Show all versions of aci-java Show documentation
AutoCheck Insurance API Java Client
The newest version!
/*
* ACI Services API
* API for methods pertaining to all ACI services
*
* OpenAPI spec version: 3.0.2
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.autocheckinsurance.sdk.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.time.LocalDate;
/**
* historical activity for the vehicle
*/
@ApiModel(description = "historical activity for the vehicle")
public class HistoryRecord {
@SerializedName("accidentSequenceNumber")
private String accidentSequenceNumber = null;
@SerializedName("activityCode")
private Integer activityCode = null;
@SerializedName("caseNumber")
private String caseNumber = null;
@SerializedName("category")
private String category = null;
@SerializedName("checklistGroup")
private Integer checklistGroup = null;
@SerializedName("city")
private String city = null;
@SerializedName("color")
private String color = null;
@SerializedName("date")
private LocalDate date = null;
@SerializedName("lease")
private Boolean lease = null;
@SerializedName("lien")
private Boolean lien = null;
@SerializedName("odometer")
private Integer odometer = null;
@SerializedName("orgName")
private String orgName = null;
@SerializedName("originalIncidentReportedDate")
private LocalDate originalIncidentReportedDate = null;
/**
* odometer rollback use indicator of how odometer reading is used in rollback calculation
*/
@JsonAdapter(OruEnum.Adapter.class)
public enum OruEnum {
H("H"),
N("N"),
R("R");
private String value;
OruEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static OruEnum fromValue(String text) {
for (OruEnum b : OruEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final OruEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public OruEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return OruEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("oru")
private OruEnum oru = null;
@SerializedName("phone")
private String phone = null;
@SerializedName("recallCode")
private String recallCode = null;
@SerializedName("recallLinkTxt")
private String recallLinkTxt = null;
@SerializedName("recallTxt")
private String recallTxt = null;
@SerializedName("recallUrl")
private String recallUrl = null;
@SerializedName("rollback")
private Boolean rollback = null;
@SerializedName("state")
private String state = null;
@SerializedName("title")
private String title = null;
@SerializedName("titleRegStorm")
private Boolean titleRegStorm = null;
@SerializedName("type")
private String type = null;
/**
* the odometer unit of measurement
*/
@JsonAdapter(UomEnum.Adapter.class)
public enum UomEnum {
M("M"),
K("K"),
U("U");
private String value;
UomEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static UomEnum fromValue(String text) {
for (UomEnum b : UomEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final UomEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public UomEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return UomEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("uom")
private UomEnum uom = null;
@SerializedName("url")
private String url = null;
@SerializedName("urlLink")
private Boolean urlLink = null;
public HistoryRecord accidentSequenceNumber(String accidentSequenceNumber) {
this.accidentSequenceNumber = accidentSequenceNumber;
return this;
}
/**
* the accident sequence number
* @return accidentSequenceNumber
**/
@ApiModelProperty(value = "the accident sequence number")
public String getAccidentSequenceNumber() {
return accidentSequenceNumber;
}
public void setAccidentSequenceNumber(String accidentSequenceNumber) {
this.accidentSequenceNumber = accidentSequenceNumber;
}
public HistoryRecord activityCode(Integer activityCode) {
this.activityCode = activityCode;
return this;
}
/**
* the unique identifier for the activity type
* @return activityCode
**/
@ApiModelProperty(value = "the unique identifier for the activity type")
public Integer getActivityCode() {
return activityCode;
}
public void setActivityCode(Integer activityCode) {
this.activityCode = activityCode;
}
public HistoryRecord caseNumber(String caseNumber) {
this.caseNumber = caseNumber;
return this;
}
/**
* the case number
* @return caseNumber
**/
@ApiModelProperty(value = "the case number")
public String getCaseNumber() {
return caseNumber;
}
public void setCaseNumber(String caseNumber) {
this.caseNumber = caseNumber;
}
public HistoryRecord category(String category) {
this.category = category;
return this;
}
/**
* the activity category
* @return category
**/
@ApiModelProperty(value = "the activity category")
public String getCategory() {
return category;
}
public void setCategory(String category) {
this.category = category;
}
public HistoryRecord checklistGroup(Integer checklistGroup) {
this.checklistGroup = checklistGroup;
return this;
}
/**
* the checklist group
* @return checklistGroup
**/
@ApiModelProperty(value = "the checklist group")
public Integer getChecklistGroup() {
return checklistGroup;
}
public void setChecklistGroup(Integer checklistGroup) {
this.checklistGroup = checklistGroup;
}
public HistoryRecord city(String city) {
this.city = city;
return this;
}
/**
* the city in which the activity was reported
* @return city
**/
@ApiModelProperty(value = "the city in which the activity was reported")
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public HistoryRecord color(String color) {
this.color = color;
return this;
}
/**
* the color of a vehicle supplied by dealer
* @return color
**/
@ApiModelProperty(value = "the color of a vehicle supplied by dealer")
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public HistoryRecord date(LocalDate date) {
this.date = date;
return this;
}
/**
* the date of the activity
* @return date
**/
@ApiModelProperty(value = "the date of the activity")
public LocalDate getDate() {
return date;
}
public void setDate(LocalDate date) {
this.date = date;
}
public HistoryRecord lease(Boolean lease) {
this.lease = lease;
return this;
}
/**
* indicates if this is a lease record
* @return lease
**/
@ApiModelProperty(value = "indicates if this is a lease record")
public Boolean isLease() {
return lease;
}
public void setLease(Boolean lease) {
this.lease = lease;
}
public HistoryRecord lien(Boolean lien) {
this.lien = lien;
return this;
}
/**
* indicates if this is a lein record
* @return lien
**/
@ApiModelProperty(value = "indicates if this is a lein record")
public Boolean isLien() {
return lien;
}
public void setLien(Boolean lien) {
this.lien = lien;
}
public HistoryRecord odometer(Integer odometer) {
this.odometer = odometer;
return this;
}
/**
* the odometer reading
* @return odometer
**/
@ApiModelProperty(value = "the odometer reading")
public Integer getOdometer() {
return odometer;
}
public void setOdometer(Integer odometer) {
this.odometer = odometer;
}
public HistoryRecord orgName(String orgName) {
this.orgName = orgName;
return this;
}
/**
* the name of the reporting organization
* @return orgName
**/
@ApiModelProperty(value = "the name of the reporting organization")
public String getOrgName() {
return orgName;
}
public void setOrgName(String orgName) {
this.orgName = orgName;
}
public HistoryRecord originalIncidentReportedDate(LocalDate originalIncidentReportedDate) {
this.originalIncidentReportedDate = originalIncidentReportedDate;
return this;
}
/**
* the date of the incident was reported
* @return originalIncidentReportedDate
**/
@ApiModelProperty(value = "the date of the incident was reported")
public LocalDate getOriginalIncidentReportedDate() {
return originalIncidentReportedDate;
}
public void setOriginalIncidentReportedDate(LocalDate originalIncidentReportedDate) {
this.originalIncidentReportedDate = originalIncidentReportedDate;
}
public HistoryRecord oru(OruEnum oru) {
this.oru = oru;
return this;
}
/**
* odometer rollback use indicator of how odometer reading is used in rollback calculation
* @return oru
**/
@ApiModelProperty(value = "odometer rollback use indicator of how odometer reading is used in rollback calculation")
public OruEnum getOru() {
return oru;
}
public void setOru(OruEnum oru) {
this.oru = oru;
}
public HistoryRecord phone(String phone) {
this.phone = phone;
return this;
}
/**
* the phone of the reporting organization
* @return phone
**/
@ApiModelProperty(value = "the phone of the reporting organization")
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public HistoryRecord recallCode(String recallCode) {
this.recallCode = recallCode;
return this;
}
/**
* recall code supplied by manufacturer
* @return recallCode
**/
@ApiModelProperty(value = "recall code supplied by manufacturer")
public String getRecallCode() {
return recallCode;
}
public void setRecallCode(String recallCode) {
this.recallCode = recallCode;
}
public HistoryRecord recallLinkTxt(String recallLinkTxt) {
this.recallLinkTxt = recallLinkTxt;
return this;
}
/**
* used in URL text
* @return recallLinkTxt
**/
@ApiModelProperty(value = "used in URL text")
public String getRecallLinkTxt() {
return recallLinkTxt;
}
public void setRecallLinkTxt(String recallLinkTxt) {
this.recallLinkTxt = recallLinkTxt;
}
public HistoryRecord recallTxt(String recallTxt) {
this.recallTxt = recallTxt;
return this;
}
/**
* description of recall
* @return recallTxt
**/
@ApiModelProperty(value = "description of recall")
public String getRecallTxt() {
return recallTxt;
}
public void setRecallTxt(String recallTxt) {
this.recallTxt = recallTxt;
}
public HistoryRecord recallUrl(String recallUrl) {
this.recallUrl = recallUrl;
return this;
}
/**
* the URL is supplied by the manufacturer
* @return recallUrl
**/
@ApiModelProperty(value = "the URL is supplied by the manufacturer")
public String getRecallUrl() {
return recallUrl;
}
public void setRecallUrl(String recallUrl) {
this.recallUrl = recallUrl;
}
public HistoryRecord rollback(Boolean rollback) {
this.rollback = rollback;
return this;
}
/**
* indicates if this is a rollback record
* @return rollback
**/
@ApiModelProperty(value = "indicates if this is a rollback record")
public Boolean isRollback() {
return rollback;
}
public void setRollback(Boolean rollback) {
this.rollback = rollback;
}
public HistoryRecord state(String state) {
this.state = state;
return this;
}
/**
* the state in which the activity occurred
* @return state
**/
@ApiModelProperty(value = "the state in which the activity occurred")
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
public HistoryRecord title(String title) {
this.title = title;
return this;
}
/**
* the title record
* @return title
**/
@ApiModelProperty(value = "the title record")
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public HistoryRecord titleRegStorm(Boolean titleRegStorm) {
this.titleRegStorm = titleRegStorm;
return this;
}
/**
* indicates there has been a title or registration event in a major storm area sometime prior to the storm
* @return titleRegStorm
**/
@ApiModelProperty(value = "indicates there has been a title or registration event in a major storm area sometime prior to the storm")
public Boolean isTitleRegStorm() {
return titleRegStorm;
}
public void setTitleRegStorm(Boolean titleRegStorm) {
this.titleRegStorm = titleRegStorm;
}
public HistoryRecord type(String type) {
this.type = type;
return this;
}
/**
* the type of activity
* @return type
**/
@ApiModelProperty(value = "the type of activity")
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public HistoryRecord uom(UomEnum uom) {
this.uom = uom;
return this;
}
/**
* the odometer unit of measurement
* @return uom
**/
@ApiModelProperty(value = "the odometer unit of measurement")
public UomEnum getUom() {
return uom;
}
public void setUom(UomEnum uom) {
this.uom = uom;
}
public HistoryRecord url(String url) {
this.url = url;
return this;
}
/**
* the URL supplied by a dealer
* @return url
**/
@ApiModelProperty(value = "the URL supplied by a dealer")
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public HistoryRecord urlLink(Boolean urlLink) {
this.urlLink = urlLink;
return this;
}
/**
* indicates whether or not the url needs to display as a hyperlink in the history section of the report
* @return urlLink
**/
@ApiModelProperty(value = "indicates whether or not the url needs to display as a hyperlink in the history section of the report")
public Boolean isUrlLink() {
return urlLink;
}
public void setUrlLink(Boolean urlLink) {
this.urlLink = urlLink;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
HistoryRecord historyRecord = (HistoryRecord) o;
return Objects.equals(this.accidentSequenceNumber, historyRecord.accidentSequenceNumber) &&
Objects.equals(this.activityCode, historyRecord.activityCode) &&
Objects.equals(this.caseNumber, historyRecord.caseNumber) &&
Objects.equals(this.category, historyRecord.category) &&
Objects.equals(this.checklistGroup, historyRecord.checklistGroup) &&
Objects.equals(this.city, historyRecord.city) &&
Objects.equals(this.color, historyRecord.color) &&
Objects.equals(this.date, historyRecord.date) &&
Objects.equals(this.lease, historyRecord.lease) &&
Objects.equals(this.lien, historyRecord.lien) &&
Objects.equals(this.odometer, historyRecord.odometer) &&
Objects.equals(this.orgName, historyRecord.orgName) &&
Objects.equals(this.originalIncidentReportedDate, historyRecord.originalIncidentReportedDate) &&
Objects.equals(this.oru, historyRecord.oru) &&
Objects.equals(this.phone, historyRecord.phone) &&
Objects.equals(this.recallCode, historyRecord.recallCode) &&
Objects.equals(this.recallLinkTxt, historyRecord.recallLinkTxt) &&
Objects.equals(this.recallTxt, historyRecord.recallTxt) &&
Objects.equals(this.recallUrl, historyRecord.recallUrl) &&
Objects.equals(this.rollback, historyRecord.rollback) &&
Objects.equals(this.state, historyRecord.state) &&
Objects.equals(this.title, historyRecord.title) &&
Objects.equals(this.titleRegStorm, historyRecord.titleRegStorm) &&
Objects.equals(this.type, historyRecord.type) &&
Objects.equals(this.uom, historyRecord.uom) &&
Objects.equals(this.url, historyRecord.url) &&
Objects.equals(this.urlLink, historyRecord.urlLink);
}
@Override
public int hashCode() {
return Objects.hash(accidentSequenceNumber, activityCode, caseNumber, category, checklistGroup, city, color, date, lease, lien, odometer, orgName, originalIncidentReportedDate, oru, phone, recallCode, recallLinkTxt, recallTxt, recallUrl, rollback, state, title, titleRegStorm, type, uom, url, urlLink);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class HistoryRecord {\n");
sb.append(" accidentSequenceNumber: ").append(toIndentedString(accidentSequenceNumber)).append("\n");
sb.append(" activityCode: ").append(toIndentedString(activityCode)).append("\n");
sb.append(" caseNumber: ").append(toIndentedString(caseNumber)).append("\n");
sb.append(" category: ").append(toIndentedString(category)).append("\n");
sb.append(" checklistGroup: ").append(toIndentedString(checklistGroup)).append("\n");
sb.append(" city: ").append(toIndentedString(city)).append("\n");
sb.append(" color: ").append(toIndentedString(color)).append("\n");
sb.append(" date: ").append(toIndentedString(date)).append("\n");
sb.append(" lease: ").append(toIndentedString(lease)).append("\n");
sb.append(" lien: ").append(toIndentedString(lien)).append("\n");
sb.append(" odometer: ").append(toIndentedString(odometer)).append("\n");
sb.append(" orgName: ").append(toIndentedString(orgName)).append("\n");
sb.append(" originalIncidentReportedDate: ").append(toIndentedString(originalIncidentReportedDate)).append("\n");
sb.append(" oru: ").append(toIndentedString(oru)).append("\n");
sb.append(" phone: ").append(toIndentedString(phone)).append("\n");
sb.append(" recallCode: ").append(toIndentedString(recallCode)).append("\n");
sb.append(" recallLinkTxt: ").append(toIndentedString(recallLinkTxt)).append("\n");
sb.append(" recallTxt: ").append(toIndentedString(recallTxt)).append("\n");
sb.append(" recallUrl: ").append(toIndentedString(recallUrl)).append("\n");
sb.append(" rollback: ").append(toIndentedString(rollback)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" title: ").append(toIndentedString(title)).append("\n");
sb.append(" titleRegStorm: ").append(toIndentedString(titleRegStorm)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" uom: ").append(toIndentedString(uom)).append("\n");
sb.append(" url: ").append(toIndentedString(url)).append("\n");
sb.append(" urlLink: ").append(toIndentedString(urlLink)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy