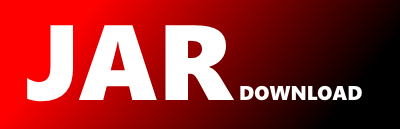
com.axibase.tsd.driver.jdbc.content.json.WarningSection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of atsd-jdbc Show documentation
Show all versions of atsd-jdbc Show documentation
JDBC driver for SQL API using
/*
* Copyright 2016 Axibase Corporation or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* https://www.axibase.com/atsd/axibase-apache-2.0.pdf
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.axibase.tsd.driver.jdbc.content.json;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.calcite.avatica.com.fasterxml.jackson.annotation.*;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"state",
"exception",
"message"
})
public class WarningSection implements AtsdExceptionRepresentation {
@JsonProperty("state")
private String state;
@JsonProperty("exception")
private List exception = new ArrayList<>();
@JsonProperty("message")
private String message;
@JsonIgnore
private final Map additionalProperties = new HashMap<>();
@Override
@JsonProperty("state")
public String getState() {
return state;
}
@JsonProperty("state")
public void setState(String state) {
this.state = state;
}
public WarningSection withState(String state) {
this.state = state;
return this;
}
@Override
@JsonProperty("exception")
public List getException() {
return exception;
}
@JsonProperty("exception")
public void setException(List exception) {
this.exception = exception;
}
public WarningSection withException(List exception) {
this.exception = exception;
return this;
}
@Override
@JsonProperty("message")
public String getMessage() {
return message;
}
@JsonProperty("message")
public void setMessage(String message) {
this.message = message;
}
public WarningSection withMessage(String message) {
this.message = message;
return this;
}
@JsonAnyGetter
public Map getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
public WarningSection withAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
return this;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((exception == null) ? 0 : exception.hashCode());
result = prime * result + ((message == null) ? 0 : message.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
WarningSection other = (WarningSection) obj;
if (exception == null) {
if (other.exception != null)
return false;
} else if (!exception.equals(other.exception))
return false;
if (message == null) {
if (other.message != null)
return false;
} else if (!message.equals(other.message))
return false;
return true;
}
@Override
public String toString() {
return "WarningSection [state=" + state + ", exception=" + exception + ", message=" + message + "]";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy