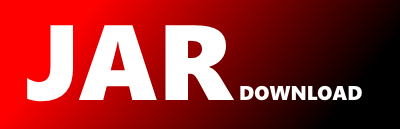
geotrellis.raster.io.geotiff.SinglebandGeoTiff.scala Maven / Gradle / Ivy
package geotrellis.raster.io.geotiff
import geotrellis.raster._
import geotrellis.raster.io.geotiff.reader.GeoTiffReader
import geotrellis.vector.Extent
import geotrellis.proj4.CRS
case class SinglebandGeoTiff(
val tile: Tile,
val extent: Extent,
val crs: CRS,
val tags: Tags,
val options: GeoTiffOptions
) extends GeoTiff[Tile] {
val cellType = tile.cellType
def mapTile(f: Tile => Tile): SinglebandGeoTiff =
SinglebandGeoTiff(f(tile), extent, crs, tags, options)
def imageData: GeoTiffImageData =
tile match {
case gtt: GeoTiffTile => gtt
case _ => tile.toGeoTiffTile(options)
}
}
object SinglebandGeoTiff {
def apply(
tile: Tile,
extent: Extent,
crs: CRS
): SinglebandGeoTiff =
new SinglebandGeoTiff(tile, extent, crs, Tags.empty, GeoTiffOptions.DEFAULT)
/** Read a single-band GeoTIFF file from a byte array.
* The GeoTIFF will be fully decompressed and held in memory.
*/
def apply(bytes: Array[Byte]): SinglebandGeoTiff =
GeoTiffReader.readSingleband(bytes)
/** Read a single-band GeoTIFF file from a byte array.
* If decompress = true, the GeoTIFF will be fully decompressed and held in memory.
*/
def apply(bytes: Array[Byte], decompress: Boolean): SinglebandGeoTiff =
GeoTiffReader.readSingleband(bytes, decompress)
/** Read a single-band GeoTIFF file from the file at the given path.
* The GeoTIFF will be fully decompressed and held in memory.
*/
def apply(path: String): SinglebandGeoTiff =
GeoTiffReader.readSingleband(path)
/** Read a single-band GeoTIFF file from the file at the given path.
* If decompress = true, the GeoTIFF will be fully decompressed and held in memory.
*/
def apply(path: String, decompress: Boolean): SinglebandGeoTiff =
GeoTiffReader.readSingleband(path, decompress)
/** Read a single-band GeoTIFF file from the file at a given path.
* The tile data will remain tiled/striped and compressed in the TIFF format.
*/
def compressed(path: String): SinglebandGeoTiff =
GeoTiffReader.readSingleband(path, false)
/** Read a single-band GeoTIFF file from a byte array.
* The tile data will remain tiled/striped and compressed in the TIFF format.
*/
def compressed(bytes: Array[Byte]): SinglebandGeoTiff =
GeoTiffReader.readSingleband(bytes, false)
implicit def singlebandGeoTiffToTile(sbg: SinglebandGeoTiff): Tile =
sbg.tile
implicit def singlebandGeoTiffToRaster(sbg: SinglebandGeoTiff): SinglebandRaster =
sbg.raster
implicit def singlebandGeoTiffToProjectedRaster(sbg: SinglebandGeoTiff): ProjectedRaster[Tile] =
sbg.projectedRaster
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy