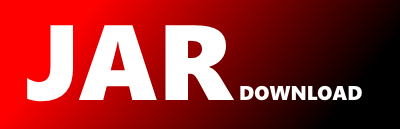
com.aventstack.extentreports.cucumber.adapter.ExtentCucumberAdapter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of extentreports-cucumber2-adapter Show documentation
Show all versions of extentreports-cucumber2-adapter Show documentation
Cucumber-JVM 2 adapter for Extent Framework
The newest version!
package com.aventstack.extentreports.cucumber.adapter;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.aventstack.extentreports.ExtentTest;
import com.aventstack.extentreports.GherkinKeyword;
import com.aventstack.extentreports.markuputils.MarkupHelper;
import com.aventstack.extentreports.service.ExtentService;
import cucumber.api.Result;
import cucumber.api.TestCase;
import cucumber.api.TestStep;
import cucumber.api.event.EmbedEvent;
import cucumber.api.event.EventHandler;
import cucumber.api.event.EventPublisher;
import cucumber.api.event.TestCaseStarted;
import cucumber.api.event.TestRunFinished;
import cucumber.api.event.TestSourceRead;
import cucumber.api.event.TestStepFinished;
import cucumber.api.event.TestStepStarted;
import cucumber.api.event.WriteEvent;
import cucumber.api.formatter.Formatter;
import gherkin.ast.Background;
import gherkin.ast.DataTable;
import gherkin.ast.DocString;
import gherkin.ast.Examples;
import gherkin.ast.Feature;
import gherkin.ast.Node;
import gherkin.ast.ScenarioDefinition;
import gherkin.ast.ScenarioOutline;
import gherkin.ast.Step;
import gherkin.ast.TableCell;
import gherkin.ast.TableRow;
import gherkin.ast.Tag;
import gherkin.pickles.Argument;
import gherkin.pickles.PickleCell;
import gherkin.pickles.PickleRow;
import gherkin.pickles.PickleString;
import gherkin.pickles.PickleTable;
/**
* A port of Cucumber-JVM (MIT licensed) HtmlFormatter for Extent Framework
* Original source: https://github.com/cucumber/cucumber-jvm/blob/master/core/src/main/java/cucumber/runtime/formatter/HTMLFormatter.java
*
*/
public class ExtentCucumberAdapter
implements Formatter {
private static ThreadLocal featureTestThreadLocal = new InheritableThreadLocal<>();
private static ThreadLocal scenarioOutlineThreadLocal = new InheritableThreadLocal<>();
private static ThreadLocal scenarioThreadLocal = new InheritableThreadLocal<>();
private static ThreadLocal stepTestThreadLocal = new InheritableThreadLocal<>();
private final TestSourcesModel testSources = new TestSourcesModel();
private String currentFeatureFile;
private Map currentTestCaseMap;
private ScenarioOutline currentScenarioOutline;
private Examples currentExamples;
private EventHandler testSourceReadHandler = new EventHandler() {
@Override
public void receive(TestSourceRead event) {
handleTestSourceRead(event);
}
};
private EventHandler caseStartedHandler= new EventHandler() {
@Override
public void receive(TestCaseStarted event) {
handleTestCaseStarted(event);
}
};
private EventHandler stepStartedHandler = new EventHandler() {
@Override
public void receive(TestStepStarted event) {
handleTestStepStarted(event);
}
};
private EventHandler stepFinishedHandler = new EventHandler() {
@Override
public void receive(TestStepFinished event) {
handleTestStepFinished(event);
}
};
private EventHandler embedEventhandler = new EventHandler() {
@Override
public void receive(EmbedEvent event) {
handleEmbed(event);
}
};
private EventHandler writeEventhandler = new EventHandler() {
@Override
public void receive(WriteEvent event) {
handleWrite(event);
}
};
private EventHandler runFinishedHandler = new EventHandler() {
@Override
public void receive(TestRunFinished event) {
finishReport();
}
};
public ExtentCucumberAdapter(String s) { }
@Override
public void setEventPublisher(EventPublisher publisher) {
publisher.registerHandlerFor(TestSourceRead.class, testSourceReadHandler);
publisher.registerHandlerFor(TestCaseStarted.class, caseStartedHandler);
publisher.registerHandlerFor(TestStepStarted.class, stepStartedHandler);
publisher.registerHandlerFor(TestStepFinished.class, stepFinishedHandler);
publisher.registerHandlerFor(EmbedEvent.class, embedEventhandler);
publisher.registerHandlerFor(WriteEvent.class, writeEventhandler);
publisher.registerHandlerFor(TestRunFinished.class, runFinishedHandler);
}
private void handleTestSourceRead(TestSourceRead event) {
testSources.addTestSourceReadEvent(event.uri, event);
}
private void handleTestCaseStarted(TestCaseStarted event) {
handleStartOfFeature(event.testCase);
handleScenarioOutline(event.testCase);
createTestCase(event.testCase);
if (testSources.hasBackground(currentFeatureFile, event.testCase.getLine())) {
createBackground(event.testCase);
}
}
private void handleTestStepStarted(TestStepStarted event) {
if (!event.testStep.isHook()) {
if (isFirstStepAfterBackground(event.testStep)) {
jsFunctionCall("scenario", currentTestCaseMap);
currentTestCaseMap = null;
}
createTestStep(event.testStep);
}
}
private void handleTestStepFinished(TestStepFinished event) {
if (!event.testStep.isHook()) {
createMatchMap(event.testStep, event.result);
createResultMap(event.result);
} else {
createResultMap(event.result);
}
}
private void handleEmbed(EmbedEvent event) {
/*String mimeType = event.mimeType;
if(mimeType.startsWith("text/")) {
// just pass straight to the formatter to output in the html
jsFunctionCall("embedding", mimeType, new String(event.data));
} else {
// Creating a file instead of using data urls to not clutter the js file
String extension = MIME_TYPES_EXTENSIONS.get(mimeType);
if (extension != null) {
StringBuilder fileName = new StringBuilder("embedded").append(embeddedIndex++).append(".").append(extension);
writeBytesToURL(event.data, toUrl(fileName.toString()));
jsFunctionCall("embedding", mimeType, fileName);
}
}*/
}
private void handleWrite(WriteEvent event) { }
private void finishReport() {
ExtentService.getInstance().flush();
}
private void handleStartOfFeature(TestCase testCase) {
if (currentFeatureFile == null || !currentFeatureFile.equals(testCase.getUri())) {
currentFeatureFile = testCase.getUri();
createFeature(testCase);
}
}
private Map createFeature(TestCase testCase) {
Map featureMap = new HashMap();
Feature feature = testSources.getFeature(testCase.getUri());
if (feature != null) {
ExtentTest t = ExtentService.getInstance()
.createTest(com.aventstack.extentreports.gherkin.model.Feature.class, feature.getName(), feature.getDescription());
featureTestThreadLocal.set(t);
List tagList = createTagsList(feature.getTags());
tagList.forEach(featureTestThreadLocal.get()::assignCategory);
}
return featureMap;
}
private List createTagsList(List tags) {
List tagList = new ArrayList<>();
for (Tag tag : tags) {
tagList.add(tag.getName());
}
return tagList;
}
private void handleScenarioOutline(TestCase testCase) {
TestSourcesModel.AstNode astNode = testSources.getAstNode(currentFeatureFile, testCase.getLine());
if (TestSourcesModel.isScenarioOutlineScenario(astNode)) {
ScenarioOutline scenarioOutline = (ScenarioOutline)TestSourcesModel.getScenarioDefinition(astNode);
if (currentScenarioOutline == null || !currentScenarioOutline.equals(scenarioOutline)) {
currentScenarioOutline = scenarioOutline;
createScenarioOutline(currentScenarioOutline);
addOutlineStepsToReport(scenarioOutline);
}
Examples examples = (Examples)astNode.parent.node;
if (currentExamples == null || !currentExamples.equals(examples)) {
currentExamples = examples;
createExamples(currentExamples);
}
} else {
scenarioOutlineThreadLocal.set(null);
currentScenarioOutline = null;
currentExamples = null;
}
}
private void createScenarioOutline(ScenarioOutline scenarioOutline) {
if (scenarioOutlineThreadLocal.get() == null) {
ExtentTest t = featureTestThreadLocal.get()
.createNode(com.aventstack.extentreports.gherkin.model.ScenarioOutline.class, scenarioOutline.getName(), scenarioOutline.getDescription());
scenarioOutlineThreadLocal.set(t);
List tags = createTagsList(scenarioOutline.getTags());
tags.forEach(scenarioOutlineThreadLocal.get()::assignCategory);
}
}
private void addOutlineStepsToReport(ScenarioOutline scenarioOutline) {
for (Step step : scenarioOutline.getSteps()) {
if (step.getArgument() != null) {
Node argument = step.getArgument();
if (argument instanceof DocString) {
createDocStringMap((DocString)argument);
} else if (argument instanceof DataTable) {
createDataTableList((DataTable)argument);
}
}
}
}
private Map createDocStringMap(DocString docString) {
Map docStringMap = new HashMap();
docStringMap.put("value", docString.getContent());
return docStringMap;
}
private List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy