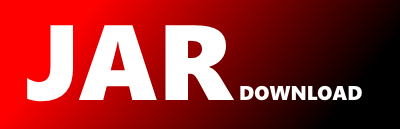
com.aventstack.extentreports.ExtentReports Maven / Gradle / Ivy
package com.aventstack.extentreports;
import java.io.File;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.util.Arrays;
import java.util.List;
import com.aventstack.extentreports.gherkin.GherkinDialectManager;
import com.aventstack.extentreports.gherkin.model.IGherkinFormatterModel;
import com.aventstack.extentreports.model.Media;
import com.aventstack.extentreports.model.ReportStats;
import com.aventstack.extentreports.model.SystemEnvInfo;
import com.aventstack.extentreports.model.service.TestService;
import com.aventstack.extentreports.observer.ExtentObserver;
/**
*
* The ExtentReports report client for starting reporters and building reports.
* For most applications, you should have one ExtentReports instance for the
* entire JVM.
*
*
*
* ExtentReports itself does not build any reports, but allows reporters to
* access information, which in turn build the said reports. An example of
* building an HTML report and adding information to ExtentX:
*
*
*
* ExtentHtmlReporter html = new ExtentHtmlReporter("Extent.html");
* ExtentXReporter extentx = new ExtentXReporter("localhost");
*
* ExtentReports extent = new ExtentReports();
* extent.attachReporter(html, extentx);
*
* extent.createTest("TestName").pass("Test Passed");
*
* extent.flush();
*
*
*
* A few notes:
*
*
*
* - It is mandatory to call the
flush
method to ensure
* information is written to the started reporters.
* - You can create standard and BDD-style tests using the
*
createTest
method
*
*
* @see ExtentTest
* @see GherkinKeyword
* @see IGherkinFormatterModel
* @see Status
*/
public class ExtentReports extends AbstractProcessor implements Writable, AnalysisTypeConfigurable {
/**
* Attach a {@link ExtentObserver} reporter, allowing it to access all
* started tests, nodes and logs
*
* @param observer
* {@link ExtentObserver} reporter
*/
@SuppressWarnings("rawtypes")
public void attachReporter(ExtentObserver... observer) {
attachReporter(Arrays.asList(observer));
}
/**
* Creates a BDD-style test with description representing one of the
* {@link IGherkinFormatterModel} classes such as:
*
*
* - {@link com.aventstack.extentreports.gherkin.model.Feature}
* - {@link com.aventstack.extentreports.gherkin.model.Background}
* - {@link com.aventstack.extentreports.gherkin.model.Scenario}
* - {@link com.aventstack.extentreports.gherkin.model.Given}
* - {@link com.aventstack.extentreports.gherkin.model.When}
* - {@link com.aventstack.extentreports.gherkin.model.Then}
* - {@link com.aventstack.extentreports.gherkin.model.And}
* - {@link com.aventstack.extentreports.gherkin.model.But}
*
*
*
* Example:
*
*
*
* extent.createTest(Feature.class, "feature", "description");
* extent.createTest(Scenario.class, "scenario", "description");
* extent.createTest(Given.class, "given", "description");
*
*
* @param type
* A {@link IGherkinFormatterModel} type
* @param name
* Name of test
* @param description
* A short description of the test
*
* @return {@link ExtentTest} object
*/
public ExtentTest createTest(Class extends IGherkinFormatterModel> type, String name,
String description) {
ExtentTest t = new ExtentTest(this, type, name, description);
onTestCreated(t.getModel());
return t;
}
/**
* Creates a BDD-style test representing one of the
* {@link IGherkinFormatterModel} classes such as:
*
*
* - {@link com.aventstack.extentreports.gherkin.model.Feature}
* - {@link com.aventstack.extentreports.gherkin.model.Background}
* - {@link com.aventstack.extentreports.gherkin.model.Scenario}
* - {@link com.aventstack.extentreports.gherkin.model.Given}
* - {@link com.aventstack.extentreports.gherkin.model.When}
* - {@link com.aventstack.extentreports.gherkin.model.Then}
* - {@link com.aventstack.extentreports.gherkin.model.And}
* - {@link com.aventstack.extentreports.gherkin.model.But}
*
*
*
* Example:
*
*
*
* extent.createTest(Feature.class, "feature");
* extent.createTest(Scenario.class, "scenario");
* extent.createTest(Given.class, "given");
*
*
* @param type
* A {@link IGherkinFormatterModel} type
* @param name
* Name of test
*
* @return {@link ExtentTest} object
*/
public ExtentTest createTest(Class extends IGherkinFormatterModel> type, String name) {
return createTest(type, name, null);
}
/**
* Creates a BDD-style test with description using name of the Gherkin model
* such as:
*
*
* - {@link com.aventstack.extentreports.gherkin.model.Feature}
* - {@link com.aventstack.extentreports.gherkin.model.Background}
* - {@link com.aventstack.extentreports.gherkin.model.Scenario}
* - {@link com.aventstack.extentreports.gherkin.model.Given}
* - {@link com.aventstack.extentreports.gherkin.model.When}
* - {@link com.aventstack.extentreports.gherkin.model.Then}
* - {@link com.aventstack.extentreports.gherkin.model.And}
* - {@link com.aventstack.extentreports.gherkin.model.But}
*
*
*
* Example:
*
*
*
* extent.createTest(new GherkinKeyword("Feature"), "feature", "description");
* extent.createTest(new GherkinKeyword("Scenario"), "scenario", "description");
* extent.createTest(new GherkinKeyword("Given"), "given", "description");
*
*
* @param gherkinKeyword
* Name of the {@link GherkinKeyword}
* @param name
* Name of test
* @param description
* A short description of the test
*
* @return {@link ExtentTest} object
*/
public ExtentTest createTest(GherkinKeyword gherkinKeyword, String name, String description) {
Class extends IGherkinFormatterModel> clazz = gherkinKeyword.getKeyword().getClass();
return createTest(clazz, name, description);
}
/**
* Creates a BDD-style test using name of the Gherkin model such as:
*
*
* - {@link com.aventstack.extentreports.gherkin.model.Feature}
* - {@link com.aventstack.extentreports.gherkin.model.Background}
* - {@link com.aventstack.extentreports.gherkin.model.Scenario}
* - {@link com.aventstack.extentreports.gherkin.model.Given}
* - {@link com.aventstack.extentreports.gherkin.model.When}
* - {@link com.aventstack.extentreports.gherkin.model.Then}
* - {@link com.aventstack.extentreports.gherkin.model.And}
* - {@link com.aventstack.extentreports.gherkin.model.But}
*
*
*
* Example:
*
*
*
* extent.createTest(new GherkinKeyword("Feature"), "feature");
* extent.createTest(new GherkinKeyword("Scenario"), "scenario");
* extent.createTest(new GherkinKeyword("Given"), "given");
*
*
* @param gherkinKeyword
* Name of the {@link GherkinKeyword}
* @param testName
* Name of test
*
* @return {@link ExtentTest} object
*/
public ExtentTest createTest(GherkinKeyword gherkinKeyword, String testName) {
return createTest(gherkinKeyword, testName, null);
}
/**
* Creates a test with description
*
* @param name
* Name of test
* @param description
* A short test description
*
* @return {@link ExtentTest} object
*/
public ExtentTest createTest(String name, String description) {
ExtentTest t = new ExtentTest(this, name, description);
onTestCreated(t.getModel());
return t;
}
/**
* Creates a test
*
* @param name
* Name of test
*
* @return {@link ExtentTest} object
*/
public ExtentTest createTest(String name) {
return createTest(name, null);
}
/**
* Removes a test
*
* @param test
* {@link ExtentTest} object
*/
public void removeTest(ExtentTest test) {
onTestRemoved(test.getModel());
}
/**
* Removes a test by name
*
* @param name
* The test name
*/
public void removeTest(String name) {
TestService.findTest(getReport().getTestList(), name)
.ifPresent(this::onTestRemoved);
}
/**
* Writes test information from the started reporters to their output view
*
*
* - extent-html-formatter: flush output to HTML file
* - extent-klov-reporter: updates MongoDB collections
* - extent-email-formatter (pro-only): creates or appends to an HTML
* file
* - ConsoleLogger: no action taken
*
*/
@Override
public void flush() {
onFlush();
}
/**
* Adds any applicable system information to all started reporters
*
*
* Example:
*
*
*
* extent.setSystemInfo("HostName", "AventStack");
*
*
* @param k
* Name of system variable
* @param v
* Value of system variable
*/
public void setSystemInfo(String k, String v) {
onSystemInfoAdded(new SystemEnvInfo(k, v));
}
/**
* Adds logs from test framework tools to the test-runner logs view (if
* available in the reporter)
*
*
* TestNG usage example:
*
*
*
* extent.setTestRunnerOutput(Reporter.getOutput());
*
*
* @param log
* Log string from the test runner frameworks such as TestNG or
* JUnit
*/
public void addTestRunnerOutput(List log) {
log.forEach(this::addTestRunnerOutput);
}
/**
* Adds logs from test framework tools to the test-runner logs view (if
* available in the reporter)
*
*
* TestNG usage example:
*
*
*
* for (String s : Reporter.getOutput()) {
* extent.setTestRunnerOutput(s);
* }
*
*
* @param log
* Log string from the test runner frameworks such as TestNG or
* JUnit
*/
public void addTestRunnerOutput(String log) {
onReportLogAdded(log);
}
/**
* Tries to resolve a {@link Media} location if the supplied path is not
* found using supplied locations. This can resolve cases where the default
* path was supplied to be relative for a FileReporter. If the absolute path
* is not determined, the supplied will be used.
*
* @param path
* Dirs used to create absolute path of the {@link Media} object
*
* @return {@link ExtentReports}
*/
public ExtentReports tryResolveMediaPath(String[] path) {
setMediaResolverPath(path);
return this;
}
/**
* Creates the internal models by consuming a JSON archive from a previous
* run session. This provides the same functionality as available in earlier
* versions via appendExisting
, with the exception of being
* accessible by all reporters instead of just one.
*
* @param jsonFile
* The JSON archive file
* @throws IOException
* Exception thrown if the jsonFile is not found
*/
public void createDomainFromJsonArchive(File jsonFile) throws IOException {
convertRawEntities(this, jsonFile);
}
/**
* Creates the internal models by consuming a JSON archive from a previous
* run session. This provides the same functionality as available in earlier
* versions via appendExisting
, with the exception of being
* accessible by all reporters instead of just one.
*
* @param jsonFilePath
* The JSON archive file
* @throws IOException
* Exception thrown if the jsonFilePath is not found
*/
public void createDomainFromJsonArchive(String jsonFilePath) throws IOException {
createDomainFromJsonArchive(new File(jsonFilePath));
}
/**
* Use this setting when building post-execution reports, such as from
* TestNG IReporter. This setting allows setting test with your own
* time-stamps. With this enabled, Extent does not use time-stamps for tests
* at the time they were created.
*
*
* If this setting is enabled and time-stamps are not specified explicitly,
* the time-stamps of test creation are used.
*
* @param useManualConfig
* Set to true if building reports at the end of execution with
* manual configuration
*/
public void setReportUsesManualConfiguration(boolean useManualConfig) {
setUsingNaturalConf(!useManualConfig);
}
/**
* Type of AnalysisStrategy for the reporter. Not all reporters support this
* setting.
*
*
* There are 2 types of strategies available:
*
*
* - TEST: Shows analysis at the test and step level
* - SUITE: Shows analysis at the suite, test and step level
*
*
* @param strategy
* {@link AnalysisStrategy} determines the type of analysis
* (dashboard) created for the reporter. Not all reporters will
* support this setting.
*/
@Override
public void setAnalysisStrategy(AnalysisStrategy strategy) {
getReport().getStats().setAnalysisStrategy(strategy);
}
/**
* Allows setting the target language for Gherkin keywords.
*
*
* Default setting is "en"
*
* @param language
* A valid dialect from gherkin-languages.json
*
* @throws UnsupportedEncodingException
* Thrown if the language is one of the supported language from
* gherkin-languages.json
*/
public void setGherkinDialect(String language) throws UnsupportedEncodingException {
GherkinDialectManager.setLanguage(language);
}
/**
* Returns an instance of {@link ReportStats} with counts of tests executed
* by their status (pass, fail, skip etc)
*
* @return an instance of {@link ReportStats}
*/
public ReportStats getStats() {
return getReport().getStats();
}
}