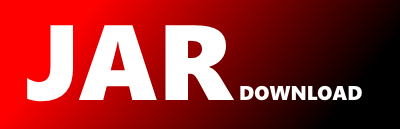
com.aylien.newsapi.models.Category Maven / Gradle / Ivy
/*
* AYLIEN News API
* The AYLIEN News API is the most powerful way of sourcing, searching and syndicating analyzed and enriched news content. It is accessed by sending HTTP requests to our server, which returns information to your client.
*
* The version of the OpenAPI document: 3.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.aylien.newsapi.models;
import java.util.Objects;
import java.util.Arrays;
import com.aylien.newsapi.models.CategoryLinks;
import com.aylien.newsapi.models.CategoryTaxonomy;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Category
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2020-01-09T15:04:35.817Z[Europe/Dublin]")
public class Category {
public static final String SERIALIZED_NAME_CONFIDENT = "confident";
@SerializedName(SERIALIZED_NAME_CONFIDENT)
private Boolean confident;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_LEVEL = "level";
@SerializedName(SERIALIZED_NAME_LEVEL)
private Integer level;
public static final String SERIALIZED_NAME_LINKS = "links";
@SerializedName(SERIALIZED_NAME_LINKS)
private CategoryLinks links;
public static final String SERIALIZED_NAME_SCORE = "score";
@SerializedName(SERIALIZED_NAME_SCORE)
private Double score;
public static final String SERIALIZED_NAME_TAXONOMY = "taxonomy";
@SerializedName(SERIALIZED_NAME_TAXONOMY)
private CategoryTaxonomy taxonomy;
public Category confident(Boolean confident) {
this.confident = confident;
return this;
}
/**
* It defines whether the extracted category is confident or not
* @return confident
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "It defines whether the extracted category is confident or not")
public Boolean getConfident() {
return confident;
}
public void setConfident(Boolean confident) {
this.confident = confident;
}
public Category id(String id) {
this.id = id;
return this;
}
/**
* The ID of the category
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The ID of the category")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public Category level(Integer level) {
this.level = level;
return this;
}
/**
* The level of the category
* @return level
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The level of the category")
public Integer getLevel() {
return level;
}
public void setLevel(Integer level) {
this.level = level;
}
public Category links(CategoryLinks links) {
this.links = links;
return this;
}
/**
* Get links
* @return links
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public CategoryLinks getLinks() {
return links;
}
public void setLinks(CategoryLinks links) {
this.links = links;
}
public Category score(Double score) {
this.score = score;
return this;
}
/**
* The score of the category
* @return score
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The score of the category")
public Double getScore() {
return score;
}
public void setScore(Double score) {
this.score = score;
}
public Category taxonomy(CategoryTaxonomy taxonomy) {
this.taxonomy = taxonomy;
return this;
}
/**
* Get taxonomy
* @return taxonomy
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public CategoryTaxonomy getTaxonomy() {
return taxonomy;
}
public void setTaxonomy(CategoryTaxonomy taxonomy) {
this.taxonomy = taxonomy;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Category category = (Category) o;
return Objects.equals(this.confident, category.confident) &&
Objects.equals(this.id, category.id) &&
Objects.equals(this.level, category.level) &&
Objects.equals(this.links, category.links) &&
Objects.equals(this.score, category.score) &&
Objects.equals(this.taxonomy, category.taxonomy);
}
@Override
public int hashCode() {
return Objects.hash(confident, id, level, links, score, taxonomy);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Category {\n");
sb.append(" confident: ").append(toIndentedString(confident)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" level: ").append(toIndentedString(level)).append("\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append(" score: ").append(toIndentedString(score)).append("\n");
sb.append(" taxonomy: ").append(toIndentedString(taxonomy)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy