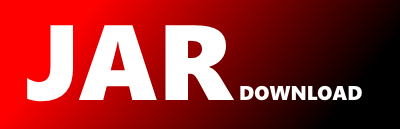
com.aylien.newsapi.models.Error Maven / Gradle / Ivy
/*
* AYLIEN News API
* The AYLIEN News API is the most powerful way of sourcing, searching and syndicating analyzed and enriched news content. It is accessed by sending HTTP requests to our server, which returns information to your client.
*
* The version of the OpenAPI document: 3.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.aylien.newsapi.models;
import java.util.Objects;
import java.util.Arrays;
import com.aylien.newsapi.models.ErrorLinks;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Error
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2020-01-09T15:04:35.817Z[Europe/Dublin]")
public class Error {
public static final String SERIALIZED_NAME_CODE = "code";
@SerializedName(SERIALIZED_NAME_CODE)
private String code;
public static final String SERIALIZED_NAME_DETAIL = "detail";
@SerializedName(SERIALIZED_NAME_DETAIL)
private String detail;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_LINKS = "links";
@SerializedName(SERIALIZED_NAME_LINKS)
private ErrorLinks links;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private String status;
public static final String SERIALIZED_NAME_TITLE = "title";
@SerializedName(SERIALIZED_NAME_TITLE)
private String title;
public Error code(String code) {
this.code = code;
return this;
}
/**
* Get code
* @return code
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getCode() {
return code;
}
public void setCode(String code) {
this.code = code;
}
public Error detail(String detail) {
this.detail = detail;
return this;
}
/**
* Get detail
* @return detail
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getDetail() {
return detail;
}
public void setDetail(String detail) {
this.detail = detail;
}
public Error id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public Error links(ErrorLinks links) {
this.links = links;
return this;
}
/**
* Get links
* @return links
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ErrorLinks getLinks() {
return links;
}
public void setLinks(ErrorLinks links) {
this.links = links;
}
public Error status(String status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public Error title(String title) {
this.title = title;
return this;
}
/**
* Get title
* @return title
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Error error = (Error) o;
return Objects.equals(this.code, error.code) &&
Objects.equals(this.detail, error.detail) &&
Objects.equals(this.id, error.id) &&
Objects.equals(this.links, error.links) &&
Objects.equals(this.status, error.status) &&
Objects.equals(this.title, error.title);
}
@Override
public int hashCode() {
return Objects.hash(code, detail, id, links, status, title);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Error {\n");
sb.append(" code: ").append(toIndentedString(code)).append("\n");
sb.append(" detail: ").append(toIndentedString(detail)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" title: ").append(toIndentedString(title)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy