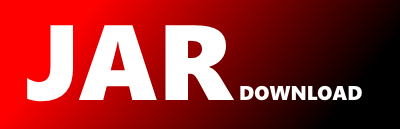
com.autocheckinsurance.sdk.model.VehicleHistory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aci-java Show documentation
Show all versions of aci-java Show documentation
AutoCheck Insurance API Java Client
The newest version!
/*
* ACI Services API
* API for methods pertaining to all ACI services
*
* OpenAPI spec version: 3.0.2
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.autocheckinsurance.sdk.model;
import java.util.Objects;
import java.util.Arrays;
import com.autocheckinsurance.sdk.model.HistoryRecord;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* the historical information for a vehicle
*/
@ApiModel(description = "the historical information for a vehicle")
public class VehicleHistory {
@SerializedName("accidentCount")
private Integer accidentCount = null;
@SerializedName("assured")
private Boolean assured = null;
/**
* Decides the BBP badge to be displayed on report. This attribute will not be displayed if the Assured feature is not paid for or if the VIN has no history
*/
@JsonAdapter(BbpEnum.Adapter.class)
public enum BbpEnum {
_0("0"),
_1("1"),
_3("3");
private String value;
BbpEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static BbpEnum fromValue(String text) {
for (BbpEnum b : BbpEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final BbpEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public BbpEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return BbpEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("bbp")
private BbpEnum bbp = null;
@SerializedName("conditionRpt")
private Boolean conditionRpt = null;
@SerializedName("count")
private Integer count = null;
@SerializedName("estimatedAverageMiles")
private Integer estimatedAverageMiles = null;
@SerializedName("historyRecords")
private java.util.List historyRecords = null;
@SerializedName("lastOdometer")
private Integer lastOdometer = null;
@SerializedName("mixedOdometer")
private Boolean mixedOdometer = null;
@SerializedName("odometer")
private Boolean odometer = null;
@SerializedName("recallCount")
private Integer recallCount = null;
@SerializedName("recallDataAvailable")
private Boolean recallDataAvailable = null;
@SerializedName("rollback")
private Boolean rollback = null;
public VehicleHistory accidentCount(Integer accidentCount) {
this.accidentCount = accidentCount;
return this;
}
/**
* the number of accidents
* @return accidentCount
**/
@ApiModelProperty(value = "the number of accidents")
public Integer getAccidentCount() {
return accidentCount;
}
public void setAccidentCount(Integer accidentCount) {
this.accidentCount = accidentCount;
}
public VehicleHistory assured(Boolean assured) {
this.assured = assured;
return this;
}
/**
* if the vehicle is considered to be AutoCheck Assured or not. If this feature is not paid for or no data is available, the attribute will not be displayed via the xml
* @return assured
**/
@ApiModelProperty(value = "if the vehicle is considered to be AutoCheck Assured or not. If this feature is not paid for or no data is available, the attribute will not be displayed via the xml")
public Boolean isAssured() {
return assured;
}
public void setAssured(Boolean assured) {
this.assured = assured;
}
public VehicleHistory bbp(BbpEnum bbp) {
this.bbp = bbp;
return this;
}
/**
* Decides the BBP badge to be displayed on report. This attribute will not be displayed if the Assured feature is not paid for or if the VIN has no history
* @return bbp
**/
@ApiModelProperty(value = "Decides the BBP badge to be displayed on report. This attribute will not be displayed if the Assured feature is not paid for or if the VIN has no history")
public BbpEnum getBbp() {
return bbp;
}
public void setBbp(BbpEnum bbp) {
this.bbp = bbp;
}
public VehicleHistory conditionRpt(Boolean conditionRpt) {
this.conditionRpt = conditionRpt;
return this;
}
/**
* if a condition report is available for a vehicle. If this feature is not paid for or no data is available, the attribute will not be displayed via the xml. When a condition report is available for the vehicle then the Condition report URL will be displayed in the history HDATA event section
* @return conditionRpt
**/
@ApiModelProperty(value = "if a condition report is available for a vehicle. If this feature is not paid for or no data is available, the attribute will not be displayed via the xml. When a condition report is available for the vehicle then the Condition report URL will be displayed in the history HDATA event section")
public Boolean isConditionRpt() {
return conditionRpt;
}
public void setConditionRpt(Boolean conditionRpt) {
this.conditionRpt = conditionRpt;
}
public VehicleHistory count(Integer count) {
this.count = count;
return this;
}
/**
* the number of historical activity records
* @return count
**/
@ApiModelProperty(value = "the number of historical activity records")
public Integer getCount() {
return count;
}
public void setCount(Integer count) {
this.count = count;
}
public VehicleHistory estimatedAverageMiles(Integer estimatedAverageMiles) {
this.estimatedAverageMiles = estimatedAverageMiles;
return this;
}
/**
* the estimated average miles
* @return estimatedAverageMiles
**/
@ApiModelProperty(value = "the estimated average miles")
public Integer getEstimatedAverageMiles() {
return estimatedAverageMiles;
}
public void setEstimatedAverageMiles(Integer estimatedAverageMiles) {
this.estimatedAverageMiles = estimatedAverageMiles;
}
public VehicleHistory historyRecords(java.util.List historyRecords) {
this.historyRecords = historyRecords;
return this;
}
public VehicleHistory addHistoryRecordsItem(HistoryRecord historyRecordsItem) {
if (this.historyRecords == null) {
this.historyRecords = new java.util.ArrayList<>();
}
this.historyRecords.add(historyRecordsItem);
return this;
}
/**
* the list of historical activity for the vehicle
* @return historyRecords
**/
@ApiModelProperty(value = "the list of historical activity for the vehicle")
public java.util.List getHistoryRecords() {
return historyRecords;
}
public void setHistoryRecords(java.util.List historyRecords) {
this.historyRecords = historyRecords;
}
public VehicleHistory lastOdometer(Integer lastOdometer) {
this.lastOdometer = lastOdometer;
return this;
}
/**
* the last odometer reading
* @return lastOdometer
**/
@ApiModelProperty(value = "the last odometer reading")
public Integer getLastOdometer() {
return lastOdometer;
}
public void setLastOdometer(Integer lastOdometer) {
this.lastOdometer = lastOdometer;
}
public VehicleHistory mixedOdometer(Boolean mixedOdometer) {
this.mixedOdometer = mixedOdometer;
return this;
}
/**
* indicates a mixed odometer
* @return mixedOdometer
**/
@ApiModelProperty(value = "indicates a mixed odometer")
public Boolean isMixedOdometer() {
return mixedOdometer;
}
public void setMixedOdometer(Boolean mixedOdometer) {
this.mixedOdometer = mixedOdometer;
}
public VehicleHistory odometer(Boolean odometer) {
this.odometer = odometer;
return this;
}
/**
* indicates if an odometer reading exists
* @return odometer
**/
@ApiModelProperty(value = "indicates if an odometer reading exists")
public Boolean isOdometer() {
return odometer;
}
public void setOdometer(Boolean odometer) {
this.odometer = odometer;
}
public VehicleHistory recallCount(Integer recallCount) {
this.recallCount = recallCount;
return this;
}
/**
* the number of recalls
* @return recallCount
**/
@ApiModelProperty(value = "the number of recalls")
public Integer getRecallCount() {
return recallCount;
}
public void setRecallCount(Integer recallCount) {
this.recallCount = recallCount;
}
public VehicleHistory recallDataAvailable(Boolean recallDataAvailable) {
this.recallDataAvailable = recallDataAvailable;
return this;
}
/**
* indicates if recall data is available
* @return recallDataAvailable
**/
@ApiModelProperty(value = "indicates if recall data is available")
public Boolean isRecallDataAvailable() {
return recallDataAvailable;
}
public void setRecallDataAvailable(Boolean recallDataAvailable) {
this.recallDataAvailable = recallDataAvailable;
}
public VehicleHistory rollback(Boolean rollback) {
this.rollback = rollback;
return this;
}
/**
* indicates if the vehicle's history determines that the odometer has been tampered with
* @return rollback
**/
@ApiModelProperty(value = "indicates if the vehicle's history determines that the odometer has been tampered with")
public Boolean isRollback() {
return rollback;
}
public void setRollback(Boolean rollback) {
this.rollback = rollback;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
VehicleHistory vehicleHistory = (VehicleHistory) o;
return Objects.equals(this.accidentCount, vehicleHistory.accidentCount) &&
Objects.equals(this.assured, vehicleHistory.assured) &&
Objects.equals(this.bbp, vehicleHistory.bbp) &&
Objects.equals(this.conditionRpt, vehicleHistory.conditionRpt) &&
Objects.equals(this.count, vehicleHistory.count) &&
Objects.equals(this.estimatedAverageMiles, vehicleHistory.estimatedAverageMiles) &&
Objects.equals(this.historyRecords, vehicleHistory.historyRecords) &&
Objects.equals(this.lastOdometer, vehicleHistory.lastOdometer) &&
Objects.equals(this.mixedOdometer, vehicleHistory.mixedOdometer) &&
Objects.equals(this.odometer, vehicleHistory.odometer) &&
Objects.equals(this.recallCount, vehicleHistory.recallCount) &&
Objects.equals(this.recallDataAvailable, vehicleHistory.recallDataAvailable) &&
Objects.equals(this.rollback, vehicleHistory.rollback);
}
@Override
public int hashCode() {
return Objects.hash(accidentCount, assured, bbp, conditionRpt, count, estimatedAverageMiles, historyRecords, lastOdometer, mixedOdometer, odometer, recallCount, recallDataAvailable, rollback);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class VehicleHistory {\n");
sb.append(" accidentCount: ").append(toIndentedString(accidentCount)).append("\n");
sb.append(" assured: ").append(toIndentedString(assured)).append("\n");
sb.append(" bbp: ").append(toIndentedString(bbp)).append("\n");
sb.append(" conditionRpt: ").append(toIndentedString(conditionRpt)).append("\n");
sb.append(" count: ").append(toIndentedString(count)).append("\n");
sb.append(" estimatedAverageMiles: ").append(toIndentedString(estimatedAverageMiles)).append("\n");
sb.append(" historyRecords: ").append(toIndentedString(historyRecords)).append("\n");
sb.append(" lastOdometer: ").append(toIndentedString(lastOdometer)).append("\n");
sb.append(" mixedOdometer: ").append(toIndentedString(mixedOdometer)).append("\n");
sb.append(" odometer: ").append(toIndentedString(odometer)).append("\n");
sb.append(" recallCount: ").append(toIndentedString(recallCount)).append("\n");
sb.append(" recallDataAvailable: ").append(toIndentedString(recallDataAvailable)).append("\n");
sb.append(" rollback: ").append(toIndentedString(rollback)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy