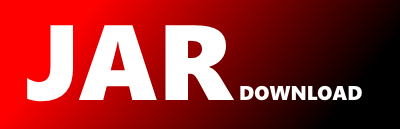
com.autocheckinsurance.sdk.model.RecallRecord Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aci-java Show documentation
Show all versions of aci-java Show documentation
AutoCheck Insurance API Java Client
The newest version!
/*
* ACI Services API
* API for methods pertaining to all ACI services
*
* OpenAPI spec version: 3.0.2
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.autocheckinsurance.sdk.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.time.LocalDate;
/**
* recall information for the vehicle
*/
@ApiModel(description = "recall information for the vehicle")
public class RecallRecord {
@SerializedName("consequence")
private Boolean consequence = null;
@SerializedName("date")
private LocalDate date = null;
@SerializedName("nhtsaCampaignNumber")
private String nhtsaCampaignNumber = null;
@SerializedName("remedy")
private String remedy = null;
@SerializedName("status")
private String status = null;
@SerializedName("summary")
private String summary = null;
@SerializedName("title")
private String title = null;
public RecallRecord consequence(Boolean consequence) {
this.consequence = consequence;
return this;
}
/**
* the consequence of the recall
* @return consequence
**/
@ApiModelProperty(value = "the consequence of the recall")
public Boolean isConsequence() {
return consequence;
}
public void setConsequence(Boolean consequence) {
this.consequence = consequence;
}
public RecallRecord date(LocalDate date) {
this.date = date;
return this;
}
/**
* the date of the recall
* @return date
**/
@ApiModelProperty(value = "the date of the recall")
public LocalDate getDate() {
return date;
}
public void setDate(LocalDate date) {
this.date = date;
}
public RecallRecord nhtsaCampaignNumber(String nhtsaCampaignNumber) {
this.nhtsaCampaignNumber = nhtsaCampaignNumber;
return this;
}
/**
* the NHTSA compaign number
* @return nhtsaCampaignNumber
**/
@ApiModelProperty(value = "the NHTSA compaign number")
public String getNhtsaCampaignNumber() {
return nhtsaCampaignNumber;
}
public void setNhtsaCampaignNumber(String nhtsaCampaignNumber) {
this.nhtsaCampaignNumber = nhtsaCampaignNumber;
}
public RecallRecord remedy(String remedy) {
this.remedy = remedy;
return this;
}
/**
* the remedy for the recall
* @return remedy
**/
@ApiModelProperty(value = "the remedy for the recall")
public String getRemedy() {
return remedy;
}
public void setRemedy(String remedy) {
this.remedy = remedy;
}
public RecallRecord status(String status) {
this.status = status;
return this;
}
/**
* the status of the recall
* @return status
**/
@ApiModelProperty(value = "the status of the recall")
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public RecallRecord summary(String summary) {
this.summary = summary;
return this;
}
/**
* the recall summary
* @return summary
**/
@ApiModelProperty(value = "the recall summary")
public String getSummary() {
return summary;
}
public void setSummary(String summary) {
this.summary = summary;
}
public RecallRecord title(String title) {
this.title = title;
return this;
}
/**
* the recall title
* @return title
**/
@ApiModelProperty(value = "the recall title")
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
RecallRecord recallRecord = (RecallRecord) o;
return Objects.equals(this.consequence, recallRecord.consequence) &&
Objects.equals(this.date, recallRecord.date) &&
Objects.equals(this.nhtsaCampaignNumber, recallRecord.nhtsaCampaignNumber) &&
Objects.equals(this.remedy, recallRecord.remedy) &&
Objects.equals(this.status, recallRecord.status) &&
Objects.equals(this.summary, recallRecord.summary) &&
Objects.equals(this.title, recallRecord.title);
}
@Override
public int hashCode() {
return Objects.hash(consequence, date, nhtsaCampaignNumber, remedy, status, summary, title);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class RecallRecord {\n");
sb.append(" consequence: ").append(toIndentedString(consequence)).append("\n");
sb.append(" date: ").append(toIndentedString(date)).append("\n");
sb.append(" nhtsaCampaignNumber: ").append(toIndentedString(nhtsaCampaignNumber)).append("\n");
sb.append(" remedy: ").append(toIndentedString(remedy)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" summary: ").append(toIndentedString(summary)).append("\n");
sb.append(" title: ").append(toIndentedString(title)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy