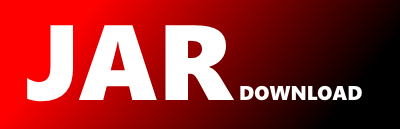
com.aylien.newsapi.models.Clusters Maven / Gradle / Ivy
/*
* AYLIEN News API
* The AYLIEN News API is the most powerful way of sourcing, searching and syndicating analyzed and enriched news content. It is accessed by sending HTTP requests to our server, which returns information to your client.
*
* The version of the OpenAPI document: 3.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.aylien.newsapi.models;
import java.util.Objects;
import java.util.Arrays;
import com.aylien.newsapi.models.Cluster;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* Clusters
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2020-01-09T15:04:35.817Z[Europe/Dublin]")
public class Clusters {
public static final String SERIALIZED_NAME_CLUSTER_COUNT = "cluster_count";
@SerializedName(SERIALIZED_NAME_CLUSTER_COUNT)
private Long clusterCount;
public static final String SERIALIZED_NAME_CLUSTERS = "clusters";
@SerializedName(SERIALIZED_NAME_CLUSTERS)
private List clusters = null;
public static final String SERIALIZED_NAME_NEXT_PAGE_CURSOR = "next_page_cursor";
@SerializedName(SERIALIZED_NAME_NEXT_PAGE_CURSOR)
private String nextPageCursor;
public Clusters clusterCount(Long clusterCount) {
this.clusterCount = clusterCount;
return this;
}
/**
* The total number of clusters
* @return clusterCount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total number of clusters")
public Long getClusterCount() {
return clusterCount;
}
public void setClusterCount(Long clusterCount) {
this.clusterCount = clusterCount;
}
public Clusters clusters(List clusters) {
this.clusters = clusters;
return this;
}
public Clusters addClustersItem(Cluster clustersItem) {
if (this.clusters == null) {
this.clusters = new ArrayList();
}
this.clusters.add(clustersItem);
return this;
}
/**
* An array of clusters
* @return clusters
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An array of clusters")
public List getClusters() {
return clusters;
}
public void setClusters(List clusters) {
this.clusters = clusters;
}
public Clusters nextPageCursor(String nextPageCursor) {
this.nextPageCursor = nextPageCursor;
return this;
}
/**
* The next page cursor
* @return nextPageCursor
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The next page cursor")
public String getNextPageCursor() {
return nextPageCursor;
}
public void setNextPageCursor(String nextPageCursor) {
this.nextPageCursor = nextPageCursor;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Clusters clusters = (Clusters) o;
return Objects.equals(this.clusterCount, clusters.clusterCount) &&
Objects.equals(this.clusters, clusters.clusters) &&
Objects.equals(this.nextPageCursor, clusters.nextPageCursor);
}
@Override
public int hashCode() {
return Objects.hash(clusterCount, clusters, nextPageCursor);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Clusters {\n");
sb.append(" clusterCount: ").append(toIndentedString(clusterCount)).append("\n");
sb.append(" clusters: ").append(toIndentedString(clusters)).append("\n");
sb.append(" nextPageCursor: ").append(toIndentedString(nextPageCursor)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy