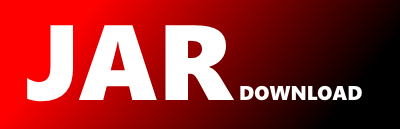
com.aylien.newsapi.models.Entity Maven / Gradle / Ivy
/*
* AYLIEN News API
* The AYLIEN News API is the most powerful way of sourcing, searching and syndicating analyzed and enriched news content. It is accessed by sending HTTP requests to our server, which returns information to your client.
*
* The version of the OpenAPI document: 3.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.aylien.newsapi.models;
import java.util.Objects;
import java.util.Arrays;
import com.aylien.newsapi.models.EntityLinks;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* Entity
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2020-01-09T15:04:35.817Z[Europe/Dublin]")
public class Entity {
public static final String SERIALIZED_NAME_INDICES = "indices";
@SerializedName(SERIALIZED_NAME_INDICES)
private List> indices = null;
public static final String SERIALIZED_NAME_LINKS = "links";
@SerializedName(SERIALIZED_NAME_LINKS)
private EntityLinks links;
public static final String SERIALIZED_NAME_SCORE = "score";
@SerializedName(SERIALIZED_NAME_SCORE)
private Double score;
public static final String SERIALIZED_NAME_TEXT = "text";
@SerializedName(SERIALIZED_NAME_TEXT)
private String text;
public static final String SERIALIZED_NAME_TYPES = "types";
@SerializedName(SERIALIZED_NAME_TYPES)
private List types = null;
public Entity indices(List> indices) {
this.indices = indices;
return this;
}
public Entity addIndicesItem(List indicesItem) {
if (this.indices == null) {
this.indices = new ArrayList>();
}
this.indices.add(indicesItem);
return this;
}
/**
* The indices of the entity text
* @return indices
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The indices of the entity text")
public List> getIndices() {
return indices;
}
public void setIndices(List> indices) {
this.indices = indices;
}
public Entity links(EntityLinks links) {
this.links = links;
return this;
}
/**
* Get links
* @return links
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public EntityLinks getLinks() {
return links;
}
public void setLinks(EntityLinks links) {
this.links = links;
}
public Entity score(Double score) {
this.score = score;
return this;
}
/**
* The entity score
* minimum: 0
* maximum: 1
* @return score
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The entity score")
public Double getScore() {
return score;
}
public void setScore(Double score) {
this.score = score;
}
public Entity text(String text) {
this.text = text;
return this;
}
/**
* The entity text
* @return text
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The entity text")
public String getText() {
return text;
}
public void setText(String text) {
this.text = text;
}
public Entity types(List types) {
this.types = types;
return this;
}
public Entity addTypesItem(String typesItem) {
if (this.types == null) {
this.types = new ArrayList();
}
this.types.add(typesItem);
return this;
}
/**
* An array of the dbpedia types
* @return types
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An array of the dbpedia types")
public List getTypes() {
return types;
}
public void setTypes(List types) {
this.types = types;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Entity entity = (Entity) o;
return Objects.equals(this.indices, entity.indices) &&
Objects.equals(this.links, entity.links) &&
Objects.equals(this.score, entity.score) &&
Objects.equals(this.text, entity.text) &&
Objects.equals(this.types, entity.types);
}
@Override
public int hashCode() {
return Objects.hash(indices, links, score, text, types);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Entity {\n");
sb.append(" indices: ").append(toIndentedString(indices)).append("\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append(" score: ").append(toIndentedString(score)).append("\n");
sb.append(" text: ").append(toIndentedString(text)).append("\n");
sb.append(" types: ").append(toIndentedString(types)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy