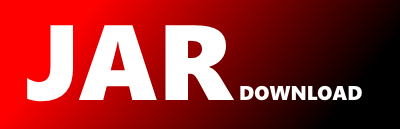
com.aylien.newsapi.models.Location Maven / Gradle / Ivy
/*
* AYLIEN News API
* The AYLIEN News API is the most powerful way of sourcing, searching and syndicating analyzed and enriched news content. It is accessed by sending HTTP requests to our server, which returns information to your client.
*
* The version of the OpenAPI document: 3.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.aylien.newsapi.models;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Location
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2020-01-09T15:04:35.817Z[Europe/Dublin]")
public class Location {
public static final String SERIALIZED_NAME_CITY = "city";
@SerializedName(SERIALIZED_NAME_CITY)
private String city;
public static final String SERIALIZED_NAME_COUNTRY = "country";
@SerializedName(SERIALIZED_NAME_COUNTRY)
private String country;
public static final String SERIALIZED_NAME_STATE = "state";
@SerializedName(SERIALIZED_NAME_STATE)
private String state;
public Location city(String city) {
this.city = city;
return this;
}
/**
* The city of the location
* @return city
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The city of the location")
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public Location country(String country) {
this.country = country;
return this;
}
/**
* The country code of the location. It supports [ISO 3166-1 alpha-2](https://en.wikipedia.org/wiki/ISO_3166-1_alpha-2) country codes.
* @return country
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The country code of the location. It supports [ISO 3166-1 alpha-2](https://en.wikipedia.org/wiki/ISO_3166-1_alpha-2) country codes. ")
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
public Location state(String state) {
this.state = state;
return this;
}
/**
* The state of the location
* @return state
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The state of the location")
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Location location = (Location) o;
return Objects.equals(this.city, location.city) &&
Objects.equals(this.country, location.country) &&
Objects.equals(this.state, location.state);
}
@Override
public int hashCode() {
return Objects.hash(city, country, state);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Location {\n");
sb.append(" city: ").append(toIndentedString(city)).append("\n");
sb.append(" country: ").append(toIndentedString(country)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy