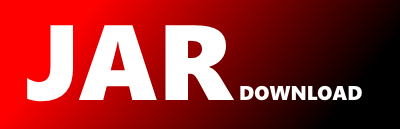
com.aylien.newsapi.models.TimeSeriesList Maven / Gradle / Ivy
/*
* AYLIEN News API
* The AYLIEN News API is the most powerful way of sourcing, searching and syndicating analyzed and enriched news content. It is accessed by sending HTTP requests to our server, which returns information to your client.
*
* The version of the OpenAPI document: 3.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.aylien.newsapi.models;
import java.util.Objects;
import java.util.Arrays;
import com.aylien.newsapi.models.TimeSeries;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.threeten.bp.OffsetDateTime;
/**
* TimeSeriesList
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2020-01-09T15:04:35.817Z[Europe/Dublin]")
public class TimeSeriesList {
public static final String SERIALIZED_NAME_PERIOD = "period";
@SerializedName(SERIALIZED_NAME_PERIOD)
private String period;
public static final String SERIALIZED_NAME_PUBLISHED_AT_END = "published_at.end";
@SerializedName(SERIALIZED_NAME_PUBLISHED_AT_END)
private OffsetDateTime publishedAtEnd;
public static final String SERIALIZED_NAME_PUBLISHED_AT_START = "published_at.start";
@SerializedName(SERIALIZED_NAME_PUBLISHED_AT_START)
private OffsetDateTime publishedAtStart;
public static final String SERIALIZED_NAME_TIME_SERIES = "time_series";
@SerializedName(SERIALIZED_NAME_TIME_SERIES)
private List timeSeries = null;
public TimeSeriesList period(String period) {
this.period = period;
return this;
}
/**
* The size of each date range expressed as an interval to be added to the lower bound.
* @return period
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The size of each date range expressed as an interval to be added to the lower bound. ")
public String getPeriod() {
return period;
}
public void setPeriod(String period) {
this.period = period;
}
public TimeSeriesList publishedAtEnd(OffsetDateTime publishedAtEnd) {
this.publishedAtEnd = publishedAtEnd;
return this;
}
/**
* The end published date of the time series
* @return publishedAtEnd
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The end published date of the time series")
public OffsetDateTime getPublishedAtEnd() {
return publishedAtEnd;
}
public void setPublishedAtEnd(OffsetDateTime publishedAtEnd) {
this.publishedAtEnd = publishedAtEnd;
}
public TimeSeriesList publishedAtStart(OffsetDateTime publishedAtStart) {
this.publishedAtStart = publishedAtStart;
return this;
}
/**
* The start published date of the time series
* @return publishedAtStart
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The start published date of the time series")
public OffsetDateTime getPublishedAtStart() {
return publishedAtStart;
}
public void setPublishedAtStart(OffsetDateTime publishedAtStart) {
this.publishedAtStart = publishedAtStart;
}
public TimeSeriesList timeSeries(List timeSeries) {
this.timeSeries = timeSeries;
return this;
}
public TimeSeriesList addTimeSeriesItem(TimeSeries timeSeriesItem) {
if (this.timeSeries == null) {
this.timeSeries = new ArrayList();
}
this.timeSeries.add(timeSeriesItem);
return this;
}
/**
* An array of time series
* @return timeSeries
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An array of time series")
public List getTimeSeries() {
return timeSeries;
}
public void setTimeSeries(List timeSeries) {
this.timeSeries = timeSeries;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TimeSeriesList timeSeriesList = (TimeSeriesList) o;
return Objects.equals(this.period, timeSeriesList.period) &&
Objects.equals(this.publishedAtEnd, timeSeriesList.publishedAtEnd) &&
Objects.equals(this.publishedAtStart, timeSeriesList.publishedAtStart) &&
Objects.equals(this.timeSeries, timeSeriesList.timeSeries);
}
@Override
public int hashCode() {
return Objects.hash(period, publishedAtEnd, publishedAtStart, timeSeries);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TimeSeriesList {\n");
sb.append(" period: ").append(toIndentedString(period)).append("\n");
sb.append(" publishedAtEnd: ").append(toIndentedString(publishedAtEnd)).append("\n");
sb.append(" publishedAtStart: ").append(toIndentedString(publishedAtStart)).append("\n");
sb.append(" timeSeries: ").append(toIndentedString(timeSeries)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy