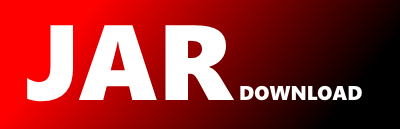
com.axway.ats.log.autodb.model.IDbReadAccess Maven / Gradle / Ivy
/*
* Copyright 2017 Axway Software
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.axway.ats.log.autodb.model;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.axway.ats.log.autodb.entities.Checkpoint;
import com.axway.ats.log.autodb.entities.CheckpointSummary;
import com.axway.ats.log.autodb.entities.LoadQueue;
import com.axway.ats.log.autodb.entities.Machine;
import com.axway.ats.log.autodb.entities.Message;
import com.axway.ats.log.autodb.entities.Run;
import com.axway.ats.log.autodb.entities.RunMetaInfo;
import com.axway.ats.log.autodb.entities.Scenario;
import com.axway.ats.log.autodb.entities.ScenarioMetaInfo;
import com.axway.ats.log.autodb.entities.Statistic;
import com.axway.ats.log.autodb.entities.StatisticDescription;
import com.axway.ats.log.autodb.entities.Suite;
import com.axway.ats.log.autodb.entities.Testcase;
import com.axway.ats.log.autodb.exceptions.DatabaseAccessException;
public interface IDbReadAccess {
public List getRuns(
int startRecord,
int recordsCount,
String whereClause,
String sortColumn,
boolean ascending,
int utcTimeOffset ) throws DatabaseAccessException;
public int getRunsCount(
String whereClause ) throws DatabaseAccessException;
public List getSuites(
int startRecord,
int recordsCount,
String whereClause,
String sortColumn,
boolean ascending,
int utcTimeOffset ) throws DatabaseAccessException;
public int getSuitesCount(
String whereClause ) throws DatabaseAccessException;
public List getScenarios(
int startRecord,
int recordsCount,
String whereClause,
String sortColumn,
boolean ascending,
int utcTimeOffset ) throws DatabaseAccessException;
public int getScenariosCount(
String whereClause ) throws DatabaseAccessException;
public List getTestcases(
int startRecord,
int recordsCount,
String whereClause,
String sortColumn,
boolean ascending,
int utcTimeOffset ) throws DatabaseAccessException;
public int getTestcasesCount(
String whereClause ) throws DatabaseAccessException;
public List getMachines() throws DatabaseAccessException;
public List getMessages(
int startRecord,
int recordsCount,
String whereClause,
String sortColumn,
boolean ascending,
int utcTimeOffset ) throws DatabaseAccessException;
public List getRunMessages(
int startRecord,
int recordsCount,
String whereClause,
String sortColumn,
boolean ascending,
int utcTimeOffset ) throws DatabaseAccessException;
public List getSuiteMessages(
int startRecord,
int recordsCount,
String whereClause,
String sortColumn,
boolean ascending,
int utcTimeOffset ) throws DatabaseAccessException;
public int getMessagesCount(
String whereClause ) throws DatabaseAccessException;
public int getRunMessagesCount(
String whereClause ) throws DatabaseAccessException;
public int getSuiteMessagesCount(
String whereClause ) throws DatabaseAccessException;
public List getSystemStatisticDescriptions(
float timeOffset,
String whereClause,
Map testcaseAliases,
int utcTimeOffset,
boolean dayLightSavingOn ) throws DatabaseAccessException;
public List getCheckpointStatisticDescriptions(
float timeOffset,
String whereClause,
Set expectedSingleActionUIDs,
int utcTimeOffset,
boolean dayLightSavingOn ) throws DatabaseAccessException;
public Map
getNumberOfCheckpointsPerQueue( String testcaseIds ) throws DatabaseAccessException;
public List getSystemStatistics(
float timeOffset,
String testcaseIds,
String machineIds,
String statsTypeIds,
int utcTimeOffset,
boolean dayLightSavingOn ) throws DatabaseAccessException;
public List getCheckpoints( String testcaseId,
int loadQueueId,
String checkpointName,
int utcTimeOffset,
boolean dayLightSavingOn ) throws DatabaseAccessException;
public List getCheckpointStatistics(
float timeOffset,
String testcaseIds,
String actionNames,
String actionParents,
Set expectedSingleActionUIDs,
Set expectedCombinedActionUIDs,
int utcTimeOffset,
boolean dayLightSavingOn ) throws DatabaseAccessException;
public List getLoadQueues(
String whereClause,
String sortColumn,
boolean ascending,
int utcTimeOffset ) throws DatabaseAccessException;
public List getCheckpointsSummary(
String whereClause,
String sortColumn,
boolean ascending ) throws DatabaseAccessException;
public List getRunMetaInfo( int runId ) throws DatabaseAccessException;
public List getScenarioMetaInfo( int scenarioId ) throws DatabaseAccessException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy