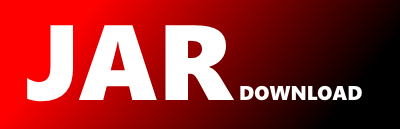
com.aylien.newsapi.models.Sentiments Maven / Gradle / Ivy
/*
* AYLIEN News API
* The AYLIEN News API is the most powerful way of sourcing, searching and syndicating analyzed and enriched news content. It is accessed by sending HTTP requests to our server, which returns information to your client.
*
* The version of the OpenAPI document: 3.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.aylien.newsapi.models;
import java.util.Objects;
import java.util.Arrays;
import com.aylien.newsapi.models.Sentiment;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Sentiments
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2020-01-09T15:04:35.817Z[Europe/Dublin]")
public class Sentiments {
public static final String SERIALIZED_NAME_BODY = "body";
@SerializedName(SERIALIZED_NAME_BODY)
private Sentiment body;
public static final String SERIALIZED_NAME_TITLE = "title";
@SerializedName(SERIALIZED_NAME_TITLE)
private Sentiment title;
public Sentiments body(Sentiment body) {
this.body = body;
return this;
}
/**
* Get body
* @return body
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Sentiment getBody() {
return body;
}
public void setBody(Sentiment body) {
this.body = body;
}
public Sentiments title(Sentiment title) {
this.title = title;
return this;
}
/**
* Get title
* @return title
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Sentiment getTitle() {
return title;
}
public void setTitle(Sentiment title) {
this.title = title;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Sentiments sentiments = (Sentiments) o;
return Objects.equals(this.body, sentiments.body) &&
Objects.equals(this.title, sentiments.title);
}
@Override
public int hashCode() {
return Objects.hash(body, title);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Sentiments {\n");
sb.append(" body: ").append(toIndentedString(body)).append("\n");
sb.append(" title: ").append(toIndentedString(title)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy